Mastering the Subsequence Sum Equal to K Problem on LeetCode
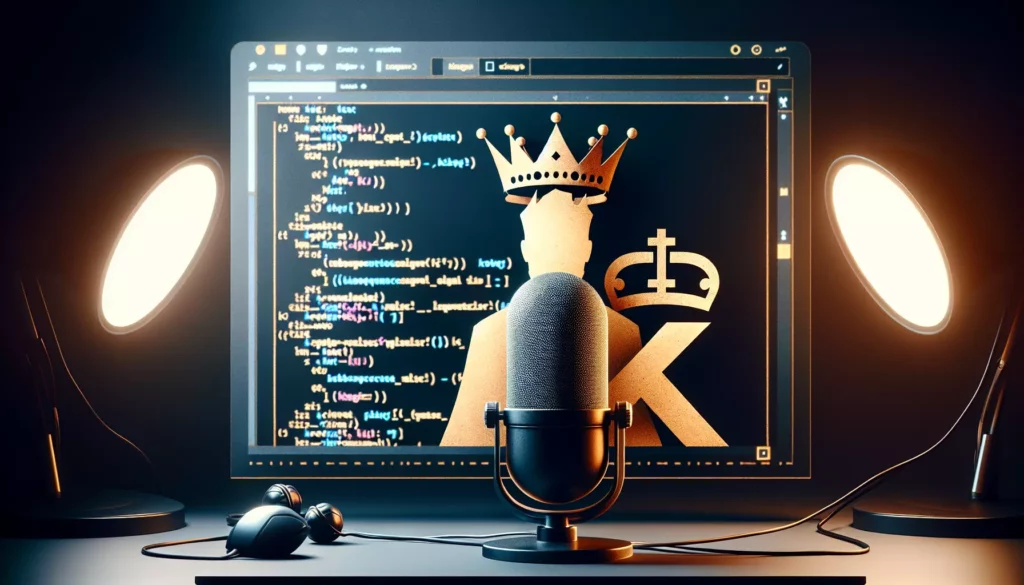
Welcome to another exciting deep dive into algorithmic problem-solving! Today, we’re tackling a classic yet challenging problem that often appears in coding interviews and competitions: finding a subsequence with a sum equal to a given target K. This problem is not only a favorite among interviewers at top tech companies but also a fundamental building block for more complex algorithmic concepts.
Understanding the Problem
Before we dive into the solution, let’s break down what the problem is asking:
Given an array of integers and a target sum K, determine if there exists a subsequence in the array that adds up to exactly K.
A subsequence is a sequence that can be derived from the array by deleting some or no elements without changing the order of the remaining elements. This is different from a subarray, which requires contiguous elements.
The Importance of This Problem
Understanding how to solve the subsequence sum problem is crucial for several reasons:
- It tests your ability to think recursively and use dynamic programming.
- It’s a stepping stone to more complex problems involving subsets and combinations.
- It’s frequently asked in interviews at companies like Google, Amazon, and Facebook.
- The concepts used in solving this problem are applicable to many real-world scenarios involving optimization and decision-making.
Approaching the Solution
There are multiple ways to approach this problem, each with its own trade-offs in terms of time and space complexity. We’ll explore three main approaches:
- Recursive (Brute Force) Approach
- Dynamic Programming Approach
- Optimized Dynamic Programming Approach
1. Recursive (Brute Force) Approach
The recursive approach is the most intuitive way to solve this problem. The idea is to consider every possible subsequence and check if its sum equals the target K.
class Solution {
public:
bool isSubsetSum(vector<int>& nums, int n, int sum) {
// Base cases
if (sum == 0)
return true;
if (n == 0)
return false;
// If last element is greater than sum, ignore it
if (nums[n - 1] > sum)
return isSubsetSum(nums, n - 1, sum);
// Check if sum can be obtained by either including or excluding the last element
return isSubsetSum(nums, n - 1, sum) || isSubsetSum(nums, n - 1, sum - nums[n - 1]);
}
bool canPartition(vector<int>& nums) {
int sum = 0;
for (int num : nums)
sum += num;
// If sum is odd, it can't be partitioned into two equal subsets
if (sum % 2 != 0)
return false;
return isSubsetSum(nums, nums.size(), sum / 2);
}
};
While this approach is straightforward, it has a time complexity of O(2^n), making it impractical for large inputs. However, it’s a good starting point to understand the problem.
2. Dynamic Programming Approach
To improve upon the recursive solution, we can use dynamic programming. This approach allows us to store and reuse previously computed results, significantly reducing the time complexity.
class Solution {
public:
bool canPartition(vector<int>& nums) {
int sum = 0;
for (int num : nums)
sum += num;
if (sum % 2 != 0)
return false;
int target = sum / 2;
int n = nums.size();
vector<vector<bool>> dp(n + 1, vector<bool>(target + 1, false));
// Initialize base cases
for (int i = 0; i <= n; i++)
dp[i][0] = true;
// Fill the dp table
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= target; j++) {
if (j < nums[i - 1])
dp[i][j] = dp[i - 1][j];
else
dp[i][j] = dp[i - 1][j] || dp[i - 1][j - nums[i - 1]];
}
}
return dp[n][target];
}
};
This solution has a time complexity of O(n * sum) and a space complexity of O(n * sum), where n is the number of elements in the array and sum is the target sum.
3. Optimized Dynamic Programming Approach
We can further optimize the dynamic programming solution by using a 1D array instead of a 2D array, reducing the space complexity to O(sum).
class Solution {
public:
bool canPartition(vector<int>& nums) {
int sum = 0;
for (int num : nums)
sum += num;
if (sum % 2 != 0)
return false;
int target = sum / 2;
vector<bool> dp(target + 1, false);
dp[0] = true;
for (int num : nums) {
for (int j = target; j >= num; j--) {
dp[j] = dp[j] || dp[j - num];
}
}
return dp[target];
}
};
This optimized solution maintains the same time complexity of O(n * sum) but reduces the space complexity to O(sum).
Time and Space Complexity Analysis
Let’s compare the time and space complexities of our three approaches:
Approach | Time Complexity | Space Complexity |
---|---|---|
Recursive | O(2^n) | O(n) (call stack) |
DP (2D array) | O(n * sum) | O(n * sum) |
DP (1D array) | O(n * sum) | O(sum) |
As we can see, the dynamic programming approaches significantly improve the time complexity compared to the recursive approach, with the 1D DP solution offering the best space efficiency.
Common Pitfalls and Edge Cases
When solving this problem, be aware of these common pitfalls and edge cases:
- Forgetting to check if the total sum is odd (which would make equal partition impossible)
- Not handling the case where the array is empty or contains only one element
- Overlooking the possibility of negative numbers in the array (if allowed by the problem statement)
- Failing to consider the case where the target sum is 0
Related Problems and Variations
Once you’ve mastered the subsequence sum equal to K problem, you can explore these related problems to further enhance your skills:
- Subset Sum Problem: Determine if there’s a subset with a given sum (similar to our problem but doesn’t require partitioning)
- Partition Equal Subset Sum: Divide the array into two subsets with equal sum (which is what we solved)
- Minimum Subset Sum Difference: Find a partition that minimizes the difference between subset sums
- Count of Subsets Sum with a Given Sum: Count the number of subsets that sum to a target value
- Target Sum: Assign + or – signs to array elements to achieve a target sum
Real-World Applications
Understanding and solving the subsequence sum problem has practical applications beyond just acing coding interviews. Some real-world scenarios where similar concepts are applied include:
- Resource Allocation: Optimizing the distribution of resources in project management or manufacturing
- Financial Portfolio Management: Selecting a subset of investments to achieve a target return
- Network Design: Optimizing data packet routing to meet specific bandwidth requirements
- Cryptography: Some encryption algorithms rely on the difficulty of solving subset sum problems
- Bin Packing: Efficiently packing items into containers, which is crucial in logistics and storage optimization
Tips for Solving Similar Problems
To improve your ability to solve problems like the subsequence sum equal to K, consider these tips:
- Start with the brute force solution to understand the problem thoroughly
- Look for overlapping subproblems, which is a key indicator that dynamic programming might be applicable
- Practice drawing out the dynamic programming table to visualize the solution
- Always consider whether you can optimize your DP solution further (e.g., reducing 2D DP to 1D)
- Test your solution with various edge cases to ensure robustness
- Study the pattern of how the current state depends on previous states in DP problems
Conclusion
The subsequence sum equal to K problem is a classic example of how dynamic programming can dramatically improve the efficiency of our solutions. By breaking down the problem into smaller subproblems and storing the results, we can avoid redundant calculations and achieve a much more efficient algorithm.
As you continue your journey in algorithmic problem-solving, remember that mastering problems like this one builds a strong foundation for tackling more complex challenges. The skills you develop here—recursive thinking, recognizing overlapping subproblems, and optimizing space usage—are invaluable in many areas of computer science and software engineering.
Keep practicing, and don’t be discouraged if you don’t arrive at the optimal solution immediately. Problem-solving is a skill that improves with consistent effort and exposure to various problem types. Happy coding, and may your subsequences always sum up to your target!