Finding the Maximum Length of Valid Subsequence: A Comprehensive Guide
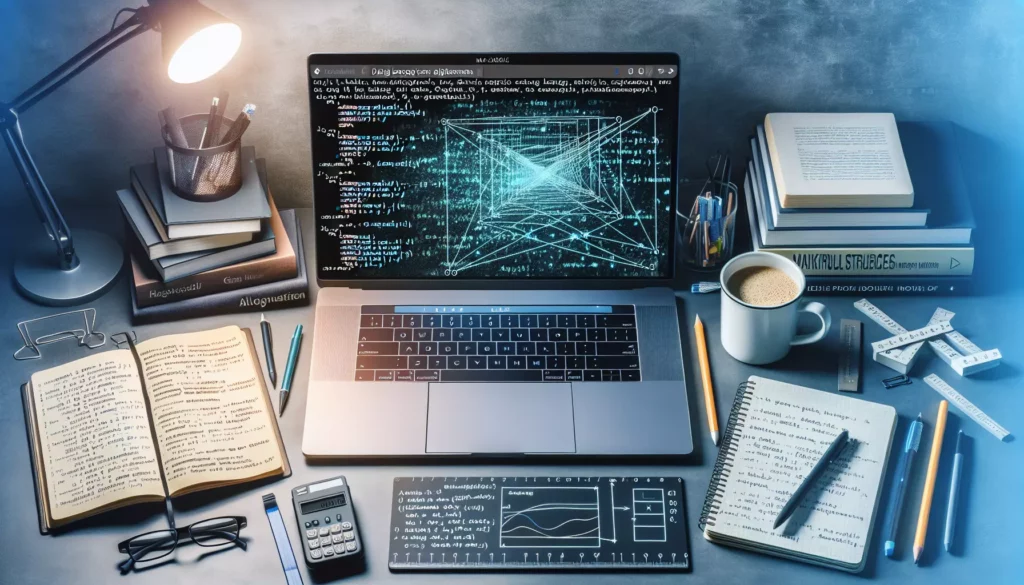
In the world of competitive programming and technical interviews, particularly for major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding and implementing efficient algorithms is crucial. One such problem that often appears in coding challenges is finding the maximum length of a valid subsequence. This article will dive deep into this problem, exploring various approaches and providing step-by-step solutions to help you master this concept.
Understanding the Problem
Before we delve into the solutions, let’s clearly define what we mean by “finding the maximum length of valid subsequence”:
Given a string or an array of characters, we need to find the length of the longest subsequence that satisfies certain conditions. These conditions can vary depending on the specific problem, but common examples include:
- Finding the longest increasing subsequence
- Finding the longest common subsequence between two strings
- Finding the longest palindromic subsequence
In this article, we’ll focus on the first example: finding the longest increasing subsequence (LIS) in an array of numbers. This problem is a classic example of dynamic programming and is often used to test a candidate’s understanding of algorithmic thinking and problem-solving skills.
Problem Statement: Longest Increasing Subsequence (LIS)
Given an unsorted array of integers, find the length of the longest subsequence such that all elements in the subsequence are sorted in increasing order.
For example, given the array [10, 9, 2, 5, 3, 7, 101, 18], the longest increasing subsequence is [2, 5, 7, 101], and the length of the LIS is 4.
Approach 1: Brute Force
Let’s start with the simplest approach: brute force. While this method is not efficient for large inputs, it helps in understanding the problem better.
Algorithm:
- Generate all possible subsequences of the given array.
- Check if each subsequence is increasing.
- Keep track of the maximum length among all increasing subsequences.
Implementation:
def longest_increasing_subsequence_brute_force(arr):
n = len(arr)
def is_increasing(seq):
return all(seq[i] < seq[i+1] for i in range(len(seq) - 1))
max_length = 0
for i in range(1, 2**n):
subsequence = [arr[j] for j in range(n) if (i & (1 << j))]
if is_increasing(subsequence):
max_length = max(max_length, len(subsequence))
return max_length
# Example usage
arr = [10, 9, 2, 5, 3, 7, 101, 18]
print(longest_increasing_subsequence_brute_force(arr)) # Output: 4
This approach has a time complexity of O(2^n * n), where n is the length of the input array. It’s not suitable for large inputs but helps in understanding the problem.
Approach 2: Dynamic Programming
Dynamic programming is a more efficient approach to solve the LIS problem. It builds the solution to larger subproblems using the solutions of smaller subproblems.
Algorithm:
- Create an array dp of the same length as the input array, initialized with 1s.
- For each element arr[i], look at all previous elements arr[j] where j < i.
- If arr[i] > arr[j], update dp[i] = max(dp[i], dp[j] + 1).
- The maximum value in dp array is the length of the longest increasing subsequence.
Implementation:
def longest_increasing_subsequence_dp(arr):
n = len(arr)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if arr[i] > arr[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
# Example usage
arr = [10, 9, 2, 5, 3, 7, 101, 18]
print(longest_increasing_subsequence_dp(arr)) # Output: 4
This approach has a time complexity of O(n^2) and space complexity of O(n), where n is the length of the input array. It’s much more efficient than the brute force approach and works well for moderate-sized inputs.
Approach 3: Dynamic Programming with Binary Search
We can further optimize the dynamic programming approach by using binary search. This method constructs the longest increasing subsequence efficiently.
Algorithm:
- Initialize an empty array tails to store the smallest tail of all increasing subsequences.
- For each number in the input array:
- If it’s larger than all tails, append it to tails.
- Otherwise, find the first tail that’s greater than or equal to the number and replace it.
- The length of tails is the length of the longest increasing subsequence.
Implementation:
import bisect
def longest_increasing_subsequence_optimized(arr):
tails = []
for num in arr:
if not tails or num > tails[-1]:
tails.append(num)
else:
idx = bisect.bisect_left(tails, num)
tails[idx] = num
return len(tails)
# Example usage
arr = [10, 9, 2, 5, 3, 7, 101, 18]
print(longest_increasing_subsequence_optimized(arr)) # Output: 4
This approach has a time complexity of O(n log n) and space complexity of O(n), where n is the length of the input array. It’s the most efficient among the three approaches we’ve discussed and is suitable for large inputs.
Variations of the Problem
The concept of finding the maximum length of a valid subsequence can be applied to various other problems. Let’s explore a few variations:
1. Longest Common Subsequence (LCS)
Given two strings, find the length of their longest common subsequence.
def longest_common_subsequence(text1, text2):
m, n = len(text1), len(text2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if text1[i-1] == text2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[m][n]
# Example usage
text1 = "abcde"
text2 = "ace"
print(longest_common_subsequence(text1, text2)) # Output: 3
2. Longest Palindromic Subsequence
Find the length of the longest palindromic subsequence in a given string.
def longest_palindromic_subsequence(s):
n = len(s)
dp = [[0] * n for _ in range(n)]
for i in range(n):
dp[i][i] = 1
for length in range(2, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and length == 2:
dp[i][j] = 2
elif s[i] == s[j]:
dp[i][j] = dp[i+1][j-1] + 2
else:
dp[i][j] = max(dp[i+1][j], dp[i][j-1])
return dp[0][n-1]
# Example usage
s = "bbbab"
print(longest_palindromic_subsequence(s)) # Output: 4
Tips for Solving Subsequence Problems
- Understand the problem: Clearly define what constitutes a valid subsequence for the given problem.
- Consider dynamic programming: Many subsequence problems can be efficiently solved using dynamic programming.
- Identify the subproblem structure: Look for ways to break down the problem into smaller, overlapping subproblems.
- Define the recurrence relation: Establish how the solution to a larger problem relates to the solutions of smaller subproblems.
- Optimize space usage: Often, you can reduce the space complexity by using only a 1D array instead of a 2D array in dynamic programming solutions.
- Consider binary search: For problems like the Longest Increasing Subsequence, using binary search can significantly improve time complexity.
- Practice with variations: Solving different types of subsequence problems will help you recognize patterns and improve your problem-solving skills.
Common Pitfalls and How to Avoid Them
- Confusing subsequence with subarray: Remember that elements in a subsequence don’t need to be contiguous, unlike in a subarray.
- Overlooking edge cases: Always consider empty arrays or strings, and arrays/strings with a single element.
- Inefficient implementations: Start with a working solution, then optimize. Don’t prematurely optimize at the cost of correctness.
- Not considering all possible subsequences: In brute force approaches, ensure you’re generating all possible subsequences.
- Incorrect initialization in DP: Pay attention to how you initialize your DP array, as it can affect the final result.
- Off-by-one errors: Be careful with array indices, especially when dealing with strings and 1-indexed vs. 0-indexed arrays.
Conclusion
Finding the maximum length of a valid subsequence is a fundamental problem in computer science and a common challenge in coding interviews. By understanding the various approaches to solve this problem – from brute force to optimized dynamic programming – you’ll be better equipped to tackle similar challenges in your coding journey.
Remember, the key to mastering these problems is practice. Try implementing these solutions, experiment with different inputs, and challenge yourself with variations of the problem. As you gain more experience, you’ll develop a intuition for recognizing patterns and choosing the most efficient approach for each problem.
Keep coding, keep learning, and don’t hesitate to dive deep into the algorithms and data structures that power these solutions. Your efforts will pay off as you become more proficient in problem-solving and algorithmic thinking – skills that are highly valued in the tech industry, especially at companies like FAANG.