The Anatomy of a Blockchain Developer Interview: What to Do at Each Step
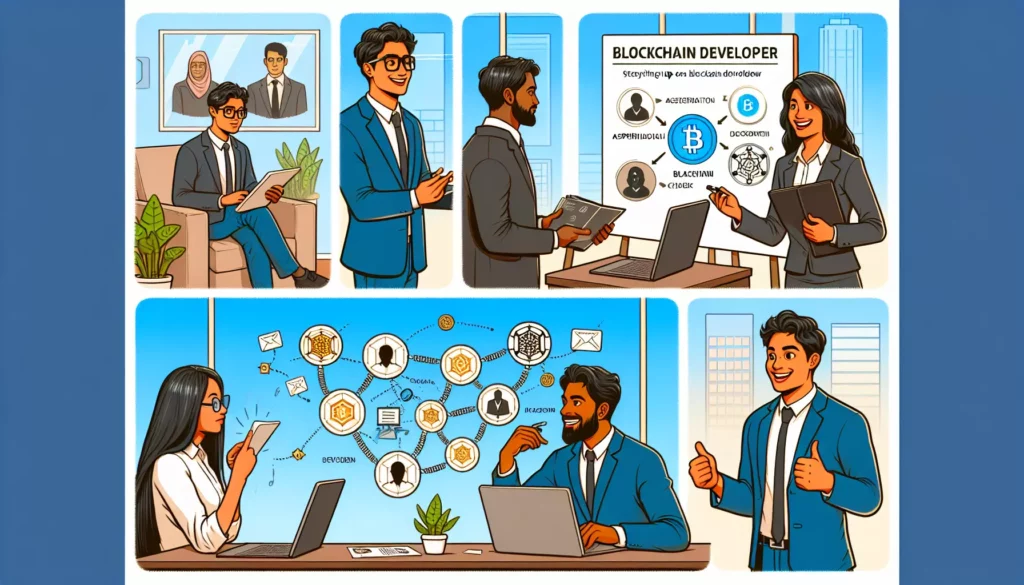
As the blockchain industry continues to grow and evolve, the demand for skilled blockchain developers has skyrocketed. Whether you’re a seasoned developer looking to transition into blockchain or a newcomer to the field, understanding the intricacies of a blockchain developer interview is crucial for landing your dream job. In this comprehensive guide, we’ll dissect the anatomy of a blockchain developer interview and provide you with actionable strategies for each step of the process.
1. Pre-Interview Preparation
Before you even step foot in the interview room (or join the video call), thorough preparation is key to success. Here’s what you should focus on:
1.1. Brush Up on Blockchain Fundamentals
Ensure you have a solid grasp of blockchain basics, including:
- Distributed ledger technology
- Consensus mechanisms (e.g., Proof of Work, Proof of Stake)
- Smart contracts
- Cryptography principles
- Blockchain architecture
1.2. Stay Updated on Latest Trends
The blockchain space evolves rapidly. Stay informed about:
- Recent developments in major blockchain platforms (e.g., Ethereum, Binance Smart Chain, Solana)
- Emerging technologies like Layer 2 solutions and interoperability protocols
- Regulatory changes affecting the blockchain industry
1.3. Review Your Projects
Be prepared to discuss your previous blockchain projects in detail. For each project:
- Outline the problem it solved
- Explain your role and contributions
- Highlight challenges faced and how you overcame them
- Discuss the technologies and tools used
1.4. Practice Coding Exercises
Many blockchain developer interviews include coding challenges. Practice:
- Smart contract development (e.g., Solidity for Ethereum)
- Data structures and algorithms relevant to blockchain
- Debugging and optimizing blockchain-specific code
2. The Initial Screening
The first step in many blockchain developer interviews is an initial screening, often conducted by a recruiter or HR representative. Here’s how to navigate this stage:
2.1. Prepare a Concise Introduction
Craft a 2-3 minute introduction that highlights:
- Your background in software development
- Your experience with blockchain technology
- Key projects or achievements relevant to the position
- Your motivation for pursuing a career in blockchain
2.2. Research the Company
Show genuine interest by researching:
- The company’s blockchain products or services
- Recent news or announcements
- The company’s mission and values
2.3. Prepare Questions
Have thoughtful questions ready about:
- The specific role and team structure
- The company’s blockchain technology stack
- Opportunities for growth and learning
2.4. Be Ready for Basic Technical Questions
While the initial screening may not be deeply technical, be prepared to answer high-level questions about:
- Your familiarity with different blockchain platforms
- Your experience with smart contract languages
- Your understanding of blockchain security principles
3. The Technical Interview
The technical interview is where your blockchain expertise will be put to the test. Here’s how to approach this crucial stage:
3.1. Whiteboard Coding
Many interviews include whiteboard coding exercises. To excel:
- Practice explaining your thought process as you code
- Start with a high-level approach before diving into details
- Consider edge cases and potential optimizations
- Be prepared to write pseudocode or actual code for blockchain-specific problems
3.2. Smart Contract Development
Expect questions or exercises related to smart contract development:
- Be ready to write simple smart contracts (e.g., a token contract or a basic DeFi protocol)
- Explain gas optimization techniques
- Discuss security considerations in smart contract development
Here’s an example of a simple ERC-20 token contract in Solidity:
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
}
3.3. Blockchain Architecture Questions
Be prepared to discuss blockchain architecture in depth:
- Explain the differences between public, private, and consortium blockchains
- Discuss scalability solutions (e.g., sharding, sidechains)
- Describe the process of adding a new block to the chain
3.4. Cryptography and Security
Demonstrate your understanding of blockchain security:
- Explain concepts like public-key cryptography and digital signatures
- Discuss common attack vectors (e.g., 51% attack, double-spending) and prevention measures
- Describe best practices for securing blockchain applications
3.5. Problem-Solving Scenarios
Interviewers may present you with blockchain-specific problem-solving scenarios. For example:
- “How would you design a decentralized voting system?”
- “Explain how you would implement a cross-chain token bridge.”
- “Describe an approach to scaling a popular DeFi protocol.”
When tackling these scenarios:
- Break down the problem into smaller components
- Consider trade-offs between different approaches
- Discuss potential challenges and how to address them
4. The System Design Interview
For more senior positions, you may encounter a system design interview focused on blockchain systems. Here’s how to approach it:
4.1. Understand the Requirements
Before diving into the design:
- Clarify the scope and constraints of the system
- Identify the key features and functionality required
- Discuss any assumptions you’re making
4.2. High-Level Design
Start with a high-level architecture:
- Sketch out the main components of the system
- Explain the role of each component
- Discuss how data flows through the system
4.3. Dive into Specifics
As you refine the design, consider:
- Choice of blockchain platform and justification
- On-chain vs. off-chain data storage
- Consensus mechanism and its implications
- Integration with external systems (e.g., oracles)
- Scalability and performance optimizations
4.4. Address Security and Privacy
Discuss how your design addresses:
- Data privacy and GDPR compliance (if applicable)
- Protection against common blockchain vulnerabilities
- Key management and access control
4.5. Consider Trade-offs
Be prepared to discuss trade-offs in your design:
- Centralization vs. decentralization
- Performance vs. security
- Complexity vs. maintainability
5. The Behavioral Interview
Don’t underestimate the importance of the behavioral interview. Blockchain projects often involve complex, collaborative work, and companies want to ensure you’ll be a good fit for their team.
5.1. Use the STAR Method
When answering behavioral questions, use the STAR method:
- Situation: Describe the context
- Task: Explain your responsibility
- Action: Detail the steps you took
- Result: Share the outcome and lessons learned
5.2. Prepare for Common Questions
Be ready to answer questions like:
- “Tell me about a time you had to explain a complex blockchain concept to a non-technical stakeholder.”
- “Describe a situation where you had to debug a particularly challenging smart contract issue.”
- “How do you stay updated with the rapidly evolving blockchain landscape?”
5.3. Highlight Soft Skills
Emphasize soft skills crucial for blockchain development:
- Adaptability to rapidly changing technologies
- Collaboration with cross-functional teams
- Clear communication of complex ideas
- Problem-solving and critical thinking
5.4. Show Your Passion
Demonstrate your enthusiasm for blockchain technology:
- Discuss personal projects or contributions to open-source blockchain initiatives
- Share your thoughts on the future of blockchain and its potential impact
- Explain what drew you to blockchain development
6. The Take-Home Assignment
Some companies may give you a take-home assignment to assess your practical skills. Here’s how to approach it:
6.1. Read the Instructions Carefully
- Understand all requirements before starting
- Note any specific technologies or frameworks you’re expected to use
- Pay attention to submission deadlines
6.2. Plan Your Approach
- Break down the project into manageable tasks
- Allocate time for coding, testing, and documentation
- Consider starting with a minimal viable solution and then adding enhancements
6.3. Focus on Quality
- Write clean, well-documented code
- Implement proper error handling and edge case management
- Include unit tests to demonstrate code reliability
6.4. Go the Extra Mile
- If time allows, add extra features or optimizations
- Provide a detailed README explaining your approach and any assumptions made
- Include instructions for running and testing your solution
7. Post-Interview Follow-Up
After the interview process, follow these steps to leave a lasting positive impression:
7.1. Send a Thank-You Note
- Email the interviewer(s) within 24 hours
- Express gratitude for their time and the opportunity
- Reiterate your interest in the position
7.2. Provide Additional Information
- If you thought of a better solution to a problem discussed, share it
- Send links to relevant projects or articles you mentioned during the interview
7.3. Ask About Next Steps
- Inquire about the timeline for the hiring decision
- Express your willingness to provide any additional information if needed
Conclusion
Navigating a blockchain developer interview can be challenging, but with thorough preparation and the right approach, you can showcase your skills and passion for the technology. Remember that blockchain development is not just about coding – it’s about understanding the broader implications of decentralized systems and being able to solve complex problems in innovative ways.
As you prepare for your interview, focus on deepening your technical knowledge, honing your problem-solving skills, and articulating your ideas clearly. Stay curious, keep learning, and don’t be afraid to tackle challenging projects to build your expertise.
The blockchain industry is still in its early stages, and companies are looking for developers who can grow with the technology. By demonstrating your technical prowess, adaptability, and passion for blockchain, you’ll be well-positioned to ace your interview and launch an exciting career in this revolutionary field.
Good luck with your blockchain developer interview journey!