How to Use Mind Maps to Break Down Coding Challenges
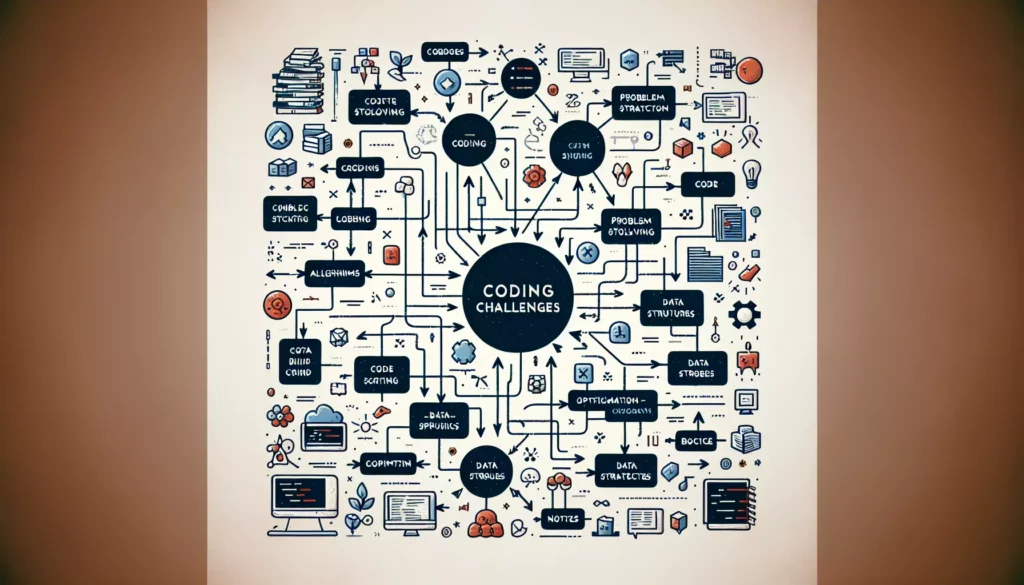
In the world of programming and software development, tackling complex coding challenges can often feel like navigating through a maze. As aspiring developers or even seasoned professionals, we frequently encounter problems that seem overwhelming at first glance. This is where the power of mind mapping comes into play. By utilizing mind maps, we can effectively break down coding challenges into manageable components, making the problem-solving process more structured and less daunting.
In this comprehensive guide, we’ll explore how to leverage mind maps to dissect coding challenges, enhance your problem-solving skills, and ultimately become a more efficient programmer. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, this technique can significantly boost your ability to tackle complex problems.
Understanding Mind Maps
Before we dive into the application of mind maps for coding challenges, let’s first understand what mind maps are and why they’re so effective.
What is a Mind Map?
A mind map is a visual diagram used to organize information and ideas around a central concept. It typically starts with a main idea in the center, with related concepts, subtopics, and details branching out from it. This radial structure allows for a natural flow of ideas and helps in visualizing connections between different elements of a problem or topic.
Why Are Mind Maps Effective?
Mind maps are particularly effective for several reasons:
- Visual Representation: They provide a clear, visual representation of complex ideas, making it easier to grasp the overall structure of a problem.
- Hierarchical Organization: Mind maps naturally organize information hierarchically, helping to break down complex problems into smaller, more manageable parts.
- Creativity Boost: The non-linear nature of mind maps encourages creative thinking and can lead to innovative solutions.
- Memory Enhancement: The visual and associative nature of mind maps can improve recall and understanding of complex concepts.
- Flexibility: They can be easily updated and expanded as new ideas or information come to light.
Applying Mind Maps to Coding Challenges
Now that we understand the basics of mind mapping, let’s explore how we can apply this technique to break down coding challenges effectively.
Step 1: Identify the Core Problem
The first step in using a mind map for a coding challenge is to identify the core problem or main objective. This will serve as the central node of your mind map. For example, if you’re tasked with creating a function to find the longest palindromic substring in a given string, your central node might be “Longest Palindromic Substring.”
Step 2: Break Down the Problem into Sub-problems
From the central node, start branching out to identify the main components or sub-problems of the challenge. For our palindromic substring example, these might include:
- Understanding what a palindrome is
- Identifying substrings
- Checking if a substring is a palindrome
- Finding the longest among palindromic substrings
Step 3: Explore Each Sub-problem
For each sub-problem or component identified in step 2, create new branches to explore them further. This is where you can start thinking about specific algorithms, data structures, or techniques that might be relevant. For instance, under “Checking if a substring is a palindrome,” you might add:
- Two-pointer technique
- String reversal comparison
- Recursive approach
Step 4: Consider Edge Cases and Constraints
As you develop your mind map, it’s crucial to consider edge cases and constraints. Create a branch specifically for these considerations. For our palindrome example, this might include:
- Empty string input
- Single character input
- String with all identical characters
- Very long input strings (performance considerations)
Step 5: Outline Potential Approaches
Based on your breakdown of the problem, start outlining potential approaches to solving the challenge. These can be high-level strategies or specific algorithms. For our example, we might have:
- Brute force approach (check all substrings)
- Dynamic programming solution
- Expand around center technique
- Manacher’s algorithm (for optimal time complexity)
Step 6: Evaluate and Choose an Approach
With your mind map now showing various aspects of the problem and potential solutions, you can more easily evaluate different approaches. Consider factors like time complexity, space complexity, and implementation difficulty. Create a branch to compare these aspects for each approach.
Step 7: Plan the Implementation
Once you’ve chosen an approach, use your mind map to plan the implementation. Break down the chosen algorithm into steps, consider necessary data structures, and outline the general structure of your code.
Example: Mind Mapping the Longest Palindromic Substring Problem
Let’s create a simple textual representation of how a mind map for the Longest Palindromic Substring problem might look:
Longest Palindromic Substring
|
+-- Understanding Palindromes
| |-- Definition
| +-- Examples
|
+-- Identifying Substrings
| |-- String slicing
| +-- Indexing
|
+-- Checking Palindromes
| |-- Two-pointer technique
| |-- String reversal
| +-- Recursive approach
|
+-- Finding the Longest
| |-- Keeping track of max length
| +-- Updating result string
|
+-- Edge Cases
| |-- Empty string
| |-- Single character
| |-- All identical characters
| +-- Very long strings
|
+-- Potential Approaches
| |-- Brute Force
| | |-- Time Complexity: O(n^3)
| | +-- Space Complexity: O(1)
| |
| |-- Dynamic Programming
| | |-- Time Complexity: O(n^2)
| | +-- Space Complexity: O(n^2)
| |
| |-- Expand Around Center
| | |-- Time Complexity: O(n^2)
| | +-- Space Complexity: O(1)
| |
| +-- Manacher's Algorithm
| |-- Time Complexity: O(n)
| +-- Space Complexity: O(n)
|
+-- Chosen Approach: Expand Around Center
|-- Implementation Steps
| |-- Function to expand palindrome
| |-- Iterate through string
| |-- Check odd and even length palindromes
| +-- Update longest found
|
+-- Code Structure
|-- Main function
+-- Helper function for expansion
This textual representation gives you an idea of how a mind map for this problem might be structured. In practice, you’d typically draw this out visually, allowing for a more intuitive and flexible representation.
Benefits of Using Mind Maps for Coding Challenges
Incorporating mind maps into your problem-solving toolkit for coding challenges offers numerous benefits:
1. Improved Problem Comprehension
By visually breaking down a problem, you gain a better understanding of its components and requirements. This comprehensive view helps ensure that you don’t overlook crucial aspects of the challenge.
2. Structured Approach to Problem-Solving
Mind maps provide a structured framework for approaching complex problems. This organization can be particularly helpful when dealing with multi-faceted coding challenges or during technical interviews where clear thinking is crucial.
3. Enhanced Creativity
The non-linear nature of mind maps can spark creative connections and lead to innovative solutions. By visualizing the problem in a different way, you might discover approaches that aren’t immediately obvious.
4. Better Time Management
With a clear breakdown of the problem and potential approaches, you can more effectively allocate your time during coding sessions or interviews. This structured view helps prevent getting stuck on one aspect of the problem for too long.
5. Effective Communication
Mind maps are excellent tools for communicating your thought process to others. In pair programming scenarios or when explaining your approach during an interview, a mind map can clearly illustrate your problem-solving strategy.
6. Comprehensive Documentation
Your mind map serves as a comprehensive document of your problem-solving process. This can be invaluable for future reference or when revisiting complex problems.
Tools for Creating Mind Maps
While you can certainly create mind maps with pen and paper, there are numerous digital tools available that can make the process more efficient and allow for easy editing and sharing. Here are some popular options:
1. XMind
XMind is a powerful mind mapping tool with a clean interface and a variety of templates. It’s available for desktop and mobile platforms.
2. MindMeister
MindMeister is a web-based mind mapping tool that allows for real-time collaboration, making it great for team problem-solving sessions.
3. Coggle
Coggle offers a simple, intuitive interface for creating colorful mind maps. It also supports real-time collaboration and easy sharing.
4. Freemind
Freemind is a free, open-source mind mapping tool. While its interface might not be as polished as some paid options, it offers robust functionality.
5. Microsoft Visio
For those already using Microsoft Office, Visio provides mind mapping capabilities along with other diagramming tools.
Integrating Mind Maps into Your Coding Workflow
To get the most out of mind mapping for coding challenges, consider integrating this technique into your regular workflow:
1. Start with a Mind Map
Before diving into coding, make it a habit to create a quick mind map of the problem. This initial step can save you time in the long run by ensuring you have a clear understanding of the challenge.
2. Use Mind Maps for Planning
Beyond just breaking down the problem, use mind maps to plan your implementation. Map out your classes, functions, and overall code structure.
3. Revisit and Refine
As you work on the problem, don’t hesitate to revisit and refine your mind map. Add new insights, remove unnecessary elements, and adjust your approach as needed.
4. Practice Regularly
Like any skill, creating effective mind maps for coding challenges improves with practice. Try to incorporate this technique into your regular problem-solving routine.
5. Share and Collaborate
If you’re working in a team or participating in pair programming, use mind maps as a collaboration tool. They can serve as an excellent focal point for discussions and brainstorming sessions.
Mind Mapping for Interview Preparation
For those preparing for technical interviews, especially at major tech companies, mind mapping can be an invaluable tool:
1. Categorize Problems
Create a master mind map categorizing different types of coding problems (e.g., arrays, strings, trees, graphs). This can help you identify areas where you need more practice.
2. Map Out Common Algorithms
Develop mind maps for common algorithms and data structures. This visual representation can aid in quick recall during high-pressure interview situations.
3. Practice Verbalization
Use your mind maps as a guide for verbalizing your problem-solving approach. This can help you articulate your thoughts more clearly during interviews.
4. Review and Reflect
After solving practice problems, create mind maps to summarize the problem, your approach, and any key learnings. This reflective practice can reinforce your understanding and help identify areas for improvement.
Conclusion
Mind mapping is a powerful technique that can significantly enhance your ability to break down and solve coding challenges. By providing a visual, structured approach to problem-solving, mind maps can help you tackle complex issues more effectively, whether you’re working on personal projects, collaborating with a team, or preparing for technical interviews.
Remember, the key to mastering this technique is practice. Start incorporating mind maps into your coding workflow, and you’ll likely find that they not only improve your problem-solving skills but also enhance your overall understanding of complex programming concepts.
As you continue your journey in coding education and skills development, consider mind mapping as another valuable tool in your programming toolkit. It’s a versatile technique that can adapt to various aspects of software development, from initial problem analysis to algorithm design and even project planning.
By harnessing the power of visual thinking through mind maps, you’re equipping yourself with a method that can help you navigate the complexities of coding challenges more efficiently and creatively. So, the next time you’re faced with a daunting programming problem, reach for a pen and paper (or your favorite digital tool) and start mapping your way to a solution!