Tips for Implementing Memoization in Dynamic Programming
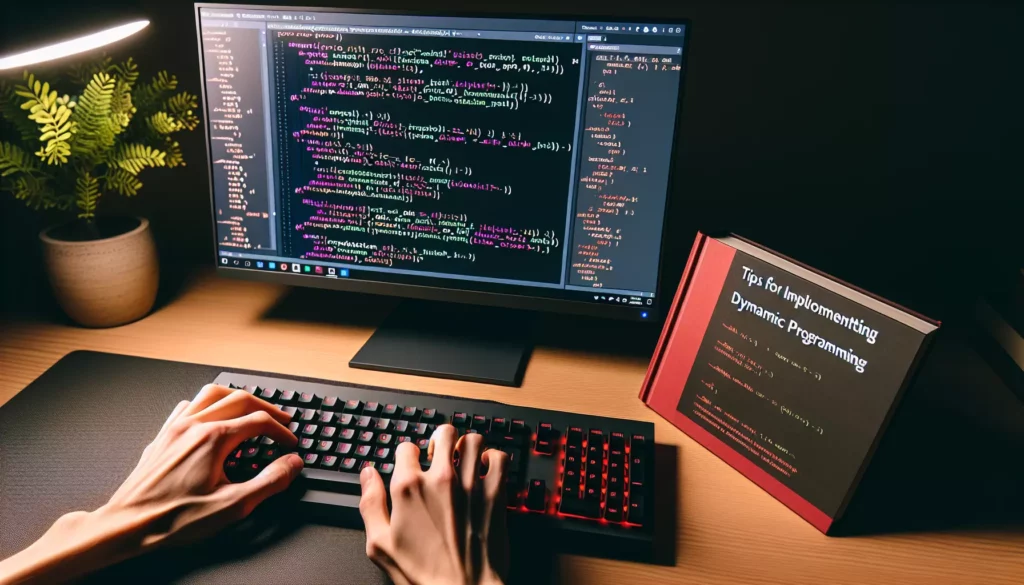
Dynamic programming is a powerful technique in computer science that can significantly improve the efficiency of algorithms by breaking down complex problems into smaller subproblems and storing their solutions for future use. One of the key components of dynamic programming is memoization, which plays a crucial role in optimizing the performance of recursive algorithms. In this comprehensive guide, we’ll explore various tips and strategies for implementing memoization in dynamic programming, helping you enhance your coding skills and prepare for technical interviews at top tech companies.
What is Memoization?
Before diving into the implementation tips, let’s briefly review what memoization is and why it’s important in dynamic programming.
Memoization is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again. It’s a specific form of caching that is used in dynamic programming to avoid redundant calculations and improve overall performance.
The basic idea behind memoization is simple:
- Store the results of expensive function calls
- Return the cached result when the same inputs occur again
By implementing memoization, we can often transform algorithms with exponential time complexity into ones with polynomial time complexity, making them much more efficient and scalable.
Tip 1: Choose the Right Data Structure for Memoization
One of the most critical decisions when implementing memoization is choosing the appropriate data structure to store the cached results. The choice of data structure can significantly impact the performance and memory usage of your algorithm. Here are some common options:
1. Arrays
Arrays are an excellent choice for memoization when dealing with problems that have a fixed range of input values, typically represented by integers. They offer constant-time access and are memory-efficient for small to medium-sized input ranges.
Example of using an array for memoization in a Fibonacci sequence calculation:
function fibonacci(n, memo = []) {
if (n <= 1) return n;
if (memo[n] !== undefined) return memo[n];
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
console.log(fibonacci(100)); // Calculates the 100th Fibonacci number efficiently
2. Hash Tables (Objects in JavaScript)
Hash tables are versatile and can be used for a wide range of input types, including strings and complex objects. They provide near-constant time access and are ideal for problems with a large or unpredictable range of inputs.
Example of using a hash table for memoization in a recursive string matching problem:
function isMatch(s, p, memo = {}) {
const key = `${s},${p}`;
if (key in memo) return memo[key];
if (p === '') return s === '';
const firstMatch = s.length > 0 && (p[0] === s[0] || p[0] === '.');
if (p.length >= 2 && p[1] === '*') {
memo[key] = isMatch(s, p.slice(2), memo) || (firstMatch && isMatch(s.slice(1), p, memo));
} else {
memo[key] = firstMatch && isMatch(s.slice(1), p.slice(1), memo);
}
return memo[key];
}
console.log(isMatch("aa", "a*")); // Returns true
3. Sets
Sets are useful for memoization when you only need to keep track of whether a particular input has been processed before, without storing the actual result. They are memory-efficient and provide fast lookup times.
Example of using a Set for memoization in a graph traversal problem:
function hasPath(graph, start, end, visited = new Set()) {
if (start === end) return true;
if (visited.has(start)) return false;
visited.add(start);
for (const neighbor of graph[start]) {
if (hasPath(graph, neighbor, end, visited)) {
return true;
}
}
return false;
}
const graph = {
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
};
console.log(hasPath(graph, 'A', 'F')); // Returns true
Tip 2: Implement Top-Down (Recursive) Memoization
Top-down memoization, also known as recursive memoization, is a straightforward and intuitive way to implement dynamic programming. It involves starting with the main problem and recursively breaking it down into smaller subproblems, while storing and reusing the results of these subproblems.
Here are some tips for implementing top-down memoization:
1. Use Default Parameters for the Memo
In languages that support default parameters, like JavaScript, you can initialize the memoization data structure as a default parameter. This keeps the function signature clean and allows for easy testing without explicitly passing the memo.
function climbStairs(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 2) return n;
memo[n] = climbStairs(n - 1, memo) + climbStairs(n - 2, memo);
return memo[n];
}
console.log(climbStairs(50)); // Efficiently calculates the number of ways to climb 50 stairs
2. Use a Unique Key for Complex Inputs
When dealing with multiple input parameters or complex objects, create a unique key to store in the memo. This ensures that each unique combination of inputs has its own cached result.
function knapsack(weights, values, capacity, index = 0, memo = {}) {
const key = `${capacity},${index}`;
if (key in memo) return memo[key];
if (index === weights.length || capacity === 0) {
return 0;
}
if (weights[index] > capacity) {
memo[key] = knapsack(weights, values, capacity, index + 1, memo);
} else {
memo[key] = Math.max(
values[index] + knapsack(weights, values, capacity - weights[index], index + 1, memo),
knapsack(weights, values, capacity, index + 1, memo)
);
}
return memo[key];
}
const weights = [10, 20, 30];
const values = [60, 100, 120];
const capacity = 50;
console.log(knapsack(weights, values, capacity)); // Returns 220
3. Consider Using a Wrapper Function
For some problems, it may be beneficial to use a wrapper function that initializes the memoization data structure and calls the main recursive function. This can help keep the code organized and make it easier to add additional functionality or preprocessing steps.
function longestCommonSubsequence(text1, text2) {
const memo = {};
function lcs(i, j) {
const key = `${i},${j}`;
if (key in memo) return memo[key];
if (i === text1.length || j === text2.length) {
return 0;
}
if (text1[i] === text2[j]) {
memo[key] = 1 + lcs(i + 1, j + 1);
} else {
memo[key] = Math.max(lcs(i + 1, j), lcs(i, j + 1));
}
return memo[key];
}
return lcs(0, 0);
}
console.log(longestCommonSubsequence("abcde", "ace")); // Returns 3
Tip 3: Implement Bottom-Up (Iterative) Memoization
While top-down memoization is intuitive and easy to implement, bottom-up memoization (also known as tabulation) can often be more efficient in terms of both time and space complexity. Bottom-up approaches start by solving the smallest subproblems and build up to the main problem, typically using iteration rather than recursion.
Here are some tips for implementing bottom-up memoization:
1. Identify the Dimensions of the Memoization Table
Determine the number of changing parameters in your recursive solution and use them to create an appropriately sized memoization table. For example, if your recursive solution has two changing parameters, you’ll likely need a 2D table.
function longestCommonSubsequence(text1, text2) {
const m = text1.length;
const n = text2.length;
const dp = Array(m + 1).fill().map(() => Array(n + 1).fill(0));
for (let i = 1; i <= m; i++) {
for (let j = 1; j <= n; j++) {
if (text1[i - 1] === text2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
} else {
dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
}
}
}
return dp[m][n];
}
console.log(longestCommonSubsequence("abcde", "ace")); // Returns 3
2. Initialize Base Cases
Start by initializing the base cases in your memoization table. These are typically the simplest subproblems that don’t require any calculation.
function coinChange(coins, amount) {
const dp = Array(amount + 1).fill(Infinity);
dp[0] = 0; // Base case: 0 coins needed to make amount 0
for (let i = 1; i <= amount; i++) {
for (const coin of coins) {
if (coin <= i) {
dp[i] = Math.min(dp[i], dp[i - coin] + 1);
}
}
}
return dp[amount] === Infinity ? -1 : dp[amount];
}
console.log(coinChange([1, 2, 5], 11)); // Returns 3
3. Optimize Space Usage
In many cases, you can optimize the space usage of your bottom-up solution by using a smaller memoization table or even a single variable if you only need the previous state to calculate the current one.
function climbStairs(n) {
if (n <= 2) return n;
let prev = 1;
let curr = 2;
for (let i = 3; i <= n; i++) {
const next = prev + curr;
prev = curr;
curr = next;
}
return curr;
}
console.log(climbStairs(50)); // Efficiently calculates the number of ways to climb 50 stairs
Tip 4: Handle Edge Cases and Input Validation
When implementing memoization, it’s crucial to handle edge cases and validate inputs properly. This not only makes your code more robust but also helps prevent unnecessary calculations and potential errors.
1. Check for Invalid Inputs
Always validate your inputs at the beginning of your function to catch any invalid or edge cases early.
function fibonacci(n, memo = {}) {
if (typeof n !== 'number' || n < 0 || !Number.isInteger(n)) {
throw new Error('Input must be a non-negative integer');
}
if (n <= 1) return n;
if (n in memo) return memo[n];
memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo);
return memo[n];
}
console.log(fibonacci(10)); // Returns 55
// console.log(fibonacci(-1)); // Throws an error
// console.log(fibonacci(3.5)); // Throws an error
2. Handle Base Cases First
Always check for base cases before accessing the memoization cache or performing any calculations. This ensures that your function returns correct results for the simplest inputs and prevents unnecessary memoization.
function editDistance(word1, word2, memo = {}) {
if (word1 === word2) return 0;
if (word1.length === 0) return word2.length;
if (word2.length === 0) return word1.length;
const key = `${word1},${word2}`;
if (key in memo) return memo[key];
if (word1[0] === word2[0]) {
memo[key] = editDistance(word1.slice(1), word2.slice(1), memo);
} else {
memo[key] = 1 + Math.min(
editDistance(word1.slice(1), word2, memo),
editDistance(word1, word2.slice(1), memo),
editDistance(word1.slice(1), word2.slice(1), memo)
);
}
return memo[key];
}
console.log(editDistance("kitten", "sitting")); // Returns 3
3. Consider Using Sentinel Values
In some cases, it may be useful to use sentinel values in your memoization cache to distinguish between cached results and uncomputed values. This can be particularly helpful when dealing with problems where the result could be any valid number, including zero.
function canSum(target, numbers, memo = {}) {
if (target in memo) return memo[target];
if (target === 0) return true;
if (target < 0) return false;
for (const num of numbers) {
if (canSum(target - num, numbers, memo)) {
memo[target] = true;
return true;
}
}
memo[target] = false;
return false;
}
console.log(canSum(7, [2, 3])); // Returns true
console.log(canSum(300, [7, 14])); // Returns false
Tip 5: Optimize Memoization for Space Efficiency
While memoization can significantly improve time complexity, it often comes at the cost of increased space usage. Here are some tips to optimize your memoization implementations for better space efficiency:
1. Use Bit Manipulation for Compact State Representation
For problems with a limited number of states, you can use bit manipulation to represent the state compactly, reducing memory usage.
function countBits(n) {
const dp = new Uint32Array(n + 1);
for (let i = 1; i <= n; i++) {
dp[i] = dp[i >> 1] + (i & 1);
}
return dp;
}
console.log(countBits(5)); // Returns [0, 1, 1, 2, 1, 2]
2. Implement a Custom Caching Mechanism
For very large problems, you might want to implement a custom caching mechanism that automatically evicts least recently used items when the cache size exceeds a certain threshold.
class LRUCache {
constructor(capacity) {
this.capacity = capacity;
this.cache = new Map();
}
get(key) {
if (!this.cache.has(key)) return -1;
const value = this.cache.get(key);
this.cache.delete(key);
this.cache.set(key, value);
return value;
}
put(key, value) {
if (this.cache.has(key)) {
this.cache.delete(key);
} else if (this.cache.size >= this.capacity) {
const oldestKey = this.cache.keys().next().value;
this.cache.delete(oldestKey);
}
this.cache.set(key, value);
}
}
function fibonacci(n, cache = new LRUCache(100)) {
if (n <= 1) return n;
const cachedResult = cache.get(n);
if (cachedResult !== -1) return cachedResult;
const result = fibonacci(n - 1, cache) + fibonacci(n - 2, cache);
cache.put(n, result);
return result;
}
console.log(fibonacci(100)); // Efficiently calculates the 100th Fibonacci number
3. Use Rolling Arrays for Problems with Limited Dependency
For problems where the current state only depends on a limited number of previous states, you can use rolling arrays to reduce space complexity.
function minCostClimbingStairs(cost) {
const n = cost.length;
let [a, b] = [cost[0], cost[1]];
for (let i = 2; i < n; i++) {
[a, b] = [b, Math.min(a, b) + cost[i]];
}
return Math.min(a, b);
}
console.log(minCostClimbingStairs([10, 15, 20])); // Returns 15
Conclusion
Implementing memoization in dynamic programming is a powerful technique that can significantly improve the performance of your algorithms. By following these tips and strategies, you’ll be better equipped to tackle complex problems efficiently and optimize your solutions for both time and space complexity.
Remember that mastering memoization and dynamic programming takes practice. As you work on more problems and implement these techniques, you’ll develop a better intuition for when and how to apply memoization effectively. Keep practicing, and don’t hesitate to revisit these tips as you continue to improve your skills in preparation for technical interviews at top tech companies.
Happy coding, and may your algorithms be ever efficient!