The Anatomy of a System Design Interview: A Comprehensive Guide
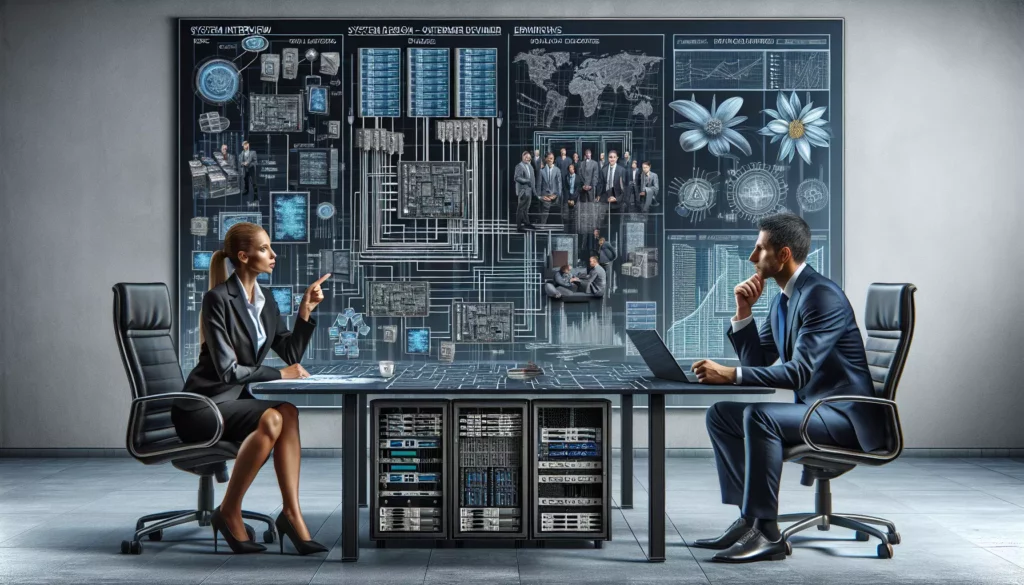
System design interviews are a crucial part of the hiring process for senior software engineers, backend engineers, and architects, especially at major tech companies. These interviews test a candidate’s ability to design large-scale distributed systems, focusing on aspects such as scalability, system architecture, database design, load balancing, API design, microservices, and security. In this comprehensive guide, we’ll break down the anatomy of a system design interview and provide you with strategies for each step of the process.
1. Understanding the Problem
The first and most critical step in any system design interview is to thoroughly understand the problem at hand. This phase typically involves the following:
1.1. Clarifying Requirements
Begin by asking questions to clarify the scope and requirements of the system you’re being asked to design. Some key questions to consider:
- What are the core features of the system?
- Who are the primary users of the system?
- What is the expected scale of the system (e.g., number of users, data volume)?
- Are there any specific performance requirements or constraints?
- What are the most important metrics for the system (e.g., latency, throughput, consistency)?
1.2. Defining Use Cases
Outline the main use cases for the system. This helps in understanding the system’s functionality and guides your design decisions. For example, if designing a Twitter-like system, some use cases might include:
- Posting a tweet
- Following/unfollowing users
- Viewing a user’s timeline
- Searching for tweets
1.3. Establishing Constraints and Assumptions
Identify any constraints or assumptions that will impact your design. This might include:
- Technical constraints (e.g., specific technology stack requirements)
- Business constraints (e.g., budget limitations)
- Scalability assumptions (e.g., expected growth rate)
- Geographic distribution of users
2. High-Level Design
Once you have a clear understanding of the problem, move on to creating a high-level design of the system. This phase involves:
2.1. System Architecture Overview
Sketch out the main components of your system. This might include:
- Client-side components (e.g., web, mobile apps)
- API Gateway
- Application servers
- Databases
- Caching layers
- Message queues
- Load balancers
Here’s a simple example of a high-level architecture for a social media platform:
<!-- ASCII art representation of a high-level system architecture -->
+--------+ +-------------+ +------------------+
| Client |-->--| API Gateway |-->--| Application |
+--------+ +-------------+ | Servers |
| +------------------+
| |
| +------------------+
| | Database Cluster |
| +------------------+
| |
+---------------+ +--------------+
| Cache Cluster | | Message Queue|
+---------------+ +--------------+
2.2. Data Model
Outline the main entities in your system and how they relate to each other. This helps in determining the database schema and API design. For a Twitter-like system, you might have entities such as:
- User
- Tweet
- Follow relationship
- Hashtag
2.3. API Design
Sketch out the main API endpoints for your system. This should cover the core functionality identified in your use cases. For example:
POST /api/tweets
GET /api/users/{userId}/timeline
POST /api/users/{userId}/follow
GET /api/search?q={query}
3. Deep Dive into Components
After establishing the high-level design, dive deeper into specific components of the system. This is where you demonstrate your technical knowledge and problem-solving skills.
3.1. Database Design
Choose appropriate database types (relational, NoSQL, or a combination) based on your data model and access patterns. Consider factors such as:
- Read vs. write heavy workloads
- Consistency requirements
- Scalability needs
For example, in a Twitter-like system, you might use:
- A relational database (e.g., PostgreSQL) for user data and relationships
- A NoSQL database (e.g., Cassandra) for storing tweets due to its ability to handle high write throughput
- A search engine (e.g., Elasticsearch) for efficient tweet searching
3.2. Caching Strategy
Discuss how you’ll implement caching to improve performance and reduce database load. Consider:
- What data to cache (e.g., user timelines, trending tweets)
- Cache invalidation strategies
- Distributed caching solutions (e.g., Redis, Memcached)
3.3. Load Balancing
Explain how you’ll distribute traffic across your application servers. Discuss:
- Load balancing algorithms (e.g., round-robin, least connections)
- Session persistence considerations
- Health checks and failover mechanisms
3.4. Data Partitioning
Describe how you’ll partition data to handle large volumes and improve performance. This might include:
- Horizontal partitioning (sharding) strategies
- Vertical partitioning (splitting features into separate services)
4. Scaling and Optimization
In this phase, discuss how your system will handle growth and optimize performance at scale.
4.1. Handling Scale
Explain how your system will handle increasing load. Consider:
- Horizontal scaling of application servers
- Database read replicas and write scalability
- Content Delivery Networks (CDNs) for static content
4.2. Performance Optimization
Discuss techniques to optimize system performance, such as:
- Asynchronous processing for non-critical operations
- Batch processing for computationally intensive tasks
- Database query optimization and indexing strategies
4.3. Monitoring and Alerting
Outline how you’ll monitor system health and performance. This might include:
- Key metrics to track (e.g., request latency, error rates, resource utilization)
- Logging and tracing strategies
- Alerting mechanisms for critical issues
5. Security and Data Privacy
Address security concerns and data privacy considerations in your design.
5.1. Authentication and Authorization
Discuss how you’ll implement user authentication and authorization. Consider:
- OAuth 2.0 for third-party integrations
- JSON Web Tokens (JWT) for stateless authentication
- Role-based access control (RBAC) for fine-grained permissions
5.2. Data Encryption
Explain your approach to data encryption, including:
- Encryption at rest (e.g., database encryption)
- Encryption in transit (e.g., TLS/SSL)
- End-to-end encryption for sensitive communications
5.3. Rate Limiting and DDoS Protection
Describe mechanisms to protect against abuse and attacks:
- API rate limiting strategies
- DDoS mitigation techniques (e.g., using a CDN or specialized services)
6. Trade-offs and Future Improvements
In the final phase of your system design interview, discuss the trade-offs in your design and potential future improvements.
6.1. Acknowledging Trade-offs
Every system design involves trade-offs. Be prepared to discuss the pros and cons of your design decisions. For example:
- Consistency vs. availability in distributed systems
- Performance vs. cost considerations
- Simplicity vs. flexibility in architecture choices
6.2. Future Improvements
Demonstrate forward-thinking by discussing potential future improvements or extensions to your system. This might include:
- Implementing a recommendation system
- Adding real-time features (e.g., live video streaming)
- Expanding to new platforms or devices
7. Common Pitfalls to Avoid
As you navigate through your system design interview, be aware of these common pitfalls:
7.1. Diving into Details Too Quickly
Avoid jumping into low-level details before establishing a solid high-level design. This can lead to missing important architectural considerations.
7.2. Neglecting to Clarify Requirements
Don’t make assumptions about the problem. Always clarify requirements and constraints with your interviewer to ensure you’re solving the right problem.
7.3. Ignoring Scale
Remember that system design interviews often focus on large-scale systems. Don’t propose solutions that would only work for small-scale applications.
7.4. Overlooking Data Consistency
In distributed systems, data consistency is a crucial consideration. Be prepared to discuss how you’ll maintain consistency across distributed components.
7.5. Failing to Justify Design Decisions
Always be ready to explain the reasoning behind your design choices. Your interviewer is interested in your thought process as much as the final design.
8. Preparation Strategies
To excel in system design interviews, consider the following preparation strategies:
8.1. Study Existing Systems
Analyze the architecture of popular large-scale systems. Resources like company engineering blogs and architecture case studies can be invaluable.
8.2. Practice with Mock Interviews
Conduct mock interviews with peers or mentors. This helps you get comfortable with the interview format and improves your ability to communicate complex ideas clearly.
8.3. Review Fundamental Concepts
Ensure you have a solid understanding of fundamental concepts in distributed systems, such as:
- CAP theorem
- Consistent hashing
- Load balancing algorithms
- Database indexing and query optimization
- Caching strategies
8.4. Stay Updated with Industry Trends
Keep abreast of current trends and technologies in system design, such as:
- Microservices architecture
- Serverless computing
- Container orchestration (e.g., Kubernetes)
- Event-driven architecture
9. Conclusion
Mastering system design interviews requires a combination of technical knowledge, problem-solving skills, and effective communication. By understanding the anatomy of these interviews and following a structured approach, you can significantly improve your performance.
Remember that the goal of a system design interview is not just to arrive at a perfect solution, but to demonstrate your ability to think through complex problems, make informed design decisions, and communicate your ideas clearly. Practice regularly, stay curious about large-scale systems, and approach each interview as an opportunity to learn and grow as an engineer.
With dedication and the right preparation, you can confidently tackle even the most challenging system design interviews and showcase your skills as a senior software engineer, backend engineer, or architect.