The Anatomy of a Mobile Developer Interview: What to Do at Each Step
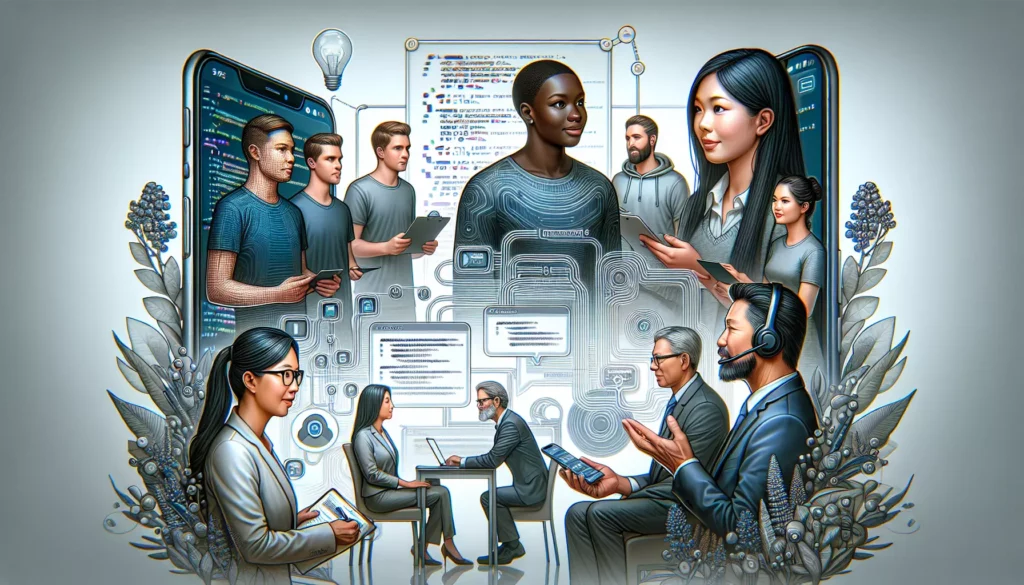
In today’s competitive tech landscape, mobile development has become one of the most sought-after skills. As companies continue to prioritize mobile-first strategies, the demand for talented mobile developers is on the rise. If you’re aspiring to land a coveted position as a mobile developer, understanding the intricacies of the interview process is crucial. This comprehensive guide will walk you through the anatomy of a mobile developer interview, providing valuable insights and actionable tips for each step along the way.
1. Pre-Interview Preparation
Before you even step foot in the interview room (or join a video call), thorough preparation is key to your success. Here’s what you should focus on:
1.1. Research the Company
Understanding the company you’re interviewing with is crucial. Look into:
- The company’s history and culture
- Their mobile products and apps
- Recent news or developments
- Their tech stack and development methodologies
This knowledge will not only help you answer questions more effectively but also demonstrate your genuine interest in the role.
1.2. Review Your Resume
Ensure you’re familiar with every detail on your resume. Be prepared to discuss:
- Your past projects and their technical challenges
- Specific contributions you made to team efforts
- Technologies and frameworks you’ve worked with
1.3. Brush Up on Mobile Development Fundamentals
Refresh your knowledge of core mobile development concepts, including:
- Platform-specific guidelines (iOS Human Interface Guidelines, Android Material Design)
- Mobile app architecture patterns (MVC, MVVM, MVP)
- Performance optimization techniques
- Security best practices for mobile apps
1.4. Practice Coding Problems
Many mobile developer interviews include coding challenges. Practice solving problems related to:
- Data structures and algorithms
- Mobile-specific scenarios (e.g., efficient list rendering, caching strategies)
- Platform-specific languages (Swift for iOS, Kotlin for Android)
2. The Initial Screening
The first step in many interview processes is an initial screening, often conducted by a recruiter or HR representative. Here’s what to expect and how to prepare:
2.1. Common Questions
Be ready to answer questions such as:
- “Tell me about yourself and your background in mobile development.”
- “Why are you interested in this position?”
- “What are your salary expectations?”
- “Can you describe a challenging mobile project you’ve worked on?”
2.2. Tips for Success
- Keep your answers concise but informative
- Highlight your most relevant experiences and skills
- Show enthusiasm for the role and company
- Be honest about your expectations and availability
2.3. Questions to Ask
Prepare a few questions to ask the recruiter, such as:
- “Can you tell me more about the team I’d be working with?”
- “What does the rest of the interview process look like?”
- “What are the key challenges the mobile team is currently facing?”
3. Technical Phone Screen
If you pass the initial screening, you’ll likely face a technical phone screen with a member of the engineering team. This step aims to assess your technical knowledge and problem-solving skills.
3.1. What to Expect
- Questions about your technical background and experience
- Mobile development concept questions
- Simple coding problems or algorithm questions
- Discussion about your approach to problem-solving
3.2. How to Prepare
- Review fundamental mobile development concepts
- Practice explaining your thought process while solving problems
- Be ready to code in a shared online editor
- Prepare examples of how you’ve tackled mobile-specific challenges in the past
3.3. Sample Technical Questions
Be prepared for questions like:
- “How would you implement offline caching in a mobile app?”
- “Explain the difference between retain cycles and memory leaks in iOS.”
- “What are the key considerations when designing a responsive layout for Android?”
- “How would you optimize battery usage in a location-based mobile app?”
4. Coding Challenge
Many companies include a take-home coding challenge as part of their interview process. This allows you to showcase your skills in a less pressured environment.
4.1. Common Types of Challenges
- Building a small mobile app from scratch
- Adding a new feature to an existing codebase
- Refactoring and optimizing poorly written code
- Implementing a specific algorithm or data structure
4.2. Tips for Success
- Read the instructions carefully and follow them precisely
- Focus on code quality, not just functionality
- Include comments to explain your thought process
- Implement error handling and edge cases
- Write unit tests if time allows
- Submit your solution before the deadline
4.3. Going Above and Beyond
To stand out, consider:
- Implementing extra features if time permits
- Providing a README with setup instructions and explanations of your choices
- Using design patterns and architecture that demonstrate your expertise
- Optimizing for performance and user experience
5. On-Site Interview (or Virtual Equivalent)
The on-site interview is often the final and most comprehensive stage of the process. It typically involves multiple rounds with different team members and focuses on various aspects of your skills and fit for the role.
5.1. Technical Interviews
Expect multiple technical interviews that may include:
- Whiteboard coding sessions
- System design questions
- In-depth discussions about your previous projects
- Mobile-specific coding challenges
5.2. Behavioral Interviews
These interviews assess your soft skills and cultural fit. Be prepared to discuss:
- Your experience working in teams
- How you handle conflicts or disagreements
- Your approach to learning new technologies
- Examples of how you’ve dealt with tight deadlines or changing requirements
5.3. Tips for On-Site Success
- Practice whiteboard coding beforehand
- Communicate clearly and think out loud during problem-solving
- Ask clarifying questions when needed
- Be prepared to discuss your solution’s time and space complexity
- Show enthusiasm and a positive attitude throughout the day
5.4. Sample On-Site Interview Questions
Here are some examples of questions you might encounter:
Technical:
- “Design a photo-sharing app with a feed and user profiles.”
- “Implement a custom view that displays a circular progress bar.”
- “How would you architect a chat application to handle real-time messages?”
Behavioral:
- “Tell me about a time when you had to meet a tight deadline. How did you manage it?”
- “Describe a situation where you disagreed with a team member. How did you resolve it?”
- “How do you stay updated with the latest mobile development trends and technologies?”
6. Coding Best Practices During Interviews
Throughout the interview process, especially during coding challenges and technical interviews, it’s crucial to demonstrate good coding practices. Here are some key points to keep in mind:
6.1. Write Clean and Readable Code
- Use meaningful variable and function names
- Follow consistent indentation and formatting
- Break down complex logic into smaller, manageable functions
6.2. Consider Edge Cases
- Think about and handle potential error scenarios
- Consider input validation and boundary conditions
- Discuss how your solution might scale with larger inputs
6.3. Optimize for Performance
- Be mindful of time and space complexity
- Discuss potential optimizations, even if you don’t implement them all
- Consider mobile-specific optimizations (e.g., battery usage, network efficiency)
6.4. Example: Implementing a Custom List View
Here’s an example of how you might approach implementing a custom list view in Android during an interview:
<?xml version="1.0" encoding="utf-8"?>
<androidx.recyclerview.widget.RecyclerView
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
In your Activity or Fragment:
class CustomListActivity : AppCompatActivity() {
private lateinit var recyclerView: RecyclerView
private lateinit var adapter: CustomAdapter
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_custom_list)
recyclerView = findViewById(R.id.recyclerView)
recyclerView.layoutManager = LinearLayoutManager(this)
// Assume we have a list of items to display
val items = fetchItems()
adapter = CustomAdapter(items)
recyclerView.adapter = adapter
}
private fun fetchItems(): List<Item> {
// In a real app, this might involve network calls or database queries
return listOf(Item("Item 1"), Item("Item 2"), Item("Item 3"))
}
}
class CustomAdapter(private val items: List<Item>) :
RecyclerView.Adapter<CustomAdapter.ViewHolder>() {
class ViewHolder(view: View) : RecyclerView.ViewHolder(view) {
val textView: TextView = view.findViewById(R.id.itemText)
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context)
.inflate(R.layout.list_item, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.textView.text = items[position].name
}
override fun getItemCount() = items.size
}
data class Item(val name: String)
When presenting this solution, you might discuss:
- Why you chose RecyclerView over ListView (better performance, view recycling)
- How you’d handle click events on list items
- Strategies for efficient loading of large datasets (pagination, infinite scrolling)
- How you’d optimize the layout for smooth scrolling
7. Post-Interview Follow-Up
After the interview process is complete, your actions can still make a difference. Here’s how to handle the post-interview phase:
7.1. Send a Thank You Note
- Email the interviewer or recruiter within 24 hours
- Express your appreciation for their time
- Reiterate your interest in the position
- Briefly mention a key point from the interview to make your note memorable
7.2. Reflect on the Experience
- Review the questions you were asked and how you answered
- Identify areas where you could improve for future interviews
- Note any topics or technologies you need to study further
7.3. Follow Up Appropriately
- If you haven’t heard back within the timeframe they specified, it’s okay to send a polite follow-up email
- Keep the email brief and professional
- Reaffirm your interest in the position and ask about the next steps
7.4. Handle the Outcome Gracefully
Whether you receive an offer or not, maintain a professional demeanor:
- If you receive an offer, take time to carefully consider it before responding
- If you don’t get the position, ask for feedback to help you improve for future opportunities
- Thank the company for their time and consideration, regardless of the outcome
8. Continuous Improvement
The mobile development field is constantly evolving. To stay competitive and perform well in future interviews, consider these strategies for ongoing improvement:
8.1. Stay Updated with Mobile Technologies
- Follow mobile development blogs and news sites
- Attend mobile development conferences and meetups
- Experiment with new frameworks and tools
8.2. Build Personal Projects
- Develop and publish your own mobile apps
- Contribute to open-source mobile projects
- Create a portfolio showcasing your best work
8.3. Practice Coding Regularly
- Solve algorithmic problems on platforms like LeetCode or HackerRank
- Participate in coding competitions
- Implement common mobile design patterns and architectures
8.4. Enhance Your Soft Skills
- Practice explaining technical concepts to non-technical people
- Work on team projects to improve collaboration skills
- Develop your communication skills through public speaking or writing technical blog posts
Conclusion
Mastering the mobile developer interview process is a combination of technical expertise, soft skills, and thorough preparation. By understanding the anatomy of the interview and what’s expected at each stage, you can approach the process with confidence and increase your chances of landing your dream job in mobile development.
Remember that each interview is also a learning experience. Even if you don’t get the job, the insights you gain from the process will help you improve and prepare for future opportunities. Stay persistent, keep learning, and approach each interview as a chance to showcase your skills and passion for mobile development.
As you continue your journey in mobile development, platforms like AlgoCademy can be invaluable resources for honing your coding skills, practicing algorithmic thinking, and preparing for technical interviews. By combining the strategies outlined in this guide with consistent practice and a commitment to ongoing learning, you’ll be well-equipped to tackle any mobile developer interview that comes your way.