Mastering Dropbox Technical Interview Prep: A Comprehensive Guide
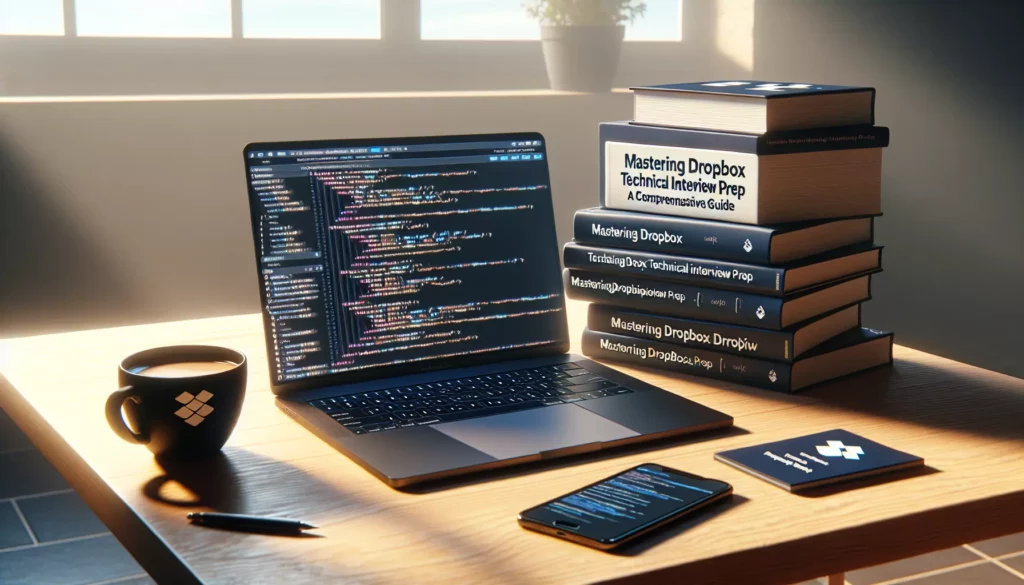
Are you gearing up for a technical interview at Dropbox? You’re in the right place. This comprehensive guide will walk you through everything you need to know to ace your Dropbox technical interview. From understanding the interview process to mastering key algorithms and data structures, we’ve got you covered.
Table of Contents
- Understanding Dropbox: Company Culture and Technology
- The Dropbox Interview Process
- Core Concepts to Master
- Common Coding Challenges and How to Approach Them
- System Design for Dropbox Interviews
- Behavioral Questions and Cultural Fit
- Effective Preparation Strategies
- What to Expect on Interview Day
- Post-Interview: Next Steps and Follow-up
- Additional Resources for Dropbox Interview Prep
1. Understanding Dropbox: Company Culture and Technology
Before diving into the technical aspects of your interview prep, it’s crucial to understand Dropbox as a company. Dropbox is known for its cloud storage and file synchronization services, but it’s much more than that. The company has a strong focus on simplifying the way people work together.
Key Aspects of Dropbox’s Technology:
- Distributed systems
- File synchronization algorithms
- Data compression and storage optimization
- Security and encryption
- Collaboration tools
Understanding these core areas will give you a significant advantage in your interview. It shows that you’ve done your homework and are genuinely interested in the company’s technology stack.
Dropbox Culture:
Dropbox values creativity, innovation, and a collaborative work environment. They look for candidates who are not only technically proficient but also align with their cultural values. Some key aspects of Dropbox culture include:
- Emphasis on simplicity and user experience
- Encouragement of diverse perspectives
- Focus on continuous learning and growth
- Commitment to work-life balance
2. The Dropbox Interview Process
The Dropbox technical interview process typically consists of several stages:
- Initial Phone Screen: This is usually a brief call with a recruiter to discuss your background and interest in Dropbox.
- Technical Phone Interview: You’ll solve coding problems in real-time, often using a shared coding environment.
- On-site Interviews: This typically includes multiple rounds of technical interviews, a system design interview, and behavioral interviews.
- Final Evaluation: The interview team meets to discuss your performance and make a hiring decision.
Each stage is designed to assess different aspects of your skills and fit for the role. Let’s break down what you need to focus on for each part of the process.
3. Core Concepts to Master
To excel in your Dropbox technical interview, you should have a strong grasp of the following concepts:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Recursion
- Greedy Algorithms
System Design Concepts:
- Scalability
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
Programming Languages:
While Dropbox doesn’t require expertise in a specific language, it’s beneficial to be proficient in at least one of the following:
- Python (Dropbox uses Python extensively)
- Java
- C++
- Go
4. Common Coding Challenges and How to Approach Them
Dropbox interviews often include coding challenges that test your problem-solving skills and coding ability. Here are some common types of problems you might encounter:
String Manipulation:
Example: Implement a function to check if two strings are anagrams of each other.
def are_anagrams(str1, str2):
# Remove spaces and convert to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if lengths are different
if len(str1) != len(str2):
return False
# Create dictionaries to store character counts
char_count1 = {}
char_count2 = {}
# Count characters in both strings
for char in str1:
char_count1[char] = char_count1.get(char, 0) + 1
for char in str2:
char_count2[char] = char_count2.get(char, 0) + 1
# Compare character counts
return char_count1 == char_count2
# Test the function
print(are_anagrams("listen", "silent")) # Should return True
print(are_anagrams("hello", "world")) # Should return False
Array Manipulation:
Example: Find the maximum subarray sum in an array of integers.
def max_subarray_sum(arr):
max_sum = float('-inf')
current_sum = 0
for num in arr:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # Should return 6
Tree Traversal:
Example: Implement an in-order traversal of a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
# Test the function
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Should return [4, 2, 5, 1, 3]
Graph Algorithms:
Example: Implement Depth-First Search (DFS) on a graph.
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def dfs_util(self, v, visited):
visited.add(v)
print(v, end=' ')
for neighbor in self.graph[v]:
if neighbor not in visited:
self.dfs_util(neighbor, visited)
def dfs(self, v):
visited = set()
self.dfs_util(v, visited)
# Test the function
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("DFS starting from vertex 2:")
g.dfs(2) # Should print: 2 0 1 3
Dynamic Programming:
Example: Implement a function to calculate the nth Fibonacci number using dynamic programming.
def fibonacci(n):
if n
5. System Design for Dropbox Interviews
System design questions are a crucial part of the Dropbox interview process, especially for more senior positions. Here’s how you can approach a system design question:
Steps to Tackle System Design Questions:
- Clarify Requirements: Ask questions to understand the scope and constraints of the system.
- Estimate Scale: Determine the scale of the system (users, data volume, etc.).
- Define API: Outline the main API endpoints the system will need.
- Define Data Model: Sketch out the main entities and their relationships.
- High-Level Design: Draw a block diagram of the major components.
- Detailed Design: Dive deeper into critical components.
- Identify Bottlenecks: Discuss potential issues and how to address them.
- Scaling the Design: Explain how the system can scale to handle growth.
Example: Design a File Sharing System (like Dropbox)
Let’s walk through a high-level design for a file sharing system:
1. Requirements:
- Users can upload and download files
- Files should be synced across devices
- Users can share files with others
- The system should be highly available and scalable
2. Scale Estimation:
- Assume 500 million users
- Average user uploads 2 GB of data
- Total storage needed: 1 Exabyte (1 million TB)
3. API Design:
uploadFile(user_id, file, file_metadata)
downloadFile(user_id, file_id)
deleteFile(user_id, file_id)
shareFile(user_id, file_id, target_user_id)
syncFile(user_id, file_id, device_id)
4. Data Model:
- User: id, name, email, password_hash
- File: id, name, size, owner_id, created_at, updated_at
- FileVersion: id, file_id, version_number, content_hash
- SharedFile: id, file_id, shared_with_user_id, permissions
5. High-Level Design:
- Client Applications (Web, Mobile, Desktop)
- Load Balancer
- Application Servers
- Metadata Database (e.g., PostgreSQL)
- Object Storage (e.g., Amazon S3)
- Notification Service
- Synchronization Service
- Caching Layer (e.g., Redis)
6. Detailed Design:
Focus on key components like the Synchronization Service:
- Use a distributed queue (e.g., Apache Kafka) to handle sync events
- Implement a diff algorithm to minimize data transfer
- Use content-based addressing to identify file changes
7. Bottlenecks and Solutions:
- Database scaling: Use sharding based on user_id
- Storage scaling: Use consistent hashing for distributed object storage
- Network bottleneck: Implement CDN for faster downloads
8. Scaling the Design:
- Use microservices architecture for better scalability
- Implement auto-scaling for application servers
- Use read replicas for the database to handle increased read traffic
Remember, in a system design interview, it’s important to think out loud and explain your reasoning. The interviewer is interested in your thought process as much as the final design.
6. Behavioral Questions and Cultural Fit
Dropbox places a high value on cultural fit. You’ll likely face behavioral questions that assess your alignment with the company’s values. Here are some common themes and example questions:
Collaboration and Teamwork:
- Describe a time when you had to work closely with someone whose personality was very different from yours.
- Tell me about a successful project you worked on as part of a team. What was your role?
Problem-Solving and Innovation:
- Can you share an example of a complex technical problem you solved? How did you approach it?
- Describe a time when you had to think outside the box to solve a problem.
Adaptability and Learning:
- Tell me about a time when you had to learn a new technology or skill quickly. How did you approach it?
- Describe a situation where you had to adapt to a significant change at work.
Leadership and Initiative:
- Can you give an example of a time when you took the lead on a project or initiative?
- Describe a situation where you saw a problem and took the initiative to correct it.
Tips for Behavioral Questions:
- Use the STAR method (Situation, Task, Action, Result) to structure your answers.
- Prepare specific examples from your past experiences.
- Be honest and authentic in your responses.
- Highlight how your actions aligned with Dropbox’s values.
- Be concise but provide enough detail to paint a clear picture.
7. Effective Preparation Strategies
To maximize your chances of success in the Dropbox technical interview, consider the following preparation strategies:
1. Review Computer Science Fundamentals:
- Brush up on data structures, algorithms, and system design concepts.
- Practice implementing these concepts from scratch.
2. Solve Coding Problems Regularly:
- Use platforms like LeetCode, HackerRank, or AlgoCademy to practice coding problems.
- Focus on medium to hard difficulty problems.
- Time yourself to simulate interview conditions.
3. Mock Interviews:
- Practice with a friend or use online platforms that offer mock interviews.
- Get comfortable explaining your thought process while coding.
4. System Design Practice:
- Study existing system designs of popular services.
- Practice designing systems from scratch.
- Focus on scalability, reliability, and performance considerations.
5. Learn About Dropbox:
- Research Dropbox’s products, technologies, and recent news.
- Understand the company’s culture and values.
6. Improve Your Communication Skills:
- Practice explaining complex technical concepts in simple terms.
- Work on articulating your thoughts clearly and concisely.
7. Review Your Own Projects:
- Be prepared to discuss your past projects in detail.
- Highlight challenges you faced and how you overcame them.
8. Stay Updated with Industry Trends:
- Keep abreast of the latest developments in cloud storage, file synchronization, and collaboration tools.
- Understand the challenges and opportunities in the industry.
8. What to Expect on Interview Day
Being well-prepared for the actual interview day can help calm your nerves and improve your performance. Here’s what you can typically expect:
Before the Interview:
- Confirm the interview schedule and format (in-person or virtual).
- Test your equipment if it’s a virtual interview.
- Review your resume and be prepared to discuss any item on it.
- Prepare questions to ask your interviewers about Dropbox and the role.
During the Interview:
- Be punctual. Aim to arrive or log in 5-10 minutes early.
- Dress professionally, even for virtual interviews.
- Bring a notebook and pen for taking notes.
- Listen carefully to each question and ask for clarification if needed.
- Think out loud during coding and system design questions.
- Be honest if you don’t know something. Show your problem-solving approach instead.
Typical Interview Structure:
- Introduction and brief chat with the interviewer
- Technical questions or coding challenge
- System design discussion (for more senior roles)
- Behavioral questions
- Time for you to ask questions
- Wrap-up and next steps
Tips for Virtual Interviews:
- Ensure you have a stable internet connection.
- Choose a quiet, well-lit location for the interview.
- Have a backup plan (phone number, alternative device) in case of technical issues.
- Maintain eye contact by looking at the camera, not the screen.
9. Post-Interview: Next Steps and Follow-up
After your interview, there are several steps you can take to leave a lasting positive impression:
1. Send Thank-You Notes:
- Email a personalized thank-you note to each interviewer within 24 hours.
- Express your appreciation for their time and reiterate your interest in the role.
- Briefly mention a specific topic from your conversation to make it more memorable.
2. Reflect on the Interview:
- Write down the questions you were asked and how you answered them.
- Note any areas where you felt you could have performed better.
- Use this reflection for future interview preparation.
3. Follow Up Appropriately:
- If you haven’t heard back within the timeframe they specified, it’s okay to send a polite follow-up email.
- Keep the email brief and reaffirm your interest in the position.
4. Continue Your Preparation:
- Don’t stop your preparation efforts, especially if you’re in the middle of the interview process.
- Use any insights gained from the interview to focus your further study.
5. Be Patient:
- The hiring process can take time, especially for larger companies like Dropbox.
- Avoid constantly checking your email or phone for a response.
6. Consider Other Opportunities:
- Continue pursuing other job opportunities until you have a firm offer.
- This keeps your options open and maintains your interview skills.
10. Additional Resources for Dropbox Interview Prep
To further enhance your preparation for the Dropbox technical interview, consider these additional resources:
Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Designing Data-Intensive Applications” by Martin Kleppmann
Online Courses:
- AlgoCademy’s coding interview preparation courses
- Coursera’s “Algorithms Specialization” by Stanford University
- Udacity’s “Design of Computer Programs” course
Websites and Platforms:
- LeetCode for coding practice
- HackerRank for algorithm challenges
- System Design Primer on GitHub
- Dropbox Engineering Blog for insights into their technology
YouTube Channels:
- MIT OpenCourseWare for computer science fundamentals
- Google Developers channel for tech talks and coding tips
- Gaurav Sen for system design explanations
Community Forums:
- Dropbox-related discussions on Reddit
- Stack Overflow for specific coding questions
- Blind for anonymous tech industry insights
Remember, while these resources are valuable, the key to success in your Dropbox interview lies in consistent practice, a deep understanding of fundamental concepts, and the ability to apply your knowledge to solve real-world problems. Good luck with your preparation!