Twitter (X) Technical Interview Prep: A Comprehensive Guide
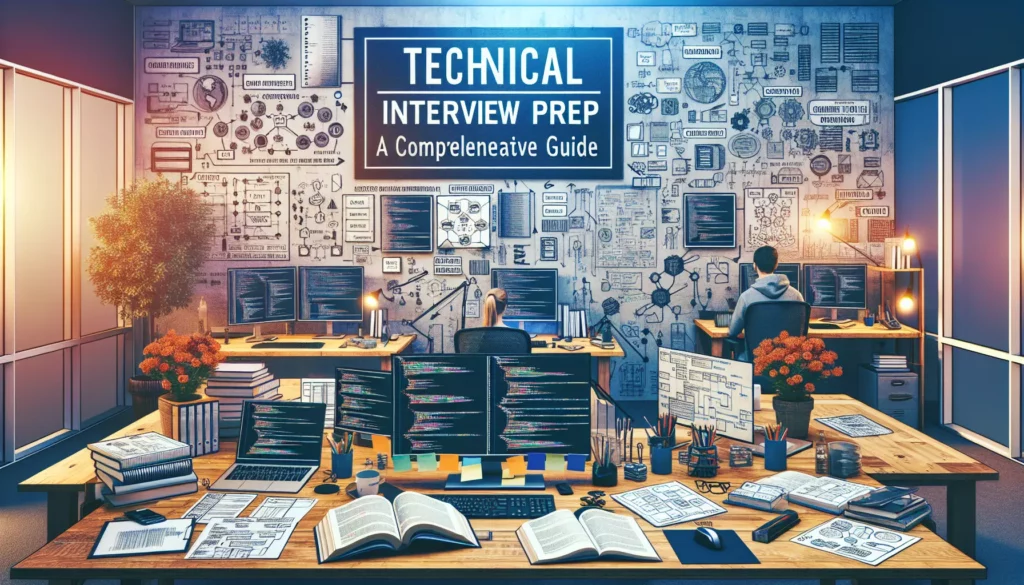
Are you gearing up for a technical interview at Twitter (now known as X)? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your Twitter technical interview. From understanding the interview process to mastering key algorithms and data structures, we’ve got you covered. Let’s dive in and prepare you for success!
Table of Contents
- Understanding the Twitter (X) Interview Process
- Key Topics to Master
- Coding Languages for Twitter Interviews
- Essential Data Structures
- Must-Know Algorithms
- System Design for Twitter
- Behavioral Questions and Soft Skills
- Practice Resources and Mock Interviews
- Interview Day Tips
- Post-Interview Follow-up
1. Understanding the Twitter (X) Interview Process
Before diving into the technical aspects, it’s crucial to understand what to expect during the Twitter interview process. Typically, it consists of several stages:
- Initial Screening: This usually involves a phone call or online assessment to gauge your basic qualifications and interest.
- Technical Phone Screen: A 45-60 minute interview focusing on coding and problem-solving skills.
- On-site Interviews: A series of 4-5 interviews, each lasting about an hour, covering various technical and non-technical aspects.
- Final Decision: The hiring committee reviews all feedback to make a decision.
Each stage is designed to assess different aspects of your skills and fit for the role. The technical interviews will focus heavily on coding, algorithms, and system design, while also evaluating your problem-solving approach and communication skills.
2. Key Topics to Master
To excel in your Twitter technical interview, focus on mastering these key areas:
- Data Structures and Algorithms
- System Design and Scalability
- Object-Oriented Programming
- Distributed Systems
- Database Management
- Network Protocols
- Concurrency and Multithreading
- Big Data Processing
Twitter’s infrastructure handles massive amounts of data and real-time interactions, so a strong foundation in these areas is crucial.
3. Coding Languages for Twitter Interviews
While Twitter uses various programming languages in its tech stack, for interviews, you’re usually free to choose the language you’re most comfortable with. However, some popular choices include:
- Java
- Python
- JavaScript
- Scala
- C++
It’s more important to demonstrate strong coding skills and problem-solving abilities rather than proficiency in a specific language. Choose a language you’re confident in and can write clean, efficient code with.
4. Essential Data Structures
A solid understanding of data structures is crucial for Twitter interviews. Make sure you’re comfortable implementing and using the following:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Hash Tables
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Heaps
- Graphs
- Tries
Let’s look at a quick example of implementing a basic Trie in Python, which could be useful for handling Twitter’s search functionality:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
# Usage
trie = Trie()
trie.insert("twitter")
print(trie.search("twitter")) # True
print(trie.search("tweet")) # False
print(trie.starts_with("twi")) # True
Understanding how to implement and use these data structures efficiently is key to solving many algorithmic problems you might encounter in your Twitter interview.
5. Must-Know Algorithms
Twitter’s technical interviews often include algorithm questions. Make sure you’re well-versed in the following types of algorithms:
- Sorting algorithms (QuickSort, MergeSort, HeapSort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
- String Manipulation
- Bit Manipulation
Here’s an example of a binary search implementation in Java, which could be relevant for efficiently searching through large datasets:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
public static void main(String[] args) {
int[] arr = {1, 3, 5, 7, 9, 11, 13, 15};
int target = 7;
int result = binarySearch(arr, target);
if (result != -1) {
System.out.println("Element found at index: " + result);
} else {
System.out.println("Element not found in the array");
}
}
}
Practice implementing these algorithms from scratch and understanding their time and space complexities. This will help you choose the most efficient solution during your interview.
6. System Design for Twitter
System design is a crucial component of Twitter’s technical interviews, especially for more senior positions. You should be prepared to discuss how you would design large-scale systems similar to Twitter’s core features. Some topics to focus on include:
- Designing a real-time tweet feed
- Implementing a trending topics feature
- Creating a scalable notification system
- Designing a distributed cache
- Implementing a URL shortener service
When approaching system design questions, remember to:
- Clarify requirements and constraints
- Estimate the scale of the system
- Design the high-level architecture
- Dive into component details
- Identify and address potential bottlenecks
- Discuss trade-offs in your design
Here’s a basic example of how you might start designing a simplified version of Twitter’s tweet feed system:
1. Requirements:
- Users can post tweets
- Users can view their home timeline (tweets from users they follow)
- Tweets should appear in real-time
2. Scale Estimation:
- 330 million monthly active users
- 500 million tweets per day
- Average user follows 100 other users
3. High-Level Design:
- Tweet Service: Handles tweet creation and storage
- Timeline Service: Generates user timelines
- User Service: Manages user data and relationships
- Notification Service: Pushes new tweets to users
4. Data Storage:
- Tweets: NoSQL database (e.g., Cassandra) for scalability
- User data: Relational database (e.g., PostgreSQL)
- User-follow relationships: Graph database (e.g., Neo4j)
5. Timeline Generation:
- Pull model: Generate timeline on-demand (good for less active users)
- Push model: Pre-generate timelines (good for active users)
- Hybrid approach: Combine both for optimal performance
6. Real-time Updates:
- Use WebSockets or long polling for real-time communication
7. Caching:
- Implement a distributed cache (e.g., Redis) for frequently accessed data
8. Load Balancing:
- Use load balancers to distribute traffic across multiple servers
This is a simplified overview and would need to be expanded upon in an actual interview.
Remember, the key is not just to memorize a design but to understand the reasoning behind each decision and be able to discuss trade-offs.
7. Behavioral Questions and Soft Skills
While technical skills are crucial, Twitter also values soft skills and cultural fit. Be prepared to answer behavioral questions that assess your:
- Problem-solving approach
- Teamwork and collaboration
- Ability to handle conflicts
- Leadership experience
- Adaptability and learning agility
- Passion for Twitter’s mission and products
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions. Here’s an example:
Question: Tell me about a time when you had to work on a challenging project with a tight deadline.
Answer using STAR method:
Situation: At my previous company, we were tasked with implementing a new feature for our main product that would significantly improve user engagement.
Task: As the lead developer, I was responsible for designing the architecture, delegating tasks, and ensuring we met our two-week deadline.
Action: I broke down the project into smaller, manageable tasks and assigned them based on team members' strengths. I implemented daily stand-ups to track progress and address blockers quickly. When we encountered unexpected technical challenges, I organized a brainstorming session to find creative solutions.
Result: We successfully launched the feature on time, and it resulted in a 20% increase in user engagement within the first month. The project also improved our team's collaboration and our ability to work under pressure.
Practice answering behavioral questions out loud and have specific examples ready from your past experiences.
8. Practice Resources and Mock Interviews
To prepare effectively for your Twitter technical interview, utilize these resources:
- LeetCode: Practice coding problems, especially those tagged as Twitter interview questions.
- HackerRank: Improve your coding skills with various challenges.
- System Design Primer: A GitHub repository with comprehensive system design resources.
- Pramp: Conduct mock interviews with peers.
- GeeksforGeeks: Access a wide range of algorithm and data structure tutorials.
- YouTube channels: Watch coding interview preparation videos from channels like “Tushar Roy – Coding Made Simple” and “Back To Back SWE”.
Additionally, consider these tips for effective practice:
- Set a timer when solving problems to simulate interview conditions.
- Practice explaining your thought process out loud as you code.
- Review and optimize your solutions after solving each problem.
- Participate in coding contests to improve your speed and accuracy.
- Join online communities (e.g., Reddit’s r/cscareerquestions) to share experiences and get advice.
9. Interview Day Tips
On the day of your Twitter technical interview, keep these tips in mind:
- Be punctual: Join the video call or arrive at the office with time to spare.
- Test your equipment: Ensure your internet connection, camera, and microphone are working properly for virtual interviews.
- Have a backup plan: Keep a phone nearby in case of technical issues with video calls.
- Dress appropriately: Aim for business casual, even for virtual interviews.
- Keep your space organized: Have a clean background for video interviews and a notepad for in-person ones.
- Stay calm: Take deep breaths and remember that the interviewer wants you to succeed.
- Communicate clearly: Explain your thought process as you work through problems.
- Ask clarifying questions: Don’t hesitate to seek more information if a problem is unclear.
- Manage your time: Keep an eye on the clock and pace yourself accordingly.
- Show enthusiasm: Demonstrate your passion for technology and Twitter’s mission.
Remember, the interview is also an opportunity for you to assess if Twitter is the right fit for your career goals. Prepare some thoughtful questions to ask your interviewers about the company culture, team dynamics, and growth opportunities.
10. Post-Interview Follow-up
After your Twitter technical interview, take these steps to leave a lasting positive impression:
- Send a thank-you email: Within 24 hours, send a brief email to your interviewer(s) or recruiter, expressing gratitude for their time and reiterating your interest in the position.
- Reflect on the experience: Take notes on the questions asked and how you performed. This will help you improve for future interviews.
- Follow up appropriately: If you haven’t heard back within the timeframe provided, it’s okay to send a polite follow-up email to check on the status of your application.
- Keep practicing: Regardless of the outcome, continue honing your skills and preparing for future opportunities.
- Stay connected: Consider connecting with your interviewers on LinkedIn, if appropriate.
Here’s a template for a post-interview thank-you email:
Subject: Thank you for the interview - [Your Name]
Dear [Interviewer's Name],
I wanted to thank you for taking the time to meet with me yesterday regarding the [Position Name] role at Twitter. I enjoyed our conversation about [specific topic discussed] and am even more excited about the possibility of joining your team.
The project you mentioned involving [specific detail] sounds particularly interesting, and I believe my experience with [relevant skill] would allow me to contribute effectively.
Thank you again for your time and consideration. I look forward to hearing about the next steps in the process.
Best regards,
[Your Name]
Remember, preparation is key to success in your Twitter technical interview. By mastering the topics we’ve covered and following these tips, you’ll be well-equipped to showcase your skills and land that dream job at Twitter (X). Good luck with your interview!