Netflix Technical Interview Prep: A Comprehensive Guide
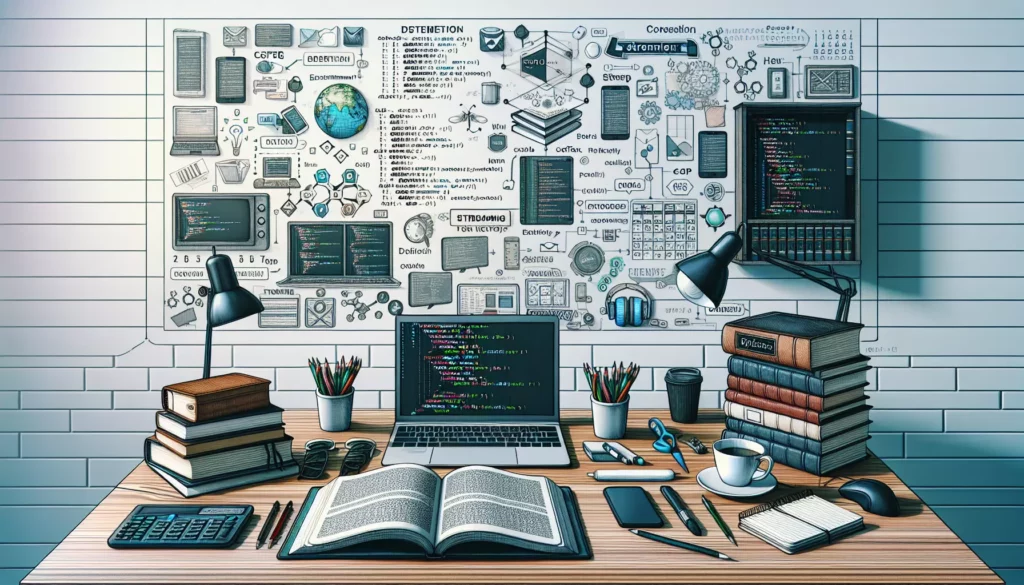
If you’re gearing up for a technical interview at Netflix, you’re in for an exciting and challenging experience. Netflix, being one of the leading streaming services and a major player in the tech industry, has a rigorous interview process designed to identify top talent. In this comprehensive guide, we’ll walk you through everything you need to know to prepare for your Netflix technical interview, from understanding the interview process to mastering key concepts and practicing essential skills.
Understanding the Netflix Interview Process
Before diving into the technical aspects, it’s crucial to understand the structure of Netflix’s interview process. Typically, it consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to discuss your background and the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call.
- On-site Interviews: A series of face-to-face interviews (or virtual equivalents) with different team members.
- System Design Interview: For more senior positions, you may be asked to design a large-scale system.
- Behavioral Interviews: Discussions about your past experiences and how you handle various situations.
Each stage is designed to assess different aspects of your skills and fit for the role. Let’s focus on preparing for the technical components of these interviews.
Key Areas to Focus On
Netflix’s technical interviews cover a wide range of topics, but some key areas consistently come up:
1. Data Structures and Algorithms
A solid understanding of data structures and algorithms is fundamental. You should be comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Sorting and Searching algorithms
- Dynamic Programming
- Recursion
2. System Design
For senior roles, you’ll likely face questions about designing large-scale systems. Key topics include:
- Scalability
- Load Balancing
- Caching
- Database Sharding
- Microservices Architecture
- Content Delivery Networks (CDNs)
3. Object-Oriented Programming (OOP)
Netflix uses a variety of programming languages, but a strong grasp of OOP principles is essential. Focus on:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Design Patterns
4. Coding Best Practices
Netflix values clean, efficient, and maintainable code. Be prepared to demonstrate:
- Code organization
- Proper naming conventions
- Error handling
- Testing strategies
- Version control (Git)
Preparing for Coding Interviews
The coding interview is a crucial part of the Netflix technical interview process. Here’s how you can prepare effectively:
1. Practice Coding Problems
Regularly solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy. Focus on medium to hard difficulty problems, as Netflix interviews tend to be challenging. Pay special attention to:
- String manipulation
- Array operations
- Tree and graph traversals
- Dynamic programming
2. Mock Interviews
Conduct mock interviews with friends or use platforms that offer this service. This will help you get comfortable with explaining your thought process while coding under pressure.
3. Time Management
Practice solving problems within a time limit. In real interviews, you’ll typically have 45-60 minutes to solve 1-2 problems.
4. Explain Your Approach
Get comfortable talking through your problem-solving approach. Interviewers want to understand your thought process, not just see the final solution.
Sample Coding Problem: Netflix Recommendation Algorithm
Let’s walk through a sample problem you might encounter in a Netflix technical interview. This problem combines algorithm design with a practical application relevant to Netflix’s business.
Problem Statement: Design a simple recommendation algorithm for Netflix. Given a list of movies a user has watched and rated, recommend the top K movies from a catalog that the user is most likely to enjoy.
Here’s a Python implementation of a basic recommendation algorithm:
class Movie:
def __init__(self, id, title, genres):
self.id = id
self.title = title
self.genres = genres
class User:
def __init__(self, id):
self.id = id
self.watched_movies = {} # movie_id: rating
def recommend_movies(user, catalog, k):
# Calculate genre preferences
genre_scores = {}
for movie_id, rating in user.watched_movies.items():
movie = next(m for m in catalog if m.id == movie_id)
for genre in movie.genres:
if genre not in genre_scores:
genre_scores[genre] = 0
genre_scores[genre] += rating
# Normalize genre scores
total_score = sum(genre_scores.values())
for genre in genre_scores:
genre_scores[genre] /= total_score
# Score unwatched movies
unwatched_movies = [m for m in catalog if m.id not in user.watched_movies]
movie_scores = []
for movie in unwatched_movies:
score = sum(genre_scores.get(genre, 0) for genre in movie.genres)
movie_scores.append((movie, score))
# Sort and return top K recommendations
movie_scores.sort(key=lambda x: x[1], reverse=True)
return [movie for movie, score in movie_scores[:k]]
# Example usage
catalog = [
Movie(1, "The Matrix", ["Sci-Fi", "Action"]),
Movie(2, "Inception", ["Sci-Fi", "Thriller"]),
Movie(3, "The Shawshank Redemption", ["Drama"]),
Movie(4, "Pulp Fiction", ["Crime", "Drama"]),
Movie(5, "Avengers: Endgame", ["Action", "Sci-Fi"]),
]
user = User(1)
user.watched_movies = {1: 5, 3: 4} # User watched and rated The Matrix and The Shawshank Redemption
recommendations = recommend_movies(user, catalog, 2)
for movie in recommendations:
print(f"Recommended: {movie.title}")
This algorithm does the following:
- Calculates genre preferences based on the user’s watch history and ratings.
- Normalizes these preferences to create a user profile.
- Scores unwatched movies based on how well they match the user’s profile.
- Returns the top K highest-scoring movies as recommendations.
While this is a simplified version, it demonstrates key concepts you might be asked to implement or discuss in a Netflix interview, such as:
- Working with custom data structures (Movie and User classes)
- Handling and processing user data
- Implementing a scoring algorithm
- Sorting and selecting top results
System Design for Netflix
For more senior positions, you may be asked to design a system component of Netflix. Let’s consider a high-level design for Netflix’s video streaming system.
Key Components:
- Content Delivery Network (CDN): Distributed network of servers to deliver content efficiently to users worldwide.
- Transcoding Service: Converts video files into multiple formats and qualities for different devices and network conditions.
- Metadata Service: Manages information about movies and TV shows (titles, descriptions, cast, etc.).
- User Profile Service: Handles user authentication, preferences, and watch history.
- Recommendation Engine: Generates personalized content recommendations for users.
- Streaming Service: Manages video playback, including adaptive bitrate streaming.
- Analytics Service: Collects and processes user behavior data for improving recommendations and content decisions.
System Architecture Diagram:
+-------------+ +----------------+ +------------------+
| Client |-----| Load Balancer |-----| API Gateway |
+-------------+ +----------------+ +------------------+
|
+------------------+ |
| CDN |<----------+
+------------------+ |
^ |
| v
+------------------+ +------------------+ +------------------+
| Transcoding | | Streaming | | User Profile |
| Service | | Service | | Service |
+------------------+ +------------------+ +------------------+
^ ^ ^
| | |
v v v
+------------------+ +------------------+ +------------------+
| Content | | Metadata | | Recommendation |
| Storage | | Service | | Engine |
+------------------+ +------------------+ +------------------+
^
|
v
+------------------+
| Analytics |
| Service |
+------------------+
In a system design interview, you’d be expected to discuss:
- How each component scales
- Data flow between components
- Handling peak loads
- Ensuring high availability and fault tolerance
- Optimizing for global distribution
- Security considerations
Behavioral Interview Preparation
While technical skills are crucial, Netflix also places a high value on cultural fit and soft skills. Prepare for behavioral questions by reflecting on your past experiences and how they demonstrate Netflix’s cultural values:
- Judgment: Be ready to discuss situations where you made difficult decisions.
- Communication: Prepare examples of how you’ve effectively communicated complex ideas.
- Impact: Highlight projects where you’ve made a significant impact.
- Curiosity: Show your passion for learning and staying updated with new technologies.
- Innovation: Discuss times when you’ve come up with creative solutions to problems.
- Courage: Prepare examples of when you’ve taken calculated risks or stood up for your ideas.
- Passion: Express your enthusiasm for Netflix’s mission and the role you’re applying for.
- Selflessness: Demonstrate how you’ve contributed to team success.
- Inclusion: Show your commitment to fostering a diverse and inclusive work environment.
Final Tips for Success
- Know Netflix’s Tech Stack: Familiarize yourself with technologies Netflix uses, such as Java, Python, Node.js, and AWS.
- Understand Netflix’s Business: Be prepared to discuss how your role contributes to Netflix’s overall business goals.
- Practice Whiteboarding: Even in virtual interviews, you may be asked to share your screen and code in real-time.
- Ask Thoughtful Questions: Prepare questions that demonstrate your interest in the role and the company.
- Stay Calm: Remember, interviewers are assessing how you approach problems, not just whether you get the perfect answer.
- Follow-up: After the interview, send a thank-you note reiterating your interest in the position.
Conclusion
Preparing for a Netflix technical interview requires a combination of strong coding skills, system design knowledge, and cultural alignment. By focusing on the areas outlined in this guide and consistently practicing, you’ll be well-equipped to showcase your skills and land that dream job at Netflix.
Remember, the key to success is not just about having the right answers, but demonstrating your problem-solving approach, your ability to communicate complex ideas, and your passion for technology and innovation. With thorough preparation and the right mindset, you’ll be ready to tackle whatever challenges the Netflix interview process throws your way.
Good luck with your preparation, and may your Netflix interview be as binge-worthy as their content!