How to Develop a Problem-Solving Mindset for Technical Interviews
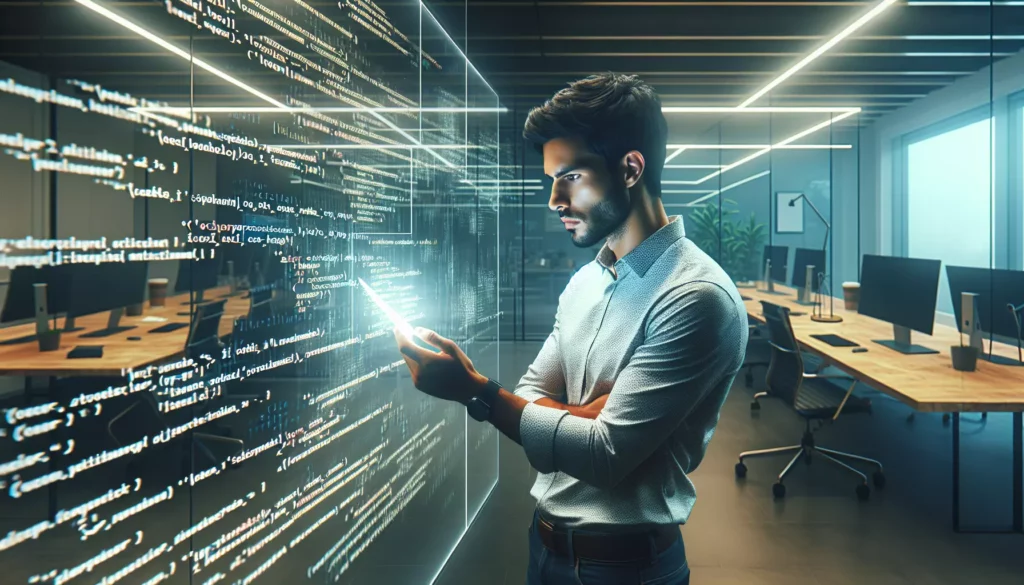
In the competitive world of tech, mastering technical interviews is crucial for landing your dream job. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, developing a strong problem-solving mindset is essential. This article will guide you through the process of cultivating this mindset, helping you approach technical interviews with confidence and skill.
Understanding the Importance of a Problem-Solving Mindset
Before diving into the strategies for developing a problem-solving mindset, it’s crucial to understand why it’s so important in the context of technical interviews. Technical interviews, especially those at top tech companies, are designed to assess not just your coding skills, but your ability to think critically, analyze complex problems, and devise efficient solutions.
A problem-solving mindset encompasses several key qualities:
- Analytical thinking
- Creativity in approaching challenges
- Resilience in the face of difficult problems
- Ability to break down complex issues into manageable parts
- Effective communication of thought processes
By developing these qualities, you’ll not only perform better in technical interviews but also become a more valuable asset to potential employers.
Strategies for Developing a Problem-Solving Mindset
1. Practice, Practice, Practice
The old adage “practice makes perfect” holds especially true when it comes to problem-solving in coding. Regular practice is essential for honing your skills and building confidence. Here are some ways to incorporate practice into your routine:
- Solve coding challenges daily: Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of problems to solve.
- Participate in coding competitions: Websites like Codeforces and TopCoder host regular competitions that can sharpen your skills under time pressure.
- Contribute to open-source projects: This gives you real-world problem-solving experience and exposure to different coding styles and approaches.
2. Study Algorithms and Data Structures
A solid understanding of algorithms and data structures is fundamental to problem-solving in coding. Focus on mastering these key areas:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Sorting and Searching algorithms
- Dynamic Programming
- Greedy Algorithms
Resources like “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein, and online courses on platforms like Coursera and edX can provide structured learning in these areas.
3. Develop a Systematic Approach to Problem-Solving
Having a structured approach to tackling problems can significantly improve your problem-solving skills. Here’s a step-by-step method you can follow:
- Understand the problem: Read the problem statement carefully and clarify any ambiguities.
- Analyze the inputs and outputs: Identify what’s given and what needs to be calculated or returned.
- Break down the problem: Divide the main problem into smaller, manageable sub-problems.
- Plan your approach: Think about potential algorithms or data structures that could be useful.
- Implement the solution: Start coding your solution, focusing on clarity and correctness first.
- Test and debug: Run your code with different test cases, including edge cases, and fix any bugs.
- Optimize: Look for ways to improve the time and space complexity of your solution.
4. Learn to Think Aloud
In technical interviews, your thought process is often as important as the final solution. Practice explaining your reasoning out loud as you solve problems. This skill, known as “thinking aloud,” helps interviewers understand your problem-solving approach and can even lead to hints or guidance during the interview.
5. Embrace the Growth Mindset
Adopting a growth mindset is crucial for continuous improvement. This means viewing challenges as opportunities to learn rather than insurmountable obstacles. When you encounter a difficult problem:
- Don’t get discouraged if you can’t solve it immediately
- Break it down into smaller parts and tackle each part separately
- Research and learn from others’ solutions after attempting the problem yourself
- Reflect on what you’ve learned from each challenge, regardless of whether you solved it or not
6. Simulate Interview Conditions
To prepare for the pressure of a real technical interview, try to simulate interview conditions during your practice sessions:
- Set a timer to mimic the time constraints of an actual interview
- Practice coding on a whiteboard or using a simple text editor instead of an IDE
- Ask a friend or mentor to conduct mock interviews with you
- Record yourself solving problems and explaining your thought process
7. Learn from Your Mistakes
Mistakes are an inevitable part of the learning process. Instead of getting discouraged, use them as learning opportunities:
- Keep a log of problems you struggled with and revisit them regularly
- Analyze why certain approaches didn’t work and what you can learn from them
- Look for patterns in the types of problems or concepts you find challenging
Advanced Techniques for Problem-Solving
1. Pattern Recognition
As you solve more problems, you’ll start to recognize common patterns. This skill can significantly speed up your problem-solving process. Some common patterns include:
- Two-pointer technique
- Sliding window
- Divide and conquer
- Breadth-first search (BFS) and Depth-first search (DFS)
- Dynamic programming
Practice identifying these patterns in different problems and understanding when to apply them.
2. Time and Space Complexity Analysis
Understanding the time and space complexity of your solutions is crucial. It demonstrates your ability to think about efficiency and scalability, which are highly valued in technical interviews. Practice analyzing the complexity of your solutions using Big O notation.
For example, consider this simple function to find the maximum element in an array:
def find_max(arr):
max_val = float('-inf')
for num in arr:
if num > max_val:
max_val = num
return max_val
The time complexity of this function is O(n), where n is the length of the array, because we iterate through each element once. The space complexity is O(1) as we only use a single variable to store the maximum value, regardless of the input size.
3. Optimization Techniques
Once you have a working solution, think about ways to optimize it. Common optimization techniques include:
- Using appropriate data structures (e.g., hash tables for fast lookups)
- Avoiding unnecessary computations
- Utilizing memoization in recursive solutions
- Applying mathematical properties or formulas to simplify the problem
4. Handling Edge Cases
A crucial aspect of problem-solving is considering and handling edge cases. These are special cases that might not be immediately obvious but can cause your solution to fail if not addressed. Common edge cases include:
- Empty input
- Input with a single element
- Very large or very small inputs
- Inputs with duplicate elements
- Negative numbers (when dealing with numeric inputs)
Always consider these cases when designing and testing your solutions.
Applying Your Problem-Solving Skills in Technical Interviews
Now that you’ve developed your problem-solving mindset, it’s important to know how to apply these skills effectively in a technical interview setting.
1. Clarify the Problem
Before diving into a solution, make sure you fully understand the problem. Ask clarifying questions such as:
- What are the input constraints?
- Are there any specific performance requirements?
- How should edge cases be handled?
This demonstrates your analytical thinking and ensures you’re solving the right problem.
2. Communicate Your Thought Process
As you work through the problem, explain your thinking out loud. This includes:
- Describing the approach you’re considering
- Explaining why you’re choosing certain data structures or algorithms
- Discussing potential trade-offs between different solutions
This gives the interviewer insight into your problem-solving process and allows them to provide guidance if needed.
3. Start with a Brute Force Solution
If you’re stuck, start by explaining a brute force solution. This shows that you can at least solve the problem, even if not optimally. Then, discuss how you might optimize this solution.
4. Write Clean, Readable Code
When implementing your solution, focus on writing clean, well-organized code. Use meaningful variable names, add comments where necessary, and structure your code logically. For example:
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
cleaned_s = ''.join(c.lower() for c in s if c.isalnum())
# Compare the string with its reverse
return cleaned_s == cleaned_s[::-1]
# Test the function
print(is_palindrome("A man, a plan, a canal: Panama")) # Should return True
print(is_palindrome("race a car")) # Should return False
5. Test Your Solution
After implementing your solution, walk through a few test cases to verify its correctness. Include:
- A normal case
- Edge cases (e.g., empty input, single element)
- Any specific cases mentioned in the problem statement
This demonstrates your attention to detail and thoroughness.
6. Analyze Time and Space Complexity
Conclude by discussing the time and space complexity of your solution. Be prepared to explain how you arrived at your analysis.
7. Be Open to Feedback
If the interviewer suggests improvements or alternative approaches, be receptive to their feedback. This shows your ability to learn and collaborate, which are valuable traits in any developer.
Conclusion
Developing a problem-solving mindset for technical interviews is a journey that requires consistent effort and practice. By following the strategies outlined in this article, you can enhance your analytical thinking, improve your coding skills, and approach technical interviews with confidence.
Remember, the goal is not just to solve the problem, but to demonstrate your thought process, communication skills, and ability to write clean, efficient code. With dedication and the right approach, you can master the art of technical interviews and open doors to exciting opportunities in the tech industry.
Keep practicing, stay curious, and embrace each challenge as an opportunity to grow. Your problem-solving mindset will not only serve you well in interviews but throughout your career as a software developer.