Mastering Sliding Window Problems for Interview Success
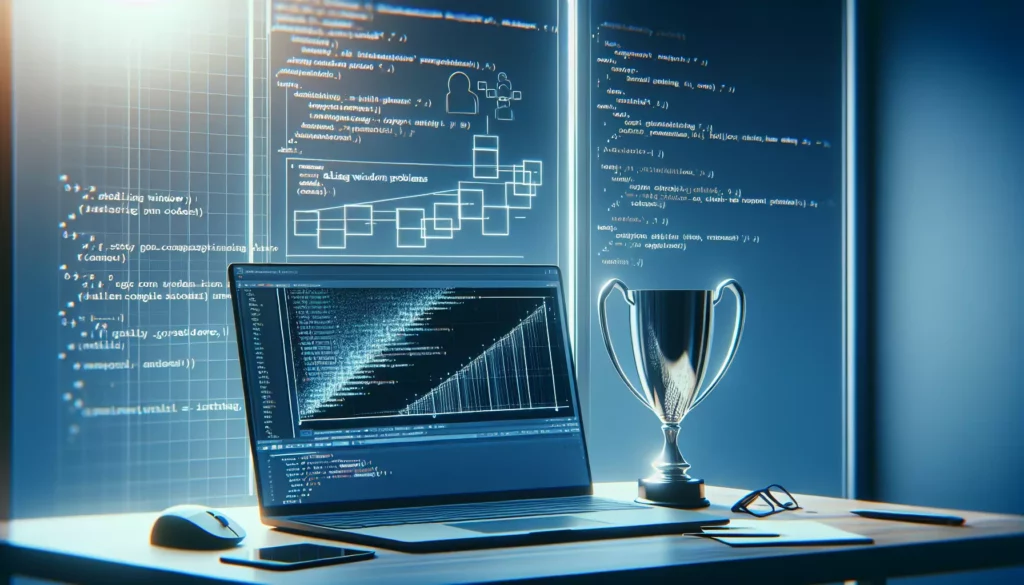
In the competitive world of technical interviews, particularly for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering various algorithmic techniques is crucial. One such technique that frequently appears in coding interviews is the Sliding Window approach. This powerful method is especially useful for solving array or string problems with contiguous elements. In this comprehensive guide, we’ll dive deep into the Sliding Window technique, understand its core concepts, and work through multiple examples to help you ace your next technical interview.
What is the Sliding Window Technique?
The Sliding Window technique is an algorithmic paradigm that involves maintaining a subset of elements (the “window”) as you iterate through a larger collection of data, typically an array or string. This window can grow, shrink, or slide as you process the elements, allowing you to solve certain problems with improved time complexity.
The key idea behind this technique is to avoid redundant computations by reusing the result from the previous step. This approach is particularly effective for problems that involve finding subarrays or substrings that satisfy certain conditions.
When to Use the Sliding Window Technique
The Sliding Window technique is most applicable in scenarios where you need to perform operations on a contiguous sequence of elements. Some common problem patterns include:
- Finding the longest/shortest substring with certain properties
- Calculating a running average or sum over a specific window size
- Finding the maximum/minimum sum of any contiguous subarray of size k
- Detecting patterns or anagrams in a string
Types of Sliding Window Problems
Sliding Window problems can generally be categorized into two types:
1. Fixed Window Size
In these problems, the size of the window remains constant throughout the iteration. You typically slide this fixed-size window across the array or string, performing calculations or checks at each step.
2. Variable Window Size
These problems involve adjusting the window size dynamically based on certain conditions. The window can grow or shrink as you iterate through the data, often to find the longest or shortest subarray that satisfies given criteria.
Basic Template for Sliding Window Problems
While the specific implementation details may vary depending on the problem, here’s a general template for approaching Sliding Window problems:
function slidingWindow(arr) {
let windowStart = 0;
let result = 0; // or any other appropriate initial value
for (let windowEnd = 0; windowEnd < arr.length; windowEnd++) {
// Expand the window by including the element at windowEnd
// Update any necessary variables or calculations
// If the window condition is violated:
while (windowViolatesCondition()) {
// Remove elements from the start of the window
// Update any necessary variables or calculations
windowStart++;
}
// Update the result if necessary
}
return result;
}
This template provides a starting point for many Sliding Window problems. Let’s now look at some specific examples to see how this technique can be applied in practice.
Example 1: Maximum Sum Subarray of Size K (Fixed Window)
Problem: Given an array of integers and a positive integer k, find the maximum sum of any contiguous subarray of size k.
This is a classic fixed-size window problem. We’ll maintain a window of size k and slide it across the array, keeping track of the maximum sum encountered.
function maxSubarraySum(arr, k) {
if (arr.length < k) {
return null; // Invalid input
}
let maxSum = 0;
let windowSum = 0;
// Calculate sum of first window
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window and update maxSum
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
// Example usage
const arr = [1, 4, 2, 10, 23, 3, 1, 0, 20];
const k = 4;
console.log(maxSubarraySum(arr, k)); // Output: 39
Time Complexity: O(n), where n is the length of the array.
Space Complexity: O(1), as we’re using only a constant amount of extra space.
Example 2: Longest Substring with K Distinct Characters (Variable Window)
Problem: Given a string and an integer k, find the length of the longest substring that contains at most k distinct characters.
This problem requires a variable-size window, as we need to adjust the window size based on the number of distinct characters it contains.
function longestSubstringKDistinct(s, k) {
let windowStart = 0;
let maxLength = 0;
let charFrequency = {};
for (let windowEnd = 0; windowEnd < s.length; windowEnd++) {
const rightChar = s[windowEnd];
if (!(rightChar in charFrequency)) {
charFrequency[rightChar] = 0;
}
charFrequency[rightChar]++;
// Shrink the window if we have more than k distinct characters
while (Object.keys(charFrequency).length > k) {
const leftChar = s[windowStart];
charFrequency[leftChar]--;
if (charFrequency[leftChar] === 0) {
delete charFrequency[leftChar];
}
windowStart++;
}
maxLength = Math.max(maxLength, windowEnd - windowStart + 1);
}
return maxLength;
}
// Example usage
const s = "araaci";
const k = 2;
console.log(longestSubstringKDistinct(s, k)); // Output: 4
Time Complexity: O(n), where n is the length of the string.
Space Complexity: O(k), as the character frequency map will contain at most k+1 characters.
Example 3: Minimum Window Substring (Variable Window)
Problem: Given two strings s and t, return the minimum window in s which will contain all the characters in t. If there is no such window in s that covers all characters in t, return the empty string “”.
This is a more complex variable window problem that requires careful tracking of character frequencies.
function minWindow(s, t) {
let charFrequency = {};
for (let char of t) {
if (!(char in charFrequency)) {
charFrequency[char] = 0;
}
charFrequency[char]++;
}
let windowStart = 0;
let matched = 0;
let minLength = Infinity;
let substrStart = 0;
for (let windowEnd = 0; windowEnd < s.length; windowEnd++) {
let rightChar = s[windowEnd];
if (rightChar in charFrequency) {
charFrequency[rightChar]--;
if (charFrequency[rightChar] === 0) {
matched++;
}
}
while (matched === Object.keys(charFrequency).length) {
if (windowEnd - windowStart + 1 < minLength) {
minLength = windowEnd - windowStart + 1;
substrStart = windowStart;
}
let leftChar = s[windowStart];
windowStart++;
if (leftChar in charFrequency) {
if (charFrequency[leftChar] === 0) {
matched--;
}
charFrequency[leftChar]++;
}
}
}
return minLength === Infinity ? "" : s.substr(substrStart, minLength);
}
// Example usage
const s = "ADOBECODEBANC";
const t = "ABC";
console.log(minWindow(s, t)); // Output: "BANC"
Time Complexity: O(n + m), where n is the length of s and m is the length of t.
Space Complexity: O(k), where k is the number of distinct characters in t.
Tips for Solving Sliding Window Problems
- Identify the window: Determine what constitutes your window. Is it a fixed number of elements, or does it vary based on certain conditions?
- Choose appropriate data structures: Depending on the problem, you might need to use additional data structures like hash maps to keep track of frequencies or other properties.
- Handle edge cases: Always consider edge cases, such as empty input or when the window size is larger than the input array/string.
- Optimize as you go: Look for opportunities to optimize your solution. Can you remove unnecessary operations or reduce space complexity?
- Practice, practice, practice: The more problems you solve, the better you’ll become at recognizing and applying the Sliding Window technique.
Common Pitfalls and How to Avoid Them
- Incorrect window updates: Make sure you’re updating your window correctly, especially when shrinking it. Double-check that you’re removing the correct elements and updating any associated counters or data structures.
- Off-by-one errors: Be careful with your loop conditions and index calculations. It’s easy to make off-by-one errors when dealing with windows and subarrays.
- Inefficient inner loops: If you find yourself using nested loops that iterate over the entire array/string, you’re probably not implementing the Sliding Window technique correctly. Look for ways to optimize your inner loop to only process necessary elements.
- Overlooking optimization opportunities: Sometimes, you can further optimize your solution by precalculating certain values or using more efficient data structures. Always look for ways to improve your initial solution.
Advanced Sliding Window Techniques
As you become more comfortable with basic Sliding Window problems, you can explore more advanced techniques and variations:
1. Two Pointers with Sliding Window
Some problems may require you to use two pointers in conjunction with the Sliding Window technique. This can be useful when you need to compare elements at different positions within the window.
2. Sliding Window with Auxiliary Data Structures
For more complex problems, you might need to use additional data structures like deques (double-ended queues) or balanced binary search trees to maintain information about your window efficiently.
3. Multi-dimensional Sliding Window
While less common, some problems may require you to apply the Sliding Window technique to 2D arrays or matrices. These problems often involve maintaining a rectangular or square window that slides across the matrix.
Practice Problems
To further hone your skills with the Sliding Window technique, here are some additional problems you can practice:
- Longest Substring Without Repeating Characters (LeetCode #3)
- Fruit Into Baskets (LeetCode #904)
- Permutation in String (LeetCode #567)
- Find All Anagrams in a String (LeetCode #438)
- Sliding Window Maximum (LeetCode #239)
- Longest Repeating Character Replacement (LeetCode #424)
- Max Consecutive Ones III (LeetCode #1004)
Conclusion
The Sliding Window technique is a powerful tool in a programmer’s arsenal, particularly useful for solving array and string problems efficiently. By mastering this technique, you’ll be well-equipped to tackle a wide range of coding interview questions, especially those asked by top tech companies.
Remember, the key to success in technical interviews is not just knowing the techniques, but also practicing regularly and learning to recognize when and how to apply them. As you work through more problems, you’ll develop an intuition for identifying Sliding Window opportunities and implementing efficient solutions.
Keep practicing, stay curious, and don’t be afraid to tackle challenging problems. With dedication and consistent effort, you’ll be well on your way to mastering the Sliding Window technique and improving your overall problem-solving skills. Good luck with your interview preparation!