The Anatomy of a C++ Engineer Interview: A Comprehensive Guide
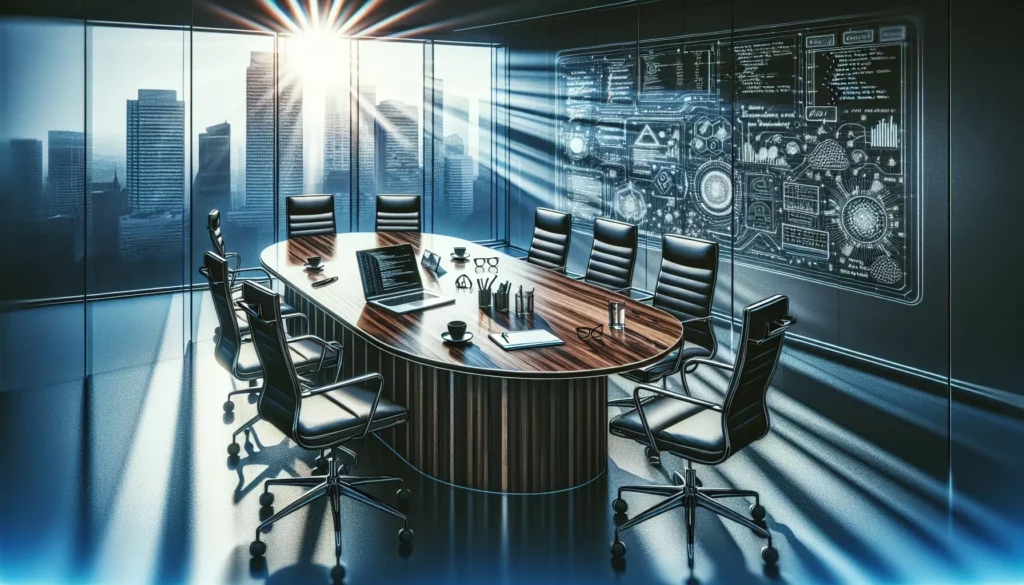
In the competitive landscape of software engineering, C++ continues to be a highly sought-after skill, especially in industries that demand high-performance computing, such as game development, financial systems, and embedded systems. For aspiring C++ engineers, understanding the intricacies of the interview process is crucial. This comprehensive guide will dissect the anatomy of a C++ engineer interview, providing you with insights and strategies to excel in your next career opportunity.
1. The Importance of C++ in Modern Software Engineering
Before diving into the interview process, it’s essential to understand why C++ remains a critical language in the software engineering ecosystem:
- Performance: C++ offers low-level memory manipulation and high-level abstractions, making it ideal for performance-critical applications.
- Versatility: It supports multiple programming paradigms, including object-oriented, procedural, and generic programming.
- Legacy Systems: Many large-scale systems and codebases are written in C++, requiring ongoing maintenance and development.
- Cross-platform Development: C++ allows for efficient cross-platform development, crucial in today’s diverse computing environments.
Given these factors, companies are always on the lookout for skilled C++ engineers who can navigate complex codebases and optimize system performance.
2. Preparing for the C++ Interview
Preparation is key to success in any interview, and C++ interviews are no exception. Here’s a structured approach to getting ready:
2.1. Brush Up on C++ Fundamentals
Ensure you have a solid grasp of C++ basics, including:
- Object-Oriented Programming (OOP) concepts
- Memory management and pointers
- Templates and generic programming
- Standard Template Library (STL)
- Exception handling
- Multithreading and concurrency
2.2. Stay Updated with Modern C++
C++ has evolved significantly over the years. Familiarize yourself with modern C++ features introduced in C++11, C++14, C++17, and C++20, such as:
- Auto keyword and type inference
- Lambda expressions
- Smart pointers
- Move semantics
- Variadic templates
- Concepts (C++20)
2.3. Practice Coding Problems
Solve C++-specific coding problems on platforms like LeetCode, HackerRank, or CodeForces. Focus on:
- Data structures implementation
- Algorithm optimization
- Memory management challenges
- Concurrency problems
2.4. Understand System Design
For senior roles, system design knowledge is crucial. Study:
- Scalable system architectures
- Design patterns relevant to C++
- Performance optimization techniques
- Multithreaded system design
3. The Structure of a C++ Engineer Interview
C++ engineer interviews often follow a multi-stage process, each designed to evaluate different aspects of your skills and knowledge:
3.1. Initial Screening
This typically involves a phone or video call with a recruiter or hiring manager. They’ll assess:
- Your background and experience with C++
- Basic technical knowledge
- Your interest in the role and company
3.2. Technical Phone Screen
This is usually conducted by an engineer and involves:
- Coding questions (often using a shared online editor)
- C++-specific conceptual questions
- Discussion about your past projects and technical decisions
3.3. Take-Home Coding Assignment
Some companies may give you a project to complete in your own time. This could involve:
- Implementing a specific feature or algorithm in C++
- Optimizing existing code
- Designing a small system or component
3.4. On-Site Interviews
The final stage usually consists of multiple rounds, including:
- Coding interviews: Solving problems on a whiteboard or computer
- System design interviews: Discussing high-level architecture and design decisions
- Behavioral interviews: Assessing your soft skills and cultural fit
- Technical deep dive: In-depth discussions about C++ internals and your expertise
4. Common C++ Interview Questions and Topics
While the specific questions can vary, certain topics frequently appear in C++ interviews:
4.1. Language Fundamentals
- Explain the difference between stack and heap memory allocation.
- What are virtual functions and how do they work?
- Describe the Rule of Three (or Rule of Five in modern C++).
- How does multiple inheritance work in C++?
4.2. Memory Management
- What are smart pointers and when would you use them?
- Explain RAII (Resource Acquisition Is Initialization).
- How do you prevent memory leaks in C++?
4.3. STL and Templates
- Compare std::vector and std::list. When would you choose one over the other?
- Explain how templates work and provide an example of a function template.
- What are the differences between std::map and std::unordered_map?
4.4. Multithreading and Concurrency
- What is a race condition and how can it be prevented?
- Explain the difference between std::mutex and std::atomic.
- How does std::async work?
4.5. Modern C++ Features
- What are lambda expressions and how are they useful?
- Explain move semantics and perfect forwarding.
- How do you use auto type deduction effectively?
5. Coding Challenges: What to Expect
Coding challenges in C++ interviews often focus on demonstrating your ability to write efficient, clean, and correct code. Here are some types of problems you might encounter:
5.1. Data Structure Implementation
You might be asked to implement a data structure from scratch, such as:
- A custom hash table
- A thread-safe queue
- A memory-efficient trie
Here’s an example of a simple thread-safe queue implementation:
#include <queue>
#include <mutex>
#include <condition_variable>
template<typename T>
class ThreadSafeQueue {
private:
std::queue<T> queue_;
mutable std::mutex mutex_;
std::condition_variable cond_;
public:
void push(T value) {
std::lock_guard<std::mutex> lock(mutex_);
queue_.push(std::move(value));
cond_.notify_one();
}
T pop() {
std::unique_lock<std::mutex> lock(mutex_);
cond_.wait(lock, [this]{ return !queue_.empty(); });
T value = std::move(queue_.front());
queue_.pop();
return value;
}
bool empty() const {
std::lock_guard<std::mutex> lock(mutex_);
return queue_.empty();
}
};
5.2. Algorithm Optimization
You may be given a problem that requires optimizing an algorithm for better time or space complexity. For example:
// Optimize this function to find the kth largest element in an unsorted array
int findKthLargest(std::vector<int>& nums, int k) {
std::sort(nums.begin(), nums.end(), std::greater<int>());
return nums[k-1];
}
An optimized solution might use the quickselect algorithm:
#include <vector>
#include <algorithm>
class Solution {
public:
int findKthLargest(std::vector<int>& nums, int k) {
return quickSelect(nums, 0, nums.size() - 1, nums.size() - k);
}
private:
int quickSelect(std::vector<int>& nums, int left, int right, int k) {
if (left == right) return nums[left];
int pivotIndex = partition(nums, left, right);
if (k == pivotIndex) return nums[k];
else if (k < pivotIndex) return quickSelect(nums, left, pivotIndex - 1, k);
else return quickSelect(nums, pivotIndex + 1, right, k);
}
int partition(std::vector<int>& nums, int left, int right) {
int pivot = nums[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (nums[j] <= pivot) {
i++;
std::swap(nums[i], nums[j]);
}
}
std::swap(nums[i + 1], nums[right]);
return i + 1;
}
};
5.3. Memory Management Challenges
You might be asked to identify and fix memory leaks or implement custom memory management. For example:
class Resource {
public:
Resource() { data = new int[100]; }
~Resource() { delete[] data; }
Resource(const Resource& other) {
data = new int[100];
std::copy(other.data, other.data + 100, data);
}
Resource& operator=(const Resource& other) {
if (this != &other) {
delete[] data;
data = new int[100];
std::copy(other.data, other.data + 100, data);
}
return *this;
}
private:
int* data;
};
In this case, you might be asked to identify potential issues (like the lack of move semantics) and implement improvements.
5.4. Concurrency Problems
Given the importance of multithreading in C++, you might encounter problems related to concurrent programming. For instance, implementing a thread-safe singleton:
#include <mutex>
class Singleton {
public:
static Singleton& getInstance() {
static Singleton instance;
return instance;
}
Singleton(const Singleton&) = delete;
Singleton& operator=(const Singleton&) = delete;
private:
Singleton() = default;
};
This implementation uses the C++11 magic static feature, which ensures thread-safe initialization.
6. System Design in C++ Interviews
For senior roles, system design questions are common. These assess your ability to architect large-scale systems using C++. Key areas to focus on include:
6.1. Scalability
Understand how to design systems that can handle increasing loads. This might involve:
- Distributed systems concepts
- Load balancing techniques
- Caching strategies
6.2. Performance Optimization
Be prepared to discuss techniques for optimizing C++ applications, such as:
- Profiling and benchmarking
- Memory pooling
- Lock-free data structures
6.3. Design Patterns
Familiarize yourself with common design patterns in C++, including:
- Singleton (as shown earlier)
- Factory Method
- Observer
- Strategy
6.4. Multithreaded System Design
Understand how to design systems that effectively utilize multiple threads, considering:
- Thread pooling
- Task-based parallelism
- Synchronization mechanisms
7. Behavioral Aspects of the Interview
While technical skills are crucial, soft skills and cultural fit are equally important. Be prepared to discuss:
7.1. Past Projects
Have detailed examples of C++ projects you’ve worked on, focusing on:
- Technical challenges you overcame
- Your role in the project
- The impact of your contributions
7.2. Teamwork and Communication
Be ready to discuss how you:
- Collaborate with team members
- Handle conflicts or disagreements
- Communicate technical concepts to non-technical stakeholders
7.3. Problem-Solving Approach
Articulate your approach to solving complex problems, including:
- How you break down large problems
- Your debugging strategies
- How you stay updated with new C++ developments
8. Post-Interview: The Follow-Up
After the interview, consider these steps:
- Send a thank-you email to your interviewers
- Reflect on the interview questions and your responses
- If you don’t get the job, ask for feedback to improve for future interviews
9. Conclusion: Mastering the C++ Engineer Interview
Succeeding in a C++ engineer interview requires a combination of deep technical knowledge, problem-solving skills, and effective communication. By understanding the anatomy of these interviews and preparing thoroughly, you can significantly increase your chances of landing your dream C++ role.
Remember, the key to success lies not just in knowing C++, but in demonstrating how you can apply that knowledge to solve real-world problems efficiently and elegantly. Stay curious, keep practicing, and approach each interview as an opportunity to learn and grow as a C++ engineer.
As you continue your journey in C++ development, platforms like AlgoCademy can be invaluable resources. They offer interactive coding tutorials, curated problem sets, and AI-powered assistance to help you sharpen your skills and prepare for technical interviews at top tech companies. Whether you’re a beginner looking to build a strong foundation in C++ or an experienced developer aiming to crack FAANG interviews, continuous learning and practice are your best allies in the ever-evolving world of software engineering.