Top Interview Coding Questions You Must Know
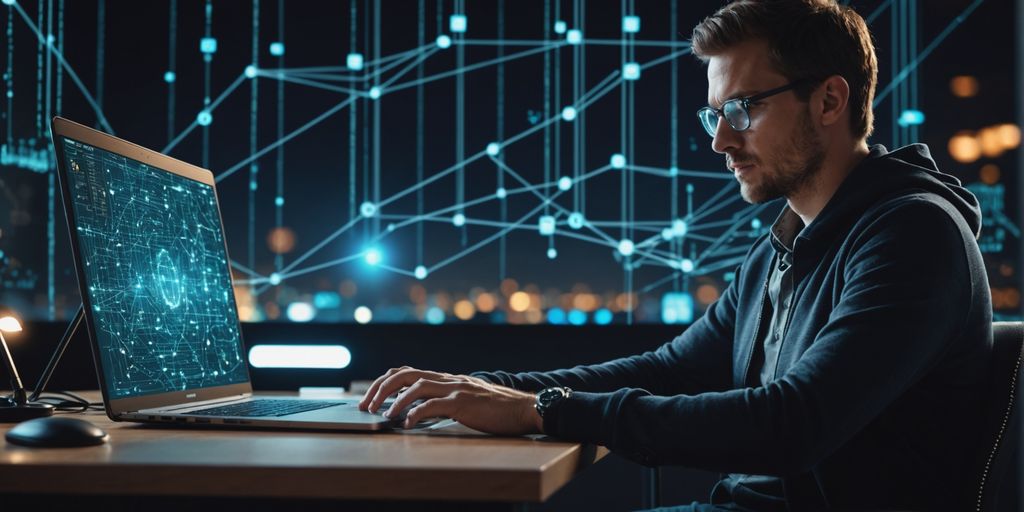
Coding interviews can be tough, but they’re a key part of landing a job in software development. Knowing the right questions and how to answer them can make all the difference. This article covers the top coding questions you should know to ace your interview.
Key Takeaways
- Reversing a string is a common interview question that tests your understanding of string manipulation.
- Knowing how to check if a string is a palindrome is essential for demonstrating your ability to work with strings.
- Counting numerical digits in a string shows your ability to handle different types of data within strings.
- Finding the occurrence of a particular character in a string is a basic yet important skill in text processing.
- Being able to identify non-matching characters in a string tests your attention to detail and comparison skills.
1. How to Reverse a String
Reversing a string is a common algorithms interview question. This question essentially asks for a function that takes a string as input and returns a new string which is the reverse of the input string.
Steps to Reverse a String
- Convert the string to a list of characters: This allows you to manipulate each character individually.
- Use a loop to swap characters: Start from the beginning and end of the list, swapping characters until you reach the middle.
- Join the list back into a string: After all characters are swapped, join the list back into a string.
Example in Python
# Function to reverse a string
def reverse_string(s):
return s[::-1]
# Test the function
input_string = "hello"
reversed_string = reverse_string(input_string)
print(reversed_string) # Output: "olleh"
Reversing a string is a simple yet fundamental task that helps in understanding basic string manipulation techniques.
2. How to Determine if a String is a Palindrome
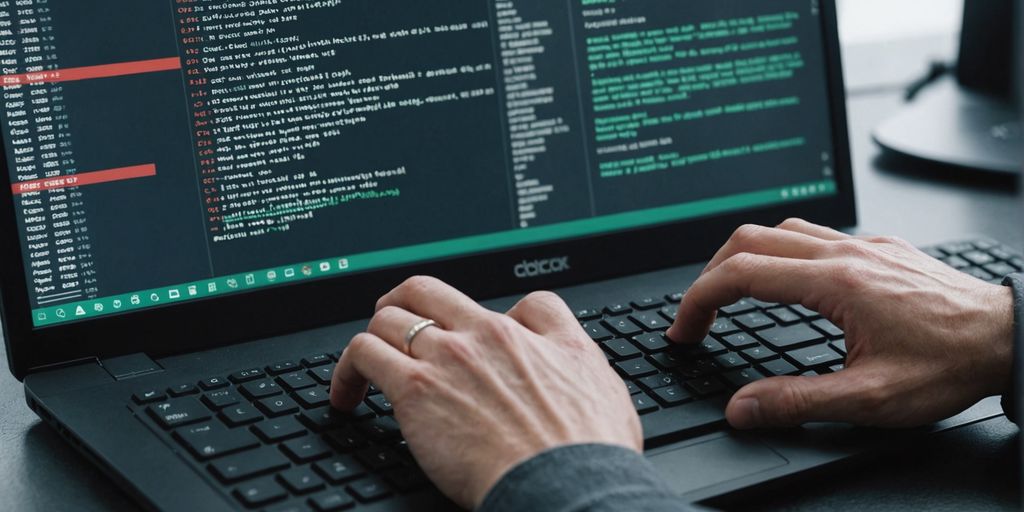
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward (ignoring spaces, punctuation, and capitalization). To determine if a string is a palindrome, you can use various methods in different programming languages.
Steps to Check for a Palindrome in Python
- Take user input: Get the string from the user.
- Reverse the string: You can use slicing (
[s == s[::-1]](https://www.geeksforgeeks.org/python-program-check-string-palindrome-not/)
) or reversing techniques (s == ''.join(reversed(s))
) to check if a string is a palindrome. - Compare the original and reversed strings: If they are the same, the string is a palindrome.
Example Code in Python
# Function to check if a string is a palindrome
def isPalindrome(s):
return s == s[::-1]
# Driver code
s = "malayalam"
ans = isPalindrome(s)
if ans:
print("Yes")
else:
print("No")
Note: This method works by comparing the original string with its reversed version. If they match, the string is a palindrome.
Example Code in Java
import java.util.Scanner;
public class Palindrome {
public static void main(String[] args) {
Scanner reader = new Scanner(System.in);
System.out.println("Please enter a String");
String input = reader.nextLine();
System.out.printf("Is %s a palindrome? : %b %n", input, isPalindrome(input));
reader.close();
}
public static boolean isPalindrome(String input) {
if (input == null || input.isEmpty()) {
return true;
}
String reverse = new StringBuilder(input).reverse().toString();
return input.equals(reverse);
}
}
Example Code in C++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[100];
cout << "Enter the string: ";
cin >> str;
int length = strlen(str);
bool isPalindrome = true;
for (int i = 0; i < length / 2; i++) {
if (str[i] != str[length - i - 1]) {
isPalindrome = false;
break;
}
}
if (isPalindrome) {
cout << "String is a palindrome";
} else {
cout << "String is not a palindrome";
}
return 0;
}
Checking if a string is a palindrome is a common coding question that tests your understanding of string manipulation and comparison techniques.
3. How to Calculate the Number of Numerical Digits in a String
Counting the number of numerical digits in a string is a common task in coding interviews. This problem can be solved using various methods, including loops and built-in functions.
Steps to Count Numerical Digits
- Initialize a Counter: Start by setting a counter to zero. This will keep track of the number of digits.
- Iterate Through the String: Loop through each character in the string.
- Check for Digits: Use a condition to check if the current character is a digit (0-9).
- Increment the Counter: If the character is a digit, increment the counter by one.
- Return the Counter: After the loop ends, the counter will hold the number of numerical digits in the string.
Example Code
Here is a simple example in Python:
string = "Hello123World"
counter = 0
for char in string:
if char.isdigit():
counter += 1
print("Number of digits:", counter)
Important Note
You can use the isdigit() method in Python to easily check if a character is a digit. This method returns True if all characters in the string are digits and there is at least one character, otherwise it returns False.
Alternative Method
Another way to count digits is by using the re
(regular expressions) module in Python:
import re
string = "Hello123World"
digits = re.findall(r'\d', string)
print("Number of digits:", len(digits))
This method uses a regular expression to find all digits in the string and then counts them.
Logarithmic Approach
For those interested in mathematical methods, we can use log10
(logarithm of base 10) to count the number of digits of positive numbers. However, this approach is more commonly used for counting digits in an integer rather than a string.
By understanding and practicing these methods, you’ll be well-prepared to tackle this common interview question.
4. How to Find the Count for the Occurrence of a Particular Character in a String
To find out how many times a specific character appears in a string, you can use a simple loop. This method is straightforward and effective. Here’s a step-by-step guide:
- Initialize a count variable to zero.
- Loop through each character in the string.
- For each character, check if it matches the character you’re looking for.
- If it matches, increment the count variable.
- After the loop, the count variable will hold the number of occurrences of the character.
Here’s a sample code snippet in Java:
int count = 0;
char search = 'a';
String str = "example string";
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == search) {
count++;
}
}
System.out.println(count);
In JavaScript, we can count the string occurrence in a string by counting the number of times the string is present in the string.
This method works similarly in other programming languages like Python, C++, and JavaScript.
5. How to Find the Non-Matching Characters in a String
Finding non-matching characters between two strings can be a common interview question. This involves identifying characters that are present in one string but not in the other.
Steps to Find Non-Matching Characters
- Initialize two sets: One for each string to store unique characters.
- Iterate through each string: Add each character to its respective set.
- Find the difference: Use set operations to find characters that are in one set but not the other.
Here’s a simple example to illustrate:
str1 = "apple"
str2 = "grape"
set1 = set(str1)
set2 = set(str2)
non_matching = set1.symmetric_difference(set2)
print(non_matching)
In this example, the non-matching characters between "apple"
and "grape"
are found using set operations.
Tip: Using sets makes it easy to find unique characters and perform operations like union, intersection, and difference.
By following these steps, you can efficiently find the non-matching characters in any two given strings.
6. How to Find Out if Two Given Strings are Anagrams
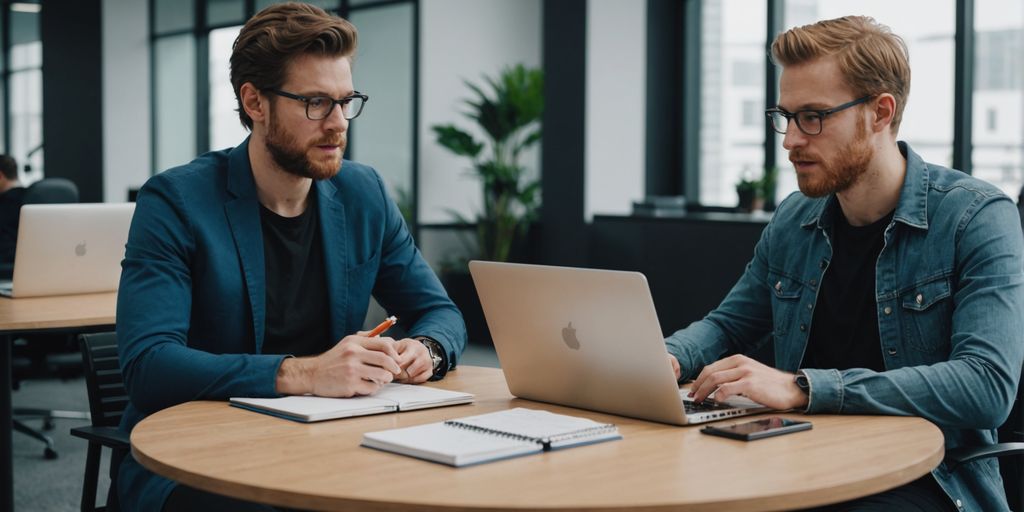
To check whether two strings are anagrams of each other, we can simply sort the two given strings and compare them – if they are equal, then the original strings are anagrams of each other.
Steps to Determine if Two Strings are Anagrams
- Check the Lengths: If the lengths of the two strings are not the same, they cannot be anagrams.
- Sort the Strings: Convert both strings to character arrays and sort them.
- Compare the Sorted Strings: If the sorted arrays are equal, the strings are anagrams; otherwise, they are not.
Example in Python
# Take user input
String1 = input('Enter the 1st string: ')
String2 = input('Enter the 2nd string: ')
# Check if lengths match
if len(String1) != len(String2):
print('Strings are not anagram')
else:
# Sort the strings
String1 = sorted(String1)
String2 = sorted(String2)
# Compare sorted strings
if String1 == String2:
print('Strings are anagram')
else:
print('Strings are not anagram')
Anagrams are words or phrases formed by rearranging the letters of another, using all the original letters exactly once.
7. How to Calculate the Number of Vowels and Consonants in a String
Counting the number of vowels and consonants in a string is a common task in programming. Here’s a simple way to do it using a loop.
Steps to Count Vowels and Consonants
- Loop through each character in the string.
- Check if the character is a vowel (a, e, i, o, u).
- If it is, increase the vowel count by one.
- If it is not a vowel and is a letter, increase the consonant count by one.
- Print the counts of both vowels and consonants.
Example Code in Python
# Function to count vowels and consonants
def count_vowels_and_consonants(s):
vowels = 0
consonants = 0
for char in s:
if char.lower() in 'aeiou':
vowels += 1
elif char.isalpha():
consonants += 1
return vowels, consonants
# Main program
string = input("Enter a string: ")
vowels, consonants = count_vowels_and_consonants(string)
print(f"Vowels: {vowels}, Consonants: {consonants}")
This program prompts the user to enter a string and then counts the number of vowels and consonants using the count_vowels_and_consonants function.
8. How to Total All of the Matching Integer Elements in an Array
When working with arrays, you might need to find the sum of all elements that match a certain value. This is a common task in coding interviews. Here’s a simple way to do it.
- Declare an array: Start by declaring an array of integers.
- Initialize a sum variable: This will hold the total of the matching elements.
- Loop through the array: Use a loop to go through each element in the array.
- Check for matching elements: Inside the loop, check if the current element matches the value you’re looking for.
- Add to sum: If it matches, add the element to the sum variable.
- Print the result: After the loop, print the sum.
Here’s a sample code snippet in Java:
int[] array = {1, 2, 3, 4, 2, 2, 5};
int target = 2;
int sum = 0;
for (int i = 0; i < array.length; i++) {
if (array[i] == target) {
sum += array[i];
}
}
System.out.println("Total of matching elements: " + sum);
This method ensures you efficiently find and sum all matching elements in the array. It’s a straightforward approach that can be easily adapted to other programming languages.
9. How to Reverse an Array
Reversing an array is a common task in coding interviews. It involves changing the order of elements so that the first element becomes the last, the second becomes the second last, and so on. This can be done in various programming languages like C, C++, Java, Python, and JavaScript.
Steps to Reverse an Array
- Initialize two pointers: One at the start (index 0) and one at the end (last index) of the array.
- Swap the elements at these two pointers.
- Move the pointers towards each other: increment the start pointer and decrement the end pointer.
- Repeat the process until the pointers meet or cross each other.
Example in Python
def reverse_array(arr):
start = 0
end = len(arr) - 1
while start < end:
arr[start], arr[end] = arr[end], arr[start]
start += 1
end -= 1
return arr
# Example usage
array = [1, 2, 3, 4, 5]
print(reverse_array(array)) # Output: [5, 4, 3, 2, 1]
Reversing an array is a fundamental skill that can be applied in various scenarios, such as data manipulation and algorithm optimization.
10. How to Find the Maximum Element in an Array
Finding the maximum element in an array is a common task in coding interviews. This problem tests your understanding of array traversal and comparison operations. Here’s a simple way to do it:
- Initialize a variable to store the maximum value. Set it to the first element of the array.
- Loop through the array starting from the second element.
- Compare each element with the current maximum value. If an element is greater, update the maximum value.
- Return the maximum value after the loop ends.
Here’s a sample code snippet in JavaScript:
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
The Math object’s Math.min() and Math.max() methods are static methods that return the minimum and maximum elements of a given array. The spread operator (…) can be used to pass the array elements as individual arguments.
This method has a time complexity of O(n), where n is the number of elements in the array. It’s efficient and straightforward, making it a go-to solution for finding the maximum element in an array.
Conclusion
Mastering coding interview questions is a crucial step in landing your dream job in software development. By familiarizing yourself with common questions and practicing your problem-solving skills, you can approach your interview with confidence. Remember, it’s not just about getting the right answer but also about demonstrating your thought process and ability to tackle challenges. Keep practicing, stay curious, and you’ll be well on your way to acing your coding interviews.
Frequently Asked Questions
What is the best way to reverse a string?
To reverse a string, you can use a loop to swap characters from the beginning and end of the string, moving towards the center. Alternatively, many programming languages offer built-in functions to reverse strings.
How can I check if a string is a palindrome?
A string is a palindrome if it reads the same backward as forward. You can check this by comparing the string to its reversed version.
What is the simplest method to count numerical digits in a string?
To count numerical digits in a string, loop through each character and check if it is a digit using a function like `isdigit()` in Python. Increment a counter for each digit you find.
How do I find how many times a character appears in a string?
You can find the count of a particular character in a string by looping through the string and incrementing a counter each time the character is found. Many languages also have built-in functions for this.
What are anagrams and how can I check if two strings are anagrams?
Anagrams are words or phrases formed by rearranging the letters of another. To check if two strings are anagrams, sort the characters in both strings and compare the sorted versions.
How can I count vowels and consonants in a string?
To count vowels and consonants, loop through the string and check each character. If it’s a vowel (a, e, i, o, u), increment the vowel counter; otherwise, if it’s a consonant, increment the consonant counter.