Mastering Online Coding Challenges: Your Gateway to Tech Success
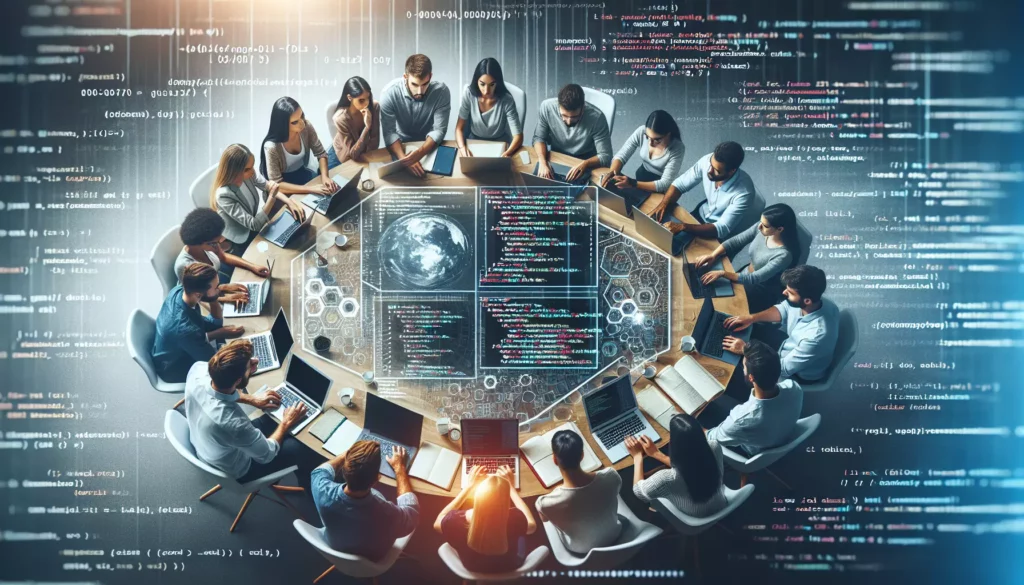
In today’s competitive tech landscape, online coding challenges have become a crucial stepping stone for aspiring software engineers and developers. Platforms like HackerRank and CodeSignal are increasingly used by companies to assess candidates’ skills, making these challenges an essential part of the job search process. This comprehensive guide will walk you through everything you need to know about online coding challenges, from their importance to strategies for success.
Understanding Online Coding Challenges
Online coding challenges are timed exercises that test a programmer’s ability to solve algorithmic problems efficiently. These challenges typically involve:
- A set of progressively difficult coding problems
- A fixed time limit for completion
- Automated grading based on predefined test cases
- Various programming languages to choose from
Popular platforms for these challenges include HackerRank, CodeSignal, LeetCode, and Codility. Each platform has its unique features, but they all share the common goal of evaluating a candidate’s coding proficiency and problem-solving skills.
The Importance of Online Coding Challenges
Coding challenges have become an integral part of the tech hiring process for several reasons:
- Objective Evaluation: They provide a standardized way to assess candidates’ skills, reducing bias in the hiring process.
- Efficiency: Companies can quickly screen a large number of applicants based on their performance in these challenges.
- Real-world Simulation: These challenges often mimic real-world problem-solving scenarios that developers encounter in their day-to-day work.
- Skill Verification: They help verify that candidates possess the necessary coding skills beyond what’s listed on their resumes.
For job seekers, excelling in these challenges can significantly boost their chances of landing interviews with top tech companies, including the coveted FAANG (Facebook, Amazon, Apple, Netflix, Google) positions.
Key Skills Tested in Online Coding Challenges
Online coding challenges are designed to evaluate a wide range of skills that are crucial for software development roles. Here are the primary areas that these challenges focus on:
1. Algorithmic Problem-Solving
This is the core skill tested in most coding challenges. Candidates are expected to:
- Understand the problem statement quickly
- Break down complex problems into smaller, manageable parts
- Devise efficient algorithms to solve the given problems
- Implement the solution in code
Problems may range from simple array manipulations to complex graph algorithms, testing the breadth and depth of a candidate’s algorithmic knowledge.
2. Time and Space Complexity Analysis
Efficiency is key in software development. Candidates are often evaluated on their ability to:
- Analyze the time complexity of their solutions
- Optimize code for better performance
- Understand and implement space-efficient solutions
- Make trade-offs between time and space complexity when necessary
A solution that passes all test cases but is inefficient may not be considered acceptable in many challenges.
3. Coding Proficiency
While problem-solving is crucial, the ability to translate thoughts into clean, working code is equally important. Challenges assess:
- Syntax knowledge and proper use of language features
- Code organization and structure
- Naming conventions and code readability
- Proper use of data structures and algorithms
Most platforms allow candidates to choose from multiple programming languages, so proficiency in at least one mainstream language is essential.
4. Attention to Detail
Online coding challenges often include edge cases and special scenarios to test a candidate’s thoroughness. This includes:
- Handling invalid inputs
- Considering boundary conditions
- Dealing with empty or null values
- Ensuring the solution works for all possible inputs within the given constraints
5. Time Management
Given the timed nature of these challenges, effective time management is crucial. Candidates must:
- Quickly assess the difficulty of each problem
- Prioritize problems based on their complexity and point value
- Allocate appropriate time for coding, testing, and optimization
- Know when to move on from a particularly challenging problem
Preparing for Online Coding Challenges
Success in online coding challenges doesn’t come overnight. It requires consistent practice and a structured approach to learning. Here’s a comprehensive strategy to help you prepare effectively:
1. Master the Fundamentals
Before diving into complex problems, ensure you have a solid grasp of fundamental concepts:
- Data Structures: Arrays, Linked Lists, Stacks, Queues, Trees, Graphs, Hash Tables
- Algorithms: Sorting, Searching, Recursion, Dynamic Programming, Greedy Algorithms
- Time and Space Complexity Analysis
- Basic mathematical concepts and problem-solving techniques
Resources like AlgoCademy offer structured learning paths that can help you build a strong foundation in these areas.
2. Practice Regularly
Consistency is key when it comes to improving your problem-solving skills:
- Set aside dedicated time each day for coding practice
- Start with easier problems and gradually increase difficulty
- Use platforms like LeetCode, HackerRank, or CodeSignal for daily practice
- Participate in coding contests to simulate the pressure of timed challenges
3. Learn from Solutions
After solving a problem (or if you’re stuck):
- Review the official solution provided by the platform
- Study solutions submitted by other users, especially highly-rated ones
- Understand different approaches to the same problem
- Analyze the time and space complexity of various solutions
4. Focus on Problem-Solving Patterns
Many coding problems follow common patterns. Familiarize yourself with these patterns:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a LinkedList
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Understanding these patterns can help you approach a wide variety of problems more efficiently.
5. Improve Your Coding Speed
Speed is crucial in timed challenges. Here’s how to improve:
- Practice typing code quickly and accurately
- Learn keyboard shortcuts for your preferred IDE or code editor
- Create templates for common code structures (e.g., class definitions, main functions)
- Familiarize yourself with the standard library of your chosen programming language
6. Mock Interviews and Peer Review
Simulate real interview conditions:
- Use platforms that offer mock interviews with real people
- Practice explaining your thought process while coding (think aloud)
- Ask friends or peers to review your code and provide feedback
- Join coding communities or study groups for collaborative learning
Strategies for Success During the Challenge
When you’re in the midst of an online coding challenge, time is of the essence. Here are some strategies to help you perform at your best:
1. Read and Understand the Problem Thoroughly
Before you start coding:
- Read the problem statement carefully, multiple times if necessary
- Identify the input format and constraints
- Understand what the expected output should be
- Look for any special cases or conditions mentioned in the problem
2. Plan Before You Code
Resist the urge to start coding immediately:
- Spend a few minutes thinking about the approach
- Consider different algorithms that could solve the problem
- Mentally walk through your solution with a simple example
- If allowed, jot down a quick pseudocode or flowchart
3. Start with a Brute Force Solution
If you’re stuck or short on time:
- Implement a simple, straightforward solution first
- This ensures you have at least a working solution, even if it’s not optimal
- Optimize the solution if time permits
4. Test Your Code
Before submitting:
- Test your code with the sample inputs provided
- Create additional test cases, including edge cases
- Use print statements or debugging tools to identify issues
5. Optimize and Refactor
If your initial solution passes all test cases:
- Look for ways to improve time or space complexity
- Refactor your code for better readability
- Remove any unnecessary variables or operations
6. Manage Your Time Wisely
Keep an eye on the clock:
- Allocate time for each problem based on its difficulty and point value
- If you’re stuck on a problem, move on and come back to it later
- Leave some time at the end for review and final submissions
7. Stay Calm and Focused
Maintain your composure:
- Take deep breaths if you feel stressed
- Remember that it’s normal to encounter difficult problems
- Focus on what you know and can do, rather than worrying about what you don’t know
Common Pitfalls to Avoid
Even experienced programmers can fall into traps during coding challenges. Here are some common pitfalls to be aware of:
1. Misreading the Problem
One of the most frequent mistakes is not fully understanding the problem requirements:
- Skimming the problem statement too quickly
- Overlooking important details or constraints
- Assuming the problem is similar to one you’ve seen before without verifying
Always take the time to read the problem carefully and make sure you understand all aspects before starting to code.
2. Overcomplicating the Solution
Sometimes, programmers try to implement an overly complex solution when a simpler one would suffice:
- Using advanced data structures or algorithms unnecessarily
- Implementing features that aren’t required by the problem
- Writing overly verbose code
Strive for simplicity and clarity in your solutions. Remember, the goal is to solve the problem efficiently, not to showcase every technique you know.
3. Neglecting Edge Cases
Failing to consider all possible inputs can lead to solutions that work for the sample cases but fail for edge cases:
- Not handling empty or null inputs
- Ignoring boundary conditions (e.g., minimum or maximum values)
- Failing to consider negative numbers or zero if not explicitly mentioned
Always think about potential edge cases and test your solution against them.
4. Inefficient Time Management
Poor time management can result in incomplete solutions:
- Spending too much time on a single problem
- Not allocating enough time for testing and debugging
- Rushing through easier problems without proper verification
Practice time management skills and learn to prioritize problems effectively.
5. Ignoring Time and Space Complexity
A solution that works but is inefficient may not be acceptable:
- Implementing solutions with poor time complexity (e.g., nested loops when unnecessary)
- Using excessive memory when a more space-efficient solution exists
- Not considering the impact of large input sizes on your algorithm’s performance
Always analyze and optimize the time and space complexity of your solutions.
6. Lack of Code Organization
Messy, disorganized code can lead to errors and make debugging difficult:
- Not using proper indentation or formatting
- Using unclear variable or function names
- Lack of comments for complex logic
Write clean, well-organized code even under time pressure. It will help you and potential reviewers understand your solution better.
7. Forgetting to Test
Submitting code without thorough testing is a recipe for failure:
- Not testing with the provided sample inputs
- Failing to create additional test cases
- Not verifying the output format matches the requirements
Always test your code with multiple inputs, including edge cases, before submitting.
Leveraging Tools and Resources
To excel in online coding challenges, it’s crucial to make use of available tools and resources. Here are some recommendations:
1. Integrated Development Environments (IDEs)
Most online coding platforms provide built-in IDEs, but familiarizing yourself with popular IDEs can be beneficial:
- Visual Studio Code: Lightweight and customizable
- PyCharm: Excellent for Python development
- IntelliJ IDEA: Great for Java and other JVM languages
- Sublime Text: Fast and minimalistic
Learn keyboard shortcuts and features of your preferred IDE to boost productivity during challenges.
2. Online Judges and Practice Platforms
These platforms offer a vast array of problems to practice:
- LeetCode: Extensive problem set with company-specific questions
- HackerRank: Offers both practice problems and competitions
- CodeSignal: Provides assessments and interview practice
- Codeforces: Hosts regular coding competitions
- AlgoCademy: Offers structured learning paths and AI-assisted problem-solving
3. Algorithm Visualizers
Visual tools can help you understand complex algorithms:
- VisuAlgo: Visualizes various data structures and algorithms
- Algorithm Visualizer: Interactive platform for algorithm visualization
- Sorting.at: Visualizes sorting algorithms
4. Documentation and Language References
Keep official documentation handy for quick reference:
- Python Documentation
- Java API Documentation
- JavaScript MDN Web Docs
- C++ Reference
5. Coding Interview Books
Some popular books that can supplement your preparation:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “Algorithm Design Manual” by Steven Skiena
6. Online Courses and Tutorials
Structured learning can help fill knowledge gaps:
- Coursera: Offers algorithm and data structure courses from top universities
- edX: Provides computer science courses, including those focused on coding interviews
- Udacity: Offers nanodegree programs in various programming disciplines
- AlgoCademy: Provides interactive coding tutorials and AI-assisted learning
7. Community Forums and Discussion Boards
Engage with fellow programmers to learn and share knowledge:
- Stack Overflow: For specific programming questions
- Reddit (r/cscareerquestions, r/learnprogramming): For career advice and learning resources
- LeetCode Discuss: Problem-specific discussions and solutions
Conclusion
Mastering online coding challenges is a journey that requires dedication, consistent practice, and a strategic approach. By understanding the nature of these challenges, honing your problem-solving skills, and leveraging the right tools and resources, you can significantly improve your performance and increase your chances of landing your dream tech job.
Remember that success in coding challenges is not just about getting the right answer, but also about demonstrating your thought process, coding style, and ability to work under pressure. As you prepare, focus on building a strong foundation in algorithms and data structures, practice regularly, and always seek to learn from each challenge you encounter.
Platforms like AlgoCademy can be invaluable in your preparation journey, offering structured learning paths, interactive tutorials, and AI-powered assistance to help you progress from beginner-level coding to mastering complex algorithms. Utilize these resources to complement your practice on coding challenge platforms and to gain a deeper understanding of the underlying concepts.
With persistence and the right approach, you can turn online coding challenges from daunting obstacles into exciting opportunities to showcase your skills and advance your tech career. Happy coding, and best of luck in your future challenges!