The Importance of Understanding Mathematical Induction for Algorithmic Thinking
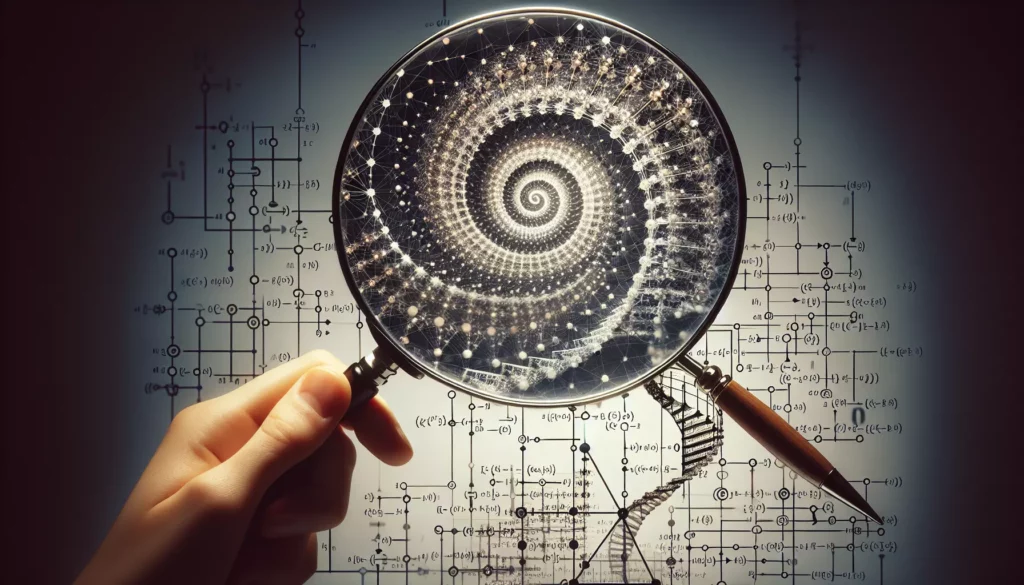
In the realm of computer science and programming, algorithmic thinking is a fundamental skill that separates proficient developers from the rest. As we delve deeper into the world of coding education and skills development, one concept stands out as particularly crucial: mathematical induction. This powerful tool not only enhances our problem-solving abilities but also plays a vital role in algorithm design and verification. In this comprehensive guide, we’ll explore the significance of mathematical induction in algorithmic thinking and how it can elevate your coding prowess to new heights.
What is Mathematical Induction?
Before we dive into its applications in algorithmic thinking, let’s first understand what mathematical induction is. Mathematical induction is a method of mathematical proof typically used to establish that a given statement is true for all natural numbers. It consists of two main steps:
- Base Case: Prove that the statement holds for the smallest natural number in the set (usually n = 1 or n = 0).
- Inductive Step: Assume the statement holds for an arbitrary natural number k, then prove that it also holds for the next natural number, k + 1.
If both these steps are proven, then by the principle of mathematical induction, the statement is true for all natural numbers.
The Connection Between Mathematical Induction and Algorithmic Thinking
At first glance, mathematical induction might seem like a purely theoretical concept with little practical application in coding. However, its principles are deeply intertwined with algorithmic thinking in several ways:
1. Recursive Algorithm Design
Recursive algorithms are a perfect example of how mathematical induction manifests in programming. When designing a recursive algorithm, we typically follow a structure similar to mathematical induction:
- Base case: Define the simplest scenario where the algorithm should terminate.
- Recursive step: Break down the problem into smaller subproblems and call the function recursively.
This structure mirrors the base case and inductive step in mathematical induction. Understanding induction can help you design more efficient and correct recursive algorithms.
2. Algorithm Correctness Proofs
Proving the correctness of an algorithm is crucial, especially in critical systems where errors can have severe consequences. Mathematical induction provides a robust framework for proving algorithm correctness, particularly for algorithms that work with sequences or iterate over natural numbers.
3. Loop Invariant Analysis
Loop invariants are conditions that remain true before, during, and after each iteration of a loop. Analyzing loop invariants is a powerful technique for understanding and verifying the correctness of iterative algorithms. The process of proving loop invariants often involves inductive reasoning, making mathematical induction an invaluable tool in this analysis.
4. Complexity Analysis
When analyzing the time and space complexity of algorithms, especially those with recursive structures, mathematical induction can be used to prove the correctness of our complexity calculations. This is particularly useful when dealing with recurrence relations in divide-and-conquer algorithms.
Practical Applications of Mathematical Induction in Algorithmic Thinking
Now that we understand the theoretical connections, let’s explore some practical applications of mathematical induction in algorithmic thinking and coding:
1. Proving the Correctness of a Recursive Factorial Function
Consider a recursive implementation of the factorial function:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
We can use mathematical induction to prove that this function correctly calculates n! for all non-negative integers n.
Base case (n = 0): factorial(0) returns 1, which is correct.
Inductive step: Assume factorial(k) correctly calculates k! for some k ≥ 0. We need to prove that factorial(k + 1) correctly calculates (k + 1)!.
factorial(k + 1) = (k + 1) * factorial(k)
= (k + 1) * k! (by inductive hypothesis)
= (k + 1)!
Therefore, by mathematical induction, the function correctly calculates n! for all non-negative integers n.
2. Analyzing the Time Complexity of the Fibonacci Sequence
Let’s consider a naive recursive implementation of the Fibonacci sequence:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
We can use mathematical induction to prove that the time complexity of this implementation is O(2^n).
Base cases:
For n = 0 or n = 1, the function makes 1 call, which is less than 2^n.
Inductive step:
Assume that for all k < n, fibonacci(k) makes at most 2^k function calls.
For fibonacci(n), we have:
T(n) = T(n-1) + T(n-2) + 1 (1 for the addition operation)
≤ 2^(n-1) + 2^(n-2) + 1 (by inductive hypothesis)
< 2^(n-1) + 2^(n-1) + 2^(n-1) (since 2^(n-2) + 1 < 2^(n-1) for n > 2)
= 3 * 2^(n-1)
< 4 * 2^(n-1) = 2^n
Therefore, by mathematical induction, the time complexity is O(2^n).
3. Proving the Correctness of a Divide-and-Conquer Algorithm
Let’s consider the merge sort algorithm, a classic example of the divide-and-conquer paradigm. We can use mathematical induction to prove its correctness.
Claim: Merge sort correctly sorts an array of n elements.
Base case: For n = 1, an array with one element is already sorted.
Inductive step: Assume merge sort correctly sorts arrays of size k < n. We need to prove it correctly sorts arrays of size n.
For an array of size n, merge sort:
1. Divides the array into two subarrays of size n/2 (or (n-1)/2 and (n+1)/2 if n is odd).
2. Recursively sorts these subarrays.
3. Merges the sorted subarrays.
By the inductive hypothesis, the subarrays are correctly sorted. If we can prove that the merge step correctly combines two sorted arrays, then we’ve proven the correctness of merge sort for size n.
The merge step can be proven correct using a loop invariant: At the start of each iteration, the output array contains the smallest k elements from the input arrays in sorted order.
Therefore, by mathematical induction, merge sort correctly sorts arrays of any size n.
Enhancing Algorithmic Thinking with Mathematical Induction
Now that we’ve seen the practical applications of mathematical induction in algorithmic thinking, let’s explore how we can use this knowledge to enhance our problem-solving skills:
1. Developing a Systematic Approach to Problem Solving
Mathematical induction encourages a systematic approach to problem-solving. When faced with a new algorithmic challenge, consider if it can be broken down into smaller subproblems. This mindset naturally leads to more efficient divide-and-conquer or dynamic programming solutions.
2. Improving Code Verification Skills
By applying inductive reasoning to your code, you can develop a more rigorous approach to verifying its correctness. This is particularly valuable when preparing for technical interviews or working on critical systems where code reliability is paramount.
3. Enhancing Algorithm Analysis Abilities
A solid understanding of mathematical induction will significantly improve your ability to analyze algorithm complexity. This skill is crucial for optimizing code performance and is often a key focus in technical interviews at major tech companies.
4. Strengthening Recursive Thinking
The parallels between mathematical induction and recursive algorithms can help reinforce your understanding of recursion. This can lead to more elegant and efficient solutions to problems that naturally lend themselves to recursive approaches.
Practical Exercises to Reinforce Mathematical Induction in Coding
To truly internalize the concepts of mathematical induction and apply them to algorithmic thinking, practice is key. Here are some exercises you can try:
1. Prove the Correctness of a Binary Search Algorithm
Implement a binary search algorithm and use mathematical induction to prove its correctness. Consider how the search space is reduced in each recursive call and how this relates to the inductive step.
2. Analyze the Time Complexity of the Tower of Hanoi Problem
Implement a recursive solution to the Tower of Hanoi problem. Use mathematical induction to prove that the number of moves required for n disks is 2^n – 1.
3. Implement and Analyze a Recursive Solution to the Coin Change Problem
Develop a recursive solution to the coin change problem (finding the minimum number of coins needed to make a certain amount). Use mathematical induction to analyze its time complexity and consider how dynamic programming can improve it.
4. Prove the Correctness of a Recursive Palindrome Checker
Implement a recursive function to check if a string is a palindrome. Use induction to prove its correctness for strings of any length.
Conclusion: The Power of Mathematical Induction in Coding
Understanding and applying mathematical induction is a game-changer for aspiring programmers and seasoned developers alike. It provides a robust framework for designing, analyzing, and verifying algorithms, which are essential skills for anyone looking to excel in coding interviews or build efficient, reliable software systems.
By incorporating mathematical induction into your algorithmic thinking toolkit, you’ll find yourself better equipped to tackle complex problems, optimize solutions, and communicate your reasoning effectively. This skill will not only make you a more proficient coder but also give you a significant edge in technical interviews at top tech companies.
Remember, like any skill, mastering the application of mathematical induction in coding takes practice. Continuously challenge yourself with algorithmic problems, always considering how inductive reasoning can be applied to solve, prove, or analyze your solutions. With time and dedication, you’ll find that this powerful mathematical concept becomes an integral part of your problem-solving approach, elevating your coding skills to new heights.
As you continue your journey in coding education and skills development, keep mathematical induction at the forefront of your algorithmic thinking. It’s not just a theoretical concept – it’s a practical tool that will serve you well throughout your programming career, from tackling challenging leetcode problems to designing robust, scalable systems in the real world.