C++ Data Types: A Comprehensive Guide for Beginners and Advanced Programmers
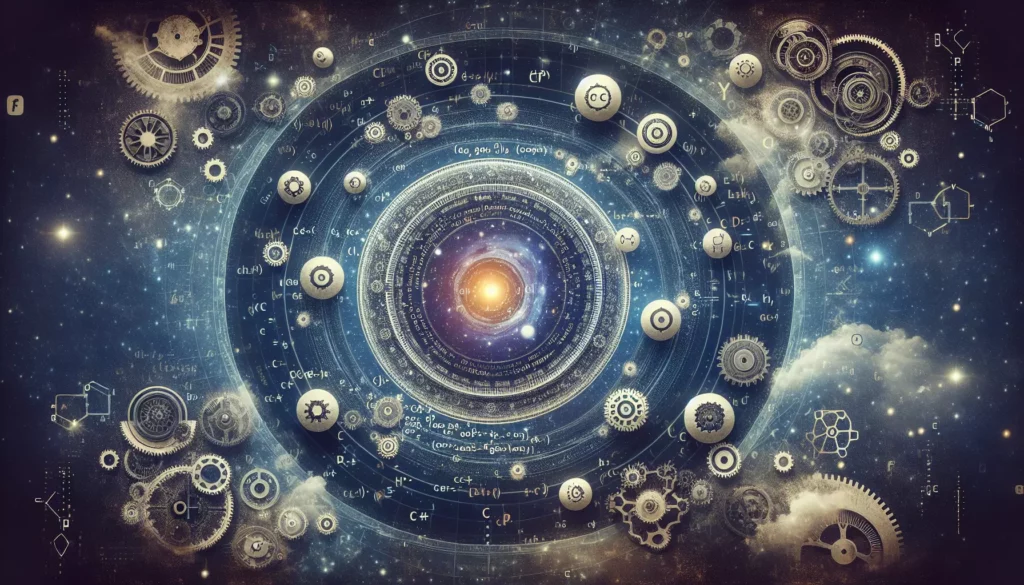
In the world of programming, understanding data types is crucial for writing efficient and error-free code. C++, a powerful and versatile language, offers a rich set of data types that allow developers to work with various kinds of information. Whether you’re a beginner just starting your coding journey or an experienced programmer looking to brush up on your C++ skills, this comprehensive guide will walk you through everything you need to know about C++ data types.
Table of Contents
- Introduction to C++ Data Types
- Fundamental Data Types
- Derived Data Types
- User-Defined Data Types
- Type Modifiers
- Type Conversion and Casting
- Best Practices for Using Data Types
- Common Pitfalls and How to Avoid Them
- Advanced Topics in C++ Data Types
- Conclusion
1. Introduction to C++ Data Types
Data types in C++ are used to define the type of data that a variable can hold. They play a crucial role in determining how much memory is allocated for a variable and what operations can be performed on it. C++ provides a wide range of data types to accommodate different kinds of data and programming needs.
Understanding data types is essential for several reasons:
- Memory management: Different data types occupy different amounts of memory.
- Type safety: Proper use of data types helps prevent errors and unexpected behavior in your programs.
- Performance optimization: Choosing the right data type can significantly impact your program’s efficiency.
- Code readability: Appropriate data types make your code more self-explanatory and easier to maintain.
2. Fundamental Data Types
C++ has several fundamental data types that serve as the building blocks for more complex types. These include:
Integer Types
- int: Used for whole numbers. Typically 4 bytes on most systems.
- short: A smaller integer type, usually 2 bytes.
- long: A larger integer type, at least 4 bytes.
- long long: An even larger integer type, at least 8 bytes.
Example:
int age = 25;
short small_number = 100;
long big_number = 1000000L;
long long very_big_number = 1000000000000LL;
Floating-Point Types
- float: Single-precision floating-point number. Typically 4 bytes.
- double: Double-precision floating-point number. Usually 8 bytes.
- long double: Extended-precision floating-point number. Can be 8, 12, or 16 bytes.
Example:
float pi = 3.14159f;
double precise_pi = 3.141592653589793;
long double very_precise_pi = 3.141592653589793238L;
Character Types
- char: Used to store a single character. Occupies 1 byte.
- wchar_t: Wide character type, used for storing Unicode characters.
Example:
char grade = 'A';
wchar_t wide_char = L'Ω';
Boolean Type
- bool: Used to represent true or false values. Typically 1 byte.
Example:
bool is_student = true;
bool has_passed = false;
Void Type
The void type represents the absence of a type. It’s commonly used as the return type for functions that don’t return a value.
Example:
void print_hello() {
std::cout << "Hello, World!" << std::endl;
}
3. Derived Data Types
Derived data types are built from the fundamental types. They include:
Arrays
Arrays are used to store multiple elements of the same type in contiguous memory locations.
Example:
int numbers[5] = {1, 2, 3, 4, 5};
char name[] = "John";
Pointers
Pointers store memory addresses of variables.
Example:
int x = 10;
int* ptr = &x;
References
References provide an alias for an existing variable.
Example:
int y = 20;
int& ref = y;
4. User-Defined Data Types
C++ allows programmers to create their own data types using the following constructs:
Structures (struct)
Structures group related data elements of different types under a single name.
Example:
struct Person {
std::string name;
int age;
float height;
};
Classes
Classes are the foundation of object-oriented programming in C++. They encapsulate data and functions that operate on that data.
Example:
class Rectangle {
private:
double length;
double width;
public:
void set_dimensions(double l, double w) {
length = l;
width = w;
}
double area() {
return length * width;
}
};
Enumerations (enum)
Enumerations define a set of named constants.
Example:
enum Color {
RED,
GREEN,
BLUE
};
Color my_color = RED;
Unions
Unions allow storing different data types in the same memory location.
Example:
union Data {
int i;
float f;
char str[20];
};
5. Type Modifiers
C++ provides type modifiers that can be used to alter the properties of fundamental data types:
Sign Modifiers
- signed: Used for numbers that can be positive or negative (default for most integer types).
- unsigned: Used for numbers that are always non-negative.
Example:
unsigned int positive_number = 100;
signed int number_with_sign = -50;
Size Modifiers
- short: Reduces the size of an integer type.
- long: Increases the size of an integer or double type.
Example:
short int small_integer = 10;
long int large_integer = 1000000L;
long double very_precise = 3.14159265358979323846L;
Type Qualifiers
- const: Declares that the variable’s value cannot be changed.
- volatile: Tells the compiler that the variable’s value may change at any time without any action being taken by the code.
Example:
const int MAX_STUDENTS = 100;
volatile int sensor_value;
6. Type Conversion and Casting
Type conversion is the process of converting data from one type to another. C++ supports both implicit and explicit type conversions.
Implicit Type Conversion
Also known as automatic type conversion, this happens when the compiler automatically converts one data type to another.
Example:
int x = 10;
double y = x; // Implicit conversion from int to double
Explicit Type Conversion (Casting)
This is when the programmer explicitly tells the compiler to convert a value from one type to another.
C++ provides several ways to perform explicit type conversion:
- C-style cast:
(type) expression
- Function-style cast:
type(expression)
- Static cast:
static_cast<type>(expression)
- Dynamic cast:
dynamic_cast<type>(expression)
- Const cast:
const_cast<type>(expression)
- Reinterpret cast:
reinterpret_cast<type>(expression)
Example:
double pi = 3.14159;
int rounded_pi = static_cast<int>(pi); // Explicit conversion from double to int
7. Best Practices for Using Data Types
To make the most of C++ data types and write efficient, maintainable code, consider the following best practices:
- Choose the right type for the job: Use the most appropriate data type for your needs. For example, use
bool
for flags instead ofint
. - Be mindful of integer overflow: When working with integers, be aware of the limits of each type to avoid overflow errors.
- Use
size_t
for sizes and indices: When dealing with container sizes or array indices, prefersize_t
overint
. - Prefer
double
overfloat
for most floating-point calculations:double
provides more precision and is often just as fast asfloat
on modern systems. - Use
const
when appropriate: Mark variables that shouldn’t change after initialization asconst
to prevent accidental modifications. - Be cautious with implicit conversions: Be aware of potential data loss or unexpected behavior when implicit conversions occur.
- Use modern C++ features: Leverage features like
auto
for type inference andnullptr
instead ofNULL
for null pointers.
8. Common Pitfalls and How to Avoid Them
When working with C++ data types, be aware of these common pitfalls:
Integer Overflow
Problem: When an integer exceeds its maximum value, it wraps around to its minimum value, leading to unexpected results.
Solution: Use larger integer types or check for potential overflow before performing operations.
// Potential overflow
int result = INT_MAX + 1; // Undefined behavior
// Better approach
if (a > INT_MAX - b) {
// Handle potential overflow
} else {
int result = a + b;
}
Floating-Point Precision Issues
Problem: Floating-point numbers can lead to rounding errors and imprecise comparisons.
Solution: Avoid direct equality comparisons for floating-point numbers. Instead, use an epsilon value for comparisons.
// Problematic
if (a == b) { // May not work as expected for floating-point values
// Better approach
const double epsilon = 1e-9;
if (std::abs(a - b) < epsilon) {
// Consider a and b equal
}
Uninitialized Variables
Problem: Using variables before they are initialized can lead to undefined behavior.
Solution: Always initialize variables when declaring them.
// Problematic
int x;
std::cout << x; // Undefined behavior
// Better approach
int x = 0;
std::cout << x;
Incorrect Pointer Usage
Problem: Dereferencing null or dangling pointers can cause crashes or undefined behavior.
Solution: Always check if a pointer is valid before dereferencing it. Use smart pointers when possible.
// Problematic
int* ptr = nullptr;
*ptr = 10; // Crash!
// Better approach
int* ptr = nullptr;
if (ptr != nullptr) {
*ptr = 10;
}
// Even better: use smart pointers
std::unique_ptr<int> smart_ptr = std::make_unique<int>(10);
9. Advanced Topics in C++ Data Types
As you become more proficient with C++ data types, you may encounter more advanced concepts:
Custom Literals
C++11 introduced user-defined literals, allowing you to create custom suffixes for literals.
constexpr long double operator"" _kg(long double x) {
return x * 1000; // Convert kg to g
}
auto weight = 5.0_kg; // weight is 5000 grams
Type Traits
The <type_traits>
header provides a set of type traits that allow you to query and modify types at compile-time.
#include <type_traits>
template <typename T>
void process(T value) {
if constexpr (std::is_integral_v<T>) {
// Handle integral types
} else if constexpr (std::is_floating_point_v<T>) {
// Handle floating-point types
} else {
// Handle other types
}
}
Concepts (C++20)
Concepts allow you to specify constraints on template parameters, making template code more readable and providing better error messages.
#include <concepts>
template <typename T>
concept Numeric = std::integral<T> || std::floating_point<T>;
template <Numeric T>
T add(T a, T b) {
return a + b;
}
Memory Alignment
Understanding memory alignment can be crucial for performance and when working with hardware interfaces.
// Specify alignment for a struct
struct alignas(16) AlignedStruct {
int x;
char y;
double z;
};
// Check alignment of a type
std::cout << alignof(AlignedStruct) << std::endl;
10. Conclusion
Mastering C++ data types is fundamental to becoming a proficient C++ programmer. From understanding the basic types to leveraging advanced features, a solid grasp of data types will help you write more efficient, safer, and more maintainable code.
As you continue your journey in C++ programming, remember that practice is key. Experiment with different data types, explore their limitations and capabilities, and always strive to use the most appropriate type for each situation. By doing so, you’ll not only improve your C++ skills but also develop a deeper understanding of how computers store and manipulate data.
Whether you’re preparing for technical interviews, working on personal projects, or developing large-scale applications, your knowledge of C++ data types will serve as a strong foundation for your programming endeavors. Keep learning, stay curious, and happy coding!