The Anatomy of a Kotlin Engineer Interview: A Comprehensive Guide
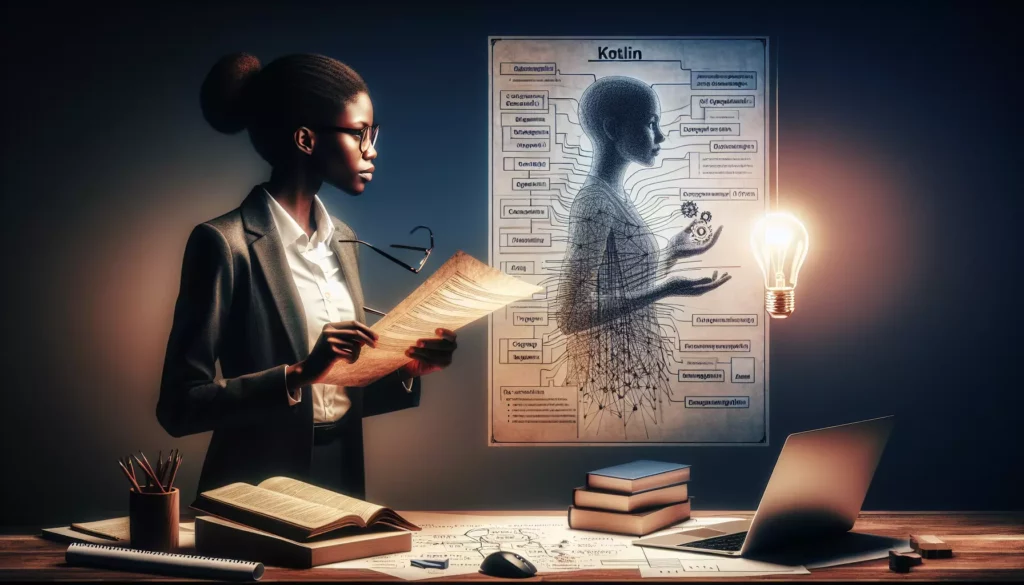
As the demand for Kotlin developers continues to rise, particularly in the Android development sphere, mastering the art of the Kotlin engineer interview has become increasingly crucial. Whether you’re a seasoned developer looking to switch to Kotlin or a newcomer to the programming world, understanding the intricacies of a Kotlin interview can be the key to landing your dream job. In this comprehensive guide, we’ll dissect the anatomy of a Kotlin engineer interview, providing you with insights, tips, and strategies to help you excel.
1. Understanding the Kotlin Landscape
Before diving into the specifics of the interview process, it’s essential to grasp the current state of Kotlin in the software development industry.
1.1 The Rise of Kotlin
Kotlin has experienced a meteoric rise since its introduction by JetBrains in 2011. Its adoption rate skyrocketed when Google announced first-class support for Kotlin in Android development in 2017. Today, Kotlin is not just an alternative to Java for Android development; it’s the preferred language for many developers and companies.
1.2 Why Companies Are Choosing Kotlin
Understanding why companies are gravitating towards Kotlin can give you an edge in interviews. Some key reasons include:
- Conciseness and expressiveness
- Null safety features
- Interoperability with Java
- Support for functional programming
- Coroutines for asynchronous programming
2. Preparing for the Technical Interview
The technical interview is often the most daunting part of the hiring process. Here’s how you can prepare effectively:
2.1 Mastering Kotlin Fundamentals
Ensure you have a solid grasp of Kotlin basics. This includes:
- Variables and data types
- Control flow (if/else, when, loops)
- Functions and lambdas
- Object-oriented programming concepts
- Null safety and smart casts
2.2 Advanced Kotlin Concepts
Be prepared to discuss and demonstrate knowledge of more advanced Kotlin features:
- Extension functions
- Higher-order functions
- Coroutines and asynchronous programming
- Kotlin-specific collections operations
- Sealed classes and data classes
2.3 Coding Challenges and Problem-Solving
Practice solving coding problems in Kotlin. Platforms like LeetCode, HackerRank, and CodeSignal offer Kotlin-specific challenges. Focus on:
- Algorithm implementation
- Data structure manipulation
- Time and space complexity analysis
2.4 Sample Coding Question
Here’s an example of a coding question you might encounter:
Question: Implement a function that finds the longest palindromic substring in a given string.
Here’s a Kotlin implementation:
fun longestPalindrome(s: String): String {
if (s.length < 2) return s
var start = 0
var maxLength = 1
fun expandAroundCenter(left: Int, right: Int) {
var l = left
var r = right
while (l >= 0 && r < s.length && s[l] == s[r]) {
if (r - l + 1 > maxLength) {
start = l
maxLength = r - l + 1
}
l--
r++
}
}
for (i in s.indices) {
expandAroundCenter(i, i)
expandAroundCenter(i, i + 1)
}
return s.substring(start, start + maxLength)
}
This solution uses the expand around center technique to find the longest palindrome. It’s efficient and demonstrates several Kotlin features like local functions and string manipulation.
3. System Design and Architecture
For more senior positions, you may be asked about system design and architecture, particularly in the context of Android development.
3.1 Android Architecture Components
Be prepared to discuss:
- ViewModel and LiveData
- Room for local data storage
- Navigation component
- WorkManager for background tasks
3.2 Design Patterns in Kotlin
Familiarize yourself with common design patterns and their implementation in Kotlin:
- Singleton (object declaration in Kotlin)
- Factory
- Observer (using Kotlin’s property delegates)
- Builder (using Kotlin’s named and default arguments)
3.3 Kotlin-specific Architectural Approaches
Discuss Kotlin-specific approaches to architecture:
- Multiplatform projects
- Coroutines for async operations
- Flow for reactive programming
4. Behavioral Questions and Soft Skills
Technical skills are crucial, but soft skills and cultural fit are equally important in many organizations.
4.1 Common Behavioral Questions
Prepare for questions like:
- “Describe a challenging project you worked on using Kotlin.”
- “How do you stay updated with the latest Kotlin features and best practices?”
- “Tell me about a time when you had to refactor a large codebase. How did you approach it?”
4.2 Demonstrating Passion for Kotlin
Show your enthusiasm for Kotlin by:
- Discussing open-source contributions
- Mentioning Kotlin conferences or meetups you’ve attended
- Sharing personal projects or experiments with Kotlin
5. Practical Tips for Interview Success
5.1 Before the Interview
- Review the company’s products or services, especially if they’re Kotlin-based
- Prepare questions about their development process and use of Kotlin
- Set up a Kotlin development environment for potential coding exercises
5.2 During the Interview
- Think out loud when solving problems
- Don’t hesitate to ask for clarification
- Showcase your knowledge of Kotlin idioms and best practices
5.3 After the Interview
- Send a thank-you note, possibly mentioning a specific topic from the interview
- Reflect on areas where you could improve for future interviews
6. Common Pitfalls to Avoid
Be aware of these common mistakes in Kotlin interviews:
- Overusing nullable types when not necessary
- Neglecting to leverage Kotlin’s concise syntax
- Misunderstanding the scope of ‘this’ in lambdas
- Incorrectly using coroutines or not understanding their lifecycle
7. Mock Interview Exercise
To help you prepare, here’s a mock interview scenario:
Interviewer: “Can you explain the difference between ‘val’ and ‘var’ in Kotlin, and when you would use each?”
Ideal Answer: “In Kotlin, ‘val’ and ‘var’ are used to declare variables, but they have different characteristics:
‘val’ is used to declare read-only variables. Once a value is assigned to a ‘val’, it cannot be reassigned. It’s similar to ‘final’ in Java. For example:
val pi = 3.14
// pi = 3.14159 // This would result in a compilation error
‘var’, on the other hand, is used to declare mutable variables. The value of a ‘var’ can be changed after its initial assignment:
var count = 0
count = 1 // This is valid
I would use ‘val’ when I want to ensure that a variable’s value remains constant throughout its lifetime, which helps in writing more predictable and safer code. This is particularly useful for variables that represent constants, configuration values, or values that shouldn’t change after initialization.
I would use ‘var’ when I need a variable whose value can change over time, such as counters, accumulators, or variables that need to be updated based on some logic or user input.
It’s generally a good practice to use ‘val’ by default and only use ‘var’ when you explicitly need mutability. This aligns with the principle of immutability, which can lead to more robust and easier-to-reason-about code.”
Interviewer: “Great explanation. Now, can you provide an example of how you might use both ‘val’ and ‘var’ in a real-world scenario?”
Ideal Answer: “Certainly! Let’s consider a simple banking application where we’re managing a user’s account balance. Here’s an example that demonstrates the use of both ‘val’ and ‘var’:”
class BankAccount(val accountNumber: String, initialBalance: Double) {
var balance: Double = initialBalance
private set
val transactionHistory: MutableList<String> = mutableListOf()
fun deposit(amount: Double) {
if (amount > 0) {
balance += amount
transactionHistory.add("Deposit: $$amount")
}
}
fun withdraw(amount: Double) {
if (amount > 0 && balance >= amount) {
balance -= amount
transactionHistory.add("Withdrawal: $$amount")
}
}
}
fun main() {
val account = BankAccount("1234567890", 1000.0)
println("Initial balance: $${account.balance}")
account.deposit(500.0)
account.withdraw(200.0)
println("Final balance: $${account.balance}")
println("Transaction history: ${account.transactionHistory}")
}
In this example:
- We use ‘val’ for ‘accountNumber’ because an account number typically doesn’t change once it’s assigned.
- We use ‘var’ for ‘balance’ because it needs to be mutable – it changes with deposits and withdrawals. However, we make the setter private to ensure that the balance can only be modified through the class’s methods.
- We use ‘val’ for ‘transactionHistory’, but it’s a mutable list. This means the reference to the list doesn’t change, but we can add or remove items from the list.
- In the ‘main’ function, we use ‘val’ for the ‘account’ variable because we don’t need to reassign it to a different BankAccount instance.
This example demonstrates how ‘val’ and ‘var’ can be used together to create a more robust and intention-revealing API. By using ‘val’ where possible, we’re making our code more predictable and easier to reason about, while still allowing for necessary mutability with ‘var’ where it’s needed.”
8. Conclusion
Mastering the Kotlin engineer interview is a journey that requires dedication, practice, and a deep understanding of both the language and its ecosystem. By focusing on core Kotlin concepts, problem-solving skills, system design, and soft skills, you’ll be well-prepared to tackle any Kotlin interview.
Remember, the key to success lies not just in knowing Kotlin, but in demonstrating how you can use it to solve real-world problems efficiently and elegantly. As you prepare, keep coding, stay curious, and don’t hesitate to dive deep into Kotlin’s more advanced features.
With the right preparation and mindset, you’ll be well-equipped to showcase your Kotlin expertise and land that dream job. Good luck with your Kotlin engineer interview!