The Role of Critical Thinking in Coding Interviews: A Comprehensive Guide
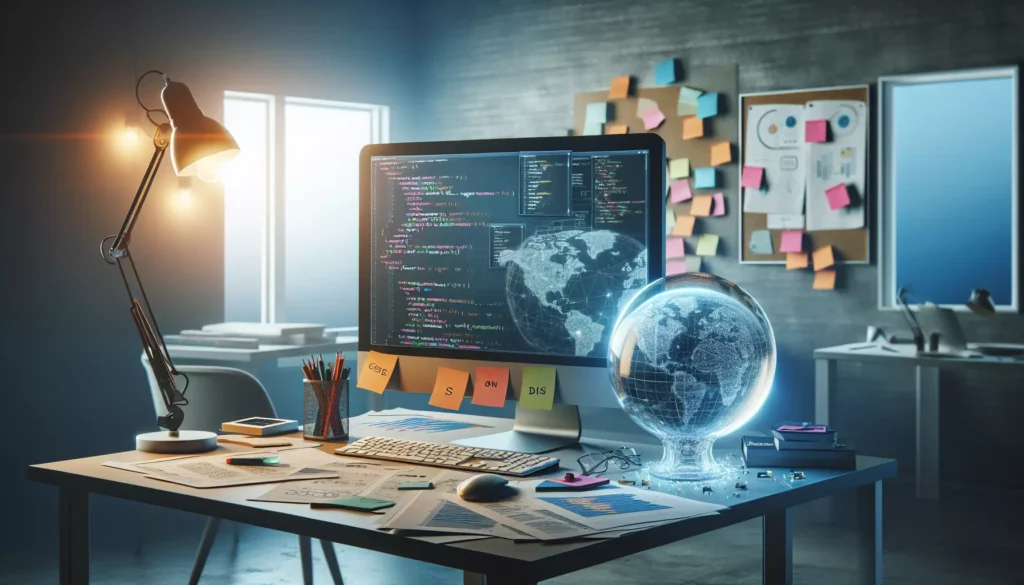
In the competitive landscape of tech industry job hunting, coding interviews have become a crucial hurdle for aspiring software engineers. While technical skills and knowledge of programming languages are undoubtedly important, there’s another key element that often separates successful candidates from the rest: critical thinking. This article delves deep into the role of critical thinking in coding interviews, exploring why it’s essential, how it’s evaluated, and most importantly, how you can cultivate and demonstrate this vital skill.
Understanding Critical Thinking in the Context of Coding
Before we dive into its application in interviews, let’s define what we mean by critical thinking in the realm of coding. Critical thinking in programming involves:
- Analyzing complex problems and breaking them down into manageable components
- Evaluating multiple potential solutions and selecting the most appropriate one
- Identifying patterns and making connections between different concepts
- Questioning assumptions and considering edge cases
- Adapting strategies when faced with new information or constraints
These skills go beyond mere coding proficiency; they represent a higher level of cognitive engagement with the task at hand. In the context of a coding interview, critical thinking is what allows you to tackle unfamiliar problems and devise elegant solutions under pressure.
Why Critical Thinking Matters in Coding Interviews
Coding interviews, especially those conducted by major tech companies often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google), are designed to assess more than just a candidate’s ability to write syntactically correct code. Here’s why critical thinking plays such a crucial role:
1. Problem-Solving in Unfamiliar Territory
Interviewers often present candidates with problems they’ve never encountered before. The ability to think critically allows you to approach these novel situations with confidence, breaking down the problem and applying known principles in new ways.
2. Efficiency and Optimization
It’s rarely enough to simply solve the problem; interviewers are looking for candidates who can identify the most efficient solution. Critical thinking enables you to analyze the time and space complexity of your algorithms and optimize them accordingly.
3. Adaptability
As you work through a problem, interviewers may introduce new constraints or ask you to consider different scenarios. Critical thinkers can pivot quickly, adjusting their approach based on new information.
4. Communication and Collaboration
Many coding interviews involve explaining your thought process as you code. Strong critical thinking skills allow you to articulate your reasoning clearly, demonstrating not just what you’re doing, but why you’re doing it.
5. Real-World Application
In actual software development roles, engineers frequently encounter complex, poorly-defined problems. Companies use coding interviews to gauge how candidates might perform in these real-world scenarios, where critical thinking is essential.
How Critical Thinking is Evaluated in Coding Interviews
Interviewers assess critical thinking through various aspects of the interview process:
1. Problem Analysis
From the moment a problem is presented, interviewers are observing how you approach it. Do you immediately start coding, or do you take time to understand the problem fully? Do you ask clarifying questions? Do you consider edge cases?
2. Solution Design
Before writing any code, many interviewers expect candidates to outline their approach. This stage is crucial for demonstrating critical thinking. Are you considering multiple solutions? Can you explain the trade-offs between different approaches?
3. Code Implementation
As you write code, interviewers are looking at how you structure your solution. Are you writing modular, readable code? Are you handling edge cases effectively? Can you explain why you’re making specific implementation choices?
4. Testing and Debugging
After implementing a solution, how do you verify its correctness? Critical thinking plays a big role in identifying potential issues and systematically debugging your code.
5. Optimization
If your initial solution works, interviewers often ask how you might improve it. This is where critical thinking really shines, as you analyze your own code for inefficiencies and propose enhancements.
6. Handling Hints and Feedback
Throughout the interview, you may receive hints or feedback. How you incorporate this information and adjust your approach is another key indicator of critical thinking skills.
Cultivating Critical Thinking for Coding Interviews
Now that we understand the importance of critical thinking in coding interviews, let’s explore strategies to develop and strengthen these skills:
1. Practice, Practice, Practice
Consistent practice is key to developing critical thinking skills for coding interviews. Platforms like AlgoCademy offer a wealth of resources, including:
- Interactive coding tutorials that guide you through problem-solving processes
- A diverse range of coding challenges, from beginner to advanced levels
- AI-powered assistance to provide hints and explanations as you work through problems
Regular engagement with these resources helps build the mental muscles needed for critical thinking in high-pressure interview situations.
2. Analyze Multiple Solutions
For each problem you encounter, challenge yourself to come up with at least two different solutions. Compare them in terms of time complexity, space complexity, and readability. This exercise strengthens your ability to evaluate trade-offs, a crucial aspect of critical thinking in coding.
3. Study Algorithm Design Paradigms
Familiarize yourself with common algorithm design paradigms such as divide-and-conquer, dynamic programming, and greedy algorithms. Understanding these paradigms provides a framework for approaching a wide variety of problems, enhancing your critical thinking capabilities.
4. Engage in Code Reviews
If possible, participate in code reviews, either in a professional setting or in online coding communities. Analyzing others’ code and receiving feedback on your own helps develop a critical eye for code quality and efficiency.
5. Verbalize Your Thought Process
Practice explaining your problem-solving approach out loud, as if you were in an interview. This not only improves your communication skills but also helps you identify gaps in your reasoning.
6. Seek Out Challenging Problems
Don’t shy away from difficult problems. Tackling challenges that are just beyond your current skill level is an excellent way to stretch your critical thinking abilities.
7. Learn from Others
Study how experienced programmers approach problems. Many coding platforms offer solution discussions where you can gain insights into different problem-solving strategies.
Demonstrating Critical Thinking During the Interview
When the day of your coding interview arrives, here are some strategies to effectively showcase your critical thinking skills:
1. Think Aloud
Verbalize your thought process as you work through the problem. This gives the interviewer insight into your critical thinking and problem-solving approach. For example:
// Thinking aloud example
"I'm considering using a hash map to store the frequency of each element, which would give us O(n) time complexity for lookup. However, this would require O(n) extra space. Let me explore if there's a way to solve this in-place..."
2. Ask Clarifying Questions
Don’t hesitate to ask questions about the problem statement. This demonstrates that you’re critically analyzing the problem before jumping into a solution. For instance:
- “Can we assume the input array is always sorted?”
- “What should the function return if there’s no valid solution?”
- “Are there any constraints on the input size or value range?”
3. Consider Edge Cases
Proactively discuss potential edge cases and how your solution handles them. This shows that you’re thinking critically about the full scope of the problem.
// Edge case consideration
"We should consider what happens if the input is an empty array. In that case, we could return null or throw an exception, depending on the desired behavior."
4. Analyze Time and Space Complexity
Before and after implementing your solution, discuss its time and space complexity. This demonstrates your ability to critically evaluate the efficiency of your code.
// Complexity analysis
"This solution has a time complexity of O(n log n) due to the sorting step, and uses O(1) extra space since we're sorting in-place. If we need to optimize for time, we could consider using a hash map approach, which would give us O(n) time complexity at the cost of O(n) space."
5. Propose Optimizations
After implementing an initial solution, proactively suggest ways to optimize it. This shows that you’re continually thinking critically about improving your code.
6. Handle Feedback Gracefully
If the interviewer provides hints or suggestions, demonstrate your critical thinking by thoughtfully incorporating this feedback into your approach.
Common Pitfalls to Avoid
While striving to demonstrate critical thinking, be aware of these common pitfalls:
1. Rushing to Code
Resist the urge to start coding immediately. Take time to think through the problem and outline your approach first.
2. Tunnel Vision
Don’t become so focused on one approach that you ignore alternative solutions. Be open to pivoting if a better approach becomes apparent.
3. Overcomplicating Solutions
Sometimes, the simplest solution is the best. Don’t overlook straightforward approaches in an attempt to appear clever.
4. Ignoring Constraints
Pay attention to any constraints mentioned in the problem statement. Critical thinking involves working within given parameters.
5. Failing to Test
Don’t consider your solution complete until you’ve tested it, including edge cases. Proactively testing your code demonstrates thorough critical thinking.
Real-World Application: A Case Study
Let’s examine how critical thinking might be applied in a typical coding interview question:
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
Here’s how a candidate might approach this problem, demonstrating critical thinking at each step:
1. Problem Analysis
"Before we begin, let me clarify a few things:
1. Can we assume the array is unsorted?
2. Are all numbers positive, or can we have negative numbers and zero?
3. Is there always exactly one solution, or could there be multiple pairs that sum to the target?
4. What should we return if no solution exists?"
2. Consider Multiple Approaches
"I can think of a few ways to approach this:
1. A brute force approach using nested loops, which would be O(n^2) time complexity.
2. Sorting the array first and then using two pointers, which would be O(n log n) time due to sorting.
3. Using a hash map to store complements, which could solve it in O(n) time.
Let's explore the hash map approach as it seems to offer the best time complexity."
3. Implementation with Explanation
public int[] twoSum(int[] nums, int target) {
// Use a hash map to store complements
Map<Integer, Integer> complementMap = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (complementMap.containsKey(complement)) {
// We've found a pair that sums to the target
return new int[] { complementMap.get(complement), i };
}
// Store this number and its index
complementMap.put(nums[i], i);
}
// If we reach here, no solution was found
throw new IllegalArgumentException("No two sum solution");
}
"This solution uses a hash map to store each number we've seen and its index. For each number, we calculate its complement (target - number) and check if we've already seen this complement. If we have, we've found our pair and can return their indices."
4. Complexity Analysis
"This solution has a time complexity of O(n) as we're making a single pass through the array, and hash map operations are generally O(1).
The space complexity is also O(n) in the worst case, where we might need to store nearly all numbers in the hash map before finding a solution."
5. Testing and Edge Cases
"Let's test this with a few cases:
1. Normal case: nums = [2, 7, 11, 15], target = 9 should return [0, 1]
2. Edge case - solution at the end: nums = [3, 2, 4], target = 6 should return [1, 2]
3. Edge case - negative numbers: nums = [-1, -2, -3, -4, -5], target = -8 should return [2, 4]
4. Edge case - no solution: nums = [1, 2, 3], target = 10 should throw an exception
We should also consider what happens with an empty array or if the array has fewer than two elements."
6. Potential Optimizations
"While this solution is already O(n) time and space, which is generally optimal for this problem, there are a couple of minor optimizations we could consider:
1. If we know the array is sorted, we could use a two-pointer approach which would be O(n) time and O(1) space.
2. If we're dealing with a very large array and memory is a concern, we could sacrifice some time complexity for space by using a two-pass hash table approach."
By walking through the problem in this manner, the candidate demonstrates strong critical thinking skills, showing their ability to analyze the problem, consider multiple approaches, implement a solution, and critically evaluate its performance and potential improvements.
Conclusion
Critical thinking is an indispensable skill in coding interviews, setting apart candidates who can not only code but also approach problems strategically and communicate their thought processes effectively. By understanding the role of critical thinking, actively cultivating these skills, and learning to demonstrate them during interviews, you can significantly enhance your performance and increase your chances of success.
Remember, the goal of coding interviews isn’t just to find someone who can write correct code, but to identify candidates who can think critically about complex problems, adapt to new challenges, and contribute meaningfully to the team’s problem-solving efforts. By focusing on developing your critical thinking skills alongside your technical knowledge, you’ll be well-prepared to tackle whatever challenges come your way in coding interviews and beyond.
Platforms like AlgoCademy provide valuable resources for honing both your coding skills and your critical thinking abilities. Through consistent practice, thoughtful analysis, and a commitment to continuous improvement, you can develop the critical thinking prowess that will serve you well not just in coding interviews, but throughout your career in software development.