Why Consistency is Key to Success as a Self-Taught Programmer
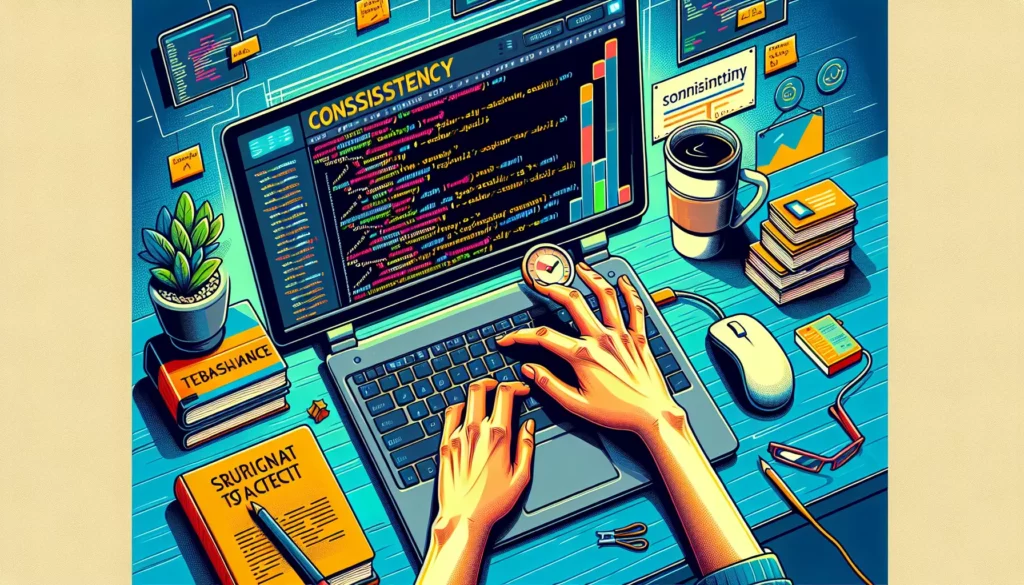
In the ever-evolving world of technology, becoming a self-taught programmer has become an increasingly popular path for those looking to break into the tech industry. With countless online resources, tutorials, and coding bootcamps available, it’s easier than ever to start learning programming on your own. However, the journey from novice to proficient developer is not without its challenges. One of the most crucial factors that can make or break your success as a self-taught programmer is consistency. In this article, we’ll explore why consistency is so important and how you can cultivate it to achieve your coding goals.
The Power of Consistent Practice
Consistency in programming is all about regular, dedicated practice. It’s not about cramming for hours on end or having sporadic bursts of intense study. Instead, it’s about making coding a part of your daily routine. Here’s why consistent practice is so powerful:
1. Building Muscle Memory
Programming, like any skill, requires muscle memory. The more you code, the more natural it becomes. Consistent practice helps you internalize syntax, common patterns, and problem-solving approaches. Over time, you’ll find yourself writing code more fluently and with fewer errors.
2. Reinforcing Concepts
Programming concepts can be complex and abstract. Regular practice helps reinforce these concepts, making them easier to understand and apply. Each time you revisit a concept or use it in a new context, you’re strengthening your understanding and building a more robust knowledge base.
3. Overcoming Plateaus
Learning to code isn’t always a smooth upward trajectory. You’ll encounter plateaus where progress seems to slow down. Consistent practice helps you push through these plateaus by ensuring you’re continually challenging yourself and expanding your skills.
The Compound Effect of Consistency
One of the most powerful aspects of consistency is the compound effect it creates over time. Small, consistent efforts can lead to significant results in the long run. This principle is often illustrated by the concept of the “1% rule” or “marginal gains.”
If you improve by just 1% each day, over the course of a year, you’ll be 37 times better than when you started. This concept applies perfectly to programming. Each day you practice, you’re making small improvements that compound over time:
// Pseudo-code to illustrate the compound effect
let skill_level = 1;
const days_in_year = 365;
const daily_improvement = 1.01; // 1% improvement each day
for (let day = 1; day <= days_in_year; day++) {
skill_level *= daily_improvement;
}
console.log(`After one year of consistent 1% daily improvement:`);
console.log(`Your skill level is now ${skill_level.toFixed(2)} times higher than when you started!`);
This simple illustration shows how powerful consistent, small improvements can be over time. In programming, these improvements might come in the form of learning a new method, understanding a complex algorithm, or optimizing your code writing process.
Consistency and the Learning Process
Consistency plays a crucial role in the learning process, especially when it comes to mastering a complex skill like programming. Here’s how consistency affects different aspects of learning:
1. Retention of Information
Consistent practice leverages the power of spaced repetition, a learning technique that involves reviewing information at gradually increasing intervals. This method has been proven to enhance long-term retention of information. In programming, this might mean revisiting concepts you’ve learned, applying them in different contexts, or explaining them to others.
2. Skill Development
Programming is not just about memorizing syntax; it’s about developing problem-solving skills, logical thinking, and the ability to break down complex problems into manageable parts. These skills are honed through consistent practice and exposure to various coding challenges.
3. Building a Coding Habit
Consistency helps in forming a coding habit. When you code regularly, it becomes a natural part of your routine, reducing the mental friction that can sometimes make it hard to start a coding session.
Overcoming Challenges to Consistency
While the benefits of consistency are clear, maintaining a consistent practice routine can be challenging, especially for self-taught programmers. Here are some common obstacles and strategies to overcome them:
1. Lack of Time
Challenge: Many aspiring programmers struggle to find time for coding amidst work, family, and other commitments.
Solution: Start small. Even 15-30 minutes of focused coding practice each day can make a significant difference. Use time management techniques like the Pomodoro Technique to make the most of short coding sessions.
2. Motivation Fluctuations
Challenge: It’s natural for motivation to ebb and flow, especially when facing difficult concepts or frustrating bugs.
Solution: Set small, achievable goals for each coding session. Celebrate your progress, no matter how small. Join coding communities or find a coding buddy for mutual support and accountability.
3. Information Overload
Challenge: The vast amount of resources and technologies available can be overwhelming, leading to decision paralysis.
Solution: Create a structured learning plan. Focus on one language or technology at a time. Use platforms like AlgoCademy that provide structured learning paths and interactive coding tutorials.
Strategies for Maintaining Consistency
Now that we’ve discussed the importance of consistency and some common challenges, let’s explore strategies to help you maintain a consistent coding practice:
1. Set Clear Goals
Having clear, achievable goals gives your practice purpose and direction. Set both short-term and long-term goals. For example:
- Short-term: Complete one coding challenge on AlgoCademy every day
- Long-term: Build a full-stack web application in 3 months
2. Create a Schedule
Allocate specific times for coding practice in your daily or weekly schedule. Treat these time slots as non-negotiable appointments with yourself. For example:
// Example weekly coding schedule
const weeklySchedule = {
Monday: "7:00 PM - 8:00 PM: AlgoCademy Data Structures Tutorial",
Tuesday: "6:30 AM - 7:30 AM: Coding Challenge",
Wednesday: "8:00 PM - 9:30 PM: Work on Personal Project",
Thursday: "6:30 AM - 7:30 AM: Coding Challenge",
Friday: "7:00 PM - 8:30 PM: Review and Refactor Code",
Saturday: "10:00 AM - 12:00 PM: Deep Dive into New Concept",
Sunday: "2:00 PM - 3:30 PM: Plan Next Week's Goals"
};
3. Track Your Progress
Keep a log of your coding activities. This can be as simple as marking an ‘X’ on a calendar for each day you code, or as detailed as maintaining a coding journal. Seeing your progress visually can be a great motivator.
4. Join a Community
Engaging with other learners can provide motivation, support, and opportunities for collaboration. Join coding forums, attend local meetups, or participate in online coding communities. Platforms like AlgoCademy often have built-in community features where you can connect with other learners.
5. Use the “Don’t Break the Chain” Method
This method, popularized by comedian Jerry Seinfeld, involves marking a calendar each day you complete your coding practice. The goal is to create a chain of marked days and not break it. This visual representation of your consistency can be highly motivating.
6. Embrace the Process, Not Just the Outcome
Focus on enjoying the learning process rather than fixating solely on end goals. Cultivate curiosity and a love for problem-solving. This mindset shift can make consistent practice feel less like a chore and more like an engaging activity.
The Role of Structured Learning in Consistency
While self-taught programming offers flexibility, incorporating structured learning can significantly enhance consistency. Platforms like AlgoCademy provide several advantages:
1. Guided Learning Paths
Structured courses and learning paths take the guesswork out of what to learn next. This can prevent decision fatigue and keep you moving forward consistently.
2. Interactive Coding Environments
Integrated development environments (IDEs) allow you to practice coding directly in your browser, reducing barriers to getting started each day.
3. Progressive Difficulty
Well-designed curricula gradually increase in difficulty, ensuring you’re consistently challenged without becoming overwhelmed.
4. Immediate Feedback
Features like automated code checking provide immediate feedback, helping you learn from mistakes quickly and maintain motivation.
5. Gamification Elements
Many platforms incorporate gamification elements like streaks, badges, or points systems, which can boost motivation and encourage consistent engagement.
The Long-Term Impact of Consistency
As you maintain consistency in your programming practice, you’ll start to see significant long-term benefits:
1. Accelerated Skill Development
Consistent practice leads to faster skill acquisition and mastery. You’ll find yourself tackling increasingly complex problems with greater ease.
2. Improved Problem-Solving Abilities
Regular exposure to coding challenges enhances your analytical and problem-solving skills, which are valuable not just in programming but in many aspects of life and work.
3. Increased Confidence
As you consistently overcome challenges and build your skills, your confidence in your abilities will grow. This confidence can be crucial when applying for jobs or taking on new projects.
4. Better Job Prospects
Employers value self-taught programmers who demonstrate consistency and dedication to their craft. Your commitment to regular practice can set you apart in the job market.
5. Adaptability to New Technologies
Consistent learning habits make it easier to adapt to new programming languages, frameworks, and technologies as they emerge.
Case Studies: Success Through Consistency
Let’s look at a couple of hypothetical case studies to illustrate the power of consistency in self-taught programming:
Case Study 1: Sarah’s Journey to Full-Stack Development
Sarah, a marketing professional, decided to transition to web development. She committed to coding for at least one hour every day after work. Here’s her journey:
- Months 1-3: Focused on HTML, CSS, and basic JavaScript using online tutorials and AlgoCademy’s front-end track.
- Months 4-6: Dove deeper into JavaScript and began learning React, building small projects along the way.
- Months 7-9: Started backend development with Node.js and Express, while continuing to enhance her front-end skills.
- Months 10-12: Built a full-stack project, combining all her learned skills.
- Result: After 12 months of consistent daily practice, Sarah successfully transitioned to a junior full-stack developer role.
Case Study 2: Mike’s Algorithm Mastery
Mike, a computer science student, aimed to excel in coding interviews. He established a consistent practice routine:
- Daily: Solved one algorithmic problem on AlgoCademy, gradually increasing difficulty.
- Weekly: Participated in one online coding contest.
- Monthly: Conducted a mock interview with a peer.
- Result: After 6 months, Mike successfully interviewed and secured an internship at a major tech company.
Measuring Your Consistency
To help you gauge and improve your consistency, consider tracking these metrics:
1. Coding Streak
Keep track of how many consecutive days you’ve coded. Tools like GitHub’s contribution graph can help visualize this.
2. Time Spent Coding
Log the amount of time you spend coding each day or week. Aim to maintain or gradually increase this over time.
3. Problems Solved
Track the number of coding challenges or exercises you complete. Platforms like AlgoCademy often have built-in tracking for this.
4. Concepts Mastered
Keep a list of programming concepts you’ve learned and feel confident about. Regularly review and update this list.
5. Projects Completed
Document the projects you’ve built, no matter how small. This provides a tangible record of your progress.
Here’s a simple JavaScript object to help you track these metrics:
const consistencyTracker = {
streak: 0,
totalCodingTime: 0,
problemsSolved: 0,
conceptsMastered: [],
projectsCompleted: [],
updateStreak(didCodeToday) {
this.streak = didCodeToday ? this.streak + 1 : 0;
},
addCodingTime(hours) {
this.totalCodingTime += hours;
},
solveProblem() {
this.problemsSolved++;
},
masterConcept(concept) {
this.conceptsMastered.push(concept);
},
completeProject(projectName) {
this.projectsCompleted.push(projectName);
},
getProgress() {
return `
Current Streak: ${this.streak} days
Total Coding Time: ${this.totalCodingTime} hours
Problems Solved: ${this.problemsSolved}
Concepts Mastered: ${this.conceptsMastered.join(', ')}
Projects Completed: ${this.projectsCompleted.join(', ')}
`;
}
};
Conclusion: Embracing the Journey of Consistent Growth
As we’ve explored throughout this article, consistency is indeed the key to success for self-taught programmers. It’s the foundation upon which all other aspects of learning and skill development are built. By maintaining a consistent practice routine, you’re not just accumulating knowledge; you’re developing a programmer’s mindset, building resilience, and cultivating the habits that will serve you throughout your career.
Remember, the journey of a self-taught programmer is not always smooth or linear. There will be days when motivation is low, when concepts seem impossibly complex, or when bugs in your code feel insurmountable. It’s during these times that your commitment to consistency becomes most valuable. Each time you push through these challenges and show up for your practice, you’re not just learning to code – you’re learning perseverance, problem-solving, and the art of continuous improvement.
As you move forward on your programming journey, keep these key takeaways in mind:
- Start small and build up gradually. Consistency is more important than intensity.
- Use tools and platforms like AlgoCademy to provide structure and support to your learning.
- Connect with other learners for motivation and accountability.
- Celebrate your progress, no matter how small.
- Focus on the process of learning, not just the end goals.
- Be patient with yourself. Skill development takes time.
Remember, every expert was once a beginner. The programmers you admire, the developers behind the apps and websites you use daily, all started their journey with the fundamentals. What sets them apart is their commitment to consistent practice and lifelong learning.
As you embark on or continue your journey as a self-taught programmer, let consistency be your guiding principle. Embrace the daily practice, the small victories, and even the frustrations as part of your growth. With time and persistence, you’ll not only develop the technical skills you seek but also the problem-solving abilities, creativity, and resilience that characterize successful programmers.
Your journey in programming is uniquely yours. By staying consistent, you’re not racing against others; you’re continuously evolving into a better version of yourself. So, open that code editor, tackle that next tutorial on AlgoCademy, solve that coding challenge, and take one more step forward in your programming journey. Your future self will thank you for the consistency you maintain today.
Happy coding, and here’s to your consistent growth and success in the exciting world of programming!