Mastering Adobe Technical Interviews: A Comprehensive Preparation Guide
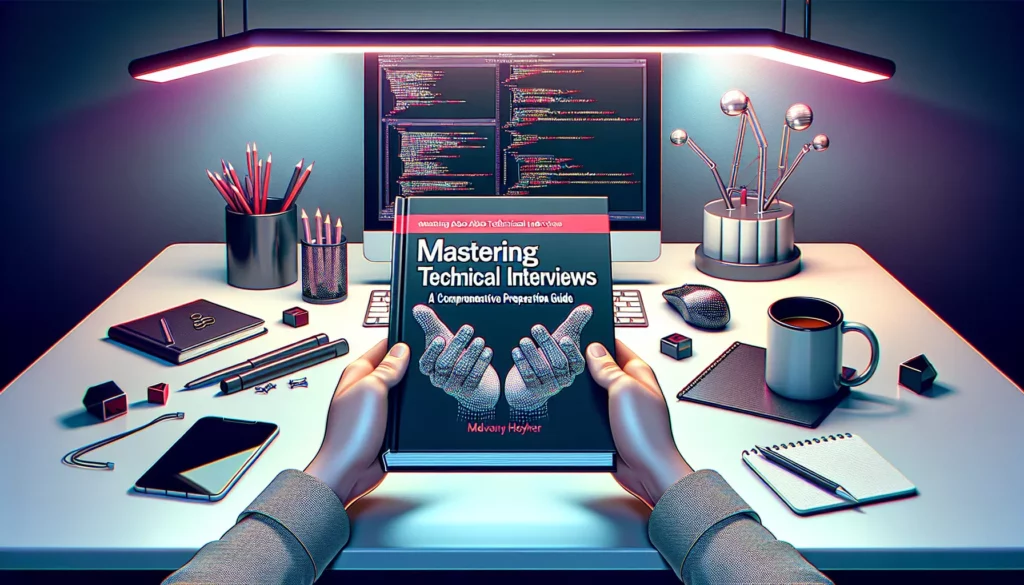
Preparing for a technical interview at Adobe can be both exciting and challenging. As one of the world’s leading software companies, Adobe is known for its innovative products and cutting-edge technology. To succeed in their interview process, you’ll need a combination of technical skills, problem-solving abilities, and a good understanding of Adobe’s products and culture. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Adobe technical interview.
Understanding Adobe’s Interview Process
Before diving into the specific preparation strategies, it’s essential to understand Adobe’s typical interview process. While it may vary depending on the position and team, the general structure often includes:
- Initial phone screening with a recruiter
- Technical phone interview or online coding assessment
- On-site interviews (or virtual equivalent) consisting of:
- Multiple technical interviews
- Behavioral interviews
- System design discussion (for more senior positions)
Key Areas to Focus On
To prepare effectively for an Adobe technical interview, concentrate on the following areas:
1. Data Structures and Algorithms
A solid grasp of fundamental data structures and algorithms is crucial. Adobe, like many tech giants, often tests candidates on their ability to solve algorithmic problems efficiently. Focus on:
- Arrays and Strings
- Linked Lists
- Trees and Graphs
- Stacks and Queues
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Recursion
2. Programming Languages
While Adobe uses various programming languages depending on the team and project, it’s important to be proficient in at least one of the following:
- C++
- Java
- JavaScript
- Python
Be prepared to write clean, efficient code during your interview. Practice implementing algorithms and data structures in your chosen language.
3. Object-Oriented Programming (OOP)
Adobe’s software is built on OOP principles, so a strong understanding of OOP concepts is essential. Be ready to discuss and implement:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Design patterns
4. Web Technologies
Depending on the position, you may need to demonstrate knowledge of web technologies. Key areas include:
- HTML5 and CSS3
- JavaScript and popular frameworks (e.g., React, Angular, Vue.js)
- RESTful APIs
- Web security principles
5. Adobe-Specific Technologies
Familiarize yourself with Adobe’s products and technologies relevant to the position you’re applying for. This might include:
- Adobe Creative Cloud suite (Photoshop, Illustrator, InDesign, etc.)
- Adobe Experience Cloud (Analytics, Target, Campaign, etc.)
- Adobe Document Cloud (Acrobat, Sign)
- Adobe’s open-source projects (e.g., PhoneGap, Apache Flex)
6. System Design
For more senior positions, you may be asked to participate in a system design discussion. Be prepared to:
- Design scalable architectures
- Discuss trade-offs between different design choices
- Explain how you would handle large amounts of data or high traffic
Preparing for the Technical Interview
Now that we’ve covered the key areas to focus on, let’s dive into specific strategies to prepare for your Adobe technical interview.
1. Review Computer Science Fundamentals
Start by refreshing your knowledge of core computer science concepts. This includes:
- Time and space complexity analysis
- Big O notation
- Memory management
- Concurrency and multithreading
- Operating system basics
- Database fundamentals (SQL and NoSQL)
2. Practice Coding Problems
Consistent practice is key to performing well in coding interviews. Here’s how to approach this:
- Solve problems on platforms like LeetCode, HackerRank, or CodeSignal
- Focus on medium to hard difficulty problems
- Time yourself to simulate interview conditions
- Practice explaining your thought process out loud as you solve problems
- Review and learn from optimal solutions after solving a problem
Here’s an example of a typical coding problem you might encounter:
Problem: Implement a function to find the longest palindromic substring in a given string.
Input: "babad"
Output: "bab" or "aba" (both are valid)
Input: "cbbd"
Output: "bb"
def longest_palindromic_substring(s):
# Your implementation here
pass
# Test cases
print(longest_palindromic_substring("babad")) # Expected: "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # Expected: "bb"
3. Mock Interviews
Participate in mock interviews to get comfortable with the interview process:
- Use platforms like Pramp or InterviewBit for peer mock interviews
- Ask a friend or colleague to conduct a mock interview
- Practice whiteboarding or using a shared code editor
- Get feedback on your communication and problem-solving approach
4. Study Adobe’s Products and Technologies
Demonstrating knowledge of Adobe’s ecosystem can set you apart:
- Read Adobe’s official documentation and developer resources
- Experiment with Adobe’s APIs and SDKs
- Stay updated on Adobe’s latest product releases and technology trends
- Understand Adobe’s cloud offerings and how they integrate
5. Prepare for Behavioral Questions
While technical skills are crucial, Adobe also values soft skills and cultural fit. Be ready to discuss:
- Your past projects and experiences
- How you handle challenges and conflicts
- Your approach to teamwork and collaboration
- Your passion for technology and innovation
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions.
Common Interview Questions at Adobe
While specific questions will vary, here are some types of questions you might encounter in an Adobe technical interview:
1. Coding and Algorithm Questions
- Implement a data structure (e.g., a hash table or a binary search tree)
- Write a function to reverse a linked list
- Find the kth largest element in an unsorted array
- Implement a LRU (Least Recently Used) cache
- Detect a cycle in a linked list
2. System Design Questions
- Design a distributed file storage system
- How would you design Adobe’s Creative Cloud sync feature?
- Design a real-time collaboration feature for a document editing application
- How would you implement a scalable image processing pipeline?
3. Object-Oriented Design Questions
- Design a class hierarchy for shapes in a graphics application
- Implement a simple version control system
- Design the classes and interfaces for a multi-player game
4. Adobe-Specific Questions
- Explain how you would implement a feature in Adobe Photoshop (e.g., layer blending modes)
- Discuss the challenges in implementing real-time collaboration in Adobe XD
- How would you optimize PDF rendering performance in Adobe Acrobat?
5. Behavioral Questions
- Tell me about a time when you had to learn a new technology quickly.
- Describe a situation where you had to work with a difficult team member.
- How do you stay updated with the latest trends in technology?
- Give an example of a project where you had to meet a tight deadline.
Tips for Interview Day
As your interview day approaches, keep these tips in mind:
1. Technical Preparation
- Review your chosen programming language’s syntax and standard libraries
- Be prepared to code on a whiteboard or in a shared online editor
- Have a good understanding of time and space complexity for common algorithms
2. Communication
- Clearly explain your thought process as you solve problems
- Ask clarifying questions when needed
- Be open to hints and guidance from the interviewer
3. Problem-Solving Approach
- Start with a high-level approach before diving into coding
- Consider edge cases and potential optimizations
- If stuck, try to break down the problem into smaller parts
4. Behavioral Aspects
- Show enthusiasm for Adobe’s products and technologies
- Demonstrate your ability to work in a team
- Highlight your problem-solving skills and adaptability
5. Questions for the Interviewer
Prepare thoughtful questions to ask your interviewer, such as:
- What are the biggest challenges facing your team right now?
- How does Adobe foster innovation within the company?
- Can you tell me about a recent project your team worked on?
- What opportunities are there for professional growth and learning at Adobe?
After the Interview
Once your interview is complete:
- Send a thank-you email to your interviewer(s) and recruiter
- Reflect on the experience and note areas for improvement
- Be patient while waiting for a response
- If you don’t get the position, ask for feedback to help you improve for future interviews
Conclusion
Preparing for an Adobe technical interview requires dedication, practice, and a broad understanding of computer science concepts and Adobe’s technologies. By focusing on the key areas we’ve discussed and following the preparation strategies outlined in this guide, you’ll be well-equipped to showcase your skills and land that dream job at Adobe.
Remember, the interview process is not just about demonstrating your technical prowess but also about showing your passion for technology, your ability to learn and adapt, and your potential to contribute to Adobe’s innovative culture. Stay confident, be yourself, and let your skills and enthusiasm shine through.
Good luck with your Adobe technical interview!