Get Started with Coding Challenges for Beginners
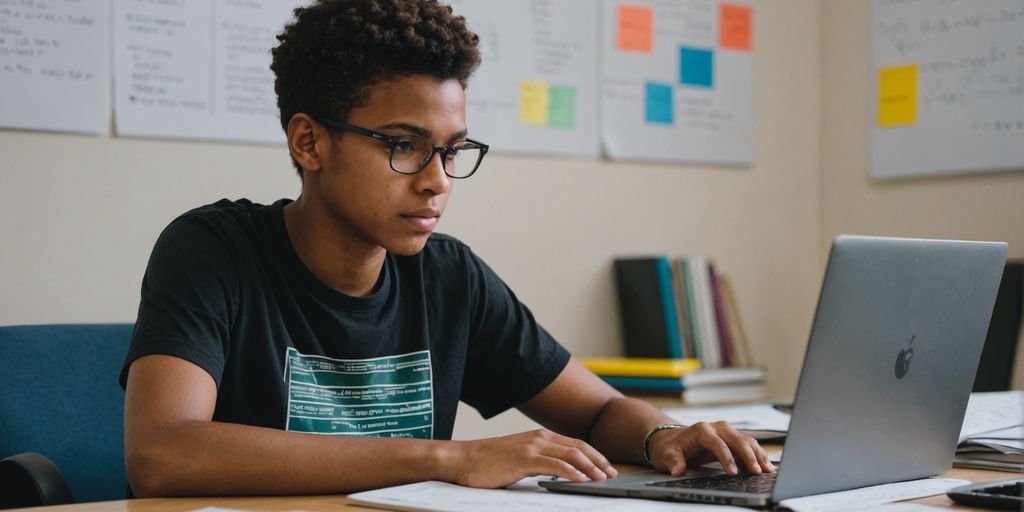
Jumping into the world of coding challenges can be both exciting and a bit overwhelming for beginners. Coding challenges are a great way to practice what you’ve learned, improve your problem-solving skills, and prepare for real-world programming tasks. This guide will help you get started with coding challenges, provide beginner-friendly examples, and offer tips for success.
Key Takeaways
- Start with the basics of programming before attempting coding challenges.
- Choose a programming language that you are comfortable with and stick to it.
- Use online resources and communities to find help and improve your skills.
- Begin with simple problems and gradually move to more complex challenges.
- Practicing coding challenges regularly can enhance your problem-solving skills and prepare you for job interviews.
Getting Started with Coding Challenges for Beginners
Before diving into coding challenges, it’s important to grasp the basics of programming. Here’s a step-by-step guide to help you get started.
Learn Programming Basics
Start with the fundamentals such as:
- What variables are and the different types (like text, numbers, true or false values)
- How to use basic math and compare things
- How to make decisions in your code with if statements
- How to repeat actions with loops
- How to group code into functions
- Understanding simple lists and collections
Pick a Language
Choose one programming language to begin with. Some beginner-friendly options include:
- Python – really friendly for beginners and easy to read
- JavaScript – great for making websites interactive
- Java – used in a lot of back-end development
- C++ – a bit more complex but great for understanding how computers work
Understand the Problem
When you see a coding problem:
- Read it well to know what it’s asking
- Think about special cases and limits
- Plan what inputs go in and what should come out
- Figure out the steps before coding
Use Online Resources
If you’re stuck, look up help like:
- The official guides for the programming language
- Stack Overflow for specific questions
- Online tutorials for more practice
Start Simple
Try easy problems first, then slowly move to harder ones:
- Work with variables, join texts
- Do basic math, comparisons
- Go through lists
- Make functions that give back results
Understanding the basics well will make tackling harder problems easier.
Beginner-Friendly Coding Challenges
Hello World Variations
The first program most people learn in a new programming language is the "Hello World" program. It’s a simple code that shows the message "Hello World" on your screen. Here are some easy and fun ways to change up the Hello World program:
- Try it in a different language
- Add some colors or styles to the text
- Make it interactive by asking for the user’s name and then greeting them
Basic Math Problems
Math problems are a great way to start coding. They help you understand how to use variables and basic operations. Here are some simple math challenges:
- Write a program to add, subtract, multiply, and divide two numbers
- Create a function to find the average of a list of numbers
- Make a program that calculates the area of a circle given its radius
String Manipulation Tasks
Working with text is another fundamental skill in programming. Here are some beginner-friendly string manipulation tasks:
- Reverse a string
- Count the number of vowels in a string
- Check if a string is a palindrome (reads the same backward as forward)
Simple Algorithms
Algorithms are step-by-step instructions to solve a problem. Start with these simple ones:
- Write a function to find the largest number in a list
- Create a program to sort a list of numbers in ascending order
- Implement the FizzBuzz challenge: print numbers from 1 to 100, but for multiples of three, print "Fizz" instead of the number, and for multiples of five, print "Buzz". For numbers that are multiples of both three and five, print "FizzBuzz".
Bold: Coding is best learned through hands-on practice. For educators, there’s nothing more rewarding than watching students solve problems and see their results on the screen.
Why Practice Coding Challenges
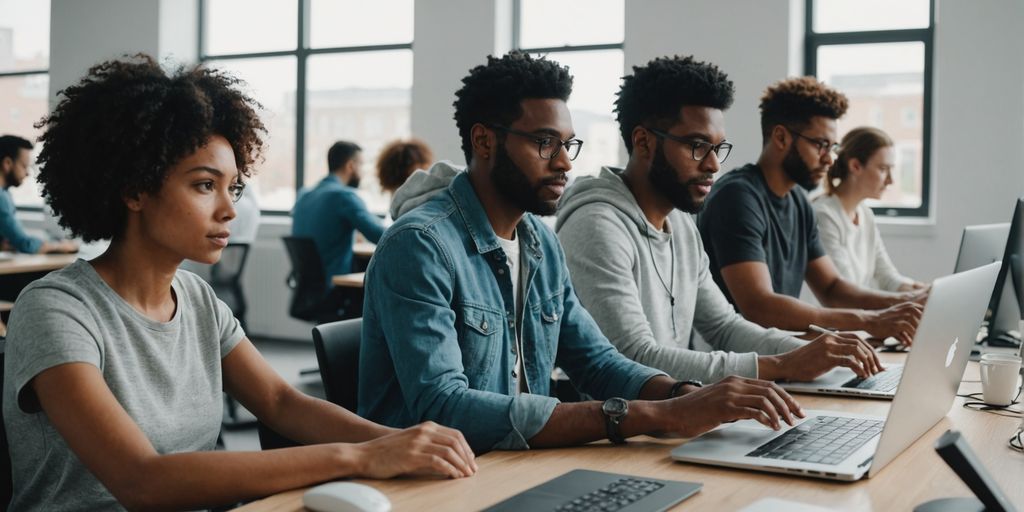
Develop Problem-Solving Skills
Coding challenges are designed to help beginners build a strong foundation in coding by tackling diverse problems that hone different skill sets. By practicing coding challenges, you can improve your problem-solving skills. This is because you learn to break down complex problems into smaller, manageable parts.
Understand Programming Languages Better
When you work on coding challenges, you get to know the programming language you are using more deeply. This hands-on experience helps you understand the syntax and functions better. You also get to see how theoretical concepts apply in real-world scenarios.
Prepare for Job Interviews
Coding challenges are a common part of technical job interviews. Hiring managers use them to assess your coding skills. Practicing these challenges can help you get ready for job interviews by giving you a taste of what to expect. This way, you can show that you not only know the theory but can also write code effectively.
Coding challenges are convenient because they allow you to exercise your skills on a bite-sized problem and rarely require you to build a complete application, so you can usually complete them rather quickly.
Best Websites for Coding Challenges
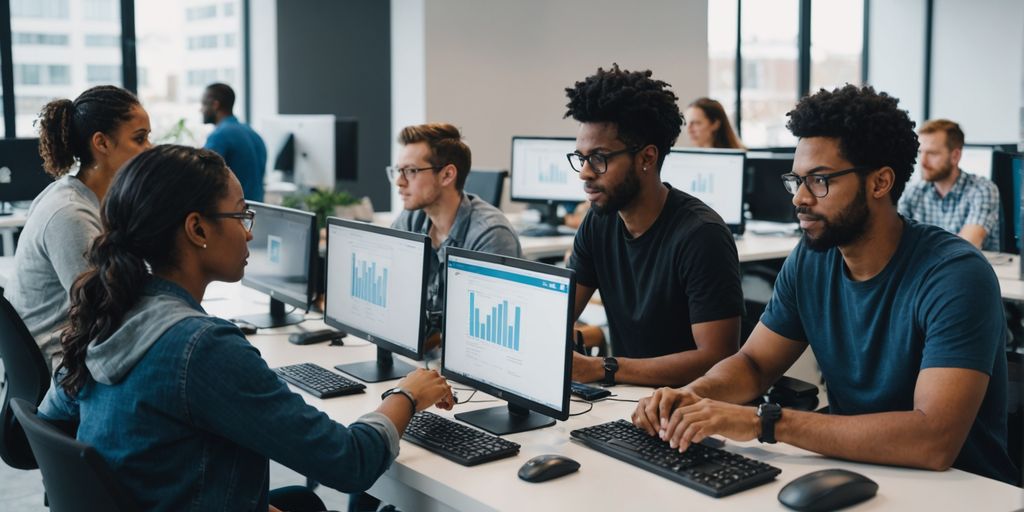
HackerRank
HackerRank offers a variety of challenges for different skill levels. Some challenges are even sponsored by companies, making it a great place to practice and get noticed by potential employers.
LeetCode
LeetCode is excellent for preparing for technical job interviews. It has a strong community where you can discuss solutions and learn from others.
Codewars
Codewars makes learning fun with a game-like environment. It’s especially good for beginners who want to learn different programming languages.
HackerEarth
HackerEarth hosts competitions and challenges that are often used for hiring. It’s a good platform to test your skills and compete with others.
Codility
Codility is used by companies to test candidates with coding tasks. It’s a great way to practice for job interviews and improve your problem-solving skills.
Tips for Success with Coding Challenges
Read the Problem Carefully
Before you start coding, make sure you understand the problem. Read it multiple times if needed. Look for special cases and limits. This will help you avoid mistakes later.
Plan Before You Code
Take a moment to plan your approach. Write down the steps you need to take. This will make your coding process smoother and more efficient.
Test Your Solutions
Always test your code with different inputs. This helps you catch errors and edge cases. Testing ensures your solution works in all scenarios.
Learn from Others
Don’t hesitate to look at other people’s solutions. You can learn new techniques and improve your skills. Just make sure to understand why their code works.
Building a strong coding habit is key to achieving your goal as a software developer. Keep practicing and learning from your mistakes.
Common Mistakes to Avoid
Skipping the Basics
Many beginners make the mistake of skipping the basics. It’s crucial to understand fundamental concepts like variables, loops, and conditionals before moving on to more complex topics. Without a solid foundation, you’ll find it difficult to solve even simple problems.
Not Reading Instructions Thoroughly
Another common error is not reading the instructions thoroughly. Always take the time to read the problem statement carefully. Missing out on key details can lead to incorrect solutions and wasted effort.
Ignoring Edge Cases
Beginners often overlook edge cases, which are unusual or extreme conditions that the code should handle. Ignoring these can result in bugs and unexpected behavior. Always consider edge cases when planning your solution.
Giving Up Too Soon
It’s easy to get frustrated and give up when you encounter difficulties. However, persistence is key to becoming a good programmer. Don’t give up too soon; instead, take a break and revisit the problem with a fresh perspective.
Remember, skipping the planning phase can lead to poorly structured and inefficient code. Always plan before you start coding.
Resources to Help You Improve
Online Tutorials
Online tutorials are a great way to learn at your own pace. Websites like Codecademy and freeCodeCamp offer interactive lessons that cover a wide range of topics. Consistent practice is key to becoming skilled in programming.
Coding Communities
Joining coding communities can provide support and motivation. Platforms like Stack Overflow and Reddit have active forums where you can ask questions and share knowledge. Being part of a community helps you stay updated with the latest trends and best practices.
Books and Courses
Books and courses offer in-depth knowledge on specific topics. Websites like Coursera and Udemy provide courses from beginner to advanced levels. Books can be a valuable resource for understanding complex concepts in detail.
Practice Platforms
Practice platforms like HackerRank and LeetCode offer a variety of coding challenges to help you improve your skills. These platforms provide a unique and engaging way to improve coding skills by solving puzzles and challenges through interactive games. The more you practice, the better you get at problem-solving and coding.
Conclusion
Starting with coding challenges can be a fun and rewarding way to dive into programming. By tackling simple problems first and gradually moving to more complex ones, you’ll build a strong foundation in coding. Remember, the key is to practice regularly and not be afraid to make mistakes. Each challenge you solve will boost your confidence and improve your problem-solving skills. So, keep coding, stay curious, and enjoy the journey of learning to program!
Frequently Asked Questions
What are coding challenges?
Coding challenges are small tasks or problems that you solve using programming. They help you practice and improve your coding skills.
Which programming language should I start with?
For beginners, Python, JavaScript, Java, and C++ are good choices. Python is especially beginner-friendly.
Where can I find coding challenges?
Websites like HackerRank, LeetCode, Codewars, HackerEarth, and Codility offer a wide range of coding challenges for different skill levels.
Why should I practice coding challenges?
Practicing coding challenges helps you develop problem-solving skills, understand programming languages better, and prepare for job interviews.
What should I do if I get stuck on a coding challenge?
If you get stuck, use online resources like tutorials, forums, and official guides. Sometimes, taking a break and coming back later can also help.
How can I avoid common mistakes in coding challenges?
To avoid common mistakes, make sure to read the problem carefully, plan your solution before coding, test your code thoroughly, and consider edge cases.