Mastering Airbnb Technical Interview Prep: A Comprehensive Guide
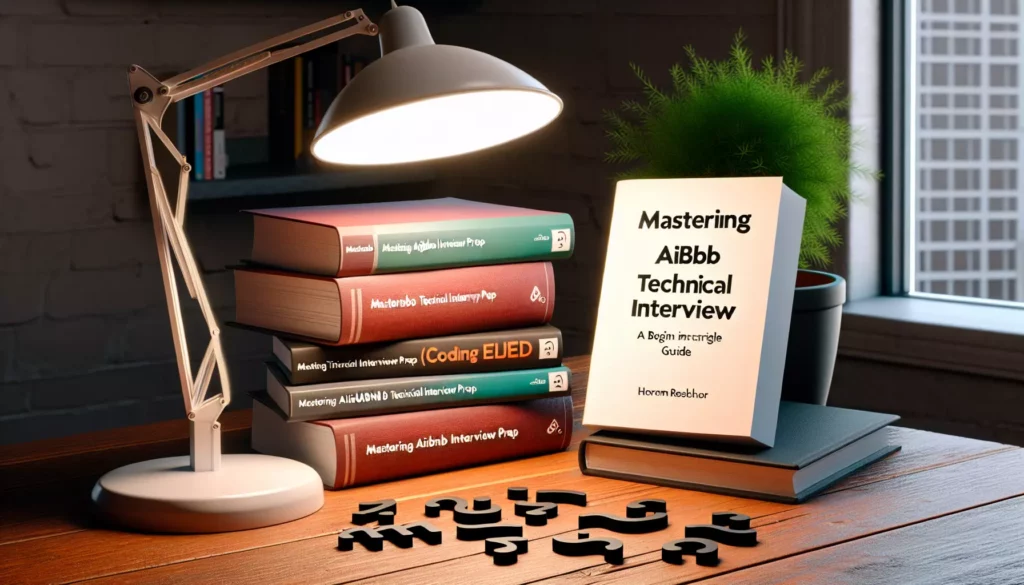
As one of the leading tech companies in the hospitality and travel industry, Airbnb has become a coveted destination for software engineers and developers. Landing a job at Airbnb not only offers the opportunity to work on innovative projects but also provides a chance to be part of a company that’s reshaping how people travel and experience new places. However, the path to securing a position at Airbnb is challenging, particularly when it comes to the technical interview process. In this comprehensive guide, we’ll dive deep into Airbnb technical interview prep, equipping you with the knowledge and strategies you need to excel.
Understanding the Airbnb Interview Process
Before we delve into the specific preparation strategies, it’s crucial to understand the overall structure of Airbnb’s interview process. Typically, it consists of several stages:
- Initial Phone Screen: This is usually a brief conversation with a recruiter to assess your background and interest in the role.
- Technical Phone Interview: This involves coding and problem-solving questions, often conducted via a shared coding platform.
- On-site Interviews: These consist of multiple rounds, including coding interviews, system design discussions, and behavioral questions.
- Final Decision: Based on the feedback from all interviewers, a hiring decision is made.
Our focus in this guide will be primarily on preparing for the technical aspects of these interviews.
Key Areas to Focus On
Airbnb’s technical interviews cover a wide range of topics, but some key areas consistently come up:
1. Data Structures and Algorithms
A solid grasp of fundamental data structures and algorithms is crucial. You should be comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Recursion
2. Problem-Solving Skills
Airbnb places a high emphasis on problem-solving abilities. You’ll be expected to:
- Understand the problem quickly
- Ask clarifying questions
- Propose and discuss multiple approaches
- Analyze time and space complexity
- Implement a solution efficiently
3. System Design
For more senior positions, system design questions are common. You should be familiar with:
- Scalability concepts
- Database design
- Caching mechanisms
- Load balancing
- Microservices architecture
4. Coding Proficiency
You’ll be expected to write clean, efficient, and bug-free code. Focus on:
- Code organization and structure
- Proper naming conventions
- Error handling
- Testing and edge cases
Preparation Strategies
Now that we’ve outlined the key areas, let’s dive into specific strategies to help you prepare effectively for Airbnb’s technical interviews.
1. Master the Fundamentals
Start by ensuring you have a strong foundation in computer science fundamentals. Review your knowledge of data structures, algorithms, and their time and space complexities. Resources like “Introduction to Algorithms” by Cormen et al. or online platforms like Coursera and edX offer comprehensive courses on these topics.
2. Practice Coding Problems
Consistent practice is key to improving your problem-solving skills. Utilize platforms like LeetCode, HackerRank, or CodeSignal to solve a variety of coding problems. Aim to solve at least 2-3 problems daily, focusing on different difficulty levels and problem types.
Here’s an example of a typical coding problem you might encounter:
// Problem: Implement a function to find the longest palindromic substring in a given string.
// Example: Input: "babad" Output: "bab" or "aba"
function longestPalindrome(s) {
if (!s || s.length < 2) {
return s;
}
let start = 0;
let maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
if (right - left + 1 > maxLength) {
start = left;
maxLength = right - left + 1;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
expandAroundCenter(i, i); // Odd length palindromes
expandAroundCenter(i, i + 1); // Even length palindromes
}
return s.substring(start, start + maxLength);
}
// Test the function
console.log(longestPalindrome("babad")); // Output: "bab" or "aba"
console.log(longestPalindrome("cbbd")); // Output: "bb"
This problem tests your understanding of string manipulation, dynamic programming concepts, and efficiency in implementation.
3. Study Airbnb-Specific Questions
While it’s important to have a broad knowledge base, it’s also beneficial to focus on questions that have been reported in actual Airbnb interviews. Websites like Glassdoor, LeetCode, and Blind often have user-reported interview questions. Some Airbnb-specific problems include:
- Implement a calendar booking system
- Design a rate limiter
- Implement an autocomplete feature
- Design an algorithm for matching hosts and guests
4. Improve Your System Design Skills
For system design preparation, study how popular systems work and practice designing scalable systems. Some topics to focus on include:
- Designing a distributed cache
- Implementing a search functionality for Airbnb listings
- Designing a notification system
- Creating a recommendation system for travel experiences
Here’s a high-level example of how you might approach a system design question for Airbnb:
// System Design: Design a simplified version of Airbnb's search functionality
1. Requirements:
- Users should be able to search for listings based on location, dates, and number of guests
- The system should return relevant results quickly
- It should handle high traffic and be scalable
2. High-level design:
- Web servers to handle user requests
- Application servers for business logic
- Database to store listing information
- Search service (e.g., Elasticsearch) for efficient searching
- Caching layer (e.g., Redis) to store frequently accessed data
3. Data model:
Listing {
id: string,
host_id: string,
location: {
latitude: float,
longitude: float,
city: string,
country: string
},
price: float,
amenities: string[],
availability: date[],
max_guests: int
}
4. API design:
searchListings(location, check_in, check_out, guests) -> List<Listing>
5. Scalability considerations:
- Use a load balancer to distribute traffic across multiple web servers
- Implement database sharding based on geographical location
- Use a CDN to serve static content
- Implement caching at various levels (e.g., application-level caching, database query caching)
6. Additional features:
- Implement a recommendation system based on user preferences and booking history
- Add real-time availability updates using websockets
- Implement a review and rating system for listings
5. Mock Interviews
Participate in mock interviews to simulate the actual interview experience. Platforms like Pramp or interviewing.io offer peer-to-peer mock interviews. Additionally, consider asking friends or mentors in the industry to conduct mock interviews for you.
6. Understand Airbnb’s Culture and Values
While technical skills are crucial, Airbnb also values cultural fit. Familiarize yourself with Airbnb’s core values and be prepared to discuss how you align with them. Some of Airbnb’s key values include:
- Champion the Mission
- Be a Host
- Embrace the Adventure
- Be a Cereal Entrepreneur
The Interview Day: What to Expect
On the day of your technical interview with Airbnb, whether it’s a phone screen or an on-site interview, here’s what you can typically expect:
1. Introduction and Icebreaker
The interviewer will likely start with a brief introduction and maybe a few casual questions to help you feel at ease. Use this time to calm your nerves and build a rapport with the interviewer.
2. Technical Questions
The bulk of the interview will consist of technical questions. These may include:
- Coding problems: You’ll be asked to solve one or more coding problems, either on a whiteboard (for on-site interviews) or via a shared coding platform (for remote interviews).
- Algorithm discussions: You might be asked to explain the logic behind certain algorithms or to optimize a given solution.
- System design questions: For more senior positions, you may be asked to design a system or feature similar to what Airbnb uses.
3. Behavioral Questions
Expect questions about your past experiences, how you handle challenges, and how you work in a team. Some example questions might be:
- “Tell me about a time when you had to deal with a difficult team member.”
- “How do you approach learning new technologies?”
- “Describe a project you’re particularly proud of and why.”
4. Q&A Session
At the end of the interview, you’ll usually have the opportunity to ask questions. Have some thoughtful questions prepared about the role, team, or company culture.
Tips for Success
To maximize your chances of success in your Airbnb technical interview, keep these tips in mind:
1. Think Aloud
As you work through problems, verbalize your thought process. This gives the interviewer insight into how you approach problems and allows them to provide hints if needed.
2. Clarify the Problem
Before diving into a solution, make sure you fully understand the problem. Ask clarifying questions and discuss any assumptions you’re making.
3. Consider Multiple Approaches
Don’t just go with the first solution that comes to mind. Consider multiple approaches and discuss their pros and cons before deciding on the best one.
4. Analyze Complexity
After proposing a solution, analyze its time and space complexity. Be prepared to discuss potential optimizations.
5. Write Clean Code
Even under pressure, strive to write clean, well-organized code. Use meaningful variable names, proper indentation, and add comments where necessary.
6. Test Your Code
After implementing a solution, walk through it with a few test cases, including edge cases. This demonstrates thoroughness and attention to detail.
7. Stay Calm and Positive
If you get stuck, don’t panic. Take a deep breath, and calmly work through the problem. It’s okay to ask for hints if you’re truly stuck.
After the Interview
Once your interview is complete, there are a few final steps to consider:
1. Send a Thank You Note
Send a brief email thanking the interviewer for their time and reiterating your interest in the position.
2. Reflect on the Experience
Take some time to reflect on what went well and what you could improve. This will help you in future interviews, regardless of the outcome.
3. Follow Up
If you don’t hear back within the timeframe specified by the recruiter, it’s appropriate to send a polite follow-up email inquiring about the status of your application.
Conclusion
Preparing for a technical interview at Airbnb is a challenging but rewarding process. By focusing on strong fundamentals, practicing regularly, and understanding the company’s values and interview process, you can significantly increase your chances of success. Remember, the goal of these interviews is not just to test your technical skills, but also to assess how you think, how you communicate, and how you might fit into the Airbnb team.
Whether you’re a seasoned developer or just starting your career, the key is to approach the preparation process with dedication, curiosity, and a growth mindset. Every problem you solve, every system you design, and every mock interview you participate in brings you one step closer to your goal.
Good luck with your Airbnb technical interview prep! With the right preparation and mindset, you’ll be well-equipped to showcase your skills and land that dream job at one of the most innovative companies in the tech industry.