How to Build a Personal Website as a Self-Taught Developer
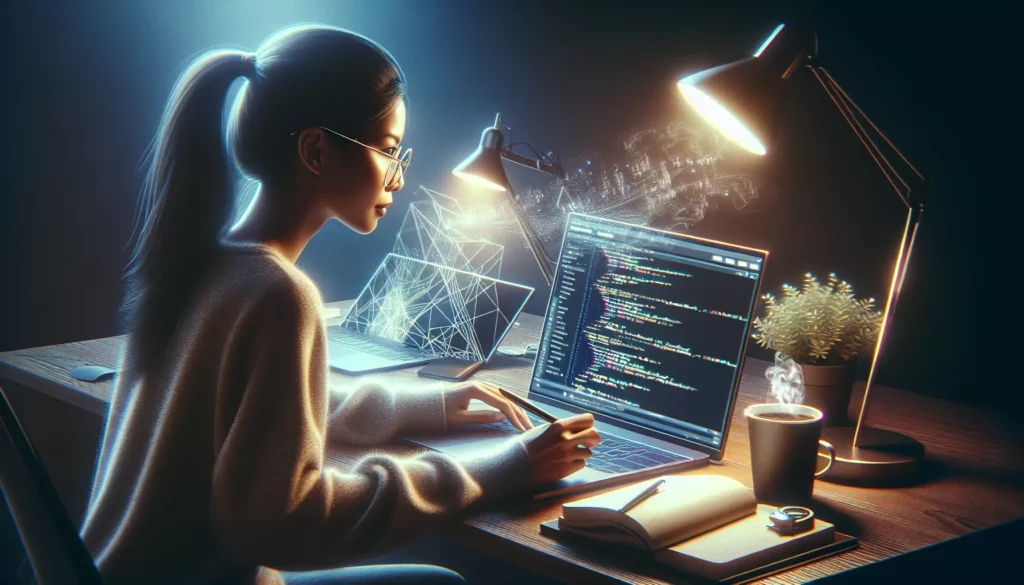
As a self-taught developer, one of the most valuable projects you can undertake is building your own personal website. Not only does it serve as a showcase for your skills and projects, but it also provides hands-on experience in web development. In this comprehensive guide, we’ll walk you through the process of creating your personal website from scratch, covering everything from planning and design to deployment and maintenance.
Table of Contents
- Why Build a Personal Website?
- Planning Your Website
- Designing Your Website
- Choosing Your Technology Stack
- Development Process
- Creating Content
- Optimization and Performance
- Deployment
- Maintenance and Updates
- Advanced Features and Improvements
- Conclusion
1. Why Build a Personal Website?
Before diving into the technical aspects, let’s consider why building a personal website is crucial for self-taught developers:
- Portfolio Showcase: Your website serves as a living portfolio, displaying your projects, skills, and achievements.
- Professional Presence: It establishes your online presence and personal brand in the tech industry.
- Learning Opportunity: The process of building and maintaining a website reinforces your coding skills and introduces you to new technologies.
- Networking Tool: It provides a platform for potential employers or clients to learn about you and your work.
- Blog Platform: You can share your knowledge, experiences, and thoughts with the developer community.
2. Planning Your Website
Effective planning is crucial for a successful website. Here’s how to approach it:
Define Your Goals
Determine the primary purpose of your website. Is it to showcase your portfolio, blog about your learning journey, or attract potential clients?
Identify Your Target Audience
Consider who will be visiting your site. Are you targeting potential employers, fellow developers, or clients?
Outline Your Content
Decide what content you want to include. Common sections for a developer’s personal site include:
- Home/About Me
- Portfolio/Projects
- Skills/Technologies
- Blog (if applicable)
- Contact Information
- Resume/CV
Sketch Your Site Structure
Create a simple sitemap or wireframe to visualize how your content will be organized and how pages will link together.
3. Designing Your Website
Design plays a crucial role in how your website is perceived. Here are some tips for effective design:
Keep It Simple and Clean
As a developer, focus on a clean, minimalist design that highlights your work and skills without unnecessary clutter.
Ensure Responsiveness
Your site should look good and function well on all devices, from smartphones to desktop computers.
Choose a Consistent Color Scheme
Select a color palette that reflects your personal brand. Stick to 2-3 main colors for consistency.
Typography Matters
Choose readable fonts. A common practice is to use a sans-serif font for headings and a serif font for body text.
Use Whitespace Effectively
Don’t be afraid of empty space. It can help make your content more readable and your design more appealing.
4. Choosing Your Technology Stack
As a self-taught developer, you have numerous options for building your website. Here are some popular choices:
Frontend Technologies
- HTML5, CSS3, and JavaScript: The foundation of web development.
- Frontend Frameworks: Consider using React, Vue.js, or Angular for more complex sites.
- CSS Frameworks: Bootstrap or Tailwind CSS can speed up your design process.
Backend Technologies (if needed)
- Node.js with Express.js: A popular choice for JavaScript developers.
- Python with Django or Flask: Great for those comfortable with Python.
- Ruby on Rails: Known for its convention over configuration approach.
Static Site Generators
For a simple personal website, a static site generator might be sufficient:
- Jekyll: Ruby-based, integrates well with GitHub Pages.
- Hugo: Go-based, known for its speed.
- Gatsby: React-based, great for performance and SEO.
Content Management Systems (CMS)
If you plan to update your content frequently:
- WordPress: Widely used and beginner-friendly.
- Ghost: Focused on blogging and publishing.
- Strapi: A headless CMS that gives you more flexibility.
5. Development Process
Now that you’ve planned your site and chosen your tech stack, it’s time to start building. Here’s a step-by-step process:
Set Up Your Development Environment
Install necessary software such as a code editor (e.g., Visual Studio Code), version control system (Git), and any required frameworks or libraries.
Create Your Project Structure
Organize your files and folders in a logical manner. A basic structure might look like this:
project-root/
├── index.html
├── css/
│ └── styles.css
├── js/
│ └── main.js
├── images/
└── pages/
├── about.html
├── portfolio.html
└── contact.html
Develop Your HTML Structure
Start with your main index.html
file and create the basic structure of your site. Here’s a simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Your Name - Personal Website</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<header>
<nav>
<!-- Add your navigation menu items here -->
</nav>
</header>
<main>
<section id="hero">
<h1>Welcome to My Personal Website</h1>
<p>I'm a self-taught developer passionate about creating web solutions.</p>
</section>
<!-- Add more sections for your content -->
</main>
<footer>
<p>© 2023 Your Name. All rights reserved.</p>
</footer>
<script src="js/main.js"></script>
</body>
</html>
Style Your Website with CSS
Create your styles.css
file and start styling your HTML elements. Here’s a basic example:
/* Reset default styles */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
line-height: 1.6;
color: #333;
}
header {
background-color: #f4f4f4;
padding: 1rem;
}
nav ul {
list-style-type: none;
}
nav ul li {
display: inline;
margin-right: 10px;
}
main {
padding: 2rem;
}
#hero {
text-align: center;
padding: 2rem 0;
}
footer {
background-color: #333;
color: #fff;
text-align: center;
padding: 1rem;
position: absolute;
bottom: 0;
width: 100%;
}
Add Interactivity with JavaScript
Use JavaScript to add dynamic elements to your site. Here’s a simple example that changes the header color on scroll:
// main.js
window.addEventListener('scroll', function() {
const header = document.querySelector('header');
if (window.scrollY > 50) {
header.style.backgroundColor = '#333';
header.style.color = '#fff';
} else {
header.style.backgroundColor = '#f4f4f4';
header.style.color = '#333';
}
});
Implement Responsive Design
Ensure your website looks good on all devices by using media queries in your CSS:
@media screen and (max-width: 768px) {
/* Styles for tablets and smaller screens */
nav ul li {
display: block;
margin-bottom: 10px;
}
}
@media screen and (max-width: 480px) {
/* Styles for mobile phones */
#hero h1 {
font-size: 1.5rem;
}
}
6. Creating Content
With the structure in place, it’s time to focus on your content:
Write an Engaging “About Me” Section
Introduce yourself, your skills, and your passion for development. Keep it concise but informative.
Showcase Your Projects
Create a portfolio section highlighting your best work. For each project, include:
- Project name and description
- Technologies used
- Your role in the project
- Screenshots or live demo links
- GitHub repository link (if applicable)
List Your Skills and Technologies
Create a section that clearly outlines your technical skills. You can categorize them (e.g., Programming Languages, Frameworks, Tools) for better organization.
Include a Blog (Optional)
If you decide to include a blog, write a few initial posts about your learning journey, project experiences, or tech topics you’re passionate about.
Add a Contact Form or Information
Make it easy for visitors to get in touch with you. You can use a simple contact form or just list your professional email address.
7. Optimization and Performance
Optimizing your website is crucial for a good user experience and better search engine rankings:
Optimize Images
Compress your images and use appropriate formats (JPEG for photographs, PNG for graphics with transparency).
Minify CSS and JavaScript
Use tools like UglifyJS for JavaScript and cssnano for CSS to minify your code.
Implement Lazy Loading
Use lazy loading for images and videos to improve initial page load times.
Optimize for SEO
Use descriptive titles, meta descriptions, and heading tags. Ensure your content includes relevant keywords naturally.
Improve Page Speed
Use tools like Google PageSpeed Insights to identify and fix performance issues.
8. Deployment
Once your website is ready, it’s time to make it live:
Choose a Hosting Provider
Options include:
- GitHub Pages: Free and easy to use, especially for static sites.
- Netlify: Offers free hosting with easy deployment from Git repositories.
- Vercel: Great for React-based projects, offers serverless functions.
- Traditional hosts: Like HostGator or Bluehost for more complex setups.
Set Up a Domain Name
Purchase a domain name that reflects your personal brand. Many hosting providers offer domain registration services.
Deploy Your Website
Follow your chosen hosting provider’s instructions to deploy your site. This often involves pushing your code to a Git repository or uploading files via FTP.
Set Up HTTPS
Ensure your site uses HTTPS for security. Many modern hosting providers offer free SSL certificates.
9. Maintenance and Updates
Your website is not a “set it and forget it” project. Regular maintenance is important:
Regular Content Updates
Keep your projects, skills, and any blog content up to date.
Technical Maintenance
Regularly update your dependencies, frameworks, and plugins to ensure security and compatibility.
Monitor Performance
Use tools like Google Analytics to track visitor behavior and identify areas for improvement.
Backup Your Site
Regularly backup your website files and database (if applicable) to prevent data loss.
10. Advanced Features and Improvements
As you grow as a developer, consider adding more advanced features to your site:
Implement a Custom CMS
Build your own content management system to manage your blog or portfolio more efficiently.
Add Interactive Elements
Implement features like dark mode toggle, animated transitions, or interactive project showcases.
Integrate with External APIs
Display your latest GitHub contributions or integrate with other platforms you use.
Implement Server-Side Rendering
If using a framework like React, consider implementing server-side rendering for improved performance and SEO.
Create a Progressive Web App (PWA)
Transform your website into a PWA for better performance and offline capabilities.
Conclusion
Building a personal website as a self-taught developer is an invaluable experience. It allows you to apply your skills, learn new technologies, and create a powerful tool for your professional growth. Remember, your website is a reflection of you as a developer, so take pride in its creation and maintenance.
As you continue to learn and grow, your website will evolve with you. Don’t be afraid to experiment with new technologies or redesign as your skills improve. Your personal website is not just a showcase of your current abilities, but a platform for your future growth in the world of web development.
Happy coding, and may your personal website be the stepping stone to exciting opportunities in your development career!