How to Improve Your Ability to Think Under Pressure: A Programmer’s Guide
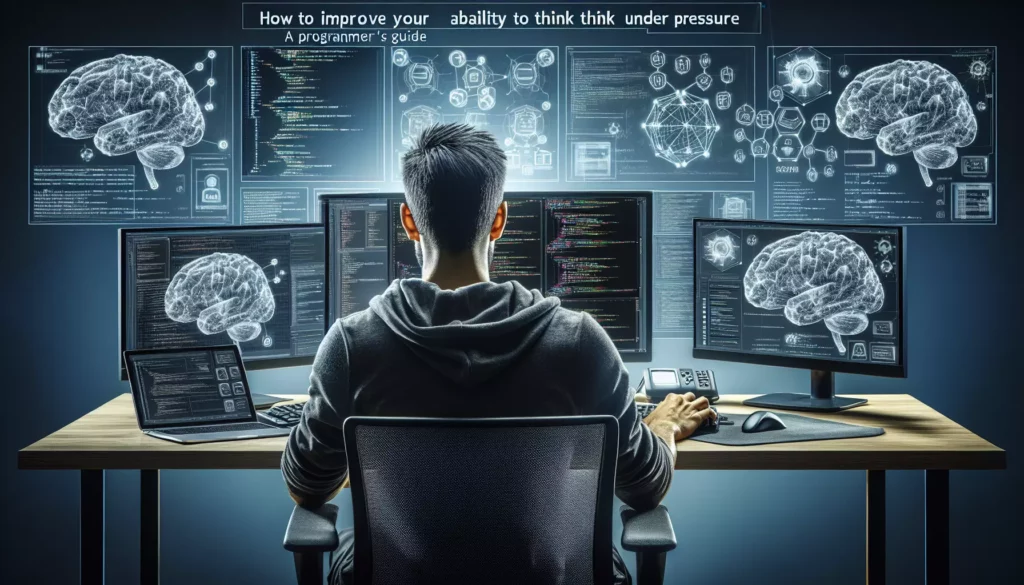
In the fast-paced world of software development, the ability to think clearly and solve problems under pressure is a crucial skill. Whether you’re facing a tight deadline, debugging a critical issue, or tackling a challenging technical interview, your capacity to maintain composure and deliver results when the heat is on can make or break your success. This comprehensive guide will explore various strategies and techniques to enhance your ability to think under pressure, with a specific focus on programming and coding challenges.
Understanding Pressure in Programming Contexts
Before diving into improvement strategies, it’s essential to understand what pressure looks like in programming contexts:
- Technical interviews at major tech companies (FAANG)
- Tight project deadlines
- Live coding sessions or pair programming
- Debugging critical production issues
- Competitive programming contests
- Code reviews and presentations
In these situations, the stakes are high, time is limited, and your cognitive abilities are put to the test. The good news is that with the right mindset and practice, you can significantly improve your performance under pressure.
1. Develop a Strong Foundation in Algorithmic Thinking
One of the best ways to improve your ability to think under pressure is to build a solid foundation in algorithmic thinking and problem-solving skills. This foundation acts as a safety net, giving you confidence and a structured approach when faced with challenging situations.
Key strategies:
- Master fundamental data structures (arrays, linked lists, trees, graphs, etc.)
- Study and practice common algorithms (sorting, searching, dynamic programming, etc.)
- Solve diverse coding problems regularly
- Analyze time and space complexity of algorithms
By strengthening your algorithmic thinking skills, you’ll be better equipped to break down complex problems into manageable parts, even when under pressure.
Practice example:
Let’s consider a common interview question: reversing a linked list. Having a strong foundation in data structures will help you approach this problem calmly and methodically, even under pressure.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev
Understanding the structure of a linked list and the concept of pointer manipulation allows you to implement this solution confidently, even in a high-pressure interview setting.
2. Practice Time Management and Prioritization
Effective time management is crucial when working under pressure. In programming, this skill is particularly important during technical interviews, competitive coding contests, or when facing tight project deadlines.
Strategies to improve time management:
- Use the Pomodoro Technique for focused work sessions
- Break larger tasks into smaller, manageable chunks
- Prioritize tasks based on importance and urgency
- Learn to estimate task completion times accurately
- Use time-boxing for open-ended problems
When practicing coding problems, set time limits for yourself to simulate pressure situations. This will help you get comfortable with working efficiently under time constraints.
Example: Time-boxing in a technical interview
Suppose you’re given a complex algorithmic problem during an interview with a 30-minute time limit. Here’s how you might approach it using time-boxing:
- Spend 5 minutes understanding the problem and asking clarifying questions
- Allocate 10 minutes for designing a solution and discussing the approach with the interviewer
- Use 10 minutes to implement the core logic of your solution
- Reserve the final 5 minutes for testing and optimization
By following this structured approach, you can maintain composure and make progress even when faced with a challenging problem under time pressure.
3. Simulate Pressure Situations
One of the most effective ways to improve your ability to think under pressure is to regularly expose yourself to pressure situations in a controlled environment. This helps you build resilience and familiarity with the physiological and psychological effects of stress.
Ways to simulate pressure in coding contexts:
- Participate in timed coding competitions (e.g., LeetCode contests, HackerRank challenges)
- Conduct mock interviews with peers or mentors
- Set up coding sessions with artificial time constraints
- Practice explaining your code and thought process while solving problems
The more you expose yourself to these simulated pressure situations, the more comfortable and confident you’ll become when facing real high-stakes scenarios.
Example: Mock Interview Simulation
Here’s a sample structure for a mock interview session to help you practice thinking under pressure:
- Choose a challenging algorithmic problem
- Set a timer for 45 minutes
- Have a friend or mentor act as the interviewer
- Solve the problem while explaining your thought process out loud
- Implement your solution on a whiteboard or in a simple text editor (avoid using IDE features)
- Answer follow-up questions and discuss optimizations
Regularly engaging in such simulations will help you become more comfortable with the interview process and improve your ability to think clearly under pressure.
4. Develop Stress Management Techniques
Managing stress is crucial for maintaining clear thinking under pressure. By developing effective stress management techniques, you can keep your mind sharp and focused even in high-pressure situations.
Stress management strategies for programmers:
- Practice deep breathing exercises
- Use progressive muscle relaxation techniques
- Implement mindfulness and meditation practices
- Maintain a healthy work-life balance
- Exercise regularly to reduce overall stress levels
Incorporating these techniques into your daily routine can help you stay calm and focused when facing pressure in your programming work or interviews.
Example: Deep Breathing Exercise
Try this simple deep breathing exercise before a high-pressure coding session or interview:
- Sit comfortably with your back straight
- Inhale slowly through your nose for a count of 4
- Hold your breath for a count of 4
- Exhale slowly through your mouth for a count of 4
- Repeat this cycle 5-10 times
This technique, known as “box breathing,” can help reduce anxiety and improve focus, allowing you to think more clearly under pressure.
5. Enhance Your Problem-Solving Process
Having a structured problem-solving process can provide a valuable framework when you’re under pressure. It helps you approach problems methodically, even when your mind might be racing due to stress.
Steps to enhance your problem-solving process:
- Understand the problem thoroughly
- Break down the problem into smaller, manageable parts
- Identify patterns or similarities to known problems
- Consider multiple approaches before implementation
- Start with a brute-force solution, then optimize
- Test your solution with various inputs, including edge cases
By internalizing this process, you’ll have a reliable approach to fall back on when faced with challenging problems under pressure.
Example: Applying the problem-solving process
Let’s apply this process to a common coding interview question: finding the longest palindromic substring in a given string.
def longestPalindrome(s):
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
By following a structured problem-solving process, you can approach this problem step-by-step, even under pressure:
- Understand the problem: We need to find the longest substring that reads the same forwards and backwards.
- Break it down: We can check palindromes centered at each character (odd length) and between each pair of characters (even length).
- Identify patterns: This is similar to the “expand around center” approach used in other palindrome problems.
- Consider approaches: We could use dynamic programming, but the expand around center approach is more intuitive and space-efficient.
- Implement: Start with the core logic of expanding around a center, then build the full solution.
- Test: Check with various inputs, including empty strings, single characters, and strings with multiple palindromes.
6. Build a Strong Knowledge Base
Having a deep understanding of programming concepts, languages, and tools can significantly boost your confidence when working under pressure. The more knowledge you have at your fingertips, the less likely you are to be caught off guard by unexpected challenges.
Areas to focus on:
- Master your primary programming language(s)
- Study design patterns and architectural principles
- Understand operating systems and computer networks
- Learn about databases and data modeling
- Explore cloud computing and distributed systems
Continuously expanding your knowledge base will help you approach problems from multiple angles and adapt to new challenges more easily.
Example: Applying design patterns under pressure
Suppose you’re in a system design interview and need to design a logging system for a distributed application. Knowledge of the Observer pattern can help you quickly propose a solution:
from abc import ABC, abstractmethod
class Subject:
def __init__(self):
self._observers = []
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self, message):
for observer in self._observers:
observer.update(message)
class Observer(ABC):
@abstractmethod
def update(self, message):
pass
class LoggingService(Subject):
def log(self, message):
print(f"Logging: {message}")
self.notify(message)
class FileLogger(Observer):
def update(self, message):
print(f"Writing to file: {message}")
class DatabaseLogger(Observer):
def update(self, message):
print(f"Writing to database: {message}")
# Usage
logging_service = LoggingService()
file_logger = FileLogger()
db_logger = DatabaseLogger()
logging_service.attach(file_logger)
logging_service.attach(db_logger)
logging_service.log("Application started")
By having a strong grasp of design patterns, you can quickly propose and implement flexible, scalable solutions even under the pressure of an interview or tight deadline.
7. Develop Resilience and a Growth Mindset
Resilience and a growth mindset are crucial for maintaining composure and continuing to perform well under pressure, especially when faced with setbacks or particularly challenging problems.
Strategies to build resilience and cultivate a growth mindset:
- Embrace challenges as opportunities for growth
- View failures as learning experiences
- Practice self-compassion and avoid negative self-talk
- Set realistic goals and celebrate small victories
- Seek feedback and learn from criticism
- Develop a support network of peers and mentors
By adopting these mindsets, you’ll be better equipped to handle the emotional and psychological challenges that come with high-pressure situations in programming.
Example: Reframing a coding challenge
Imagine you’re struggling with a difficult algorithm problem during a coding interview. Instead of thinking, “I can’t solve this. I’m not good enough,” try reframing your thoughts:
“This is a challenging problem, but it’s an opportunity for me to learn and grow. Even if I don’t solve it completely, I can demonstrate my problem-solving process and learn from this experience.”
This mindset shift can help you stay calm and focused, allowing you to think more clearly under pressure.
8. Improve Your Debugging and Troubleshooting Skills
Strong debugging and troubleshooting skills are invaluable when working under pressure, especially when dealing with critical issues or tight deadlines. The ability to quickly identify and resolve problems can significantly reduce stress and improve your overall performance.
Tips for enhancing debugging skills:
- Master your IDE’s debugging tools
- Learn to read and understand error messages effectively
- Practice rubber duck debugging
- Develop a systematic approach to isolating issues
- Familiarize yourself with common error patterns in your programming language
- Use logging and monitoring tools effectively
Example: Debugging under pressure
Consider a scenario where you’re given a piece of code that’s throwing an unexpected exception, and you need to fix it quickly. Here’s an approach you might take:
def process_data(data):
result = []
for item in data:
value = item['value']
processed = value * 2
result.append(processed)
return result
# This code is throwing a KeyError exception
sample_data = [{'id': 1}, {'id': 2, 'value': 5}, {'id': 3, 'value': 10}]
try:
output = process_data(sample_data)
print(output)
except KeyError as e:
print(f"KeyError occurred: {e}")
# Add debugging information
for i, item in enumerate(sample_data):
print(f"Item {i}: {item}")
# Fix the issue
def safe_process_data(data):
result = []
for item in data:
value = item.get('value', 0) # Use get() with a default value
processed = value * 2
result.append(processed)
return result
output = safe_process_data(sample_data)
print("Fixed output:", output)
In this example, we quickly identify the issue (missing ‘value’ key in some dictionary items), add debugging information to understand the data structure, and implement a fix using the `get()` method with a default value. This systematic approach to debugging can help you resolve issues efficiently, even under pressure.
9. Cultivate Effective Communication Skills
Strong communication skills are crucial when working under pressure, especially in collaborative environments or during technical interviews. The ability to articulate your thoughts clearly and concisely can help you navigate challenging situations more effectively.
Ways to improve communication skills:
- Practice explaining complex technical concepts in simple terms
- Learn to ask clarifying questions effectively
- Develop active listening skills
- Improve your written communication for documentation and code comments
- Practice giving and receiving constructive feedback
Example: Communicating during a technical interview
Imagine you’re asked to solve a complex problem during an interview. Here’s how effective communication can help:
- Restate the problem to ensure understanding:
“So, if I understand correctly, we need to implement a function that finds the kth smallest element in an unsorted array. Is that right?” - Ask clarifying questions:
“Are there any constraints on the input size or the range of values in the array? Can we assume all elements are unique?” - Explain your approach before coding:
“I’m thinking of using a min-heap to solve this problem. We can push all elements into the heap and then pop k times to get the kth smallest element. This would give us a time complexity of O(n log n) for building the heap and O(k log n) for the k pop operations. Does that approach sound reasonable to you?” - Think aloud while implementing:
“Now, I’ll import the heapq module to use Python’s built-in min-heap implementation. Then, I’ll define the function and start by creating a heap from the input array…” - Discuss potential optimizations:
“We could potentially optimize this further by using a max-heap of size k instead, which would reduce our space complexity to O(k) and time complexity to O(n log k). Would you like me to implement that version?”
By communicating clearly throughout the process, you demonstrate your problem-solving skills and thought process, even if you encounter difficulties along the way.
10. Continuously Refine Your Skills
Improving your ability to think under pressure is an ongoing process. Continuous learning and refinement of your skills will help you stay prepared for any challenges you might face in your programming career.
Strategies for continuous improvement:
- Regularly participate in coding challenges and competitions
- Contribute to open-source projects
- Attend programming workshops and conferences
- Read technical blogs and stay updated with industry trends
- Teach or mentor others to reinforce your own knowledge
- Seek out challenging projects at work or in personal projects
By consistently pushing yourself to learn and grow, you’ll build confidence in your abilities and be better equipped to handle pressure situations.
Conclusion
Improving your ability to think under pressure is a valuable skill that can significantly enhance your performance as a programmer. By developing a strong foundation in algorithmic thinking, practicing time management, simulating pressure situations, managing stress effectively, and continuously refining your skills, you can build the confidence and composure needed to excel in high-stakes programming scenarios.
Remember that improvement is a journey, not a destination. Be patient with yourself, celebrate your progress, and keep pushing your boundaries. With consistent effort and the right mindset, you can transform pressure situations from sources of stress into opportunities for growth and achievement in your programming career.