Mastering LeetCode with C: A Deep Dive into Recursion
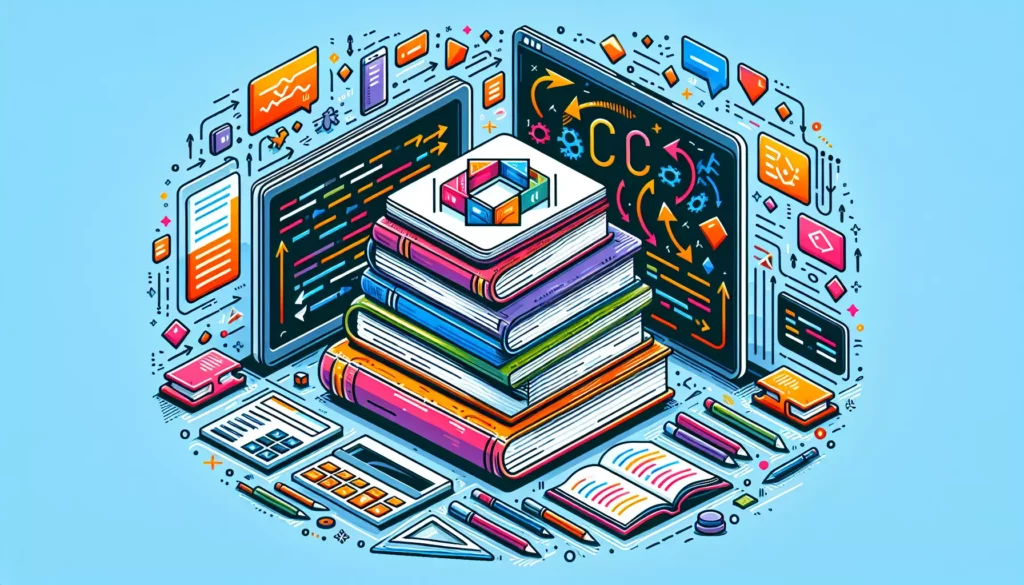
Welcome to AlgoCademy’s comprehensive guide on tackling LeetCode problems using recursion in C. As you embark on your journey to become a proficient programmer and ace those technical interviews, understanding recursion is crucial. This powerful technique is not only a favorite among interviewers but also a fundamental concept in computer science that can help you solve complex problems with elegant solutions.
Table of Contents
- Introduction to Recursion
- The Basics of Recursion in C
- Anatomy of a Recursive Function
- LeetCode Examples Using Recursion
- Tips for Solving Recursive Problems
- Common Pitfalls and How to Avoid Them
- Optimizing Recursive Solutions
- Interview Strategies for Recursive Problems
- Advanced Recursive Concepts
- Conclusion
1. Introduction to Recursion
Recursion is a programming technique where a function calls itself to solve a problem. It’s based on the principle of solving a complex problem by breaking it down into simpler sub-problems. In the context of LeetCode and coding interviews, recursion is often used to solve problems involving trees, graphs, and divide-and-conquer algorithms.
The power of recursion lies in its ability to express complex processes in a concise and often more intuitive manner compared to iterative approaches. However, it’s important to understand both its strengths and limitations to use it effectively.
2. The Basics of Recursion in C
In C, implementing recursion is straightforward. Here’s a simple example of a recursive function that calculates the factorial of a number:
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
}
return n * factorial(n - 1);
}
This function demonstrates the two essential components of any recursive solution:
- Base case: The condition that stops the recursion (when n is 0 or 1).
- Recursive case: The function calling itself with a modified argument (n – 1).
3. Anatomy of a Recursive Function
Every recursive function typically follows this structure:
return_type recursive_function(parameters) {
// Base case(s)
if (base_condition) {
return base_result;
}
// Recursive case(s)
// Process data
// Make recursive call(s)
return recursive_function(modified_parameters);
}
Understanding this structure is crucial for solving LeetCode problems efficiently. Let’s break it down:
- Base case(s): These are the simplest scenarios where the function can return a result without making any recursive calls. They prevent infinite recursion.
- Recursive case(s): Here, the function processes some data and then calls itself with modified parameters to solve a smaller subproblem.
4. LeetCode Examples Using Recursion
Let’s explore some popular LeetCode problems that can be solved using recursion in C:
Example 1: Reverse a Linked List (LeetCode #206)
struct ListNode* reverseList(struct ListNode* head) {
// Base case: if list is empty or has only one node
if (head == NULL || head->next == NULL) {
return head;
}
// Recursive case
struct ListNode* rest = reverseList(head->next);
head->next->next = head;
head->next = NULL;
return rest;
}
This solution recursively reverses the rest of the list and then adjusts the current node’s pointers.
Example 2: Binary Tree Inorder Traversal (LeetCode #94)
void inorderTraversal(struct TreeNode* root, int* result, int* returnSize) {
if (root == NULL) {
return;
}
inorderTraversal(root->left, result, returnSize);
result[(*returnSize)++] = root->val;
inorderTraversal(root->right, result, returnSize);
}
This function performs an inorder traversal of a binary tree recursively, adding nodes to the result array as it goes.
Example 3: Generate Parentheses (LeetCode #22)
void backtrack(int n, int open, int close, char* current, int index, char** result, int* returnSize) {
if (index == 2 * n) {
result[*returnSize] = strdup(current);
(*returnSize)++;
return;
}
if (open < n) {
current[index] = '(';
backtrack(n, open + 1, close, current, index + 1, result, returnSize);
}
if (close < open) {
current[index] = ')';
backtrack(n, open, close + 1, current, index + 1, result, returnSize);
}
}
This recursive backtracking solution generates all valid combinations of n pairs of parentheses.
5. Tips for Solving Recursive Problems
- Identify the base case(s): Always start by determining the simplest scenario(s) where the function should return without making a recursive call.
- Define the recursive case: Figure out how to break down the problem into smaller subproblems.
- Trust the recursion: Assume that your recursive calls will work correctly for smaller inputs.
- Ensure progress towards the base case: Make sure each recursive call brings you closer to the base case to avoid infinite recursion.
- Use helper functions: Sometimes, it’s useful to create a separate recursive helper function with additional parameters to track state.
6. Common Pitfalls and How to Avoid Them
When working with recursion, be aware of these common issues:
- Stack overflow: Excessive recursive calls can lead to stack overflow. To avoid this, consider tail recursion or iterative solutions for deep recursions.
- Infinite recursion: Always ensure your recursive calls progress towards the base case.
- Redundant computations: In some cases, recursive solutions may recalculate the same subproblems multiple times. Use memoization or dynamic programming to optimize these scenarios.
- Incorrect base cases: Ensure your base cases cover all possible scenarios to avoid unexpected behavior.
7. Optimizing Recursive Solutions
While recursion can lead to elegant solutions, it’s not always the most efficient approach. Here are some techniques to optimize recursive solutions:
Tail Recursion
Tail recursion is a special form of recursion where the recursive call is the last operation in the function. Many compilers can optimize tail-recursive functions to be as efficient as iterative solutions.
int factorial_tail(int n, int acc) {
if (n == 0 || n == 1) {
return acc;
}
return factorial_tail(n - 1, n * acc);
}
int factorial(int n) {
return factorial_tail(n, 1);
}
Memoization
Memoization involves caching the results of expensive function calls to avoid redundant computations. This is particularly useful in problems like computing Fibonacci numbers:
#define MAX_N 100
int memo[MAX_N];
int fib(int n) {
if (n <= 1) {
return n;
}
if (memo[n] != 0) {
return memo[n];
}
memo[n] = fib(n - 1) + fib(n - 2);
return memo[n];
}
8. Interview Strategies for Recursive Problems
When tackling recursive problems in coding interviews, consider these strategies:
- Think out loud: Explain your thought process as you develop the recursive solution. This gives the interviewer insight into your problem-solving approach.
- Start with the simplest case: Begin by solving the problem for the most basic input, then build up to more complex scenarios.
- Draw the recursion tree: Visualizing the recursive calls can help you understand the problem better and identify potential optimizations.
- Consider space complexity: Be prepared to discuss the space complexity of your recursive solution, including the call stack usage.
- Have an iterative solution ready: Sometimes, interviewers might ask you to convert your recursive solution to an iterative one. Be prepared for this follow-up.
9. Advanced Recursive Concepts
As you become more comfortable with basic recursion, explore these advanced concepts to further enhance your problem-solving skills:
Divide and Conquer
This technique involves breaking a problem into smaller subproblems, solving them recursively, and then combining the results. Merge Sort is a classic example:
void merge(int arr[], int l, int m, int r) {
// Implementation of merge function
}
void mergeSort(int arr[], int l, int r) {
if (l < r) {
int m = l + (r - l) / 2;
mergeSort(arr, l, m);
mergeSort(arr, m + 1, r);
merge(arr, l, m, r);
}
}
Backtracking
Backtracking is a refined brute-force approach that builds candidates for the solution incrementally and abandons those that cannot possibly lead to a valid solution. The N-Queens problem is a classic backtracking example:
bool isSafe(int board[N][N], int row, int col) {
// Check if a queen can be placed on board[row][col]
}
bool solveNQueensUtil(int board[N][N], int col) {
if (col >= N) {
return true;
}
for (int i = 0; i < N; i++) {
if (isSafe(board, i, col)) {
board[i][col] = 1;
if (solveNQueensUtil(board, col + 1)) {
return true;
}
board[i][col] = 0; // Backtrack
}
}
return false;
}
Tree-based Recursion
Many problems involving trees or graphs naturally lend themselves to recursive solutions. Here’s an example of finding the maximum depth of a binary tree:
int maxDepth(struct TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return 1 + (leftDepth > rightDepth ? leftDepth : rightDepth);
}
10. Conclusion
Mastering recursion is a crucial step in becoming a proficient programmer and excelling in coding interviews. Through this guide, we’ve explored the fundamentals of recursion in C, tackled LeetCode problems, and delved into advanced concepts and optimization techniques.
Remember, the key to mastering recursion is practice. Continue to solve diverse problems on platforms like LeetCode, focusing on understanding the recursive thought process rather than memorizing solutions. As you gain experience, you’ll develop an intuition for when and how to apply recursive techniques effectively.
At AlgoCademy, we’re committed to helping you build strong algorithmic thinking skills. Our interactive coding tutorials and AI-powered assistance are designed to guide you from beginner-level coding to advanced problem-solving techniques required for technical interviews at top tech companies.
Keep practicing, stay curious, and don’t hesitate to explore the wealth of resources available on AlgoCademy. With dedication and the right approach, you’ll be well-prepared to tackle any recursive problem that comes your way in your coding journey and future technical interviews. Happy coding!