Using GitFlow: A Detailed Guide to This Branching Model
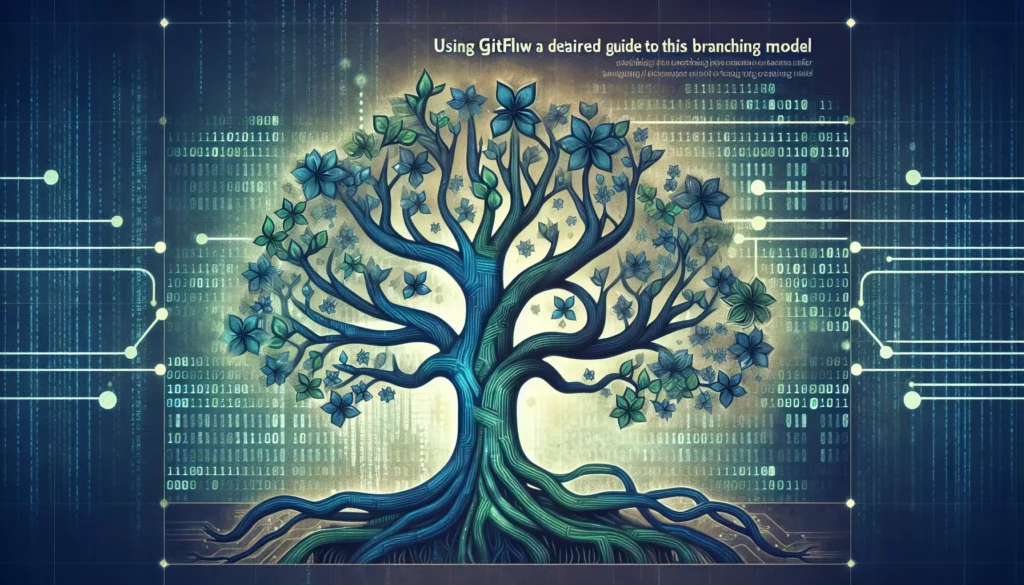
In the world of software development, version control is crucial for managing code changes, collaborating with team members, and maintaining a stable codebase. Git, the most widely used version control system, offers various branching strategies to organize development workflows. One popular and effective branching model is GitFlow. In this comprehensive guide, we’ll explore GitFlow in detail, discussing its principles, benefits, and how to implement it in your projects.
What is GitFlow?
GitFlow is a branching model for Git, created by Vincent Driessen in 2010. It defines a strict branching structure designed around project releases, providing a robust framework for managing larger projects. GitFlow is particularly well-suited for projects that have a scheduled release cycle and for teams practicing continuous delivery.
The core idea behind GitFlow is to isolate your work into different types of branches. There are five different branch types:
- Master
- Develop
- Feature
- Release
- Hotfix
Each of these branches has a specific purpose and strict rules as to which branches may be their originating branch and which branches must be their merge targets.
The Main Branches
In the GitFlow model, there are two main branches with infinite lifetime:
1. Master Branch
The master branch stores the official release history. All code in the master branch should be production-ready.
2. Develop Branch
The develop branch serves as an integration branch for features. It contains the complete history of the project, whereas master contains an abridged version. When the source code in the develop branch reaches a stable point and is ready to be released, all changes should be merged back into master and tagged with a release number.
Supporting Branches
GitFlow uses a variety of supporting branches to aid parallel development, ease tracking of features, prepare for production releases, and assist in quickly fixing live production problems. Unlike the main branches, these branches always have a limited lifetime.
3. Feature Branches
Feature branches are used to develop new features for the upcoming or a distant future release. When starting development of a feature, the target release in which this feature will be incorporated may well be unknown at that point. The essence of a feature branch is that it exists as long as the feature is in development, but will eventually be merged back into develop or discarded.
Creating a feature branch
To create a feature branch, use the following Git command:
git checkout -b feature/my-feature develop
Finishing a feature branch
When a feature is complete, it should be merged back into the develop branch. To do this, use:
git checkout develop
git merge --no-ff feature/my-feature
git branch -d feature/my-feature
git push origin develop
4. Release Branches
Release branches support preparation of a new production release. They allow for minor bug fixes and preparing meta-data for a release (version number, build dates, etc.). By doing all of this work on a release branch, the develop branch is cleared to receive features for the next big release.
Creating a release branch
Release branches are created from the develop branch. For example, say version 1.1.5 is the current production release and we have a big release coming up. The state of develop is ready for the “next release” and we have decided that this will become version 1.2 (rather than 1.1.6 or 2.0). So we branch off and give the release branch a name reflecting the new version number:
git checkout -b release-1.2 develop
./bump-version.sh 1.2
git commit -a -m "Bumped version number to 1.2"
Finishing a release branch
When the state of the release branch is ready to become a real release, some actions need to be carried out. First, the release branch is merged into master (since every commit on master is a new release by definition). Next, that commit on master must be tagged for easy future reference to this historical version. Finally, the changes made on the release branch need to be merged back into develop, so that future releases also contain these bug fixes.
git checkout master
git merge --no-ff release-1.2
git tag -a 1.2
git checkout develop
git merge --no-ff release-1.2
git branch -d release-1.2
5. Hotfix Branches
Hotfix branches are very much like release branches in that they are also meant to prepare for a new production release, albeit unplanned. They arise from the necessity to act immediately upon an undesired state of a live production version. When a critical bug in a production version must be resolved immediately, a hotfix branch may be branched off from the corresponding tag on the master branch that marks the production version.
Creating a hotfix branch
Hotfix branches are created from the master branch. For example, say version 1.2 is the current production release running live and causing troubles due to a severe bug. But changes on develop are yet unstable. We may then branch off a hotfix branch and start fixing the problem:
git checkout -b hotfix-1.2.1 master
./bump-version.sh 1.2.1
git commit -a -m "Bumped version number to 1.2.1"
# Fix the bug and commit the fix
git commit -m "Fixed severe production problem"
Finishing a hotfix branch
When finished, the bugfix needs to be merged back into master, but also needs to be merged back into develop, in order to safeguard that the bugfix is included in the next release as well. This is completely similar to how release branches are finished.
git checkout master
git merge --no-ff hotfix-1.2.1
git tag -a 1.2.1
git checkout develop
git merge --no-ff hotfix-1.2.1
git branch -d hotfix-1.2.1
Benefits of Using GitFlow
GitFlow offers several advantages for development teams:
- Parallel Development: GitFlow makes parallel development very easy by isolating new development from finished work.
- Collaboration: Feature branches make it easy for two or more developers to collaborate on the same feature, while keeping changes isolated.
- Release Staging Area: As new development is completed, it gets merged back into the develop branch, which is a staging area for all completed features that haven’t yet been released.
- Support for Emergency Fixes: GitFlow supports hotfix branches – branches made from a tagged release. You can use these to make an emergency change, safe in the knowledge that the hotfix will only contain your emergency fix.
- Clear Structure: The defined structure of GitFlow makes it easier for team members to understand the workflow and current stage of the project.
Implementing GitFlow in Your Project
To start using GitFlow in your project, you can either use the git-flow command line tools or implement the flow manually using standard Git commands. Here’s how to set up GitFlow using the command line tools:
1. Install git-flow
On macOS with Homebrew:
brew install git-flow-avh
On Linux:
apt-get install git-flow
2. Initialize git-flow in your repository
Navigate to your Git repository and run:
git flow init
This command will ask you a series of questions about which branches you want to use for development and production, and how you want your prefixes named. You can press Enter to accept the defaults.
3. Start a new feature
git flow feature start feature_name
4. Finish a feature
git flow feature finish feature_name
5. Start a release
git flow release start 0.1.0
6. Finish a release
git flow release finish '0.1.0'
7. Start a hotfix
git flow hotfix start hotfix_branch
8. Finish a hotfix
git flow hotfix finish hotfix_branch
Best Practices When Using GitFlow
While GitFlow provides a solid structure for managing your Git branches, here are some best practices to ensure smooth implementation:
- Commit Often: Make small, frequent commits. This makes it easier to track changes and rollback if necessary.
- Use Descriptive Commit Messages: Write clear, concise commit messages that explain what changes were made and why.
- Keep Your Branches Updated: Regularly merge changes from the develop branch into your feature branches to avoid large, complex merges later.
- Delete Old Branches: Once a feature or hotfix branch has been merged, delete it to keep your repository clean.
- Use Pull Requests: Even if you’re using GitFlow, pull requests can add an extra layer of code review before merging features into develop.
- Tag Your Releases: Always tag your releases in the master branch for easy reference and rollback if needed.
- Don’t Break the Build: Ensure that the develop and master branches always contain production-ready code.
- Use GitFlow Commands: If you’re using the git-flow extension, stick to its commands for consistency.
GitFlow vs. Other Branching Models
While GitFlow is popular, it’s not the only branching model available. Let’s compare it with a few other common models:
GitFlow vs. GitHub Flow
GitHub Flow is a simpler alternative to GitFlow. It has only feature branches and a master branch. Features are merged directly into master, which is always deployable. This model is simpler but provides less structure for releases.
GitFlow vs. GitLab Flow
GitLab Flow is somewhat of a middle ground between GitFlow and GitHub Flow. It introduces environment branches (like production, pre-production) in addition to the master branch, providing some extra structure without the full complexity of GitFlow.
GitFlow vs. Trunk-Based Development
Trunk-Based Development involves making smaller, more frequent commits directly to the master branch (or a develop branch in some variations). This model emphasizes continuous integration but can be challenging for larger teams or projects with longer release cycles.
When to Use GitFlow
GitFlow is particularly well-suited for:
- Projects with a scheduled release cycle
- Large teams working on a single project
- Projects that require maintaining multiple versions in production
However, for smaller projects or teams practicing continuous deployment, a simpler model like GitHub Flow might be more appropriate.
Conclusion
GitFlow provides a robust framework for managing complex projects with multiple developers and scheduled releases. By clearly defining branch roles and providing strict rules for how changes flow between branches, GitFlow helps teams maintain a clean, organized repository and streamline their development process.
While it may seem complex at first, the structure it provides can be invaluable as projects and teams grow. However, it’s important to remember that no single workflow is perfect for every project. Teams should evaluate their specific needs and choose (or adapt) a Git workflow that best suits their requirements.
Whether you choose to adopt GitFlow or another branching model, the key is to establish clear processes, communicate effectively with your team, and consistently apply your chosen workflow. With practice and discipline, you’ll find that a well-implemented branching strategy like GitFlow can significantly enhance your team’s productivity and the quality of your software releases.