Mastering the Debugging Interview: Essential Skills for Software Engineers
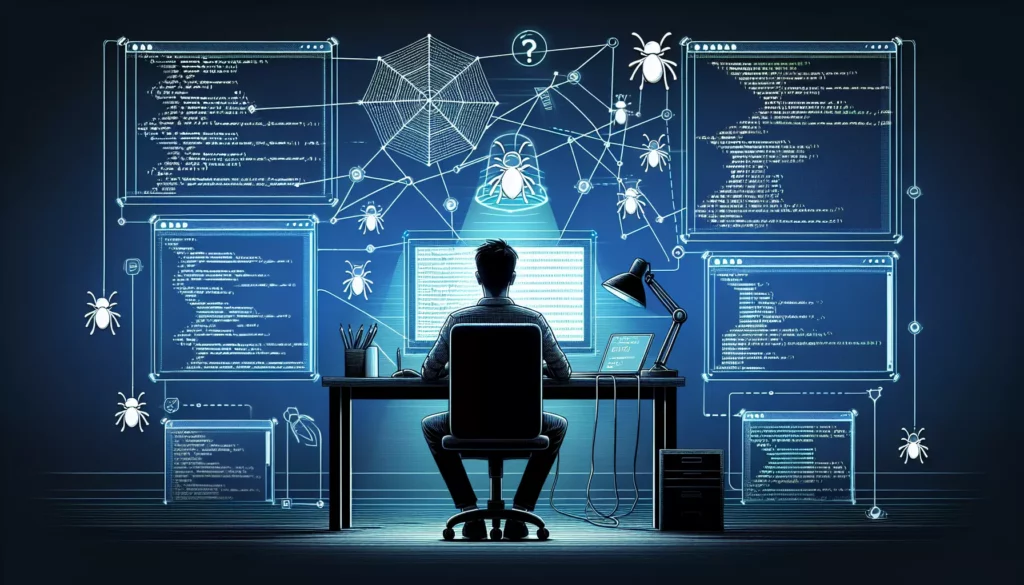
In the world of software engineering, debugging is an essential skill that separates great developers from good ones. As companies increasingly focus on hiring engineers who can efficiently diagnose and fix issues in complex codebases, the debugging interview has become a crucial part of the technical interview process. This article will dive deep into the art of debugging, exploring the skills tested, common scenarios, and strategies to excel in debugging interviews.
Understanding the Debugging Interview
A debugging interview is a specialized type of technical interview that focuses on a candidate’s ability to identify and resolve issues in existing code. Unlike coding interviews that test your ability to write algorithms from scratch, debugging interviews evaluate your problem-solving skills in the context of an already implemented (and potentially flawed) solution.
Key Focus Areas:
- Diagnosing issues in broken or inefficient codebases
- Fixing bugs and optimizing performance
- Reading and understanding code quickly
- Applying logical reasoning to identify root causes
- Communicating effectively about technical problems and solutions
Skills Tested in Debugging Interviews
Debugging interviews assess a wide range of skills that are crucial for day-to-day software development. Let’s explore these skills in detail:
1. Code Comprehension
One of the primary skills tested in a debugging interview is your ability to quickly read and understand unfamiliar code. This involves:
- Grasping the overall structure and flow of the program
- Identifying key components and their interactions
- Understanding the purpose of individual functions and classes
- Recognizing common design patterns and architectural styles
To improve your code comprehension skills:
- Practice reading open-source codebases
- Contribute to open-source projects
- Review code written by others regularly
- Familiarize yourself with various programming paradigms and design patterns
2. Analytical Thinking
Debugging requires strong analytical skills to break down complex problems into smaller, manageable parts. This involves:
- Identifying potential causes of bugs
- Formulating and testing hypotheses
- Eliminating unlikely scenarios
- Tracing program execution mentally
To enhance your analytical thinking:
- Practice solving logic puzzles and brain teasers
- Engage in root cause analysis exercises
- Learn and apply systematic debugging methodologies
- Develop a habit of questioning assumptions and validating information
3. Debugging Techniques
Familiarity with various debugging techniques and tools is crucial. Some common techniques include:
- Print debugging (adding print statements to trace execution)
- Using breakpoints and step-through debugging
- Analyzing stack traces and error messages
- Employing logging and monitoring tools
- Utilizing memory profilers and performance analyzers
To improve your debugging skills:
- Master your IDE’s debugging features
- Learn to use language-specific debugging tools (e.g., pdb for Python, gdb for C/C++)
- Practice debugging in different environments (local, remote, production)
- Familiarize yourself with common error patterns and their solutions
4. Problem-Solving
Debugging is essentially a specialized form of problem-solving. Key aspects include:
- Developing a systematic approach to isolate issues
- Thinking creatively to find alternative solutions
- Balancing quick fixes with long-term maintainability
- Prioritizing issues in complex systems
To enhance your problem-solving skills:
- Practice algorithmic problem-solving on platforms like LeetCode or HackerRank
- Participate in coding competitions and hackathons
- Work on personal projects that challenge you to solve real-world problems
- Study design patterns and software architecture principles
5. Communication
Effective communication is crucial in debugging interviews. You need to articulate your thought process, explain your reasoning, and discuss potential solutions clearly. This involves:
- Explaining technical concepts in simple terms
- Asking clarifying questions when needed
- Discussing trade-offs between different approaches
- Providing clear and concise explanations of your debugging process
To improve your communication skills:
- Practice explaining technical concepts to non-technical friends or family
- Participate in coding workshops or mentorship programs
- Write technical blog posts or contribute to documentation projects
- Record yourself explaining solutions and review for areas of improvement
Common Debugging Interview Scenarios
While debugging interviews can vary widely, there are some common scenarios you’re likely to encounter:
1. Fixing a Broken Function
You might be presented with a function that doesn’t produce the expected output. Your task would be to identify the issue and fix it.
Example:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# This function incorrectly returns 0 for fibonacci(0)
# Can you identify and fix the issue?
2. Optimizing Inefficient Code
You may be given a working but inefficient implementation and asked to optimize it for better performance.
Example:
def is_prime(n):
for i in range(2, n):
if n % i == 0:
return False
return True
# This function works but is inefficient for large numbers
# How would you optimize it?
3. Fixing a Memory Leak
In languages with manual memory management, you might be asked to identify and fix a memory leak in a given piece of code.
Example (C++):
class ResourceManager {
private:
int* data;
public:
ResourceManager() {
data = new int[1000];
}
~ResourceManager() {
// Destructor is empty
}
};
// This class has a memory leak. Can you identify and fix it?
4. Debugging Concurrency Issues
You might be presented with code that exhibits race conditions or deadlocks and asked to identify and resolve the issues.
Example (Java):
public class BankAccount {
private int balance = 0;
public void deposit(int amount) {
balance += amount;
}
public void withdraw(int amount) {
balance -= amount;
}
}
// This class is not thread-safe. How would you fix it?
5. Identifying and Fixing Security Vulnerabilities
You may be asked to review code for potential security issues and propose fixes.
Example (PHP):
$username = $_GET['username'];
$query = "SELECT * FROM users WHERE username = '$username'";
$result = mysqli_query($connection, $query);
// This code is vulnerable to SQL injection. How would you fix it?
Strategies for Excelling in Debugging Interviews
To perform well in debugging interviews, consider the following strategies:
1. Develop a Systematic Approach
Having a structured method for approaching debugging problems can help you stay organized and efficient. Consider following these steps:
- Reproduce the issue: Ensure you can consistently recreate the problem.
- Isolate the problem: Narrow down the scope of the issue to a specific component or function.
- Form a hypothesis: Based on the symptoms, guess what might be causing the problem.
- Test the hypothesis: Use debugging tools or add logging to confirm or refute your guess.
- Fix the issue: Once you’ve identified the root cause, implement a solution.
- Verify the fix: Ensure the problem is resolved and no new issues have been introduced.
- Reflect and learn: Consider what you’ve learned and how to prevent similar issues in the future.
2. Think Aloud
Verbalize your thought process as you work through the problem. This helps the interviewer understand your reasoning and problem-solving approach. It also allows them to provide hints or guidance if needed.
3. Ask Clarifying Questions
Don’t hesitate to ask for more information or clarification. This shows that you’re thorough and helps ensure you’re solving the right problem. Some useful questions might include:
- What is the expected behavior of this code?
- Are there any known constraints or assumptions?
- Can you provide more context about the environment where this code runs?
- Are there any specific performance requirements or limitations?
4. Use Debugging Tools Effectively
Demonstrate your proficiency with debugging tools. Even if you’re working in a simplified environment during the interview, explain which tools you would use in a real-world scenario and how you would apply them.
5. Consider Edge Cases
Show thoroughness by considering edge cases and boundary conditions. This might include:
- Empty inputs or null values
- Very large or very small inputs
- Negative numbers (if applicable)
- Concurrent access in multi-threaded scenarios
6. Optimize Incrementally
If asked to optimize code, start with a working solution and improve it step by step. Explain the trade-offs of each optimization and why you’re choosing a particular approach.
7. Consider Both Correctness and Efficiency
While fixing bugs, also think about the efficiency of the solution. Sometimes, a bug fix might solve the immediate problem but introduce performance issues. Be prepared to discuss the time and space complexity of your solutions.
8. Practice, Practice, Practice
The key to excelling in debugging interviews is consistent practice. Here are some ways to hone your skills:
- Contribute to open-source projects and help fix reported issues
- Participate in online coding challenges that focus on debugging
- Review and refactor your old code or projects
- Offer to debug friends’ or colleagues’ code
- Set up a study group to practice debugging scenarios together
Conclusion
Debugging interviews are a crucial part of the technical interview process, testing a wide range of skills that are essential for real-world software development. By focusing on improving your code comprehension, analytical thinking, problem-solving, and communication skills, you can significantly enhance your performance in these interviews.
Remember that debugging is as much an art as it is a science. While systematic approaches and technical knowledge are important, creativity, intuition, and experience also play significant roles. The more you practice debugging in various contexts, the more intuitive and efficient you’ll become at identifying and resolving issues.
As you prepare for debugging interviews, leverage resources like AlgoCademy, which offers interactive coding tutorials and tools to help you progress from beginner-level coding to mastering advanced concepts. With its focus on algorithmic thinking and problem-solving, platforms like AlgoCademy can be invaluable in developing the skills needed to excel in debugging interviews and beyond.
Ultimately, success in debugging interviews comes down to a combination of technical proficiency, logical reasoning, and effective communication. By honing these skills and approaching each debugging challenge with a systematic and thoughtful mindset, you’ll be well-prepared to tackle even the most complex debugging scenarios in your interviews and your future career as a software engineer.