The Benefits of Learning Git Early in Your Coding Journey
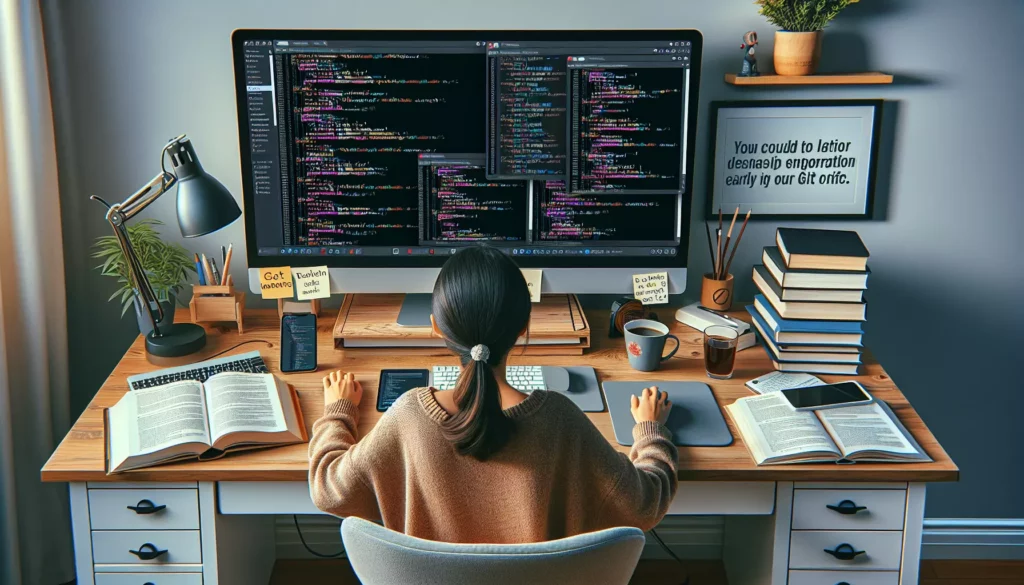
Embarking on a coding journey is an exciting and challenging endeavor. As you dive into the world of programming, you’ll encounter various tools and technologies that are essential for modern software development. One such tool that stands out for its importance and ubiquity is Git. In this comprehensive guide, we’ll explore why learning Git early in your coding journey can be immensely beneficial and how it can set you up for success in your future career as a developer.
What is Git?
Before we delve into the benefits, let’s briefly explain what Git is for those who might be unfamiliar. Git is a distributed version control system that helps developers track changes in their code, collaborate with others, and manage different versions of their projects. Created by Linus Torvalds in 2005, Git has become the de facto standard for version control in the software development industry.
Why Learning Git Early Matters
Now that we have a basic understanding of Git, let’s explore the numerous benefits of learning this powerful tool early in your coding journey:
1. Version Control from the Start
One of the primary advantages of learning Git early is that you can start implementing version control practices from the very beginning of your coding journey. This means:
- You can track changes in your code over time
- You can revert to previous versions if something goes wrong
- You can experiment with new features without fear of breaking your main codebase
By incorporating Git into your workflow from the start, you’ll develop good habits that will serve you well throughout your career.
2. Better Understanding of Code Evolution
Git allows you to see how your code evolves over time. This is particularly valuable for beginners who are still learning the ropes. By reviewing your commit history, you can:
- Understand how your coding style and approach have improved
- Identify patterns in your problem-solving techniques
- Learn from your mistakes by seeing what changes led to bugs or inefficiencies
This retrospective view of your code’s evolution can be an excellent learning tool, helping you grow as a developer more quickly.
3. Collaboration Skills
In the real world of software development, collaboration is key. Git is the foundation of collaborative coding, and learning it early will prepare you for working in teams. You’ll gain experience with:
- Merging code from different contributors
- Resolving conflicts when multiple people work on the same file
- Using pull requests to review and discuss code changes
These skills are invaluable in any professional setting and will make you a more attractive candidate to potential employers.
4. Portfolio Building
As a beginner, building a portfolio is crucial for showcasing your skills to potential employers or clients. Git, coupled with platforms like GitHub or GitLab, provides an excellent way to:
- Host your projects online for free
- Demonstrate your coding activity through your commit history
- Collaborate on open-source projects to gain real-world experience
By learning Git early, you can start building your portfolio from day one, giving you a head start in your job search.
5. Industry Standard Practice
Git is not just a tool; it’s an industry standard. Nearly every software development company uses Git or a similar version control system. By learning Git early, you’re:
- Aligning yourself with industry best practices
- Making yourself more marketable to potential employers
- Preparing for real-world development scenarios
This familiarity with industry standards will give you a significant advantage when you start applying for jobs or internships.
6. Backup and Recovery
One of the most practical benefits of using Git is its ability to serve as a backup system for your code. This is particularly important for beginners who might accidentally delete important files or make irreversible changes. With Git, you can:
- Recover deleted files from previous commits
- Undo changes that broke your code
- Maintain multiple versions of your project simultaneously
This safety net allows you to code with confidence, knowing that your work is always protected.
7. Enhanced Problem-Solving Skills
Learning Git involves more than just memorizing commands. It requires you to think about your code in terms of logical chunks of work (commits) and how different parts of your project relate to each other (branches). This mindset can enhance your problem-solving skills by:
- Encouraging you to break down large tasks into smaller, manageable pieces
- Teaching you to think about the dependencies and relationships within your code
- Helping you visualize the structure of your project more clearly
These skills translate directly to writing better, more organized code in general.
8. Preparation for Advanced Concepts
As you progress in your coding journey, you’ll encounter more advanced concepts and tools. Many of these build upon the foundational knowledge provided by Git. For example:
- Continuous Integration/Continuous Deployment (CI/CD) pipelines often integrate with Git
- Advanced branching strategies like GitFlow are based on Git’s branching model
- Code review processes in professional settings typically leverage Git’s features
By learning Git early, you’re laying the groundwork for understanding these more complex topics later on.
Getting Started with Git
Now that we’ve explored the benefits, you might be wondering how to get started with Git. Here’s a quick guide to help you begin:
1. Install Git
First, you’ll need to install Git on your computer. You can download it from the official Git website: https://git-scm.com/downloads
2. Configure Git
After installation, you’ll need to configure Git with your name and email address. Open a terminal or command prompt and run the following commands:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
3. Create Your First Repository
To create a new Git repository, navigate to your project directory in the terminal and run:
git init
4. Make Your First Commit
After making some changes to your files, you can stage them for commit using:
git add .
Then, commit the changes with a descriptive message:
git commit -m "Initial commit: Add project files"
5. Create a GitHub Account
To take full advantage of Git’s collaborative features, create an account on GitHub: https://github.com/
6. Push Your Repository to GitHub
Create a new repository on GitHub, then push your local repository to it using:
git remote add origin https://github.com/yourusername/your-repo-name.git
git branch -M main
git push -u origin main
Essential Git Commands for Beginners
As you start using Git, there are several commands you’ll use frequently. Here’s a quick reference:
git status
: Check the status of your working directorygit add
: Stage changes for commitgit commit
: Create a new commit with staged changesgit push
: Upload local repository content to a remote repositorygit pull
: Fetch and download content from a remote repositorygit branch
: List, create, or delete branchesgit checkout
: Switch branches or restore working tree filesgit merge
: Merge one or more branches into your current branch
Best Practices for Using Git
As you incorporate Git into your coding workflow, keep these best practices in mind:
1. Commit Often
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and revert if necessary.
2. Write Clear Commit Messages
Use descriptive commit messages that explain what changes were made and why. This helps you and others understand the project’s history.
3. Use Branches
Create separate branches for different features or experiments. This keeps your main branch clean and makes it easier to manage multiple aspects of your project.
4. Review Before Committing
Always review your changes before committing. Use git diff
to see what changes you’re about to commit.
5. Keep Your Repository Clean
Use a .gitignore
file to exclude unnecessary files (like build artifacts or IDE-specific files) from version control.
Overcoming Common Git Challenges
As a beginner, you might encounter some challenges when using Git. Here are some common issues and how to address them:
1. Merge Conflicts
Merge conflicts occur when Git can’t automatically merge changes. To resolve:
- Open the conflicting files and look for the conflict markers (<<<<<<<, =======, >>>>>>>)
- Manually edit the files to resolve the conflicts
- Stage the resolved files with
git add
- Complete the merge with
git commit
2. Accidentally Committing to the Wrong Branch
If you commit to the wrong branch, you can move the commit to the correct branch using:
git checkout correct-branch
git cherry-pick commit-hash
git checkout original-branch
git reset --hard HEAD~1
3. Undoing Changes
To undo uncommitted changes in your working directory:
git checkout -- filename
To undo the last commit while keeping the changes:
git reset --soft HEAD~1
Advanced Git Features to Explore
As you become more comfortable with Git, consider exploring these advanced features:
1. Interactive Rebasing
Interactive rebasing allows you to modify a series of commits. It’s useful for cleaning up your commit history before merging or pushing.
git rebase -i HEAD~3
2. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They can be used to enforce coding standards or run tests automatically.
3. Git Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository. This is useful for including external libraries or shared components in your project.
4. Git Bisect
Git bisect helps you find the commit that introduced a bug by performing a binary search through your commit history.
git bisect start
git bisect bad
git bisect good commit-hash
Integrating Git with Your Development Workflow
As you progress in your coding journey, you’ll want to integrate Git more deeply into your development workflow. Here are some ways to do that:
1. Use Git with Your IDE
Most modern Integrated Development Environments (IDEs) have built-in Git support. Familiarize yourself with your IDE’s Git features to streamline your workflow.
2. Implement a Branching Strategy
Adopt a branching strategy like GitFlow or GitHub Flow to organize your development process, especially when working on larger projects or in teams.
3. Automate with Git Aliases
Create Git aliases for commands you use frequently. For example:
git config --global alias.co checkout
git config --global alias.br branch
git config --global alias.ci commit
git config --global alias.st status
4. Use Git in Continuous Integration
Integrate Git with CI/CD tools like Jenkins, Travis CI, or GitHub Actions to automate testing and deployment processes.
Conclusion
Learning Git early in your coding journey is an investment that will pay dividends throughout your career. It not only provides you with a powerful tool for managing your code but also aligns you with industry best practices, enhances your problem-solving skills, and prepares you for collaborative development environments.
As you continue to grow as a developer, Git will remain a constant companion, evolving with you and supporting your increasingly complex projects and workflows. By mastering Git early, you’re setting yourself up for success and demonstrating to potential employers that you’re serious about your craft.
Remember, like any skill, proficiency with Git comes with practice. Don’t be discouraged if you encounter challenges along the way. Each merge conflict resolved or complex rebase completed is a step towards becoming a more competent and confident developer.
So, as you embark on your coding journey, make learning Git a priority. It’s more than just a version control system; it’s a fundamental skill that will shape how you approach software development and collaboration throughout your career. Happy coding, and may your commits always be clear, your merges smooth, and your repositories well-organized!