The Anatomy of a Java Engineer Interview: What to Expect and How to Prepare
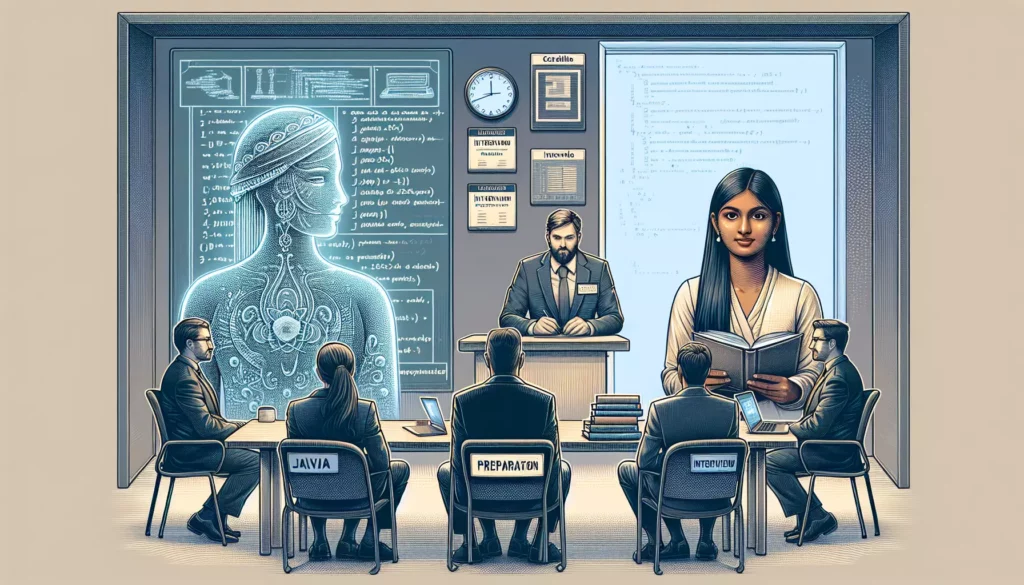
As the tech industry continues to grow and evolve, Java remains one of the most popular programming languages in the world. With its versatility and widespread use in enterprise applications, web development, and Android app creation, Java engineers are in high demand. If you’re aspiring to become a Java engineer or looking to advance your career in this field, understanding the interview process is crucial. In this comprehensive guide, we’ll dissect the anatomy of a Java engineer interview, providing you with valuable insights on what to expect and how to prepare effectively.
1. Understanding the Java Engineer Interview Process
Before diving into the specifics, it’s important to understand that the interview process for Java engineers can vary depending on the company and position. However, most interviews typically follow a similar structure:
- Initial phone or video screening
- Technical phone interview
- On-site interviews (or multiple rounds of virtual interviews for remote positions)
- Coding challenges or take-home assignments
- System design discussions
- Behavioral interviews
Each stage of the process is designed to evaluate different aspects of your skills, knowledge, and cultural fit within the organization. Let’s break down each component and explore what you can expect.
2. Initial Screening: Making a Strong First Impression
The initial screening is often conducted by a recruiter or HR representative. This stage is primarily used to:
- Verify your basic qualifications
- Assess your communication skills
- Determine your interest in the position
- Discuss salary expectations and availability
To prepare for this stage:
- Review the job description thoroughly
- Prepare a brief introduction about yourself and your experience with Java
- Research the company and be ready to explain why you’re interested in the role
- Have a clear understanding of your salary expectations and availability
Remember, while this stage may seem less technical, it’s crucial for moving forward in the process. A strong first impression can set a positive tone for the rest of your interviews.
3. Technical Phone Interview: Demonstrating Your Java Expertise
The technical phone interview is typically conducted by a senior Java engineer or a technical hiring manager. This stage aims to assess your technical knowledge and problem-solving skills. You can expect questions on:
- Core Java concepts
- Object-oriented programming principles
- Data structures and algorithms
- Java frameworks and libraries
- Basic system design concepts
To prepare for the technical phone interview:
- Review core Java concepts, including OOP principles, collections, multithreading, and exception handling
- Practice explaining complex concepts in simple terms
- Be prepared to write code or pseudo-code over the phone or in a shared online editor
- Familiarize yourself with common Java frameworks like Spring, Hibernate, and JUnit
- Brush up on basic data structures and algorithms
Here’s an example of a question you might encounter during a technical phone interview:
Interviewer: Can you explain the difference between an abstract class and an interface in Java?
Your response: Certainly! Both abstract classes and interfaces are used to achieve abstraction in Java, but they have some key differences:
1. Methods:
- Abstract class: Can have both abstract and non-abstract (concrete) methods.
- Interface: Prior to Java 8, could only have abstract methods. Since Java 8, can have default and static methods.
2. Variables:
- Abstract class: Can have non-final variables.
- Interface: Variables are implicitly public, static, and final.
3. Inheritance:
- Abstract class: A class can extend only one abstract class.
- Interface: A class can implement multiple interfaces.
4. Constructor:
- Abstract class: Can have a constructor.
- Interface: Cannot have a constructor.
5. Access modifiers:
- Abstract class: Can have any access modifier for members.
- Interface: Members are implicitly public.
Here's a quick example to illustrate:
Abstract class:
public abstract class Animal {
protected String name;
public abstract void makeSound();
public void eat() {
System.out.println(name + " is eating.");
}
}
Interface:
public interface Flyable {
void fly();
default void glide() {
System.out.println("Gliding...");
}
}
In general, use an abstract class when you want to provide a common base implementation for a group of related classes, and use an interface when you want to define a contract for a capability that classes can implement.
This type of response demonstrates not only your understanding of the concept but also your ability to explain it clearly and provide relevant examples.
4. On-site Interviews: Diving Deep into Your Java Skills
On-site interviews (or multiple rounds of virtual interviews for remote positions) are typically the most comprehensive part of the Java engineer interview process. These interviews usually consist of several sessions, each focusing on different aspects of your skills and knowledge. Here’s what you can expect:
4.1 Coding Interviews
Coding interviews are a crucial part of the process. You’ll be asked to solve coding problems in Java, often on a whiteboard or using a shared coding environment. These problems test your ability to:
- Write clean, efficient, and bug-free code
- Implement data structures and algorithms
- Optimize solutions for time and space complexity
- Explain your thought process and approach to problem-solving
To prepare for coding interviews:
- Practice coding on a whiteboard or in a simple text editor without auto-completion
- Review common data structures (arrays, linked lists, trees, graphs, hash tables) and algorithms (sorting, searching, dynamic programming)
- Solve problems on platforms like LeetCode, HackerRank, or AlgoCademy
- Practice explaining your thought process out loud as you solve problems
Here’s an example of a coding question you might encounter:
Interviewer: Implement a function to reverse a linked list in Java.
Your solution:
public class ListNode {
int val;
ListNode next;
ListNode(int x) { val = x; }
}
public class Solution {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
ListNode next = null;
while (current != null) {
next = current.next;
current.next = prev;
prev = current;
current = next;
}
return prev;
}
}
Explanation:
This solution uses an iterative approach to reverse the linked list:
1. We initialize three pointers: prev (initially null), current (pointing to the head), and next (initially null).
2. We iterate through the list:
a. Save the next node
b. Reverse the current node's pointer
c. Move prev and current one step forward
3. At the end, prev will be pointing to the new head of the reversed list.
The time complexity is O(n), where n is the number of nodes in the list, as we traverse the list once.
The space complexity is O(1) as we only use a constant amount of extra space.
Remember to practice not just solving the problem, but also explaining your approach, discussing time and space complexity, and considering edge cases.
4.2 System Design Discussions
For more senior positions, you may be asked to participate in system design discussions. These questions assess your ability to design large-scale distributed systems using Java technologies. You might be asked to:
- Design a scalable web application
- Architect a distributed caching system
- Design a real-time data processing pipeline
To prepare for system design discussions:
- Study common system design patterns and architectures
- Familiarize yourself with distributed systems concepts (CAP theorem, consistency models, etc.)
- Learn about Java-specific technologies for building distributed systems (e.g., Spring Cloud, Apache Kafka)
- Practice explaining your design decisions and trade-offs
Here’s an example of a system design question:
Interviewer: Design a distributed task queue system using Java technologies.
Your approach:
1. Requirements gathering:
- Ability to enqueue and dequeue tasks
- Scalability to handle millions of tasks
- Fault tolerance and data persistence
- Task prioritization
2. High-level design:
- Use Apache Kafka as the message broker for task queue
- Implement producer services using Spring Boot to enqueue tasks
- Create consumer services using Spring Boot to process tasks
- Use Redis for task metadata and prioritization
- Utilize PostgreSQL for long-term task storage and analytics
3. Detailed design:
a. Task Producer:
- RESTful API built with Spring Boot
- Uses Kafka producer to send tasks to specific topics
b. Task Consumer:
- Spring Boot application with Kafka consumer
- Processes tasks based on priority
- Updates task status in Redis and PostgreSQL
c. Task Prioritization:
- Use Redis sorted sets to maintain task priorities
- Implement a priority-based consumer group assignment
d. Scalability and Fault Tolerance:
- Kafka partitioning for parallel processing
- Multiple consumer instances for load balancing
- Kafka's built-in replication for fault tolerance
e. Monitoring and Logging:
- Implement with Spring Boot Actuator and Micrometer
- Use ELK stack (Elasticsearch, Logstash, Kibana) for log aggregation
This design provides a scalable, fault-tolerant task queue system using Java technologies. It leverages Kafka's distributed nature for high throughput and Redis for fast access to task metadata and priorities. The use of Spring Boot simplifies development and provides robust integration with various components.
In a real interview, you would go into more detail for each component and be prepared to discuss trade-offs and alternative approaches.
4.3 Behavioral Interviews
Behavioral interviews assess your soft skills, work style, and cultural fit. You may be asked about:
- Past projects and your role in them
- How you handle conflicts or challenging situations
- Your approach to teamwork and collaboration
- Your learning process and how you stay updated with Java technologies
To prepare for behavioral interviews:
- Reflect on your past experiences and prepare specific examples
- Use the STAR method (Situation, Task, Action, Result) to structure your responses
- Practice articulating your thoughts clearly and concisely
- Research the company culture and values to align your responses
Here’s an example of a behavioral question and how you might approach it:
Interviewer: Can you describe a time when you had to optimize a Java application for better performance? What was your approach, and what was the outcome?
Your response: Certainly. In my previous role at XYZ Company, we had a Java-based web application that was experiencing significant slowdowns during peak usage times. The situation was critical as it was affecting user experience and potentially impacting our business.
Task: My task was to identify the performance bottlenecks and optimize the application to handle the increased load efficiently.
Action: I approached this challenge methodically:
1. Profiling: I used Java profiling tools like JProfiler to identify the hotspots in our code.
2. Database Optimization: I discovered that a major bottleneck was in our database queries. I optimized these by:
- Adding appropriate indexes
- Rewriting complex queries to be more efficient
- Implementing query caching using Hibernate second-level cache
3. Connection Pooling: I implemented proper connection pooling using HikariCP to reduce database connection overhead.
4. Caching: I introduced a distributed caching layer using Redis to cache frequently accessed data and reduce database load.
5. Asynchronous Processing: For non-critical operations, I implemented asynchronous processing using CompletableFuture to improve responsiveness.
6. Code Optimization: I refactored critical parts of the code, replacing inefficient data structures and algorithms with more optimal ones.
7. JVM Tuning: I fine-tuned JVM parameters, particularly garbage collection settings, to reduce pause times and improve overall performance.
Result: After implementing these optimizations:
- The application's response time improved by 60% during peak loads.
- We were able to handle 3x more concurrent users without increasing hardware resources.
- Database load reduced by 40%, leading to cost savings on our cloud infrastructure.
- User satisfaction scores improved significantly in our next customer survey.
This experience taught me the importance of systematic performance analysis and the power of various Java optimization techniques. It also highlighted the value of continuous monitoring and optimization in maintaining high-performance Java applications.
This response demonstrates your technical knowledge, problem-solving skills, and ability to deliver tangible results, which are all valuable traits for a Java engineer.
5. Coding Challenges or Take-Home Assignments
Some companies may include a coding challenge or take-home assignment as part of the interview process. These assignments are designed to assess your coding skills in a more realistic setting, allowing you to showcase your abilities without the pressure of an in-person interview.
Common types of assignments include:
- Building a small Java application or microservice
- Implementing a specific algorithm or data structure
- Solving a set of coding problems within a given timeframe
To excel in these challenges:
- Read the requirements carefully and ask for clarification if needed
- Plan your approach before starting to code
- Write clean, well-documented code
- Include unit tests to demonstrate your testing skills
- Optimize your solution for both time and space complexity
- Provide clear instructions on how to run your code
Here’s an example of a take-home assignment you might receive:
Assignment: Implement a RESTful API for a simple book management system using Spring Boot.
Requirements:
1. Create endpoints for CRUD operations on books
2. Each book should have: id, title, author, ISBN, and publication year
3. Implement proper error handling and input validation
4. Use an in-memory database (H2) for data storage
5. Include unit and integration tests
6. Provide documentation for the API endpoints
Here's a basic structure to get you started:
@RestController
@RequestMapping("/api/books")
public class BookController {
@Autowired
private BookService bookService;
@GetMapping
public List<Book> getAllBooks() {
return bookService.getAllBooks();
}
@GetMapping("/{id}")
public ResponseEntity<Book> getBookById(@PathVariable Long id) {
return bookService.getBookById(id)
.map(ResponseEntity::ok)
.orElse(ResponseEntity.notFound().build());
}
@PostMapping
public ResponseEntity<Book> createBook(@Valid @RequestBody Book book) {
Book savedBook = bookService.saveBook(book);
return ResponseEntity.created(URI.create("/api/books/" + savedBook.getId()))
.body(savedBook);
}
// Implement updateBook and deleteBook methods
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<Map<String, String>> handleValidationExceptions(MethodArgumentNotValidException ex) {
Map<String, String> errors = new HashMap<>();
ex.getBindingResult().getAllErrors().forEach((error) -> {
String fieldName = ((FieldError) error).getField();
String errorMessage = error.getDefaultMessage();
errors.put(fieldName, errorMessage);
});
return ResponseEntity.badRequest().body(errors);
}
}
// Implement BookService and Book entity
// Add necessary dependencies in pom.xml
// Configure application.properties for H2 database
// Write unit and integration tests
// Document API using Swagger or a README file
Remember to expand on this basic structure, implement all required functionalities, and follow best practices for Java and Spring Boot development.
6. Final Tips for Success
As you prepare for your Java engineer interview, keep these final tips in mind:
- Stay updated with the latest Java versions and features
- Practice coding regularly, focusing on both algorithmic problems and real-world scenarios
- Contribute to open-source Java projects to gain practical experience
- Be prepared to discuss your past projects in detail
- Show enthusiasm for learning and adapting to new technologies
- Ask thoughtful questions about the role and the company
- Follow up after the interview to express your continued interest
Conclusion
The Java engineer interview process can be challenging, but with proper preparation and the right mindset, you can showcase your skills effectively. Remember that interviewers are not just looking for technical expertise, but also for problem-solving abilities, communication skills, and cultural fit. By understanding the anatomy of a Java engineer interview and preparing thoroughly for each component, you’ll be well-equipped to succeed in your job search.
As you continue your journey in Java development, platforms like AlgoCademy can be invaluable resources for honing your skills, practicing coding problems, and preparing for technical interviews. With dedication and persistent effort, you’ll be well on your way to landing your dream job as a Java engineer. Good luck!