How to Use Git Squash for Streamlined Commit History
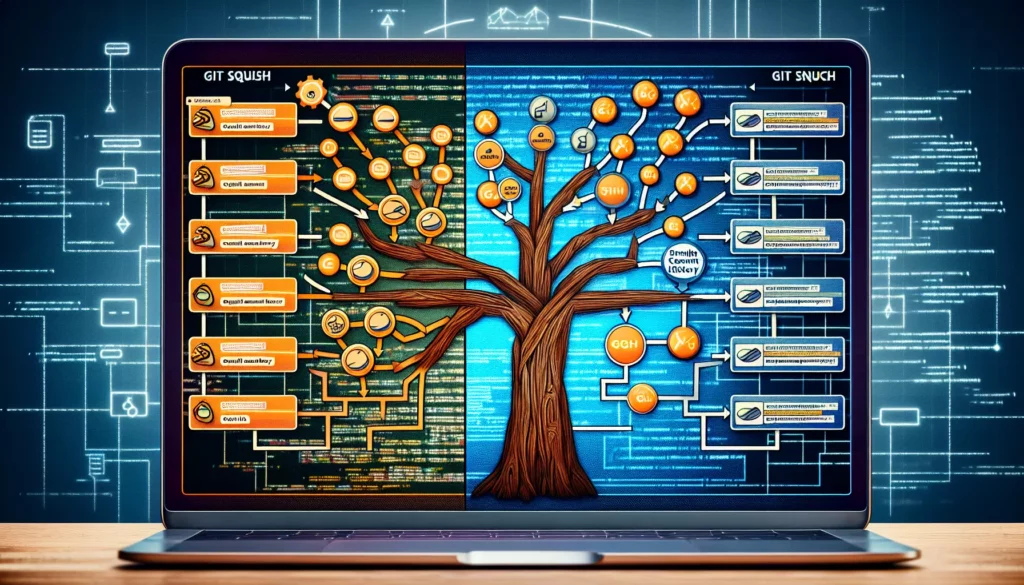
In the world of software development, version control is an essential tool for managing code changes and collaborating with team members. Git, one of the most popular version control systems, offers a powerful feature called “squashing” that allows developers to combine multiple commits into a single, more coherent commit. This technique is particularly useful for maintaining a clean and organized commit history. In this comprehensive guide, we’ll explore how to use Git squash effectively to streamline your commit history and improve your overall Git workflow.
What is Git Squash?
Before diving into the how-to, let’s first understand what Git squash is and why it’s beneficial. Git squash is a process of combining multiple commits into one. This is typically done to clean up the commit history, making it more readable and easier to understand the overall changes made to a project.
Some key benefits of using Git squash include:
- Simplifying the commit history
- Grouping related changes together
- Making code reviews easier
- Facilitating easier reverting of changes if necessary
When to Use Git Squash
While Git squash is a powerful tool, it’s important to use it judiciously. Here are some scenarios where squashing commits can be particularly useful:
- When you’ve made multiple small, incremental commits while working on a feature
- Before merging a feature branch into the main branch
- When preparing a pull request for review
- To combine fixup commits with their original commits
How to Squash Commits Using Interactive Rebase
The most common method for squashing commits is using Git’s interactive rebase feature. Here’s a step-by-step guide on how to do it:
1. Identify the Commits to Squash
First, you need to determine which commits you want to squash. Use the following command to view your recent commits:
git log --oneline
This will display a list of commits with their hash and commit messages. Identify the range of commits you want to squash.
2. Start the Interactive Rebase
Once you’ve identified the commits, start the interactive rebase by running:
git rebase -i HEAD~n
Replace ‘n’ with the number of commits you want to include in the rebase. For example, if you want to include the last 3 commits, you would use:
git rebase -i HEAD~3
3. Edit the Rebase Todo List
This command will open your default text editor with a list of the commits you selected. The list will look something like this:
pick f7f3f6d Change button color
pick 310154e Update header text
pick a5f4a0d Add new feature
To squash commits, change the word ‘pick’ to ‘squash’ (or just ‘s’ for short) for the commits you want to combine. Leave the first commit as ‘pick’. For example:
pick f7f3f6d Change button color
squash 310154e Update header text
squash a5f4a0d Add new feature
4. Save and Close the Editor
After making your changes, save the file and close the editor. Git will then combine the specified commits.
5. Edit the Commit Message
Git will then open another editor window where you can edit the commit message for the new, combined commit. Write a clear and concise message that summarizes all the changes, then save and close the editor.
6. Force Push (if necessary)
If you’ve already pushed the original commits to a remote repository, you’ll need to force push the new, squashed commit:
git push origin branch-name --force
Note: Be cautious when using force push, especially on shared branches, as it can overwrite the remote history.
Alternative Methods for Squashing Commits
While interactive rebase is the most flexible method for squashing commits, there are other approaches you can use depending on your specific situation:
1. Squashing During Merge
When merging a feature branch into the main branch, you can use the –squash option to combine all the commits from the feature branch into a single commit:
git merge --squash feature-branch
This will stage all the changes from the feature branch without actually committing them. You can then create a new commit with all these changes:
git commit -m "Implement feature X"
2. Using Git Reset
If you want to squash the last n commits on your current branch, you can use git reset:
git reset --soft HEAD~n
git commit -m "New commit message"
This will reset your branch to n commits ago, keeping all the changes as staged. You can then create a new commit with all these changes.
Best Practices for Using Git Squash
To make the most of Git squash and maintain a clean, useful commit history, consider these best practices:
- Squash related commits: Combine commits that are part of the same logical change or feature.
- Write meaningful commit messages: When squashing, take the time to write a comprehensive commit message that accurately describes all the changes.
- Preserve important information: If individual commits contain important context or information, consider including it in the squashed commit message.
- Squash before merging: It’s often best to squash commits in a feature branch before merging into the main branch.
- Communicate with your team: If you’re working on a shared repository, make sure your team is aware of and agrees with your squashing strategy.
- Don’t squash commits that have already been pushed and shared: Squashing should generally be done on local or personal branches before they’re shared with others.
Potential Pitfalls and How to Avoid Them
While Git squash is a powerful tool, there are some potential pitfalls to be aware of:
1. Loss of Detailed History
Pitfall: Squashing commits can result in the loss of detailed historical information about how a feature was developed.
Solution: Use squashing judiciously. For important development milestones or complex features, consider keeping some intermediate commits.
2. Conflicts with Shared Branches
Pitfall: Squashing commits that have already been pushed to a shared branch can cause conflicts for other developers.
Solution: Only squash commits on local or personal branches before they’re shared. If you need to squash on a shared branch, communicate clearly with your team and coordinate the process.
3. Loss of Git Bisect Functionality
Pitfall: Aggressive squashing can make it harder to use Git’s bisect feature to find the commit that introduced a bug.
Solution: Maintain a balance between a clean history and preserving useful intermediate states. Consider keeping commits that represent stable, testable states of your project.
Advanced Git Squash Techniques
Once you’re comfortable with basic squashing, you can explore some more advanced techniques:
1. Autosquashing with Fixup Commits
Git allows you to create “fixup” commits that can be automatically squashed during an interactive rebase:
git commit --fixup <commit-hash>
Then, when you run an interactive rebase with the –autosquash option, Git will automatically mark these commits for squashing:
git rebase -i --autosquash <base-commit>
2. Partial Squashing
You can squash only part of a commit by using the ‘edit’ option in interactive rebase. This allows you to make changes to a commit before continuing the rebase.
3. Squashing Across Branches
You can use cherry-pick to bring commits from one branch to another and then squash them:
git cherry-pick <commit1> <commit2> <commit3>
git reset --soft HEAD~3
git commit -m "Squashed commit message"
Git Squash and Continuous Integration
When using Git squash in a continuous integration (CI) environment, there are a few additional considerations:
- Branch Protection: Many CI systems allow you to protect certain branches (like main) from force pushes, which can interfere with squashing. Configure your CI system appropriately.
- Build Triggers: Squashing can change commit hashes, which might affect how your CI system triggers builds. Ensure your CI configuration can handle this.
- Code Review Integration: If you use a code review system integrated with your CI, squashing might affect how changes are displayed or tracked. Familiarize yourself with how your specific tools handle squashed commits.
Git Squash in Different Git Workflows
The way you use Git squash might vary depending on your team’s Git workflow:
1. Feature Branch Workflow
In this workflow, squashing is typically done before merging a feature branch into the main branch. This keeps the main branch history clean and focused on completed features.
2. Gitflow Workflow
In Gitflow, you might squash commits on feature branches before merging into the develop branch. However, you might choose to preserve more granular history on release branches.
3. Forking Workflow
In a forking workflow, contributors might squash their commits before submitting a pull request to the main repository. This makes it easier for maintainers to review and merge contributions.
Conclusion
Git squash is a powerful technique for maintaining a clean and meaningful commit history. By combining related commits, you can make your project’s history more readable and easier to understand. However, it’s important to use this feature judiciously and in accordance with your team’s workflow and best practices.
Remember, the goal of version control is not just to track changes, but to tell the story of your project’s development. Used wisely, Git squash can help you write that story more clearly and effectively.
As you continue to develop your Git skills, consider exploring other advanced Git features and techniques. The more comfortable you become with Git’s powerful toolset, the more effectively you’ll be able to manage your code and collaborate with others.