Ultimate Guide to Google Technical Interview Prep: Ace Your Coding Challenge
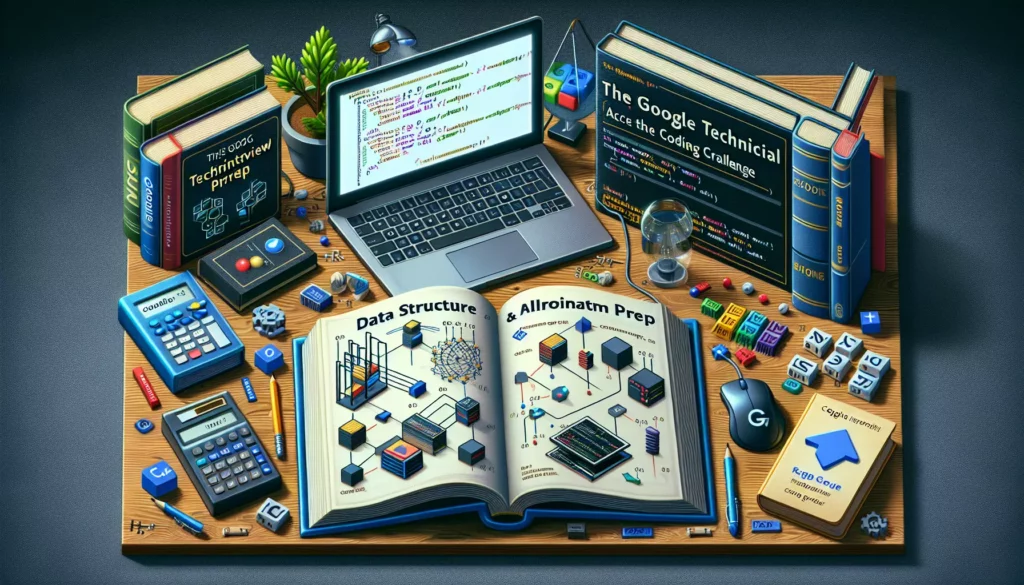
Preparing for a Google technical interview can be an intimidating process. As one of the most prestigious tech companies in the world, Google is known for its rigorous hiring standards and challenging interview process. However, with the right preparation and mindset, you can significantly increase your chances of success. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Google technical interview, from understanding the interview structure to mastering key algorithms and data structures.
Understanding the Google Interview Process
Before diving into the technical preparation, it’s crucial to understand the overall Google interview process. Typically, it consists of the following stages:
- Resume screening
- Phone screen or online coding assessment
- On-site interviews (usually 4-5 rounds)
- Hiring committee review
- Offer negotiation
The technical interview is a significant part of this process, usually occurring during the phone screen and on-site interview stages. These interviews focus on assessing your problem-solving skills, coding ability, and technical knowledge.
Key Areas to Focus On
To prepare effectively for a Google technical interview, you should concentrate on the following areas:
1. Data Structures and Algorithms
This is the cornerstone of any technical interview at Google. You should be well-versed in the following data structures and algorithms:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Dynamic Programming
- Sorting and Searching Algorithms
- Recursion and Backtracking
- Greedy Algorithms
2. Time and Space Complexity Analysis
Understanding Big O notation and being able to analyze the time and space complexity of your solutions is crucial. Google interviewers often ask about the efficiency of your code and expect you to optimize it.
3. System Design
For more senior positions, system design questions are common. You should be familiar with concepts like:
- Scalability
- Load balancing
- Caching
- Database sharding
- Microservices architecture
4. Object-Oriented Design
Understanding OOP principles and being able to design classes and interfaces is important. Focus on:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Design patterns
5. Coding Best Practices
Google places a high value on clean, maintainable code. Make sure you’re familiar with:
- Code organization
- Naming conventions
- Error handling
- Testing
- Documentation
Mastering Data Structures and Algorithms
Let’s dive deeper into some of the most important data structures and algorithms you should know for your Google technical interview:
Arrays and Strings
Arrays and strings are fundamental data structures that appear in many coding problems. Key concepts to master include:
- Two-pointer technique
- Sliding window
- Prefix sum
Here’s an example of using the two-pointer technique to reverse a string:
def reverse_string(s):
left, right = 0, len(s) - 1
s = list(s)
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return ''.join(s)
# Example usage
print(reverse_string("hello")) # Output: "olleh"
Linked Lists
Linked lists are another common data structure in interview questions. Important techniques include:
- Fast and slow pointers
- Reversing a linked list
- Detecting cycles
Here’s an example of reversing a linked list:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
# Example usage
# Create a linked list: 1 -> 2 -> 3 -> 4 -> 5
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = ListNode(5)
# Reverse the linked list
new_head = reverse_linked_list(head)
# Print the reversed list
while new_head:
print(new_head.val, end=" ")
new_head = new_head.next
# Output: 5 4 3 2 1
Trees and Graphs
Tree and graph problems are very common in Google interviews. Key concepts include:
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Binary Search Trees (BST)
- Trie
- Union Find
Here’s an example of a depth-first search on a binary tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def dfs(root):
if not root:
return
print(root.val, end=" ")
dfs(root.left)
dfs(root.right)
# Example usage
# Create a binary tree:
# 1
# / \
# 2 3
# / \
# 4 5
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print("DFS traversal:")
dfs(root)
# Output: 1 2 4 5 3
Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems. Key concepts include:
- Memoization
- Tabulation
- State transition
Here’s an example of using dynamic programming to solve the fibonacci sequence problem:
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example usage
print(fibonacci(10)) # Output: 55
Mastering Problem-Solving Techniques
In addition to knowing data structures and algorithms, it’s crucial to develop strong problem-solving skills. Here are some techniques to improve your problem-solving abilities:
1. Understand the Problem
Before diving into coding, make sure you fully understand the problem. Ask clarifying questions if needed. Consider:
- Input and output formats
- Constraints and edge cases
- Any assumptions you can make
2. Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This can help you approach complex issues more effectively.
3. Think Out Loud
During the interview, communicate your thought process. This gives the interviewer insight into how you approach problems and allows them to provide hints if needed.
4. Start with a Brute Force Solution
Begin with the simplest solution that comes to mind, even if it’s not the most efficient. This demonstrates your problem-solving ability and provides a starting point for optimization.
5. Optimize Your Solution
After implementing a basic solution, think about how you can improve it. Consider:
- Time complexity
- Space complexity
- Edge cases
- Code readability
6. Test Your Code
Before declaring your solution complete, test it with various inputs, including edge cases. This shows attention to detail and thoroughness.
Practice, Practice, Practice
The key to success in Google technical interviews is consistent practice. Here are some ways to prepare:
1. LeetCode and HackerRank
These platforms offer a wide range of coding problems similar to those you might encounter in a Google interview. Aim to solve at least 2-3 problems daily.
2. Mock Interviews
Practice with friends or use platforms like Pramp or InterviewBit that offer mock interview services. This helps you get comfortable with the interview setting and receive feedback on your performance.
3. Review Google’s Coding Practices
Familiarize yourself with Google’s style guides and best practices. This will help you write code that aligns with Google’s standards.
4. Study Computer Science Fundamentals
Refresh your knowledge of core CS concepts, including operating systems, networks, and databases.
The Day of the Interview
On the day of your Google technical interview, keep these tips in mind:
1. Stay Calm
Remember that nervousness is normal. Take deep breaths and try to relax.
2. Listen Carefully
Pay close attention to the problem statement and any hints the interviewer might provide.
3. Communicate Clearly
Explain your thought process and approach clearly. If you’re stuck, vocalize your thinking – the interviewer may provide guidance.
4. Manage Your Time
Keep an eye on the clock and make sure you’re progressing through the problem at a reasonable pace.
5. Ask Questions
Don’t hesitate to ask for clarification if something is unclear. It’s better to ask than to make incorrect assumptions.
After the Interview
After your Google technical interview:
1. Reflect on Your Performance
Think about what went well and what you could improve. This will help you in future interviews.
2. Follow Up
Send a thank-you email to your interviewer or recruiter, expressing your continued interest in the position.
3. Keep Practicing
Regardless of the outcome, continue honing your skills. The tech industry is constantly evolving, and continuous learning is key to success.
Conclusion
Preparing for a Google technical interview is a challenging but rewarding process. By focusing on core computer science concepts, practicing problem-solving techniques, and consistently working on coding challenges, you can significantly improve your chances of success. Remember, the goal is not just to pass the interview, but to demonstrate your problem-solving skills and passion for technology.
Whether you’re a fresh graduate or an experienced professional, the key to success lies in dedicated preparation and a positive attitude. With the right approach and mindset, you can tackle even the most challenging Google interview questions with confidence.
Good luck with your preparation, and may your hard work lead you to a successful career at Google!