Best Practices for Using Backtracking to Solve Puzzles
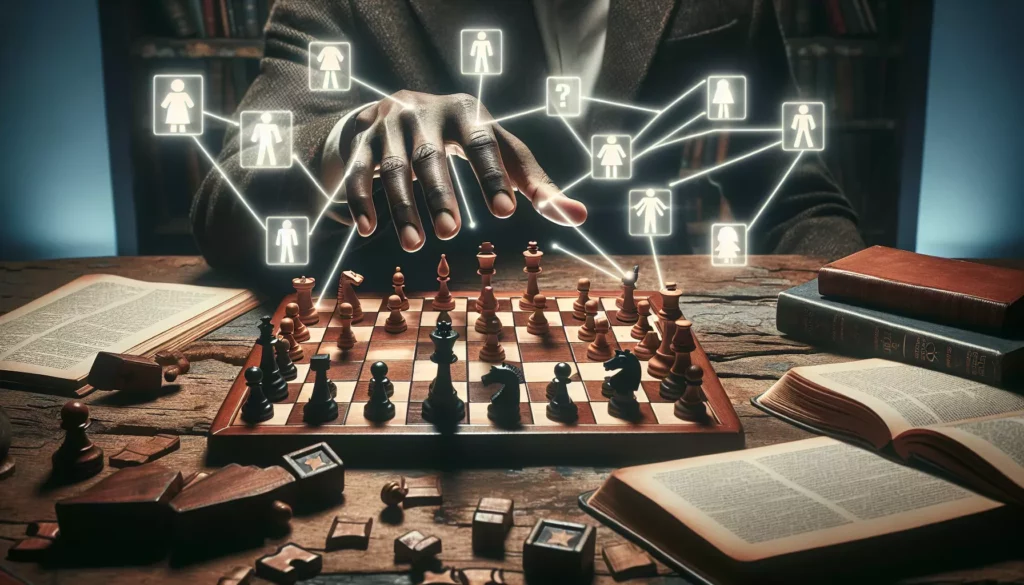
Backtracking is a powerful algorithmic technique used to solve complex puzzles and problems in computer science. It’s particularly useful when dealing with combinatorial problems where you need to find all (or some) solutions to a computational problem. In this comprehensive guide, we’ll explore the best practices for using backtracking to solve puzzles, providing you with the knowledge and skills to tackle a wide range of challenging problems.
Understanding Backtracking
Before diving into the best practices, let’s briefly review what backtracking is and how it works.
Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. It builds candidates to the solution incrementally and abandons a candidate (“backtracks”) as soon as it determines that the candidate cannot lead to a valid solution.
The general approach to backtracking problems can be summarized as follows:
- Choose: Make a choice for the next step in building the solution.
- Constraint: Check if the choice satisfies all constraints.
- Goal: Check if the current state represents a valid solution.
- Recurse: If the choice is valid and doesn’t lead to a solution yet, make a recursive call to proceed further.
- Undo: If the choice doesn’t work out, undo it and try the next option.
Best Practices for Backtracking
1. Clearly Define the Problem Space
Before implementing a backtracking solution, it’s crucial to have a clear understanding of the problem space. This includes:
- Identifying the constraints of the problem
- Defining what constitutes a valid solution
- Understanding the structure of partial solutions
For example, in the classic N-Queens problem, you need to place N chess queens on an N×N chessboard so that no two queens threaten each other. The constraints are that no two queens can be in the same row, column, or diagonal.
2. Choose an Appropriate Data Structure
Selecting the right data structure to represent your problem state is crucial for an efficient backtracking implementation. Common choices include:
- Arrays or matrices for grid-based puzzles (e.g., Sudoku, N-Queens)
- Strings for permutation problems
- Graphs for path-finding or coloring problems
Your choice should allow for easy modification, checking of constraints, and undoing of choices.
3. Implement Efficient State Checking
One of the key aspects of backtracking is quickly determining whether a partial solution is valid and worth exploring further. Implement efficient methods to check the current state against the problem constraints.
For example, in the Sudoku solver, you might maintain sets for each row, column, and 3×3 sub-grid to quickly check if a number can be placed in a given cell.
def is_valid(board, row, col, num):
# Check row
if num in board[row]:
return False
# Check column
if num in [board[i][col] for i in range(9)]:
return False
# Check 3x3 sub-grid
start_row, start_col = 3 * (row // 3), 3 * (col // 3)
for i in range(3):
for j in range(3):
if board[start_row + i][start_col + j] == num:
return False
return True
4. Optimize the Order of Choices
The order in which you explore choices can significantly impact the efficiency of your backtracking algorithm. Consider these strategies:
- Start with the most constrained choices first
- Use heuristics to guide the search towards promising solutions
- Implement pruning techniques to eliminate unnecessary branches early
For instance, in a Sudoku solver, you might want to start filling in the cells with the fewest possible valid numbers.
5. Implement Efficient Backtracking
When a choice doesn’t lead to a solution, it’s important to efficiently undo that choice and try the next option. This often involves:
- Restoring the previous state
- Updating any auxiliary data structures
- Moving to the next choice
Here’s an example of a backtracking function for solving Sudoku:
def solve_sudoku(board):
empty = find_empty(board)
if not empty:
return True # Puzzle is solved
row, col = empty
for num in range(1, 10):
if is_valid(board, row, col, num):
board[row][col] = num
if solve_sudoku(board):
return True
board[row][col] = 0 # Undo the choice
return False # No valid solution found
6. Use Memoization When Appropriate
In some backtracking problems, you might encounter the same subproblems multiple times. In such cases, memoization can significantly improve performance by storing and reusing the results of expensive function calls.
For example, in the context of solving a crossword puzzle, you might memoize the validity of word placements:
from functools import lru_cache
@lru_cache(maxsize=None)
def is_valid_word_placement(word, row, col, direction):
# Check if the word can be placed at the given position
# ...
return True # or False
7. Implement Early Termination
If you’re only interested in finding one solution (rather than all possible solutions), make sure your algorithm terminates as soon as a valid solution is found. This can significantly reduce execution time for large problem spaces.
def solve_puzzle(state):
if is_solution(state):
return state
for choice in get_possible_choices(state):
new_state = apply_choice(state, choice)
if is_valid(new_state):
result = solve_puzzle(new_state)
if result is not None:
return result # Solution found, terminate early
return None # No solution found
8. Use Iterative Deepening When Appropriate
For problems with an unknown or very large depth, consider using iterative deepening. This technique combines the space-efficiency of depth-first search with the completeness of breadth-first search.
def iterative_deepening_solve(initial_state, max_depth):
for depth in range(max_depth):
result = depth_limited_solve(initial_state, depth)
if result is not None:
return result
return None # No solution found within max_depth
def depth_limited_solve(state, depth):
if depth == 0 and is_solution(state):
return state
if depth > 0:
for choice in get_possible_choices(state):
new_state = apply_choice(state, choice)
if is_valid(new_state):
result = depth_limited_solve(new_state, depth - 1)
if result is not None:
return result
return None
9. Implement Constraint Propagation
For certain types of puzzles, especially those with interconnected constraints, implementing constraint propagation can significantly reduce the search space. This technique involves applying local constraints to eliminate invalid choices before exploring them.
A classic example is the AC-3 algorithm used in Constraint Satisfaction Problems (CSPs):
from collections import deque
def ac3(csp):
queue = deque([(Xi, Xk) for Xi in csp.variables for Xk in csp.neighbors[Xi]])
while queue:
(Xi, Xj) = queue.popleft()
if revise(csp, Xi, Xj):
if len(csp.domains[Xi]) == 0:
return False
for Xk in csp.neighbors[Xi] - {Xj}:
queue.append((Xk, Xi))
return True
def revise(csp, Xi, Xj):
revised = False
for x in csp.domains[Xi][:]:
if not any(csp.constraints(Xi, x, Xj, y) for y in csp.domains[Xj]):
csp.domains[Xi].remove(x)
revised = True
return revised
10. Profile and Optimize
After implementing your backtracking solution, it’s crucial to profile its performance and optimize where necessary. Look for:
- Bottlenecks in constraint checking
- Unnecessary function calls or object creations
- Opportunities for more aggressive pruning
Use profiling tools specific to your programming language to identify performance hotspots.
Common Backtracking Puzzles and Their Solutions
To further illustrate the application of these best practices, let’s look at some common puzzles that can be solved using backtracking:
1. N-Queens Problem
The N-Queens problem asks to place N chess queens on an N×N chessboard so that no two queens threaten each other.
def solve_n_queens(n):
def is_safe(board, row, col):
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0 # Backtrack
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0):
return board
return None
# Example usage
solution = solve_n_queens(8)
if solution:
for row in solution:
print(row)
else:
print("No solution exists")
2. Sudoku Solver
Sudoku is a logic-based number-placement puzzle. The objective is to fill a 9×9 grid with digits so that each column, row, and 3×3 sub-grid contains all digits from 1 to 9.
def solve_sudoku(board):
def find_empty(board):
for i in range(len(board)):
for j in range(len(board[0])):
if board[i][j] == 0:
return i, j
return None
def is_valid(board, num, pos):
# Check row
for j in range(len(board[0])):
if board[pos[0]][j] == num and pos[1] != j:
return False
# Check column
for i in range(len(board)):
if board[i][pos[1]] == num and pos[0] != i:
return False
# Check 3x3 box
box_x = pos[1] // 3
box_y = pos[0] // 3
for i in range(box_y * 3, box_y * 3 + 3):
for j in range(box_x * 3, box_x * 3 + 3):
if board[i][j] == num and (i, j) != pos:
return False
return True
empty = find_empty(board)
if not empty:
return True
else:
row, col = empty
for num in range(1, 10):
if is_valid(board, num, (row, col)):
board[row][col] = num
if solve_sudoku(board):
return True
board[row][col] = 0
return False
# Example usage
board = [
[5, 3, 0, 0, 7, 0, 0, 0, 0],
[6, 0, 0, 1, 9, 5, 0, 0, 0],
[0, 9, 8, 0, 0, 0, 0, 6, 0],
[8, 0, 0, 0, 6, 0, 0, 0, 3],
[4, 0, 0, 8, 0, 3, 0, 0, 1],
[7, 0, 0, 0, 2, 0, 0, 0, 6],
[0, 6, 0, 0, 0, 0, 2, 8, 0],
[0, 0, 0, 4, 1, 9, 0, 0, 5],
[0, 0, 0, 0, 8, 0, 0, 7, 9]
]
if solve_sudoku(board):
for row in board:
print(row)
else:
print("No solution exists")
3. Permutations
Generating all permutations of a given set of elements is a classic backtracking problem.
def generate_permutations(elements):
def backtrack(start):
if start == len(elements):
result.append(elements[:])
else:
for i in range(start, len(elements)):
elements[start], elements[i] = elements[i], elements[start]
backtrack(start + 1)
elements[start], elements[i] = elements[i], elements[start] # Backtrack
result = []
backtrack(0)
return result
# Example usage
elements = [1, 2, 3]
permutations = generate_permutations(elements)
for perm in permutations:
print(perm)
Conclusion
Backtracking is a powerful technique for solving a wide range of puzzles and computational problems. By following these best practices, you can implement efficient and effective backtracking solutions:
- Clearly define the problem space
- Choose appropriate data structures
- Implement efficient state checking
- Optimize the order of choices
- Implement efficient backtracking
- Use memoization when appropriate
- Implement early termination
- Consider iterative deepening for large problem spaces
- Implement constraint propagation for interconnected constraints
- Profile and optimize your solution
Remember that while backtracking can solve many complex problems, it’s not always the most efficient solution for every scenario. For some problems, dynamic programming, greedy algorithms, or other specialized techniques might be more appropriate. Always analyze the problem carefully and consider alternative approaches before settling on a backtracking solution.
By mastering backtracking and understanding when to apply it, you’ll be well-equipped to tackle a wide range of challenging puzzles and problems in computer science and programming interviews. Keep practicing with different types of problems to hone your skills and develop an intuition for when and how to apply backtracking effectively.