Solving Hard Problems with Heuristics: A Beginner’s Guide
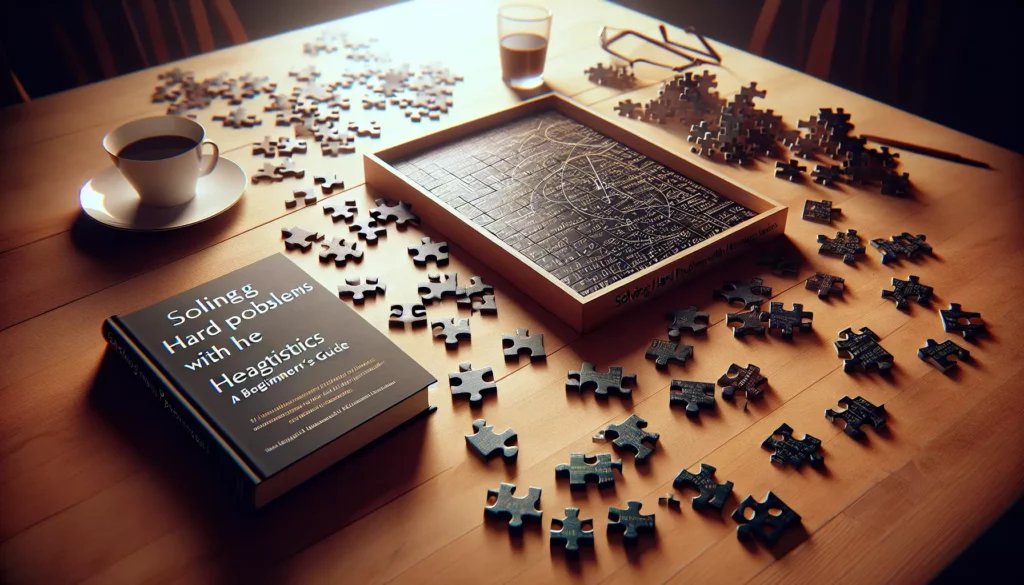
In the world of computer science and algorithmic problem-solving, we often encounter challenges that seem insurmountable at first glance. These hard problems can be frustrating, especially for beginners who are just starting their journey into the realm of coding and algorithms. However, there’s a powerful tool in our problem-solving arsenal that can help us tackle even the most daunting tasks: heuristics. In this comprehensive guide, we’ll explore what heuristics are, why they’re important, and how you can use them to solve hard problems in your coding journey.
What Are Heuristics?
Before we dive into the practical applications of heuristics, let’s start with a clear definition. A heuristic is a problem-solving approach that uses a practical method or various shortcuts to produce solutions that may not be optimal but are sufficient for reaching an immediate, short-term goal. In simpler terms, heuristics are “rules of thumb” or educated guesses that help us find good-enough solutions quickly, especially when perfect solutions are impossible or impractical to find.
In the context of computer science and algorithmic problem-solving, heuristics are particularly useful when dealing with:
- NP-hard problems (problems for which no known polynomial-time algorithm exists)
- Large-scale optimization problems
- Real-time decision-making scenarios
- Situations where approximate solutions are acceptable
Why Are Heuristics Important?
As aspiring programmers and problem-solvers, understanding and utilizing heuristics is crucial for several reasons:
- Efficiency: Heuristics often provide faster solutions compared to exhaustive search methods, making them invaluable in time-sensitive situations.
- Practicality: For many real-world problems, finding the absolute best solution is either impossible or too time-consuming. Heuristics offer practical, good-enough solutions.
- Complexity Management: Heuristics can simplify complex problems by breaking them down into more manageable parts or by focusing on the most important aspects.
- Inspiration for Better Algorithms: Many advanced algorithms are inspired by or built upon heuristic approaches.
- Applicability: Heuristics are used across various domains, from artificial intelligence and machine learning to operations research and software engineering.
Common Types of Heuristics
Before we look at how to apply heuristics to solve hard problems, let’s familiarize ourselves with some common types of heuristics used in computer science:
1. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, hoping to find a global optimum. While they don’t always produce the best solution, they’re often simple to implement and can provide good approximations.
2. Hill Climbing
This heuristic starts with an arbitrary solution and iteratively makes small changes to improve it. It’s like climbing a hill, always moving upward until you reach a peak (which may or may not be the highest peak).
3. A* Search
A* is a popular pathfinding algorithm that uses heuristics to guide its search. It combines the cost to reach a node and an estimate of the cost to get from that node to the goal.
4. Genetic Algorithms
Inspired by natural selection, genetic algorithms evolve a population of potential solutions over time, combining and mutating them to find better solutions.
5. Simulated Annealing
This heuristic is inspired by the annealing process in metallurgy. It starts with a high “temperature” (allowing big jumps in the solution space) and gradually “cools down” (restricting to smaller, more refined changes).
How to Apply Heuristics to Solve Hard Problems
Now that we understand what heuristics are and why they’re important, let’s walk through a step-by-step process of applying heuristics to solve hard problems:
Step 1: Understand the Problem
Before you can apply any heuristic, you need a clear understanding of the problem you’re trying to solve. Ask yourself:
- What are the inputs and desired outputs?
- What are the constraints?
- Is an approximate solution acceptable, or do you need an exact answer?
- What makes this problem hard? Is it the size of the input, the complexity of the relationships, or something else?
Step 2: Identify Potential Heuristics
Based on your understanding of the problem, consider which heuristics might be applicable. For example:
- If the problem involves finding an optimal path or sequence, a greedy algorithm or A* search might be appropriate.
- If you’re dealing with a large search space and need to find a good solution quickly, hill climbing or simulated annealing could be useful.
- If the problem involves optimizing multiple parameters simultaneously, genetic algorithms might be a good fit.
Step 3: Design Your Heuristic Approach
Once you’ve identified potential heuristics, design your approach. This involves:
- Defining how you’ll represent solutions
- Determining how you’ll evaluate the quality of a solution
- Deciding how you’ll generate new solutions or modify existing ones
- Setting any necessary parameters (e.g., population size for genetic algorithms, cooling rate for simulated annealing)
Step 4: Implement Your Heuristic
Translate your heuristic approach into code. Here’s a simple example of a hill climbing heuristic in Python for finding the maximum value of a function:
def objective_function(x):
return -(x ** 2) + 5 * x + 10 # Example function to maximize
def hill_climbing(start_x, step_size=0.1, max_iterations=1000):
current_x = start_x
current_value = objective_function(current_x)
for _ in range(max_iterations):
# Try moving left and right
left_x = current_x - step_size
right_x = current_x + step_size
left_value = objective_function(left_x)
right_value = objective_function(right_x)
# Move to the better position
if left_value > current_value and left_value > right_value:
current_x = left_x
current_value = left_value
elif right_value > current_value:
current_x = right_x
current_value = right_value
else:
# If no improvement, we've reached a local maximum
break
return current_x, current_value
# Use the hill climbing heuristic
best_x, best_value = hill_climbing(start_x=0)
print(f"Best x: {best_x}, Best value: {best_value}")
Step 5: Test and Refine
After implementing your heuristic, test it on various inputs, including edge cases. Analyze its performance in terms of solution quality and runtime. If the results aren’t satisfactory, consider:
- Adjusting parameters
- Modifying how solutions are generated or evaluated
- Combining multiple heuristics
- Trying a different heuristic altogether
Real-World Examples of Heuristics in Action
To better understand how heuristics are applied in practice, let’s look at some real-world examples:
1. The Traveling Salesman Problem (TSP)
The TSP is a classic NP-hard problem: given a list of cities and the distances between them, find the shortest possible route that visits each city exactly once and returns to the starting city.
Heuristic approaches for the TSP include:
- Nearest Neighbor: Always visit the closest unvisited city next.
- 2-Opt: Iteratively improve a route by swapping pairs of edges.
- Genetic Algorithms: Evolve populations of routes to find better solutions.
2. Chess Engines
Chess engines use various heuristics to evaluate positions and decide on moves, including:
- Piece-Square Tables: Assigning values to pieces based on their positions.
- Material Balance: Counting the value of pieces on the board.
- King Safety: Evaluating the safety of each player’s king.
3. Recommendation Systems
Online platforms like Netflix and Amazon use heuristic approaches in their recommendation systems, such as:
- Collaborative Filtering: Recommending items based on similar users’ preferences.
- Content-Based Filtering: Recommending items similar to those a user has liked in the past.
Common Pitfalls and How to Avoid Them
While heuristics are powerful tools, they come with potential pitfalls. Here are some common issues and how to address them:
1. Getting Stuck in Local Optima
Problem: Some heuristics, like hill climbing, can get stuck in local optima, missing better global solutions.
Solution: Use techniques like random restarts, simulated annealing, or genetic algorithms that can escape local optima.
2. Over-Reliance on a Single Heuristic
Problem: No single heuristic works best for all problems or instances.
Solution: Experiment with multiple heuristics and consider hybrid approaches that combine different techniques.
3. Ignoring Problem-Specific Knowledge
Problem: Generic heuristics might miss important problem-specific insights.
Solution: Incorporate domain knowledge into your heuristic design. For example, in a routing problem, you might use information about traffic patterns or road types.
4. Neglecting to Validate Results
Problem: Heuristics provide approximate solutions, which might not always be valid or good enough.
Solution: Always validate your solutions, even if it means using a slower, exact method on a subset of cases. Understand the trade-offs between solution quality and runtime.
Advanced Heuristic Techniques
As you become more comfortable with basic heuristics, you can explore more advanced techniques:
1. Meta-Heuristics
Meta-heuristics are higher-level procedures designed to find, generate, or select a heuristic that may provide a sufficiently good solution to an optimization problem. Examples include:
- Tabu Search
- Ant Colony Optimization
- Particle Swarm Optimization
2. Hyper-Heuristics
Hyper-heuristics automate the process of selecting or generating heuristics to solve hard computational search problems. They’re essentially heuristics to choose heuristics.
3. Machine Learning for Heuristics
Machine learning techniques can be used to improve heuristics by:
- Learning good parameter settings
- Predicting which heuristic will perform best on a given instance
- Generating new heuristics
Conclusion: Embracing the Power of Heuristics
As we’ve explored in this guide, heuristics are invaluable tools for solving hard problems in computer science and beyond. They allow us to find good solutions quickly, even when perfect solutions are out of reach. By understanding different types of heuristics, learning how to apply them, and being aware of their limitations, you’ll be well-equipped to tackle complex challenges in your coding journey.
Remember, the key to mastering heuristics is practice. Start by identifying problems in your projects or studies where exact solutions are impractical, and experiment with different heuristic approaches. As you gain experience, you’ll develop an intuition for which heuristics work best in different situations.
Embracing heuristics doesn’t mean abandoning the pursuit of optimal solutions altogether. Rather, it’s about expanding your problem-solving toolkit and recognizing when approximations are not just acceptable, but necessary. In the fast-paced world of software development and algorithmic problem-solving, the ability to quickly find good-enough solutions can be just as valuable as finding perfect ones.
So, the next time you’re faced with a seemingly insurmountable coding challenge, don’t be discouraged. Instead, ask yourself: “What heuristic approach might help me tackle this problem?” With practice and persistence, you’ll find that even the hardest problems become manageable, one heuristic at a time.