How to Master Breadth-First and Depth-First Search Algorithms
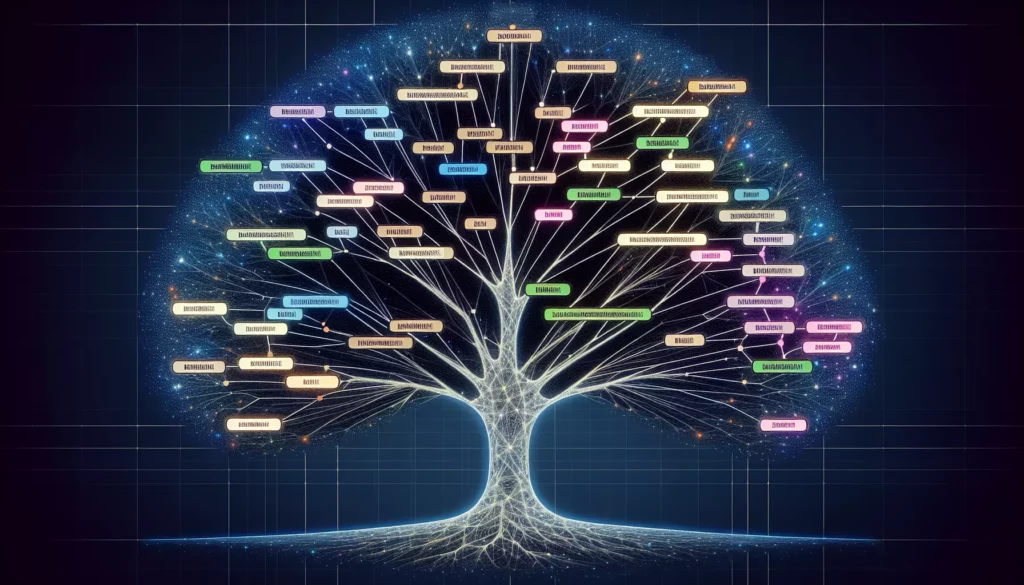
In the world of computer science and programming, mastering search algorithms is crucial for solving complex problems efficiently. Two fundamental search algorithms that every programmer should be familiar with are Breadth-First Search (BFS) and Depth-First Search (DFS). These algorithms are not only essential for technical interviews at major tech companies but also form the backbone of many advanced algorithms and applications.
In this comprehensive guide, we’ll dive deep into BFS and DFS, exploring their concepts, implementations, and applications. By the end of this article, you’ll have a solid understanding of these algorithms and be well-equipped to tackle related coding challenges.
Table of Contents
- Introduction to Graph Traversal
- Understanding Breadth-First Search (BFS)
- Implementing BFS
- Understanding Depth-First Search (DFS)
- Implementing DFS
- Comparing BFS and DFS
- Common Applications of BFS and DFS
- Practice Problems and Solutions
- Advanced Variations and Optimizations
- Conclusion
1. Introduction to Graph Traversal
Before we delve into BFS and DFS, it’s essential to understand the concept of graph traversal. In computer science, a graph is a data structure consisting of nodes (or vertices) connected by edges. Graph traversal refers to the process of visiting all the nodes in a graph in a specific order.
Graphs can be used to represent various real-world scenarios, such as:
- Social networks (users as nodes, friendships as edges)
- Road networks (intersections as nodes, roads as edges)
- Computer networks (devices as nodes, connections as edges)
- Web pages (pages as nodes, hyperlinks as edges)
BFS and DFS are two fundamental algorithms for traversing or searching graph data structures. They differ in the order in which they explore nodes, leading to different characteristics and use cases.
2. Understanding Breadth-First Search (BFS)
Breadth-First Search is an algorithm that explores a graph level by level. It starts at a chosen node (often called the “root” node) and explores all its neighbors before moving to the next level of nodes. This process continues until all reachable nodes have been visited.
Key characteristics of BFS:
- Explores nodes in order of their distance from the starting node
- Uses a queue data structure to keep track of nodes to visit
- Guarantees the shortest path in unweighted graphs
- Memory usage can be high for large graphs
BFS is particularly useful for finding the shortest path between two nodes in an unweighted graph, as it explores nodes in order of their distance from the starting point.
3. Implementing BFS
Let’s implement BFS in Python using an adjacency list representation of a graph:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("BFS traversal:")
bfs(graph, 'A')
This implementation uses a queue (implemented using Python’s deque
) to keep track of nodes to visit. It also uses a set to keep track of visited nodes to avoid revisiting them.
4. Understanding Depth-First Search (DFS)
Depth-First Search is an algorithm that explores a graph by going as deep as possible along each branch before backtracking. It starts at a chosen node and explores as far as possible along each branch before backtracking.
Key characteristics of DFS:
- Explores nodes by going deeper into the graph before exploring siblings
- Uses a stack data structure (or recursion) to keep track of nodes to visit
- Memory usage is generally lower than BFS
- Can be implemented recursively or iteratively
DFS is particularly useful for tasks like topological sorting, detecting cycles in a graph, and solving maze-like problems.
5. Implementing DFS
Let’s implement DFS in Python using both recursive and iterative approaches:
Recursive DFS:
def dfs_recursive(graph, vertex, visited=None):
if visited is None:
visited = set()
visited.add(vertex)
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
dfs_recursive(graph, neighbor, visited)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("DFS traversal (recursive):")
dfs_recursive(graph, 'A')
Iterative DFS:
def dfs_iterative(graph, start):
visited = set()
stack = [start]
while stack:
vertex = stack.pop()
if vertex not in visited:
visited.add(vertex)
print(vertex, end=' ')
for neighbor in reversed(graph[vertex]):
if neighbor not in visited:
stack.append(neighbor)
# Example usage
print("\nDFS traversal (iterative):")
dfs_iterative(graph, 'A')
The recursive implementation uses the call stack to keep track of nodes to visit, while the iterative implementation uses an explicit stack.
6. Comparing BFS and DFS
Now that we’ve explored both BFS and DFS, let’s compare their characteristics:
Characteristic | BFS | DFS |
---|---|---|
Traversal Order | Level by level | Deep into branches before backtracking |
Data Structure | Queue | Stack (or recursion) |
Memory Usage | Higher (stores all nodes at current level) | Lower (stores only nodes on current path) |
Completeness | Guaranteed to find solution if it exists | May get stuck in infinite loops if not implemented carefully |
Optimality | Finds shortest path in unweighted graphs | Not guaranteed to find shortest path |
Implementation | Typically iterative | Can be recursive or iterative |
7. Common Applications of BFS and DFS
Both BFS and DFS have numerous applications in computer science and real-world problem-solving. Here are some common use cases for each algorithm:
BFS Applications:
- Shortest path finding in unweighted graphs
- Web crawling
- Social network analysis (e.g., finding degrees of separation)
- GPS navigation systems
- Puzzle solving (e.g., Rubik’s cube)
- Finding connected components in a graph
DFS Applications:
- Topological sorting
- Cycle detection in graphs
- Maze solving
- Finding strongly connected components
- Generating permutations and combinations
- Sudoku solving
8. Practice Problems and Solutions
To solidify your understanding of BFS and DFS, let’s solve some common interview problems using these algorithms.
Problem 1: Find the shortest path between two nodes in an unweighted graph
from collections import deque
def shortest_path(graph, start, end):
queue = deque([(start, [start])])
visited = set([start])
while queue:
(vertex, path) = queue.popleft()
if vertex == end:
return path
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append((neighbor, path + [neighbor]))
return None
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print("Shortest path from A to F:")
print(shortest_path(graph, 'A', 'F'))
Problem 2: Detect a cycle in a directed graph using DFS
def has_cycle(graph):
visited = set()
rec_stack = set()
def dfs(vertex):
visited.add(vertex)
rec_stack.add(vertex)
for neighbor in graph[vertex]:
if neighbor not in visited:
if dfs(neighbor):
return True
elif neighbor in rec_stack:
return True
rec_stack.remove(vertex)
return False
for vertex in graph:
if vertex not in visited:
if dfs(vertex):
return True
return False
# Example usage
graph_with_cycle = {
'A': ['B'],
'B': ['C'],
'C': ['A', 'D'],
'D': []
}
graph_without_cycle = {
'A': ['B', 'C'],
'B': ['D'],
'C': ['D'],
'D': []
}
print("Graph with cycle:", has_cycle(graph_with_cycle))
print("Graph without cycle:", has_cycle(graph_without_cycle))
9. Advanced Variations and Optimizations
As you become more comfortable with BFS and DFS, you can explore advanced variations and optimizations:
Bidirectional BFS
This variation runs two simultaneous BFS searches: one from the start node and one from the goal node. It can be significantly faster than standard BFS for finding the shortest path between two nodes.
Iterative Deepening Depth-First Search (IDDFS)
This algorithm combines the space efficiency of DFS with the completeness of BFS. It performs a series of DFS searches with increasing depth limits until the goal is found.
A* Search
A* is a best-first search algorithm that uses heuristics to guide its search. It’s often used in pathfinding and graph traversal problems where BFS might be too slow.
Memory-Efficient BFS
For graphs with a large branching factor, standard BFS can consume a lot of memory. Techniques like frontier search can help reduce memory usage while maintaining BFS’s completeness and optimality properties.
10. Conclusion
Mastering Breadth-First Search and Depth-First Search algorithms is crucial for any programmer looking to excel in technical interviews and tackle complex problems efficiently. These algorithms form the foundation for many advanced graph algorithms and have wide-ranging applications in computer science and beyond.
As you continue your journey in algorithm mastery, remember to:
- Practice implementing BFS and DFS from scratch
- Solve diverse problems using these algorithms
- Analyze the time and space complexity of your implementations
- Explore advanced variations and optimizations
- Apply these algorithms to real-world problems in your projects
With consistent practice and application, you’ll develop a deep understanding of these fundamental search algorithms, setting a strong foundation for your programming career and preparing you for success in technical interviews at top tech companies.
Remember, the key to mastery is not just understanding the concepts but also applying them to solve diverse problems. Keep practicing, stay curious, and don’t hesitate to explore more advanced topics as you grow in your algorithmic thinking and problem-solving skills.