LeetCode Dream: Mastering Coding Challenges for Your Tech Career
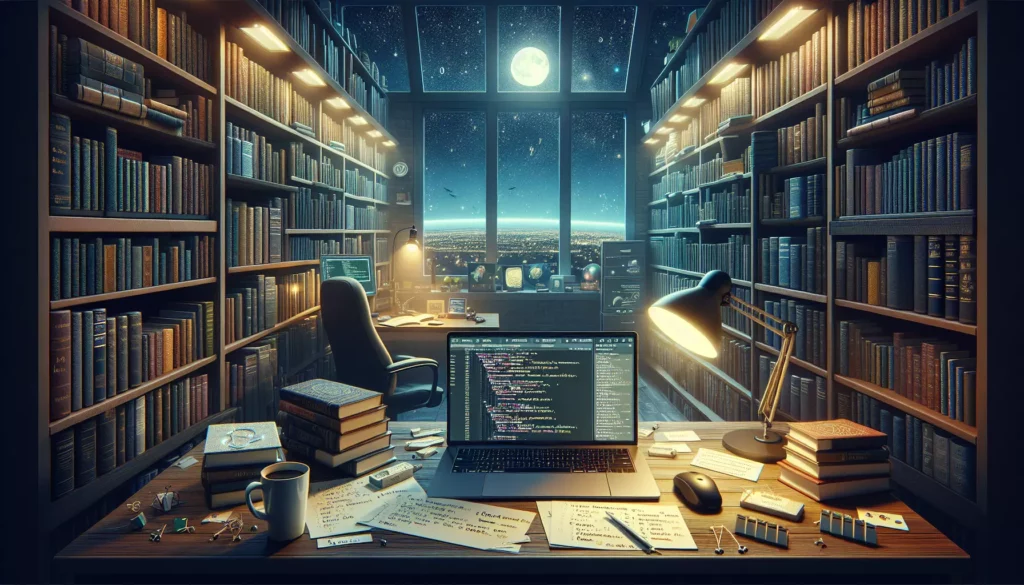
In the ever-evolving landscape of technology, aspiring developers and seasoned programmers alike share a common goal: to excel in their careers and land coveted positions at top tech companies. Enter LeetCode, a platform that has become synonymous with coding interview preparation and algorithmic mastery. This article delves into the world of LeetCode, exploring its significance, strategies for success, and how it fits into the broader context of coding education and career development.
What is LeetCode?
LeetCode is an online platform that offers a vast collection of coding challenges and interview preparation resources. It has gained immense popularity among software engineers, computer science students, and anyone looking to sharpen their programming skills. The platform provides:
- A database of over 1,500 coding problems
- Solutions and discussions for each problem
- Mock interviews and company-specific question sets
- Contests and competitions
- A vibrant community of learners and professionals
LeetCode’s problems cover a wide range of topics, including algorithms, data structures, database management, and system design. The challenges are categorized by difficulty (Easy, Medium, Hard) and are often similar to questions asked in technical interviews at major tech companies.
The LeetCode Dream: Why It Matters
For many in the tech industry, mastering LeetCode has become a rite of passage. The “LeetCode dream” represents the aspiration to excel in coding interviews and secure positions at prestigious companies like Google, Amazon, Facebook, Apple, and Netflix (often collectively referred to as FAANG). Here’s why LeetCode has become so crucial:
1. Interview Preparation
Many tech companies use coding challenges similar to those found on LeetCode during their interview process. Familiarity with these types of problems can significantly boost a candidate’s confidence and performance.
2. Skill Enhancement
Regular practice on LeetCode helps developers improve their problem-solving skills, algorithmic thinking, and coding efficiency. These skills are valuable not just for interviews but for day-to-day work as a software engineer.
3. Language Proficiency
LeetCode supports multiple programming languages, allowing users to strengthen their skills in their preferred language or learn a new one.
4. Community and Networking
The platform’s discussion forums and contest features provide opportunities to connect with other developers, share insights, and learn from peers.
Strategies for LeetCode Success
While the prospect of tackling hundreds of coding challenges can be daunting, a structured approach can help you make steady progress and achieve your LeetCode dreams. Here are some strategies to consider:
1. Start with the Fundamentals
Before diving into complex problems, ensure you have a solid grasp of basic data structures and algorithms. This includes:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Sorting and searching algorithms
- Dynamic programming
2. Follow a Structured Learning Path
LeetCode offers curated problem sets and study plans. Consider following these or creating your own structured path to ensure you cover a wide range of topics systematically.
3. Practice Consistently
Consistency is key. Set a goal to solve a certain number of problems each day or week, even if it’s just one or two. Regular practice helps reinforce concepts and improves your problem-solving speed.
4. Time Your Problem-Solving
In real interviews, you’ll be working under time constraints. Practice solving problems within a set time limit to improve your efficiency and simulate interview conditions.
5. Review and Reflect
After solving a problem, take time to review other solutions in the discussion forum. Look for more efficient approaches or elegant code implementations. Reflect on what you’ve learned from each problem.
6. Participate in Contests
LeetCode’s weekly contests are a great way to challenge yourself, gauge your progress, and experience the pressure of solving problems under time constraints.
7. Focus on Understanding, Not Memorization
While it’s tempting to memorize solutions, focus on understanding the underlying concepts and problem-solving techniques. This will help you adapt to new, unfamiliar problems during interviews.
Beyond LeetCode: Holistic Coding Education
While LeetCode is an excellent resource for honing your coding skills and preparing for interviews, it’s important to remember that it’s just one part of a comprehensive coding education. To truly excel in your tech career, consider complementing your LeetCode practice with other learning resources and activities:
1. Online Coding Platforms
Explore other platforms like HackerRank, CodeSignal, or AlgoCademy to diversify your learning experience and access different types of challenges and tutorials.
2. Computer Science Fundamentals
Strengthen your understanding of core CS concepts through online courses, textbooks, or formal education. Topics like operating systems, computer networks, and databases are crucial for a well-rounded knowledge base.
3. Project-Based Learning
Apply your skills to real-world projects. Build applications, contribute to open-source projects, or create your own software to gain practical experience and showcase your abilities.
4. Soft Skills Development
Don’t neglect the importance of communication, teamwork, and problem-solving skills. These are often just as crucial as technical abilities in the workplace.
5. Stay Updated with Industry Trends
Follow tech blogs, attend conferences or webinars, and engage with the developer community to stay informed about the latest trends and technologies in the industry.
The Role of AI in Coding Education
As we discuss the LeetCode dream and coding education, it’s important to acknowledge the growing role of artificial intelligence in this field. AI-powered tools and platforms are revolutionizing how people learn to code and prepare for technical interviews. Here are some ways AI is impacting coding education:
1. Personalized Learning Paths
AI algorithms can analyze a learner’s performance and tailor problem sets and tutorials to their specific needs and skill level. This personalized approach helps learners progress more efficiently and effectively.
2. Intelligent Code Analysis
AI-powered code review tools can provide instant feedback on code quality, efficiency, and potential improvements. This real-time guidance helps learners develop better coding practices.
3. Natural Language Processing for Problem Understanding
Advanced NLP techniques are being used to help learners better understand problem statements and requirements, bridging the gap between human language and code implementation.
4. AI-Assisted Coding
Tools like GitHub Copilot use AI to suggest code completions and entire functions based on context, helping developers write code more quickly and efficiently.
5. Automated Interview Preparation
AI-powered mock interview tools can simulate real interview scenarios, providing a more interactive and realistic preparation experience.
Coding Challenges: A Practical Example
To give you a taste of what LeetCode-style problems look like, let’s examine a classic coding challenge: reversing a linked list. This problem is often asked in interviews and is an excellent exercise for understanding data structures and pointer manipulation.
Problem: Reverse a Linked List
Given the head of a singly linked list, reverse the list, and return the reversed list.
Example:
Input: 1 -> 2 -> 3 -> 4 -> 5
Output: 5 -> 4 -> 3 -> 2 -> 1
Solution in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
class Solution:
def reverseList(self, head: ListNode) -> ListNode:
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
This solution uses an iterative approach to reverse the linked list in-place. Let’s break down the algorithm:
- We initialize two pointers:
prev
(initially set to None) andcurrent
(pointing to the head of the list). - We iterate through the list using a while loop, continuing until
current
becomes None (indicating we’ve reached the end of the original list). - In each iteration:
- We store the next node in a temporary variable (
next_temp
) to avoid losing it when we change pointers. - We reverse the current node’s pointer to point to the previous node.
- We move
prev
andcurrent
one step forward in the list.
- We store the next node in a temporary variable (
- After the loop,
prev
will be pointing to the last node of the original list, which is now the head of our reversed list.
This problem demonstrates several important concepts:
- Linked list manipulation
- In-place algorithm (O(1) space complexity)
- Pointer manipulation
- Iterative problem-solving
Understanding and being able to implement solutions like this is crucial for success in coding interviews and for developing strong algorithmic thinking skills.
The Future of Coding Interviews and Education
As the tech industry continues to evolve, so too do the methods for assessing and developing coding talent. While platforms like LeetCode remain invaluable, there’s an ongoing debate about the effectiveness of algorithm-heavy interviews and whether they truly reflect a candidate’s ability to perform in real-world software development roles.
Looking ahead, we can expect to see several trends in coding interviews and education:
1. More Holistic Assessments
Companies are increasingly adopting multi-faceted interview processes that include not just algorithmic problem-solving, but also system design questions, behavioral interviews, and practical coding tasks that more closely mimic real work scenarios.
2. Emphasis on Soft Skills
There’s growing recognition of the importance of soft skills like communication, teamwork, and adaptability. Future interview processes and educational programs are likely to place more emphasis on these areas.
3. Continuous Learning and Adaptation
With the rapid pace of technological change, the ability to learn and adapt quickly is becoming as important as existing knowledge. Education platforms and companies may focus more on assessing and developing this adaptability.
4. Project-Based Assessments
Some companies are moving towards project-based interviews or take-home assignments that allow candidates to showcase their skills in a more realistic setting.
5. AI and Machine Learning Integration
As AI continues to advance, we can expect to see more sophisticated AI-powered tools for both learning and assessment in the coding education space.
Conclusion: Embracing the LeetCode Dream
The LeetCode dream represents more than just the desire to ace coding interviews; it embodies the pursuit of excellence in software engineering and the aspiration to work at the forefront of technology. While mastering LeetCode-style problems is undoubtedly valuable, it’s essential to view it as part of a broader journey of continuous learning and skill development.
As you embark on or continue your coding education journey, remember these key points:
- Consistency and perseverance are crucial. Small, regular efforts compound over time.
- Focus on understanding concepts rather than memorizing solutions.
- Complement your LeetCode practice with real-world projects and broader computer science knowledge.
- Embrace the community aspect of coding education, learning from and contributing to discussions with peers.
- Stay adaptable and open to new learning methods and technologies as the field evolves.
Whether your goal is to land a job at a top tech company, improve your problem-solving skills, or simply become a better programmer, platforms like LeetCode and AlgoCademy offer valuable resources to help you achieve your dreams. Remember, the journey of coding education is ongoing, and each problem solved is a step towards becoming a more skilled and confident software engineer.
As you pursue your LeetCode dream, stay curious, stay persistent, and most importantly, enjoy the process of learning and growth. The skills and mindset you develop along the way will serve you well, regardless of where your tech career takes you. Happy coding!