How to Approach Problems with Multiple Constraints: A Comprehensive Guide
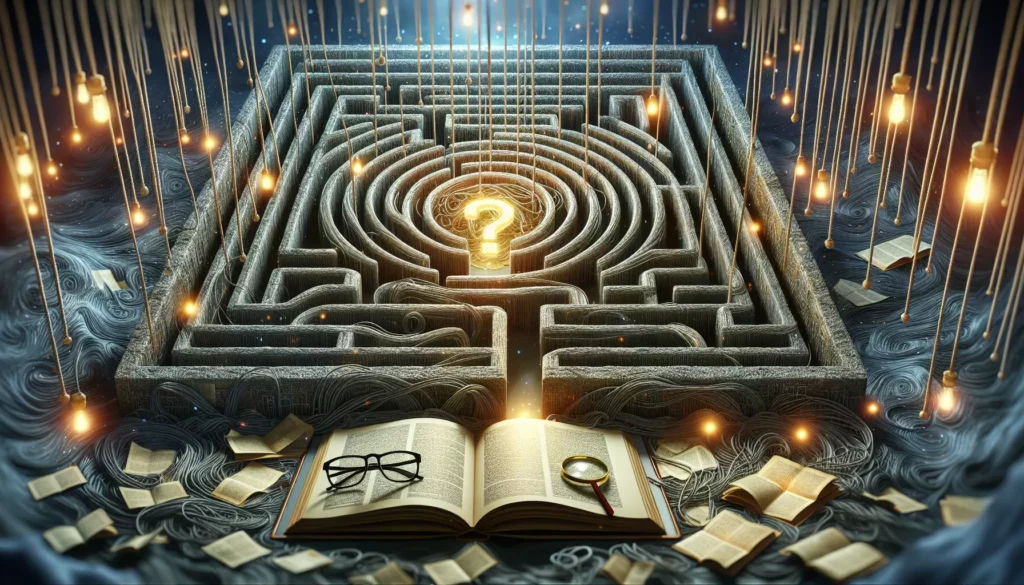
In the world of programming and algorithm design, problems with multiple constraints are a common challenge that developers face. These types of problems require a careful balance of various requirements and limitations, often making them more complex and demanding than single-constraint problems. In this comprehensive guide, we’ll explore effective strategies for approaching and solving problems with multiple constraints, providing you with the tools and techniques to tackle even the most challenging coding scenarios.
Understanding Problems with Multiple Constraints
Before diving into the strategies for solving these problems, it’s essential to understand what we mean by “problems with multiple constraints.” In programming, a constraint is a condition or limitation that must be satisfied for a solution to be considered valid. When a problem has multiple constraints, it means that the solution must simultaneously meet several different conditions or requirements.
For example, consider a scheduling problem where you need to assign tasks to workers. The constraints might include:
- Each worker can only work a maximum of 8 hours per day
- Certain tasks must be completed before others can begin
- Some tasks require specific skills that only certain workers possess
- The entire project must be completed within a given timeframe
In this scenario, any valid solution must satisfy all of these constraints simultaneously, making the problem more challenging to solve than if it had only one or two constraints.
Strategies for Approaching Multi-Constraint Problems
1. Break Down the Problem
The first step in tackling a problem with multiple constraints is to break it down into smaller, more manageable parts. This approach, often referred to as “divide and conquer,” allows you to focus on individual aspects of the problem before combining them into a comprehensive solution.
To break down a multi-constraint problem:
- Identify each constraint separately
- Analyze how each constraint affects the problem space
- Consider how the constraints interact with each other
- Look for any sub-problems that can be solved independently
By breaking down the problem, you can gain a clearer understanding of its components and potentially identify simpler solutions for individual parts.
2. Prioritize Constraints
Not all constraints are created equal. Some may have a more significant impact on the solution than others, or some may be easier to satisfy. Prioritizing constraints can help you focus your efforts and potentially simplify the problem-solving process.
To prioritize constraints:
- Assess the importance of each constraint to the overall problem
- Identify any “hard” constraints that must be satisfied versus “soft” constraints that are desirable but not essential
- Consider which constraints have the most significant impact on the solution space
- Determine if any constraints can be relaxed or approximated without significantly affecting the solution quality
By prioritizing constraints, you can focus on satisfying the most critical requirements first and then work on optimizing the solution to meet less critical constraints.
3. Use Constraint Propagation
Constraint propagation is a technique used to reduce the search space by applying known constraints to eliminate invalid solutions early in the problem-solving process. This approach can be particularly effective when dealing with problems that have a large number of possible solutions.
To apply constraint propagation:
- Start with the most restrictive constraints
- Apply these constraints to eliminate invalid solutions
- Use the results to further restrict the search space for remaining constraints
- Repeat the process until no further reductions can be made
Constraint propagation can significantly reduce the complexity of the problem by narrowing down the set of possible solutions before applying more computationally expensive techniques.
4. Employ Heuristics and Approximations
For some complex problems with multiple constraints, finding an optimal solution may be computationally infeasible. In these cases, using heuristics or approximation algorithms can help find a “good enough” solution in a reasonable amount of time.
Strategies for using heuristics and approximations include:
- Greedy algorithms: Make locally optimal choices at each step
- Local search: Start with a feasible solution and iteratively improve it
- Genetic algorithms: Use evolutionary principles to generate and refine solutions
- Simulated annealing: Gradually decrease the probability of accepting worse solutions as the algorithm progresses
While these approaches may not guarantee an optimal solution, they can often provide satisfactory results for complex multi-constraint problems.
5. Utilize Mathematical Programming
For problems that can be formulated mathematically, techniques from the field of mathematical programming can be powerful tools for solving multi-constraint problems. These methods include:
- Linear programming: Optimizing a linear objective function subject to linear constraints
- Integer programming: Similar to linear programming, but with the added constraint that some or all variables must be integers
- Constraint programming: A paradigm for solving combinatorial problems by expressing them as a set of constraints
While these techniques can be more complex to implement, they often provide optimal or near-optimal solutions for well-defined problems with multiple constraints.
Implementing Solutions for Multi-Constraint Problems
Once you’ve chosen an approach for tackling a problem with multiple constraints, the next step is to implement your solution. Here are some best practices to keep in mind during the implementation phase:
1. Choose the Right Data Structures
Selecting appropriate data structures is crucial for efficiently representing and manipulating the problem space. Consider the following factors when choosing data structures:
- The nature of the constraints and how they relate to each other
- The frequency of operations (e.g., insertions, deletions, lookups) required by your algorithm
- The size of the problem and memory constraints
- The need for dynamic resizing or fixed-size structures
Common data structures that can be useful for multi-constraint problems include:
- Arrays and matrices for representing grids or tabular data
- Hash tables for fast lookups and constraint checking
- Graphs for representing relationships and dependencies
- Priority queues for maintaining ordered sets of elements
2. Implement Efficient Algorithms
The efficiency of your implementation can have a significant impact on the ability to solve large or complex multi-constraint problems. Consider the following techniques to improve algorithm efficiency:
- Use dynamic programming to avoid redundant computations
- Implement memoization to cache intermediate results
- Utilize efficient sorting and searching algorithms when necessary
- Consider parallel or distributed computing for large-scale problems
Here’s an example of how dynamic programming can be used to solve a multi-constraint problem efficiently:
def knapsack(values, weights, capacity):
n = len(values)
dp = [[0 for _ in range(capacity + 1)] for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(dp[i-1][w], dp[i-1][w-weights[i-1]] + values[i-1])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
# Example usage
values = [60, 100, 120]
weights = [10, 20, 30]
capacity = 50
max_value = knapsack(values, weights, capacity)
print(f"Maximum value: {max_value}")
This implementation of the 0/1 Knapsack problem uses dynamic programming to efficiently solve a problem with multiple constraints (item weights and knapsack capacity).
3. Handle Edge Cases and Constraint Violations
When implementing solutions for multi-constraint problems, it’s crucial to consider edge cases and handle constraint violations gracefully. Some strategies for dealing with these situations include:
- Implement robust input validation to catch invalid inputs early
- Use exception handling to manage unexpected scenarios
- Provide meaningful error messages or feedback when constraints cannot be satisfied
- Consider implementing “soft” constraint handling, where minor violations are allowed but penalized
Here’s an example of how you might implement constraint checking and error handling in Python:
class SchedulingError(Exception):
pass
def assign_task(task, worker, schedule):
# Check worker availability constraint
if worker.daily_hours + task.duration > 8:
raise SchedulingError(f"Worker {worker.name} cannot exceed 8 hours per day")
# Check skill requirement constraint
if task.required_skill not in worker.skills:
raise SchedulingError(f"Worker {worker.name} lacks the required skill for task {task.name}")
# Check task dependency constraint
if task.dependencies:
for dep in task.dependencies:
if dep not in schedule.completed_tasks:
raise SchedulingError(f"Dependency {dep} not completed for task {task.name}")
# If all constraints are satisfied, assign the task
schedule.assign(task, worker)
# Example usage
try:
assign_task(task1, worker1, project_schedule)
except SchedulingError as e:
print(f"Error assigning task: {e}")
This example demonstrates how to check multiple constraints and raise specific errors when constraints are violated, allowing for more robust error handling in the calling code.
4. Optimize and Refine Your Solution
Once you have a working implementation, it’s often beneficial to optimize and refine your solution. This process may involve:
- Profiling your code to identify performance bottlenecks
- Refactoring to improve code readability and maintainability
- Experimenting with different algorithms or heuristics to improve solution quality
- Considering trade-offs between solution quality and computational efficiency
Remember that optimization is an iterative process, and it’s important to balance the time spent optimizing with the actual benefits gained.
Testing and Validating Multi-Constraint Solutions
Thorough testing is crucial when working with problems that have multiple constraints. Here are some strategies for effective testing and validation:
1. Develop Comprehensive Test Cases
Create a diverse set of test cases that cover various scenarios, including:
- Edge cases that test the boundaries of each constraint
- Combinations of constraints that interact in complex ways
- Large-scale problems to test performance and scalability
- Random input generation to discover unexpected issues
2. Implement Automated Testing
Use automated testing frameworks to streamline the testing process and ensure consistent validation of your solution. This may include:
- Unit tests for individual components of your solution
- Integration tests to verify the interaction between different parts of your implementation
- Performance tests to measure execution time and resource usage
Here’s an example of how you might set up unit tests for a multi-constraint problem using Python’s unittest framework:
import unittest
class TestKnapsackSolver(unittest.TestCase):
def test_basic_case(self):
values = [60, 100, 120]
weights = [10, 20, 30]
capacity = 50
expected = 220
self.assertEqual(knapsack(values, weights, capacity), expected)
def test_no_items_fit(self):
values = [60, 100, 120]
weights = [51, 52, 53]
capacity = 50
expected = 0
self.assertEqual(knapsack(values, weights, capacity), expected)
def test_all_items_fit(self):
values = [60, 100, 120]
weights = [10, 20, 30]
capacity = 60
expected = 280
self.assertEqual(knapsack(values, weights, capacity), expected)
def test_large_input(self):
values = [i for i in range(1000)]
weights = [i for i in range(1000)]
capacity = 10000
result = knapsack(values, weights, capacity)
self.assertGreater(result, 0) # Ensure we get a non-zero result
self.assertLess(result, sum(values)) # Ensure we don't exceed total value
if __name__ == '__main__':
unittest.main()
This test suite covers various scenarios for the Knapsack problem, including basic cases, edge cases, and a large input test.
3. Validate Constraint Satisfaction
Implement checks to ensure that all constraints are satisfied by your solution. This may involve:
- Creating validation functions for each constraint
- Implementing a comprehensive solution checker
- Using assertion statements to catch constraint violations during execution
4. Benchmark Against Known Solutions
For well-known problems or those with established benchmarks, compare your solution against existing implementations or known optimal results. This can help you gauge the effectiveness of your approach and identify areas for improvement.
Conclusion
Approaching problems with multiple constraints requires a combination of analytical thinking, strategic problem-solving, and careful implementation. By breaking down the problem, prioritizing constraints, and employing appropriate techniques such as constraint propagation and heuristics, you can develop effective solutions to even the most complex multi-constraint problems.
Remember that the key to success lies in understanding the problem thoroughly, choosing the right approach, implementing efficiently, and rigorously testing your solution. With practice and experience, you’ll become more adept at recognizing patterns in multi-constraint problems and selecting the most appropriate strategies for solving them.
As you continue to develop your skills in tackling these challenging problems, you’ll find that the techniques and insights gained are valuable not only in coding interviews and competitive programming but also in real-world software development and algorithm design. The ability to approach and solve problems with multiple constraints is a crucial skill that will serve you well throughout your programming career.