Why Analyzing Edge Cases is Critical for Problem Solving
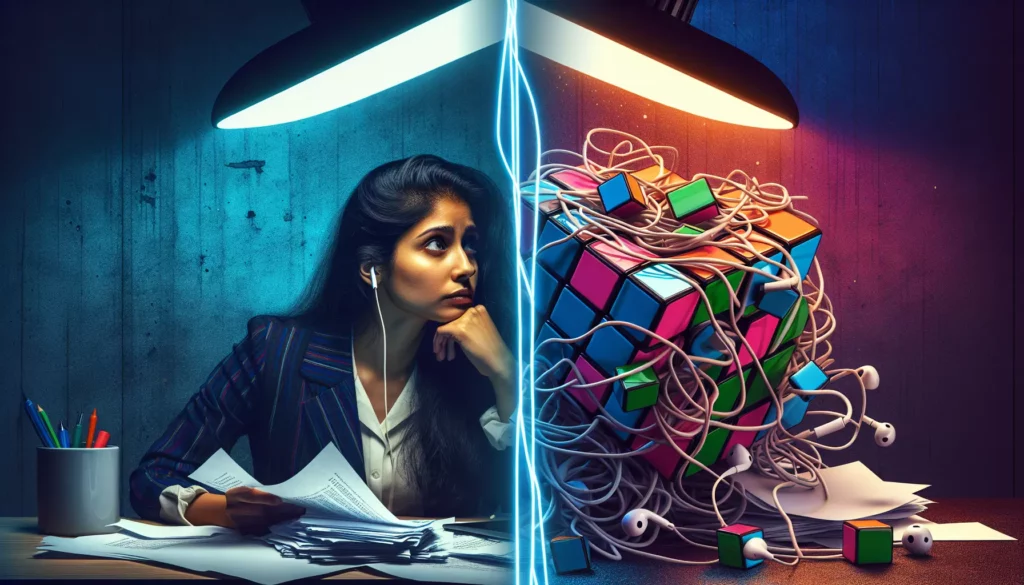
In the world of programming and software development, problem-solving is a fundamental skill that separates great developers from good ones. One crucial aspect of effective problem-solving is the ability to analyze and account for edge cases. Edge cases are situations that occur at the extremes of operating parameters or involve unexpected inputs or conditions. In this comprehensive guide, we’ll explore why analyzing edge cases is critical for problem-solving and how it can significantly improve your coding skills.
Understanding Edge Cases
Before diving into the importance of analyzing edge cases, let’s first define what they are and why they matter in programming.
What are Edge Cases?
Edge cases are scenarios that occur at the extreme ends of possible inputs or conditions in a program. These are situations that may be rare or unexpected but can still occur and potentially cause issues if not properly handled. Some common examples of edge cases include:
- Empty inputs or null values
- Extremely large or small numbers
- Boundary conditions (e.g., the first or last element of an array)
- Invalid or unexpected input formats
- Resource limitations (e.g., memory constraints)
Why Edge Cases Matter
Edge cases are important because they often reveal weaknesses or bugs in your code that may not be apparent during normal operation. Failing to account for edge cases can lead to:
- Crashes or unexpected behavior in your program
- Security vulnerabilities
- Poor user experience
- Difficulty in maintaining and scaling your code
By identifying and addressing edge cases early in the development process, you can create more robust, reliable, and secure software.
The Critical Role of Edge Case Analysis in Problem Solving
Now that we understand what edge cases are, let’s explore why analyzing them is crucial for effective problem-solving in programming.
1. Enhances Code Reliability
One of the primary benefits of analyzing edge cases is that it significantly improves the reliability of your code. By considering various scenarios that might occur, including rare or unexpected ones, you can ensure that your program behaves correctly under a wide range of conditions.
For example, consider a function that calculates the average of a list of numbers:
def calculate_average(numbers):
total = sum(numbers)
return total / len(numbers)
This function works fine for most inputs, but it fails to account for an important edge case: an empty list. If we pass an empty list to this function, it will raise a ZeroDivisionError. By analyzing edge cases, we can improve the function to handle this scenario:
def calculate_average(numbers):
if not numbers:
return 0 # or raise an exception, depending on requirements
total = sum(numbers)
return total / len(numbers)
By accounting for the edge case of an empty list, we’ve made our function more reliable and less prone to unexpected errors.
2. Improves Security
Edge cases often reveal potential security vulnerabilities in your code. Attackers frequently exploit edge cases to bypass security measures or cause unexpected behavior. By thoroughly analyzing edge cases, you can identify and mitigate these potential security risks.
For instance, consider a login function that validates user input:
def validate_login(username, password):
if len(username) > 0 and len(password) > 0:
# Perform login logic
return True
else:
return False
While this function checks for non-empty username and password, it doesn’t account for other edge cases that could pose security risks. An improved version might look like this:
def validate_login(username, password):
if not isinstance(username, str) or not isinstance(password, str):
return False
if len(username) == 0 or len(password) == 0:
return False
if len(username) > 50 or len(password) > 100: # Prevent excessively long inputs
return False
# Additional checks for valid characters, etc.
# Perform login logic
return True
By considering edge cases such as non-string inputs, empty strings, and excessively long inputs, we’ve made the function more secure against potential attacks.
3. Enhances User Experience
Analyzing edge cases helps you create a better user experience by anticipating and handling unexpected situations gracefully. When your program can handle edge cases smoothly, users are less likely to encounter frustrating errors or unexpected behavior.
Consider a function that parses a date string:
from datetime import datetime
def parse_date(date_string):
return datetime.strptime(date_string, '%Y-%m-%d')
This function works for correctly formatted date strings, but it will raise a ValueError for invalid inputs. By considering edge cases, we can improve the user experience:
from datetime import datetime
def parse_date(date_string):
try:
return datetime.strptime(date_string, '%Y-%m-%d')
except ValueError:
print(f"Invalid date format: {date_string}. Please use YYYY-MM-DD.")
return None
Now, instead of crashing, the function provides helpful feedback to the user when given an invalid input, resulting in a better user experience.
4. Facilitates Thorough Testing
Analyzing edge cases is an essential part of the testing process. By identifying potential edge cases early, you can create more comprehensive test suites that cover a wider range of scenarios. This leads to more robust and reliable code.
For example, consider a function that calculates the factorial of a number:
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
When testing this function, you might consider the following edge cases:
- n = 0 (base case)
- n = 1 (smallest positive integer)
- Large values of n (to test for potential stack overflow)
- Negative values of n (which should raise an error)
- Non-integer inputs
By considering these edge cases, you can create a more thorough test suite:
import unittest
class TestFactorial(unittest.TestCase):
def test_factorial_zero(self):
self.assertEqual(factorial(0), 1)
def test_factorial_one(self):
self.assertEqual(factorial(1), 1)
def test_factorial_positive(self):
self.assertEqual(factorial(5), 120)
def test_factorial_large(self):
self.assertEqual(factorial(20), 2432902008176640000)
def test_factorial_negative(self):
with self.assertRaises(ValueError):
factorial(-1)
def test_factorial_non_integer(self):
with self.assertRaises(TypeError):
factorial(3.14)
if __name__ == '__main__':
unittest.main()
This comprehensive test suite covers various edge cases, ensuring that the factorial function behaves correctly under different conditions.
5. Improves Problem-Solving Skills
Regularly analyzing edge cases sharpens your problem-solving skills and trains you to think more critically about your code. It encourages you to consider different scenarios and potential issues, which can lead to more elegant and efficient solutions.
For instance, let’s consider a function that finds the maximum value in an array:
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
While this function works for most cases, thinking about edge cases might lead you to consider:
- What if the array is empty?
- What if the array contains non-numeric values?
- What if the array is very large and we want to optimize for performance?
Considering these edge cases might lead to an improved version of the function:
from typing import List, Union
def find_max(arr: List[Union[int, float]]) -> Union[int, float]:
if not arr:
raise ValueError("Cannot find maximum of an empty array")
return max(arr) # Using built-in max() for better performance
This improved version handles the empty array edge case, uses type hints to ensure numeric inputs, and leverages the built-in max() function for better performance on large arrays.
Strategies for Effective Edge Case Analysis
Now that we understand the importance of analyzing edge cases, let’s explore some strategies to effectively identify and handle them in your problem-solving process.
1. Brainstorm Potential Scenarios
Before writing any code, take some time to brainstorm different scenarios that your function or program might encounter. Consider:
- Minimum and maximum possible values
- Empty or null inputs
- Invalid or unexpected input types
- Boundary conditions
- Resource limitations
By thinking through these scenarios beforehand, you can design your solution to handle them from the start.
2. Use Input Validation
Implement input validation to catch and handle unexpected inputs early. This can prevent errors from propagating through your program and make it easier to identify the source of issues.
def process_age(age):
if not isinstance(age, int):
raise TypeError("Age must be an integer")
if age < 0 or age > 150:
raise ValueError("Age must be between 0 and 150")
# Process the age
return f"Your age is {age}"
3. Implement Error Handling
Use try-except blocks to catch and handle potential errors gracefully. This allows your program to continue running or provide useful feedback instead of crashing when encountering unexpected situations.
def safe_divide(a, b):
try:
result = a / b
except ZeroDivisionError:
print("Error: Division by zero is not allowed")
result = None
except TypeError:
print("Error: Both inputs must be numbers")
result = None
return result
4. Use Assertions
Assertions can be helpful for catching unexpected conditions during development and testing. They allow you to specify conditions that should always be true and can help identify edge cases that you might have missed.
def calculate_average(numbers):
assert len(numbers) > 0, "List cannot be empty"
assert all(isinstance(n, (int, float)) for n in numbers), "All elements must be numeric"
return sum(numbers) / len(numbers)
5. Leverage Type Hinting
In languages that support it, use type hinting to clarify expected input and output types. This can help catch type-related edge cases early and make your code more self-documenting.
from typing import List, Union
def find_median(numbers: List[Union[int, float]]) -> float:
sorted_numbers = sorted(numbers)
length = len(sorted_numbers)
if length % 2 == 0:
return (sorted_numbers[length//2 - 1] + sorted_numbers[length//2]) / 2
else:
return sorted_numbers[length//2]
6. Write Comprehensive Tests
Develop a comprehensive test suite that includes tests for normal cases as well as edge cases. This helps ensure that your code behaves correctly under various conditions and makes it easier to catch regressions when making changes.
import unittest
class TestFindMedian(unittest.TestCase):
def test_odd_length(self):
self.assertEqual(find_median([1, 3, 2]), 2)
def test_even_length(self):
self.assertEqual(find_median([1, 2, 3, 4]), 2.5)
def test_single_element(self):
self.assertEqual(find_median([5]), 5)
def test_empty_list(self):
with self.assertRaises(ValueError):
find_median([])
def test_mixed_types(self):
self.assertEqual(find_median([1, 2.5, 3]), 2.5)
if __name__ == '__main__':
unittest.main()
Real-World Examples of Edge Case Analysis
To further illustrate the importance of edge case analysis, let’s look at some real-world examples where failing to consider edge cases led to significant issues.
1. The Y2K Bug
The Year 2000 problem, or Y2K bug, is a classic example of failing to consider long-term edge cases. Many early computer programs represented years with two digits (e.g., “99” for 1999) to save memory. However, this approach didn’t account for the transition to the year 2000, potentially causing date-related calculations to fail.
Lesson learned: When designing systems, consider not just immediate requirements but also potential future scenarios and long-term implications.
2. Ariane 5 Rocket Explosion
In 1996, the Ariane 5 rocket exploded just 40 seconds after launch due to a software error. The error occurred when a 64-bit floating-point number was converted to a 16-bit signed integer, which was much larger than the maximum value the 16-bit integer could hold.
Lesson learned: Always consider the range of possible values when performing type conversions, and implement proper error handling for out-of-range values.
3. Twitter’s 140-Character Limit
Twitter’s original 140-character limit was based on the 160-character limit of SMS messages, minus 20 characters for usernames. However, this limit didn’t account for certain edge cases, such as URLs, which could take up a significant portion of the character count. Twitter later introduced URL shortening and eventually doubled the character limit to 280 to address these issues.
Lesson learned: Consider how different types of content or input might interact with system limitations, and be prepared to adapt your design as usage patterns evolve.
Conclusion
Analyzing edge cases is a critical skill for effective problem-solving in programming. It enhances code reliability, improves security, creates better user experiences, facilitates thorough testing, and sharpens your overall problem-solving abilities. By considering edge cases, you can create more robust, secure, and user-friendly software.
As you continue to develop your coding skills, make edge case analysis an integral part of your problem-solving process. Regularly challenge yourself to think beyond the normal use cases and consider potential extreme or unexpected scenarios. This practice will not only improve the quality of your code but also make you a more valuable and effective developer.
Remember, the ability to anticipate and handle edge cases is what often separates great developers from good ones. It’s a skill that’s highly valued in technical interviews, especially at top tech companies. So, the next time you’re solving a coding problem, ask yourself: “What are the edge cases, and how can I handle them?” Your future self (and your users) will thank you for it.