How to Build a Curriculum for Learning to Code on Your Own
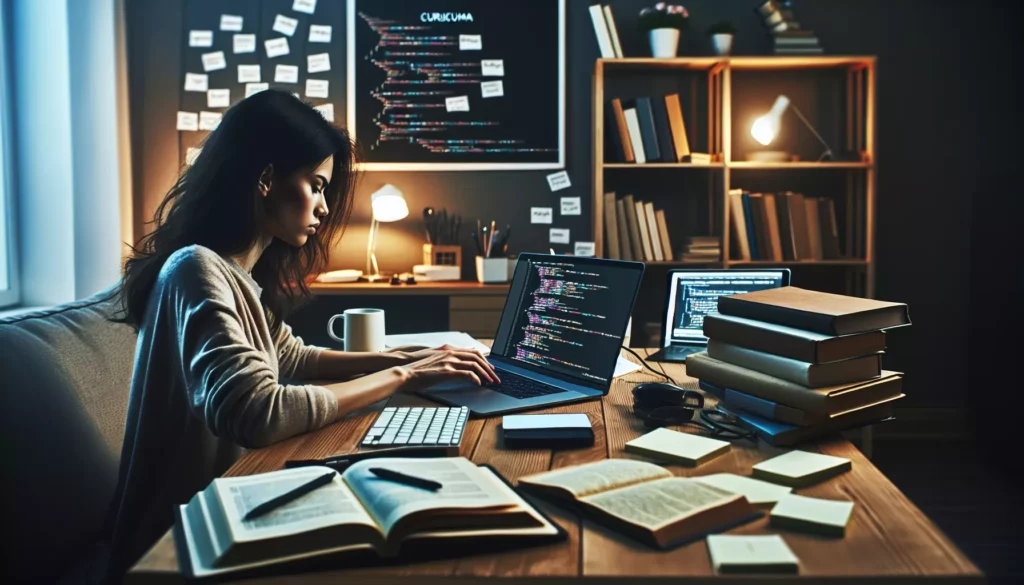
Learning to code on your own can be an exciting and rewarding journey. With the abundance of resources available online, it’s entirely possible to become a proficient programmer without formal education. However, the sheer volume of information can be overwhelming, making it crucial to have a structured approach. This comprehensive guide will walk you through the process of building a curriculum for learning to code on your own, ensuring you cover all the necessary bases and progress efficiently.
1. Determine Your Goals
Before diving into coding, it’s essential to establish clear goals. Ask yourself:
- What do you want to achieve by learning to code?
- Are you interested in web development, mobile app development, data science, or something else?
- Do you want to change careers or enhance your current skillset?
- Are you aiming to work for a specific company or in a particular industry?
Having clear objectives will help you tailor your learning path and stay motivated throughout the process.
2. Choose Your Programming Language
Based on your goals, select a programming language to start with. Here are some popular options:
- Python: Versatile, beginner-friendly, great for web development, data science, and machine learning.
- JavaScript: Essential for web development, both front-end and back-end (Node.js).
- Java: Widely used in enterprise environments and Android app development.
- C++: Powerful for system programming, game development, and high-performance applications.
- Swift: Used for iOS app development.
Remember, once you learn one language well, it becomes easier to pick up others. Focus on mastering one language before branching out.
3. Start with the Basics
Every programming journey begins with understanding the fundamentals. Your curriculum should include:
3.1. Programming Concepts
- Variables and data types
- Operators and expressions
- Control structures (if-else statements, loops)
- Functions and methods
- Arrays and lists
- Object-oriented programming (classes, objects, inheritance)
3.2. Problem-Solving Skills
Develop your logical thinking and problem-solving abilities through:
- Algorithmic thinking
- Pseudocode writing
- Flowchart creation
- Basic data structures (stacks, queues, trees)
3.3. Tools and Environment Setup
Learn to set up your development environment:
- Choosing and installing an Integrated Development Environment (IDE)
- Version control with Git and GitHub
- Command-line basics
4. Build Projects
Theory alone isn’t enough. Apply your knowledge by building projects. Start small and gradually increase complexity:
4.1. Beginner Projects
- Calculator app
- To-do list application
- Simple game (like Tic-Tac-Toe or Hangman)
- Basic web scraper
4.2. Intermediate Projects
- Weather app using API
- Blog platform with a database
- E-commerce website
- Mobile app (if focusing on mobile development)
4.3. Advanced Projects
- Social media clone
- Machine learning project
- Full-stack web application
- Contribution to open-source projects
5. Learn Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for becoming a proficient programmer. Include the following in your curriculum:
5.1. Data Structures
- Arrays and strings
- Linked lists
- Stacks and queues
- Trees and graphs
- Hash tables
- Heaps
5.2. Algorithms
- Sorting algorithms (Bubble Sort, Merge Sort, Quick Sort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic programming
- Greedy algorithms
- Graph algorithms
5.3. Big O Notation
Understand time and space complexity to analyze and optimize your code.
6. Dive into Specific Domains
Based on your goals, delve deeper into specific areas:
6.1. Web Development
- HTML, CSS, and JavaScript
- Front-end frameworks (React, Vue, Angular)
- Back-end development (Node.js, Django, Ruby on Rails)
- Database management (SQL, MongoDB)
- RESTful APIs
- Web security basics
6.2. Mobile Development
- iOS development with Swift
- Android development with Java or Kotlin
- Cross-platform development (React Native, Flutter)
- Mobile UI/UX principles
- App store deployment
6.3. Data Science and Machine Learning
- Python libraries (NumPy, Pandas, Matplotlib)
- Statistical analysis
- Machine learning algorithms
- Deep learning frameworks (TensorFlow, PyTorch)
- Data visualization
7. Practice Coding Challenges
Sharpen your skills with coding challenges and exercises. Platforms like LeetCode, HackerRank, and CodeWars offer a variety of problems to solve. Regular practice will help you:
- Improve problem-solving skills
- Learn to optimize your code
- Prepare for technical interviews
- Understand different approaches to solving problems
8. Learn Software Development Best Practices
As you progress, incorporate these essential practices into your learning:
8.1. Version Control
- Master Git for source code management
- Learn branching strategies
- Understand pull requests and code reviews
8.2. Testing
- Unit testing
- Integration testing
- Test-Driven Development (TDD)
8.3. Code Quality
- Clean code principles
- Code refactoring techniques
- Design patterns
8.4. Agile Methodologies
- Scrum basics
- Kanban principles
- Continuous Integration/Continuous Deployment (CI/CD)
9. Stay Updated and Engage with the Community
The tech world evolves rapidly. Keep yourself updated and connected:
- Follow tech blogs and news sites
- Attend webinars and virtual conferences
- Join coding communities (Stack Overflow, GitHub, Reddit)
- Participate in hackathons
- Contribute to open-source projects
10. Prepare for Job Search (If Applicable)
If your goal is to land a job in tech, include these steps in your curriculum:
- Build a strong GitHub portfolio
- Create a professional website or blog
- Practice technical interview questions
- Learn about system design for larger scale applications
- Understand common software architectures
11. Continuous Learning and Specialization
As you progress, continue to deepen your knowledge:
- Explore advanced topics in your chosen field
- Learn about emerging technologies (AI, blockchain, IoT)
- Consider obtaining relevant certifications
- Explore computer science concepts (if not covered in formal education)
12. Leveraging AI-Powered Learning Tools
In the era of artificial intelligence, leveraging AI-powered learning tools can significantly enhance your coding education. Platforms like AlgoCademy offer interactive coding tutorials with AI assistance, providing personalized learning experiences. These tools can help you:
- Get instant feedback on your code
- Receive tailored problem recommendations based on your skill level
- Understand complex concepts through AI-generated explanations
- Practice coding interviews with AI-simulated scenarios
Incorporating such tools into your curriculum can accelerate your learning process and provide a more engaging experience.
Sample Curriculum Timeline
Here’s a rough timeline to give you an idea of how to structure your learning:
Months 1-3: Foundations
- Learn programming basics in your chosen language
- Complete small projects to apply your knowledge
- Set up your development environment
- Start practicing coding challenges
Months 4-6: Intermediate Skills
- Dive deeper into your chosen language and framework
- Learn basic data structures and algorithms
- Build more complex projects
- Start contributing to open-source projects
Months 7-9: Advanced Topics
- Study advanced data structures and algorithms
- Learn about databases and APIs
- Explore software design patterns
- Build a full-stack application
Months 10-12: Specialization and Job Preparation
- Focus on your chosen specialization (web, mobile, data science, etc.)
- Prepare for technical interviews
- Build a portfolio of projects
- Network and engage with the tech community
Conclusion
Building a curriculum for learning to code on your own is a challenging but rewarding process. Remember that everyone’s learning journey is unique, and it’s okay to adjust your curriculum as you progress. The key is to stay consistent, practice regularly, and never stop learning.
By following this comprehensive guide, you’ll be well-equipped to start your coding journey and work towards becoming a proficient programmer. Whether you’re aiming for a career change, looking to enhance your current skills, or simply pursuing a passion for technology, a well-structured self-learning curriculum can help you achieve your goals.
Remember to leverage resources like AlgoCademy, which can provide structured learning paths, interactive tutorials, and AI-powered assistance to complement your self-study efforts. With dedication, persistence, and the right resources, you can successfully learn to code and open up a world of opportunities in the ever-evolving field of technology.