The Importance of Practicing Common Algorithm Patterns
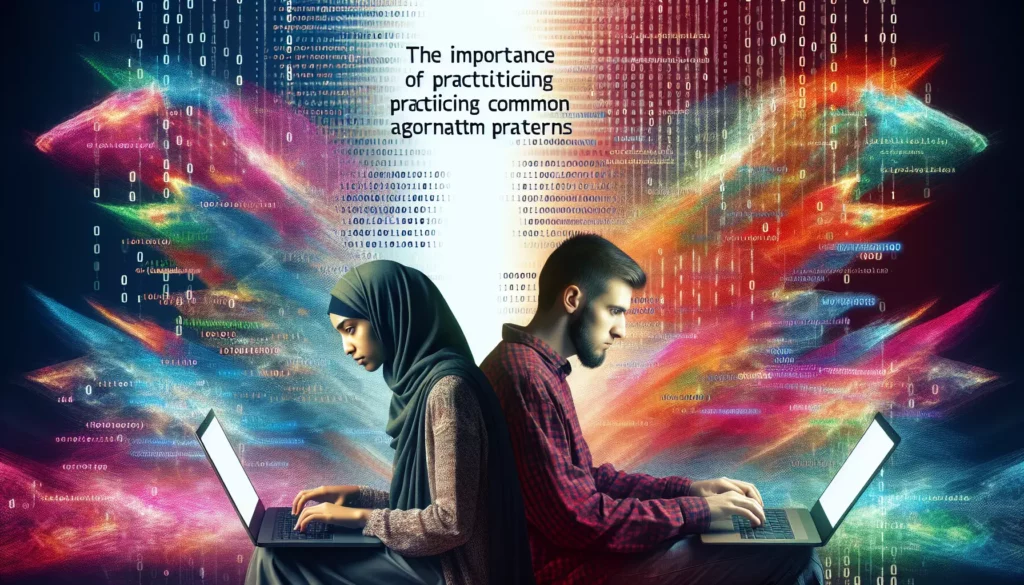
In the ever-evolving world of software development and computer science, mastering common algorithm patterns is not just a skill—it’s a necessity. Whether you’re a beginner coder or an experienced developer preparing for technical interviews at top tech companies, understanding and practicing these patterns can significantly boost your problem-solving abilities and coding prowess. In this comprehensive guide, we’ll explore why practicing common algorithm patterns is crucial, delve into some of the most important patterns, and provide strategies for mastering them.
Why Focus on Algorithm Patterns?
Before we dive into specific patterns, let’s consider why they’re so important:
- Efficiency in Problem-Solving: Recognizing patterns allows you to approach new problems with tried-and-true strategies, saving time and mental energy.
- Interview Preparation: Many technical interviews, especially at FAANG companies (Facebook, Amazon, Apple, Netflix, Google), focus heavily on algorithmic problem-solving.
- Code Optimization: Understanding these patterns helps you write more efficient and scalable code.
- Foundation for Advanced Concepts: Many complex algorithms are built upon these fundamental patterns.
- Language-Agnostic Skills: These patterns are applicable across programming languages, making you a more versatile developer.
Common Algorithm Patterns to Master
1. Two Pointers
The two pointers technique involves using two pointer variables to solve a problem, often to search pairs in a sorted array or linked list.
Example Problem: Reverse a String
function reverseString(s) {
let left = 0;
let right = s.length - 1;
while (left < right) {
[s[left], s[right]] = [s[right], s[left]];
left++;
right--;
}
return s;
}
This pattern is especially useful for problems involving sorted arrays or linked lists, where you need to find a pair of elements that satisfy certain conditions.
2. Sliding Window
The sliding window technique is used to perform operations on a specific window size of an array or linked list, such as finding the longest substring with unique characters.
Example Problem: Maximum Sum Subarray of Size K
function maxSubarraySum(arr, k) {
let maxSum = 0;
let windowSum = 0;
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
This pattern is particularly useful for problems involving subarrays or substrings, especially when dealing with contiguous sequences of elements.
3. Fast and Slow Pointers (Floyd’s Cycle-Finding Algorithm)
This pattern, also known as the “tortoise and hare” algorithm, is used to detect cycles in a linked list or to find the middle element of a linked list.
Example Problem: Detect Cycle in a Linked List
function hasCycle(head) {
if (!head || !head.next) return false;
let slow = head;
let fast = head.next;
while (slow !== fast) {
if (!fast || !fast.next) return false;
slow = slow.next;
fast = fast.next.next;
}
return true;
}
This pattern is incredibly useful for solving problems related to linked lists and can also be applied to problems involving arrays in some cases.
4. Merge Intervals
The merge intervals pattern deals with overlapping intervals. It’s useful for problems that involve combining ranges or finding overlaps.
Example Problem: Merge Overlapping Intervals
function mergeIntervals(intervals) {
if (intervals.length <= 1) return intervals;
intervals.sort((a, b) => a[0] - b[0]);
const result = [intervals[0]];
for (let i = 1; i < intervals.length; i++) {
const currentInterval = intervals[i];
const lastMergedInterval = result[result.length - 1];
if (currentInterval[0] <= lastMergedInterval[1]) {
lastMergedInterval[1] = Math.max(lastMergedInterval[1], currentInterval[1]);
} else {
result.push(currentInterval);
}
}
return result;
}
This pattern is particularly useful in scenarios involving time intervals, scheduling, or any problem where you need to merge overlapping ranges.
5. Cyclic Sort
Cyclic sort is a pattern used when dealing with arrays containing numbers in a given range. It’s especially useful for problems where you need to find missing numbers.
Example Problem: Find the Missing Number
function findMissingNumber(nums) {
let i = 0;
while (i < nums.length) {
if (nums[i] < nums.length && nums[i] !== nums[nums[i]]) {
[nums[nums[i]], nums[i]] = [nums[i], nums[nums[i]]];
} else {
i++;
}
}
for (let i = 0; i < nums.length; i++) {
if (nums[i] !== i) return i;
}
return nums.length;
}
This pattern is incredibly efficient for problems involving arrays where the numbers are in a fixed range, typically from 1 to n or 0 to n-1.
6. In-place Reversal of a Linked List
This pattern is used to reverse a linked list without using extra space. It’s a fundamental technique that comes up in many linked list problems.
Example Problem: Reverse a Linked List
function reverseLinkedList(head) {
let prev = null;
let current = head;
while (current !== null) {
let nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
This pattern is essential for any problem that involves manipulating the structure of a linked list, such as reordering or partitioning.
7. Tree Breadth First Search (BFS)
BFS is used to traverse or search tree or graph data structures. It explores all the nodes at the present depth before moving on to the nodes at the next depth level.
Example Problem: Level Order Traversal of a Binary Tree
function levelOrder(root) {
if (!root) return [];
const result = [];
const queue = [root];
while (queue.length > 0) {
const levelSize = queue.length;
const currentLevel = [];
for (let i = 0; i < levelSize; i++) {
const node = queue.shift();
currentLevel.push(node.val);
if (node.left) queue.push(node.left);
if (node.right) queue.push(node.right);
}
result.push(currentLevel);
}
return result;
}
BFS is particularly useful for problems involving the shortest path or minimum steps in a tree or graph.
8. Tree Depth First Search (DFS)
DFS is another tree traversal technique that explores as far as possible along each branch before backtracking. It’s often implemented using recursion.
Example Problem: Inorder Traversal of a Binary Tree
function inorderTraversal(root) {
const result = [];
function dfs(node) {
if (node === null) return;
dfs(node.left);
result.push(node.val);
dfs(node.right);
}
dfs(root);
return result;
}
DFS is useful for problems that require exploring all possible paths or making decisions at each step in a tree-like structure.
9. Two Heaps
The two heaps pattern uses two heap data structures, typically a min heap and a max heap, to solve problems efficiently.
Example Problem: Find the Median from a Data Stream
class MedianFinder {
constructor() {
this.maxHeap = new MaxPriorityQueue();
this.minHeap = new MinPriorityQueue();
}
addNum(num) {
if (this.maxHeap.isEmpty() || num < this.maxHeap.front().element) {
this.maxHeap.enqueue(num);
} else {
this.minHeap.enqueue(num);
}
if (this.maxHeap.size() > this.minHeap.size() + 1) {
this.minHeap.enqueue(this.maxHeap.dequeue().element);
} else if (this.minHeap.size() > this.maxHeap.size()) {
this.maxHeap.enqueue(this.minHeap.dequeue().element);
}
}
findMedian() {
if (this.maxHeap.size() === this.minHeap.size()) {
return (this.maxHeap.front().element + this.minHeap.front().element) / 2;
} else {
return this.maxHeap.front().element;
}
}
}
This pattern is particularly useful for problems involving processing streams of data or finding a median in a dynamic set of numbers.
10. Subsets
The subsets pattern is used to deal with problems where we need to find all the possible combinations (subsets) of the given set or array.
Example Problem: Generate All Subsets of a Set
function subsets(nums) {
const result = [[]];
for (const num of nums) {
const currentSubsets = result.map(subset => [...subset, num]);
result.push(...currentSubsets);
}
return result;
}
This pattern is useful for combinatorial problems and is often solved using recursion or bit manipulation.
Strategies for Mastering Algorithm Patterns
Now that we’ve covered some of the most important algorithm patterns, let’s discuss strategies for mastering them:
- Consistent Practice: Regularly solve problems that utilize these patterns. Platforms like AlgoCademy, LeetCode, and HackerRank offer a wealth of problems.
- Understand the Underlying Principles: Don’t just memorize solutions. Understand why a particular pattern works for a given problem.
- Implement from Scratch: Try to implement these patterns without referring to previous solutions. This helps solidify your understanding.
- Analyze Time and Space Complexity: For each solution, practice analyzing its time and space complexity. This will help you understand the efficiency of different approaches.
- Learn to Recognize Pattern Applicability: As you practice, you’ll start to recognize which patterns are applicable to which types of problems. This skill is crucial for technical interviews.
- Review and Reflect: After solving a problem, take time to reflect on your approach. Could you have used a different pattern? Was there a more efficient solution?
- Teach Others: Explaining these patterns to others can significantly enhance your own understanding.
- Use Visualization Tools: Many online platforms offer visualization tools that can help you understand how these algorithms work step-by-step.
- Participate in Coding Contests: Participating in timed coding contests can help you apply these patterns under pressure, similar to interview conditions.
- Read and Analyze Code: Study well-written implementations of these patterns. This can give you insights into best practices and efficient coding techniques.
The Role of AlgoCademy in Mastering Algorithm Patterns
AlgoCademy plays a crucial role in helping developers master these common algorithm patterns:
- Structured Learning Path: AlgoCademy provides a structured curriculum that covers these patterns in a logical, easy-to-follow sequence.
- Interactive Tutorials: The platform offers interactive coding tutorials that allow you to practice implementing these patterns in real-time.
- AI-Powered Assistance: Get personalized hints and feedback as you work through problems, helping you understand and apply these patterns more effectively.
- Comprehensive Problem Sets: Access a wide range of problems specifically designed to reinforce your understanding of each pattern.
- Progress Tracking: Monitor your progress and identify areas where you need more practice.
- Community Support: Engage with a community of learners and mentors to discuss different approaches and solutions.
- Interview Preparation: Specific modules focused on preparing you for technical interviews at top tech companies, with an emphasis on applying these patterns in interview-style questions.
Conclusion
Mastering common algorithm patterns is an essential skill for any software developer, particularly those aiming for positions at top tech companies. These patterns provide a framework for approaching a wide variety of coding problems efficiently and effectively. By understanding and practicing these patterns, you’ll not only improve your problem-solving skills but also be better prepared for technical interviews and real-world coding challenges.
Remember, the key to mastery is consistent practice and deep understanding. Platforms like AlgoCademy offer the tools and resources you need to develop these skills systematically. Whether you’re a beginner looking to build a strong foundation or an experienced developer preparing for your next big interview, focusing on these common algorithm patterns will significantly enhance your coding abilities and career prospects.
Happy coding, and may your journey in mastering these algorithm patterns be both challenging and rewarding!