The Anatomy of a Python Engineer Interview: Your Complete Guide
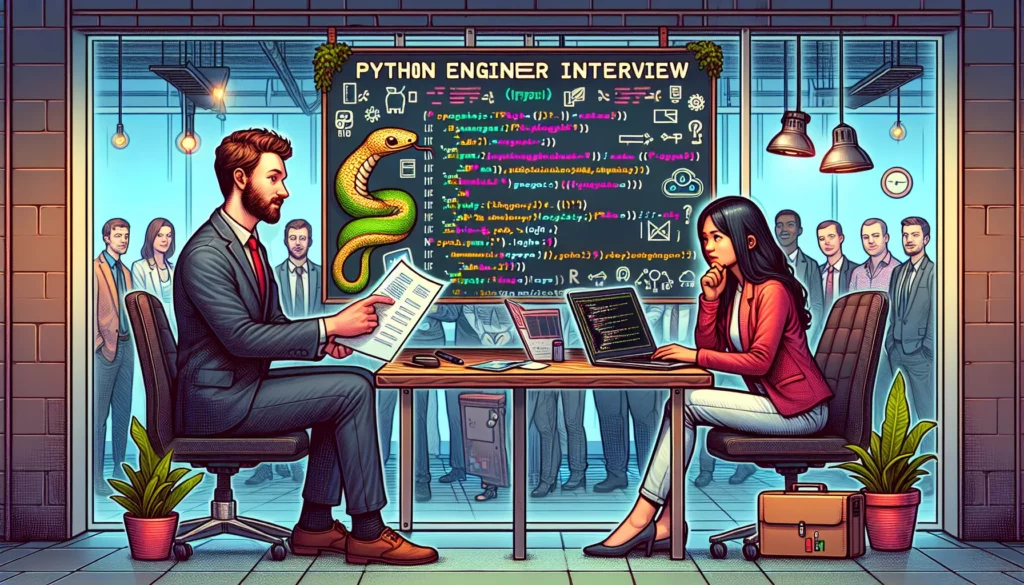
In today’s competitive tech landscape, Python has emerged as one of the most sought-after programming languages. As a result, Python engineer interviews have become increasingly common and challenging. Whether you’re a fresh graduate or an experienced developer looking to switch roles, understanding the anatomy of a Python engineer interview is crucial for success. In this comprehensive guide, we’ll dissect every aspect of the interview process, providing you with the knowledge and strategies needed to ace your next Python engineering interview.
Table of Contents
- Introduction to Python Engineer Interviews
- Preparing for the Interview
- Technical Skills Assessment
- Coding Challenges and Problem-Solving
- System Design and Architecture
- Behavioral and Cultural Fit Questions
- Python Frameworks and Libraries
- Python Best Practices and Code Quality
- Data Structures and Algorithms
- Database Knowledge and ORM
- Testing and Debugging
- Version Control and Collaboration
- Soft Skills and Communication
- Questions to Ask the Interviewer
- Common Mistakes to Avoid
- Post-Interview Follow-up
- Conclusion
1. Introduction to Python Engineer Interviews
Python engineer interviews are designed to assess your proficiency in Python programming, problem-solving skills, and your ability to apply these skills in real-world scenarios. These interviews typically consist of multiple rounds, each focusing on different aspects of your expertise.
The interview process may include:
- Phone or video screening
- Technical assessments
- Coding challenges
- System design discussions
- Behavioral interviews
Understanding the structure and expectations of each round will help you prepare effectively and increase your chances of success.
2. Preparing for the Interview
Thorough preparation is key to performing well in a Python engineer interview. Here are some essential steps to get ready:
- Review Python fundamentals: Ensure you have a solid grasp of Python syntax, data types, control structures, and object-oriented programming concepts.
- Practice coding: Solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal to sharpen your skills.
- Study common algorithms and data structures: Familiarize yourself with sorting algorithms, searching techniques, and data structures like arrays, linked lists, trees, and graphs.
- Brush up on Python-specific features: Understand generators, decorators, context managers, and other Python-specific concepts.
- Review your projects: Be prepared to discuss your past projects, challenges faced, and solutions implemented.
- Research the company: Understand the company’s products, culture, and recent news to demonstrate your interest and enthusiasm.
3. Technical Skills Assessment
The technical skills assessment is a crucial part of the Python engineer interview. Interviewers will evaluate your knowledge of Python and related technologies. Be prepared to demonstrate your expertise in:
- Python syntax and language features
- Object-oriented programming principles
- Functional programming concepts
- Python standard library
- Common Python frameworks and libraries (e.g., Django, Flask, NumPy, Pandas)
- API development and RESTful services
- Asynchronous programming (e.g., asyncio)
- Performance optimization techniques
Example technical question:
Interviewer: "Can you explain the difference between a list and a tuple in Python, and provide an example of when you would use each?"
Your response: "Certainly! Lists and tuples are both sequence data types in Python, but they have some key differences:
1. Mutability: Lists are mutable, meaning you can modify their contents after creation. Tuples are immutable, so once created, their contents cannot be changed.
2. Syntax: Lists are defined using square brackets [], while tuples use parentheses ().
3. Performance: Tuples are generally more memory-efficient and faster than lists for small sequences.
Here's an example of when to use each:
# Using a list when you need to modify the sequence
shopping_list = ['apples', 'bananas', 'oranges']
shopping_list.append('grapes') # Modifying the list
# Using a tuple for fixed data that shouldn't change
coordinates = (34.0522, -118.2437) # Latitude and longitude
Lists are ideal for collections that may change, like a shopping list or a dynamic set of database results. Tuples are better for representing fixed collections, like coordinates or configuration settings, where immutability is desired for data integrity."
4. Coding Challenges and Problem-Solving
Coding challenges are a staple of Python engineer interviews. These challenges assess your ability to translate problem statements into working code. Here are some tips for tackling coding challenges:
- Read the problem statement carefully and ask clarifying questions if needed.
- Think through your approach before starting to code.
- Start with a simple solution and optimize later if time allows.
- Write clean, readable code with proper naming conventions.
- Test your code with various inputs, including edge cases.
- Explain your thought process as you code.
Example coding challenge:
Interviewer: "Write a Python function that takes a string as input and returns the longest palindromic substring within it."
Your solution:
def longest_palindromic_substring(s):
if not s:
return ""
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return s[left + 1:right]
longest = ""
for i in range(len(s)):
# Check for odd-length palindromes
palindrome1 = expand_around_center(i, i)
if len(palindrome1) > len(longest):
longest = palindrome1
# Check for even-length palindromes
palindrome2 = expand_around_center(i, i + 1)
if len(palindrome2) > len(longest):
longest = palindrome2
return longest
# Test the function
print(longest_palindromic_substring("babad")) # Output: "bab" or "aba"
print(longest_palindromic_substring("cbbd")) # Output: "bb"
"This solution uses the 'expand around center' approach. It iterates through each character in the string, considering it as a potential center of a palindrome. For each center, it expands outwards, checking for both odd and even-length palindromes. The time complexity is O(n^2), and the space complexity is O(1), where n is the length of the input string."
5. System Design and Architecture
For more senior Python engineering roles, you may encounter system design questions. These assess your ability to design scalable, efficient, and maintainable systems. Key areas to focus on include:
- Scalability and performance considerations
- Database design and optimization
- Caching strategies
- Microservices architecture
- Load balancing and distributed systems
- API design principles
- Security considerations
Example system design question:
Interviewer: "Design a URL shortening service like bit.ly using Python."
Your response: "Certainly! Here's a high-level design for a URL shortening service:
1. Components:
- Web server (e.g., Flask or Django)
- Database (e.g., PostgreSQL)
- Caching layer (e.g., Redis)
- Load balancer for scalability
2. API Endpoints:
- POST /shorten: Create a short URL
- GET /{short_code}: Redirect to the original URL
3. Database Schema:
- Table: urls
- id: bigint (primary key)
- original_url: text
- short_code: varchar(8)
- created_at: timestamp
- expiration_date: timestamp (optional)
4. URL Shortening Algorithm:
- Generate a unique short code (e.g., base62 encoding of an auto-incrementing ID)
- Ensure uniqueness by checking against existing codes in the database
5. Redirection Mechanism:
- Look up the short code in the cache
- If not found, query the database
- If found, increment a counter (for analytics) and redirect to the original URL
- If not found, return a 404 error
6. Scaling Considerations:
- Use a distributed cache (e.g., Redis cluster) for faster lookups
- Implement database sharding for horizontal scaling
- Use a CDN for serving static content and reducing latency
7. Analytics:
- Implement a separate service for tracking clicks and gathering analytics
Here's a basic implementation of the core functionality:"
import string
import random
from flask import Flask, request, redirect, jsonify
app = Flask(__name__)
# In-memory storage for demonstration (replace with database in production)
url_mapping = {}
def generate_short_code():
characters = string.ascii_letters + string.digits
return ''.join(random.choice(characters) for _ in range(6))
@app.route('/shorten', methods=['POST'])
def shorten_url():
original_url = request.json.get('url')
if not original_url:
return jsonify({'error': 'No URL provided'}), 400
short_code = generate_short_code()
while short_code in url_mapping:
short_code = generate_short_code()
url_mapping[short_code] = original_url
short_url = f"http://short.url/{short_code}"
return jsonify({'short_url': short_url}), 201
@app.route('/')
def redirect_to_original(short_code):
original_url = url_mapping.get(short_code)
if original_url:
return redirect(original_url)
else:
return "URL not found", 404
if __name__ == '__main__':
app.run(debug=True)
"This basic implementation demonstrates the core functionality. In a production environment, you'd replace the in-memory storage with a database, implement proper error handling, add authentication and rate limiting, and consider the scaling aspects mentioned earlier."
6. Behavioral and Cultural Fit Questions
Behavioral questions assess your soft skills, problem-solving approach, and cultural fit within the organization. Common themes include:
- Teamwork and collaboration
- Conflict resolution
- Adaptability and learning
- Leadership and initiative
- Time management and prioritization
Use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
Example behavioral question:
Interviewer: "Can you describe a time when you had to work on a challenging project with a tight deadline? How did you handle it?"
Your response: "Certainly. In my previous role, we were tasked with developing a new feature for our e-commerce platform that would recommend products based on user browsing history. The project was critical for the upcoming holiday season, and we had only four weeks to complete it.
Situation: The project was complex, requiring integration with our existing recommendation engine and the development of a new machine learning model.
Task: As the lead Python developer, I was responsible for coordinating the team's efforts, developing the core algorithm, and ensuring timely delivery.
Action: I took the following steps:
1. Broke down the project into smaller, manageable tasks and created a detailed timeline.
2. Held daily stand-up meetings to track progress and address any blockers quickly.
3. Implemented pair programming for complex parts of the code to reduce errors and share knowledge.
4. Utilized Python's multiprocessing library to optimize the recommendation algorithm's performance.
5. Worked closely with the data science team to fine-tune the machine learning model.
6. Set up automated tests to ensure code quality and catch regressions early.
Result: We successfully launched the feature two days before the deadline. The new recommendation system increased average order value by 15% during the holiday season. The project's success was attributed to effective team collaboration, strategic use of Python's capabilities, and rigorous testing.
This experience taught me the importance of clear communication, efficient task management, and leveraging Python's strengths in high-pressure situations."
7. Python Frameworks and Libraries
Familiarity with popular Python frameworks and libraries is often expected in Python engineer interviews. Be prepared to discuss:
- Web frameworks: Django, Flask, FastAPI
- Data science libraries: NumPy, Pandas, SciPy
- Machine learning frameworks: TensorFlow, PyTorch, scikit-learn
- Testing frameworks: pytest, unittest
- Asynchronous programming: asyncio, aiohttp
- ORM libraries: SQLAlchemy, Django ORM
Example framework-related question:
Interviewer: "Compare and contrast Django and Flask. When would you choose one over the other for a project?"
Your response: "Django and Flask are both popular Python web frameworks, but they have different philosophies and use cases:
Django:
1. Full-featured framework with built-in ORM, admin interface, and authentication system.
2. Follows the 'batteries included' approach, providing many out-of-the-box features.
3. Enforces a specific project structure and follows the MTV (Model-Template-View) pattern.
4. Great for large, complex applications that require a lot of built-in functionality.
5. Has a steeper learning curve but can speed up development for feature-rich applications.
Flask:
1. Lightweight and flexible micro-framework.
2. Minimalist core with the ability to add extensions as needed.
3. Provides more freedom in terms of project structure and design choices.
4. Excellent for small to medium-sized applications or microservices.
5. Easier to learn and get started with, but may require more setup for complex features.
Choosing between Django and Flask depends on the project requirements:
Choose Django when:
- Building a large, complex application with many features
- Need for built-in admin interface and authentication
- Working on a project that benefits from Django's opinionated structure
- Developing applications that require robust ORM capabilities
Choose Flask when:
- Building small to medium-sized applications or microservices
- Need for more flexibility in architectural decisions
- Working on projects that require custom implementations of features
- Developing APIs or single-page applications
For example, if I were building a content management system with user authentication, an admin panel, and complex database relationships, I'd lean towards Django. On the other hand, if I were creating a simple RESTful API or a lightweight web service, Flask would be my go-to choice.
Ultimately, both frameworks are powerful and can be used for various projects. The decision often comes down to personal preference, team expertise, and specific project requirements."
8. Python Best Practices and Code Quality
Demonstrating knowledge of Python best practices and writing high-quality code is crucial in a Python engineer interview. Key areas to focus on include:
- PEP 8 style guide adherence
- Writing clean, readable, and maintainable code
- Proper error handling and exception management
- Effective use of comments and docstrings
- Code organization and modularization
- Performance optimization techniques
Example question on best practices:
Interviewer: "How would you refactor this code to improve its quality and adhere to Python best practices?"
Original code:
def process_data(d):
res = []
for k,v in d.items():
if v > 10:
res.append(k.upper())
else:
res.append(k.lower())
return res
Your refactored version:
def process_data(data):
"""
Process a dictionary and return a list of modified keys based on their values.
Args:
data (dict): A dictionary with string keys and numeric values.
Returns:
list: A list of modified keys. Keys are uppercased if their value is greater than 10,
otherwise they are lowercased.
Example:
>>> process_data({'a': 15, 'b': 5, 'c': 20})
['A', 'b', 'C']
"""
try:
return [
key.upper() if value > 10 else key.lower()
for key, value in data.items()
]
except AttributeError:
raise ValueError("Input must be a dictionary with string keys and numeric values.")
# Example usage
sample_data = {'apple': 12, 'banana': 5, 'cherry': 18}
result = process_data(sample_data)
print(result)
"This refactored version improves the original code in several ways:
1. Descriptive function and variable names: 'process_data' and 'data' are more informative than 'd'.
2. Added a comprehensive docstring explaining the function's purpose, parameters, return value, and an example.
3. Used a list comprehension for conciseness and readability.
4. Implemented error handling to catch potential AttributeError and provide a meaningful error message.
5. Followed PEP 8 guidelines for naming conventions and spacing.
6. Removed the temporary 'res' variable, making the function more streamlined.
7. Added an example usage at the end for clarity.
These changes make the code more readable, maintainable, and robust while adhering to Python best practices."
9. Data Structures and Algorithms
A solid understanding of data structures and algorithms is essential for any Python engineer. Be prepared to discuss and implement:
- Basic data structures: lists, dictionaries, sets, tuples
- Advanced data structures: trees, graphs, heaps
- Sorting algorithms: quicksort, mergesort, heapsort
- Searching algorithms: binary search, depth-first search, breadth-first search
- Dynamic programming
- Time and space complexity analysis
Example algorithm question:
Interviewer: "Implement a function to find the kth largest element in an unsorted array using Python."
Your solution:
import heapq
def find_kth_largest(nums, k):
"""
Find the kth largest element in an unsorted array.
Args:
nums (List[int]): An unsorted list of integers.
k (int): The kth largest element to find.
Returns:
int: The kth largest element in the array.
Raises:
ValueError: If k is out of range or the input list is empty.
Time Complexity: O(n log k), where n is the length of nums.
Space Complexity: O(k) for the heap.
"""
if not nums or k < 1 or k > len(nums):
raise ValueError("Invalid input: k is out of range or the list is empty.")
# Create a min-heap with the first k elements
min_heap = nums[:k]
heapq.heapify(min_heap)
# Process the remaining elements
for num in nums[k:]:
if num > min_heap[0]:
heapq.heapreplace(min_heap, num)
# The root of the min-heap is the kth largest element
return min_heap[0]
# Test the function
nums = [3, 2, 1, 5, 6, 4]
k = 2
result = find_kth_largest(nums, k)
print(f"The {k}th largest element is: {result}") # Output: The 2th largest element is: 5
"This solution uses a min-heap to efficiently find the kth largest element:
1. We create a min-heap with the first k elements of the array.
2. For each remaining element, if it's larger than the smallest element in the heap (the root), we replace the root with this new element.
3. After processing all elements, the root of the heap will be the kth largest element.
This approach is efficient for large datasets and has a time complexity of O(n log k), where n is the length of the input array. The space complexity is O(k) for storing the heap.
An alternative approach could be to use QuickSelect algorithm, which has an average time complexity of O(n) but a worst-case complexity of O(n^2). The heap-based solution provides a good balance of efficiency and consistent performance across different input distributions."
10. Database Knowledge and ORM
Python engineers often work with databases, so understanding database concepts and ORM (Object-Relational Mapping) is important. Key areas to focus on include:
- SQL fundamentals
- Database design principles
- ORM concepts and popular Python ORMs (e.g., SQLAlchemy, Django ORM)
- Query optimization
- Indexing and performance tuning
- NoSQL databases (e.g., MongoDB, Redis)
Example database-related question:
Interviewer: "Explain how you would use SQLAlchemy to define a many-to-many relationship between two entities, 'Student' and 'Course', and write a query to fetch all courses for a given student."
Your response: "Certainly! Here's how we can define a many-to-many relationship between 'Student' and 'Course' using SQLAlchemy, and then query for a student's courses:
First, let's define the models:"
from sqlalchemy import create_engine, Column, Integer, String, Table, ForeignKey
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import relationship, sessionmaker
Base = declarative_base()
# Association table for the many-to-many relationship
student_course = Table('student_course', Base.metadata,
Column('student_id', Integer, ForeignKey('students.id')),
Column('course_id', Integer, ForeignKey('courses.id'))
)
class Student(Base):
__tablename__ = 'students'
id = Column(Integer, primary_key=True)
name = Column(String)
courses = relationship('Course', secondary=student_course, back_populates='students')
class Course(Base):
__tablename__ = 'courses'
id = Column(Integer, primary_key=True)
name = Column(String)
students = relationship('Student', secondary=student_course, back_populates='courses')
"Now, let's set up the database and create a session:"
engine = create_engine('sqlite:///school.db')
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
session = Session()
"To query all courses for a given student, we can do the following:"
def get_courses_for_student(student_id):
student = session.query(Student).filter_by(id=student_id).first()
if student:
return student.courses
return []
# Example usage
student_id = 1
courses = get_courses_for_student(student_id)
for course in courses:
print(f"Course: {course.name}")
"This setup demonstrates several key concepts:
1. We use an association table 'student_course' to represent the many-to-many relationship.
2. The 'relationship' function in both Student and Course models establishes the bidirectional relationship.
3. The 'secondary' parameter in the relationship function refers to the association table.
4. The 'back_populates' parameter ensures that the relationship is synchronized in both directions.
The query to fetch all courses for a student is straightforward thanks to the ORM. We simply access the 'courses' attribute of the Student object, and SQLAlchemy generates the appropriate JOIN queries behind the scenes.
This approach offers several benefits:
- It abstracts away the complexity of SQL joins.
- It provides an intuitive, Pythonic way to work with relational data.
- It allows for easy querying and manipulation of related objects.
In a production environment, you'd want to add error handling, connection pooling, and possibly use async SQLAlchemy for better performance in web applications."
11. Testing and Debugging
Proficiency in testing and debugging is crucial for ensuring code quality and reliability. Be prepared to discuss:
- Unit testing with pytest or unittest
- Integration testing
- Test-driven development (TDD)
- Mocking and patching
- Debugging techniques and tools
- Code coverage and continuous integration
Example testing question:
Interviewer: "Write a Python function to calculate the factorial of a number, and then write unit tests for this function using pytest."
Your response: "Certainly! Let's start with the factorial function and then write tests for it using pytest."
# File: factorial.py
def factorial(n):
"""
Calculate the factorial of a non-negative integer.
Args:
n (int): A non-negative integer.
Returns:
int: The factorial of n.
Raises:
ValueError: If n is negative.
TypeError: If n is not an integer.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
if n == 0 or n == 1:
return 1
return n * factorial(n - 1)
"Now, let's write unit tests for this function using pytest:"
# File: test_factorial.py
import pytest
from factorial import factorial
def test_factorial_zero():
assert factorial(0) == 1
def test_factorial_one():
assert factorial(1) == 1
def test_factorial_positive():
assert factorial(5) == 120
assert factorial(10) == 3628800
def test_factorial_negative():
with pytest.raises(ValueError):
factorial(-1)
def test_factorial_non_integer():
with pytest.raises(TypeError):
factorial(3.14)
def test_factorial_large_number():
assert factorial(20) == 2432902008176640000
"These tests cover various scenarios:
1. Factorial of 0 and 1 (edge cases)
2. Factorial of positive integers
3. Handling of negative inputs
4. Handling of non-integer inputs
5. Factorial of a large number to check for overflow issues
To run these tests, you would use the pytest command in the terminal:
pytest test_factorial.py
This approach to testing demonstrates several best practices:
1. Testing both normal cases and edge cases
2. Testing for expected exceptions
3. Using descriptive test names
4. Separating test code from production code
In a real-world scenario, you might also want to consider:
- Parameterized tests for testing multiple inputs
- Fixtures for setup and teardown
- Mocking for testing functions with dependencies
- Integration tests if this function is part of a larger system
Testing is crucial for maintaining code quality, catching regressions, and facilitating refactoring. It's an essential skill for any Python engineer."
12. Version Control and Collaboration
Proficiency with version control systems, particularly Git, is essential for collaborative software development. Be prepared to discuss:
- Basic Git commands and workflows
- Branching strategies (e.g., Git Flow, GitHub Flow)
- Pull requests and code review processes
- Merge conflict resolution
- Collaborative features of platforms like GitHub or GitLab
Example version control question:
Interviewer: "Describe your typical Git workflow when working on a new feature in a team environment."
Your response: "Certainly! Here's a typical Git workflow I follow when working on a new feature in a team environment:
1. Update the main branch:
- git checkout main
- git pull origin main
2. Create a new feature branch:
- git checkout -b feature/new-feature-name
3. Develop the feature:
- Make small, focused commits as I work
- git add .
- git commit -m "Descriptive commit message"
4. Regularly sync with the main branch:
- git checkout main
- git pull origin main
- git checkout feature/new-feature-name
- git merge main
- Resolve any merge conflicts if they occur
5. Push the feature branch to the remote repository:
- git push origin feature/new-feature-name
6. Create a pull request:
- Use the GitHub/GitLab interface to create a pull request
- Write a detailed description of the changes and their purpose
- Request reviews from team members
7. Address review comments:
- Make necessary changes based on feedback
- Push additional commits to the feature branch
8. Once approved, merge the pull request:
- Usually done through the GitHub/GitLab interface
- Choose squash and merge if we prefer a cleaner commit history
9. Delete the feature branch:
- git branch -d feature/new-feature-name
- git push origin --delete feature/new-feature-name
10. Start the next feature by repeating from step 1
This workflow has several advantages:
- It keeps the main branch stable and always deployable
- It facilitates code review and collaboration
- It minimizes merge conflicts by regularly syncing with the main branch
- It maintains a clear history of feature development
Additionally, I follow these best practices:
- Write clear, concise commit messages
- Keep commits small and focused on a single