Mastering the Stripe Technical Interview: A Comprehensive Preparation Guide
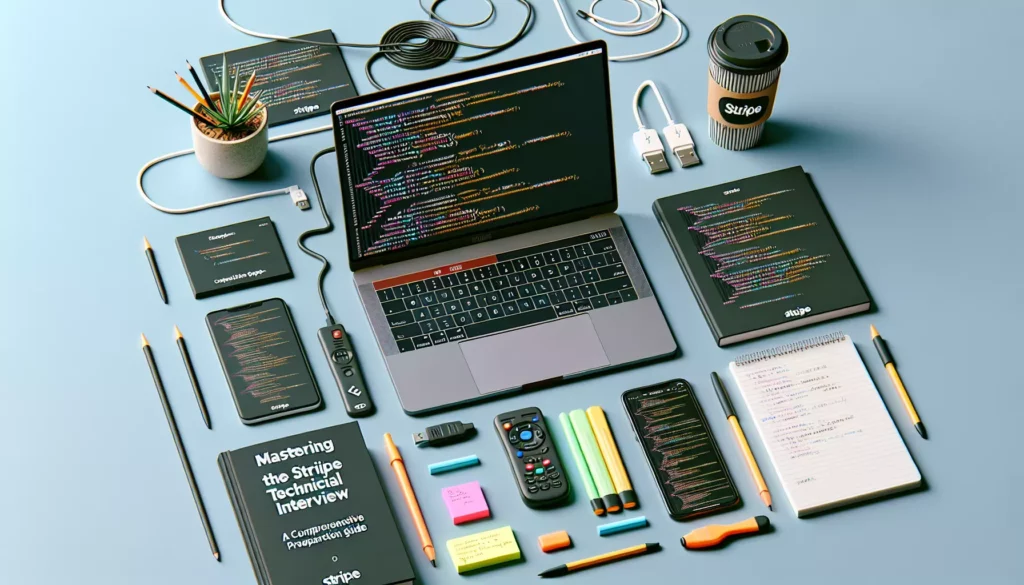
Preparing for a technical interview at Stripe can be both exciting and daunting. As one of the leading financial technology companies, Stripe is known for its rigorous interview process and high standards for engineering talent. This comprehensive guide will walk you through the key aspects of the Stripe technical interview, providing valuable insights and strategies to help you succeed.
Understanding the Stripe Interview Process
Before diving into specific preparation strategies, it’s essential to understand the overall structure of Stripe’s interview process. Typically, it consists of the following stages:
- Initial phone screen or online assessment
- Technical phone interview
- On-site interviews (or virtual equivalent)
- Final decision
Each stage is designed to evaluate different aspects of your technical skills, problem-solving abilities, and cultural fit within the company.
Key Areas to Focus On
When preparing for a Stripe technical interview, it’s crucial to concentrate on the following areas:
1. Data Structures and Algorithms
Stripe, like many top tech companies, places a strong emphasis on candidates’ understanding of fundamental data structures and algorithms. You should be comfortable with:
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Sorting and searching algorithms
- Dynamic programming
Practice implementing these data structures from scratch and solving problems that utilize them efficiently.
2. System Design
For more senior positions, system design questions are likely to be a significant part of the interview process. Be prepared to discuss:
- Scalability and performance optimization
- Distributed systems
- Database design and management
- API design
- Microservices architecture
- Load balancing and caching strategies
Familiarize yourself with Stripe’s products and think about how you would design systems to handle large-scale financial transactions securely and efficiently.
3. Coding Proficiency
Stripe values clean, efficient, and well-structured code. During your interview, you may be asked to:
- Write code on a whiteboard or in a shared coding environment
- Debug and optimize existing code
- Explain your thought process and decision-making
Practice coding without the aid of an IDE to simulate interview conditions. Focus on writing clear, readable code that you can explain easily.
4. Problem-Solving Skills
Interviewers at Stripe are particularly interested in your approach to problem-solving. They want to see how you:
- Break down complex problems into manageable components
- Analyze trade-offs between different solutions
- Optimize for both time and space complexity
- Handle edge cases and error scenarios
Practice thinking out loud as you solve problems to give interviewers insight into your thought process.
5. Financial Technology Knowledge
While not always explicitly tested, having a good understanding of the fintech industry and Stripe’s role in it can be beneficial. Familiarize yourself with:
- Payment processing systems
- Financial regulations and compliance
- Security practices in handling sensitive financial data
- Stripe’s products and services
This knowledge can help you provide context-relevant answers and demonstrate your genuine interest in the company.
Practical Preparation Strategies
Now that we’ve covered the key areas to focus on, let’s discuss some practical strategies to help you prepare effectively for your Stripe technical interview.
1. Solve Coding Problems Regularly
Consistent practice is key to improving your problem-solving skills and coding speed. Here are some ways to incorporate regular practice into your preparation:
- Use platforms like LeetCode, HackerRank, or CodeSignal to solve algorithm problems daily
- Focus on medium to hard difficulty problems, as Stripe’s questions tend to be challenging
- Time yourself to improve your speed and efficiency
- Review and understand multiple solutions for each problem
Here’s an example of a typical coding problem you might encounter:
// Problem: Implement a function to reverse a linked list
// Definition for singly-linked list:
// function ListNode(val = 0, next = null) {
// this.val = val;
// this.next = next;
// }
/**
* @param {ListNode} head
* @return {ListNode}
*/
function reverseLinkedList(head) {
let prev = null;
let current = head;
while (current !== null) {
let nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
This solution demonstrates an in-place reversal of a linked list, which is an efficient approach both in terms of time (O(n)) and space (O(1)) complexity.
2. Study System Design Concepts
For system design preparation:
- Read books like “Designing Data-Intensive Applications” by Martin Kleppmann
- Study real-world system architectures of large-scale applications
- Practice designing systems on a whiteboard, focusing on scalability and reliability
- Familiarize yourself with Stripe’s architecture and design principles, if publicly available
Here’s a high-level example of how you might approach a system design question related to Stripe’s domain:
// System Design: High-level architecture for a payment processing system
1. User Interface (Web/Mobile App)
|
2. Load Balancer
|
3. API Gateway
|
4. Microservices:
- Authentication Service
- Payment Processing Service
- Fraud Detection Service
- Reporting Service
|
5. Message Queue (e.g., Kafka)
|
6. Data Storage:
- Relational Database (e.g., PostgreSQL for transactional data)
- NoSQL Database (e.g., MongoDB for user profiles)
- Data Warehouse (e.g., Snowflake for analytics)
|
7. Caching Layer (e.g., Redis)
|
8. External Integrations:
- Banks and Credit Card Networks
- Compliance and Regulatory Systems
This simplified diagram outlines the key components of a scalable and secure payment processing system. Be prepared to dive into the details of each component and explain how they interact.
3. Mock Interviews
Practicing with mock interviews can significantly improve your performance and confidence. Consider these options:
- Use platforms like Pramp or InterviewBit for peer mock interviews
- Ask a friend or colleague in the industry to conduct a mock interview with you
- Record yourself solving problems out loud to review your communication style
During mock interviews, focus on:
- Clearly communicating your thought process
- Asking clarifying questions before diving into solutions
- Managing your time effectively
- Handling hints and feedback gracefully
4. Develop a Structured Approach
Having a structured approach to problem-solving can help you stay organized during the interview. Here’s a recommended framework:
- Clarify the problem and requirements
- Discuss and analyze potential approaches
- Choose an approach and explain your reasoning
- Write pseudocode or outline the solution
- Implement the solution
- Test and debug your code
- Analyze time and space complexity
- Discuss potential optimizations or alternative solutions
Practice applying this framework to various problems to make it second nature during the actual interview.
5. Stay Updated with Stripe’s Technology Stack
Stripe uses a diverse set of technologies in its infrastructure. While you’re not expected to be an expert in all of them, familiarity with their tech stack can be advantageous. Some key technologies used by Stripe include:
- Programming Languages: Ruby, Go, JavaScript, Python
- Frameworks: Ruby on Rails, React
- Databases: MySQL, Redis
- Infrastructure: AWS, Kubernetes
Stay updated with Stripe’s engineering blog and open-source projects to gain insights into their technology choices and engineering challenges.
Common Pitfalls to Avoid
As you prepare for your Stripe technical interview, be aware of these common mistakes:
- Neglecting communication: Don’t focus solely on solving the problem; explain your thought process clearly throughout the interview.
- Rushing to code: Take time to understand the problem and consider different approaches before starting to write code.
- Ignoring edge cases: Always consider and discuss potential edge cases and how your solution handles them.
- Failing to test: After implementing a solution, walk through it with test cases to catch any bugs or oversights.
- Not asking for help: If you’re stuck, don’t hesitate to ask for hints or clarification. It’s better to make progress with some guidance than to remain stuck.
Final Preparation Tips
As your interview date approaches, keep these final tips in mind:
- Review Stripe’s core values and how they align with your own professional goals
- Prepare thoughtful questions about the role, team, and company culture
- Get a good night’s sleep before the interview
- Test your technical setup if it’s a virtual interview
- Have a pen and paper ready for any system design or whiteboarding questions
- Stay calm and remember that the interview is also an opportunity for you to evaluate if Stripe is the right fit for you
Conclusion
Preparing for a Stripe technical interview requires dedication, practice, and a well-rounded approach. By focusing on core computer science concepts, honing your problem-solving skills, and understanding Stripe’s unique position in the fintech industry, you’ll be well-equipped to showcase your abilities during the interview process.
Remember that the goal of the interview is not just to test your technical skills, but also to assess how you approach challenges, communicate your ideas, and collaborate with others. Stay confident, be yourself, and let your passion for technology and innovation shine through.
With thorough preparation and the right mindset, you’ll be well on your way to impressing your interviewers and potentially landing a rewarding role at one of the most innovative companies in the financial technology sector. Good luck with your Stripe technical interview!