How to Conduct a Successful Code Review in GitHub
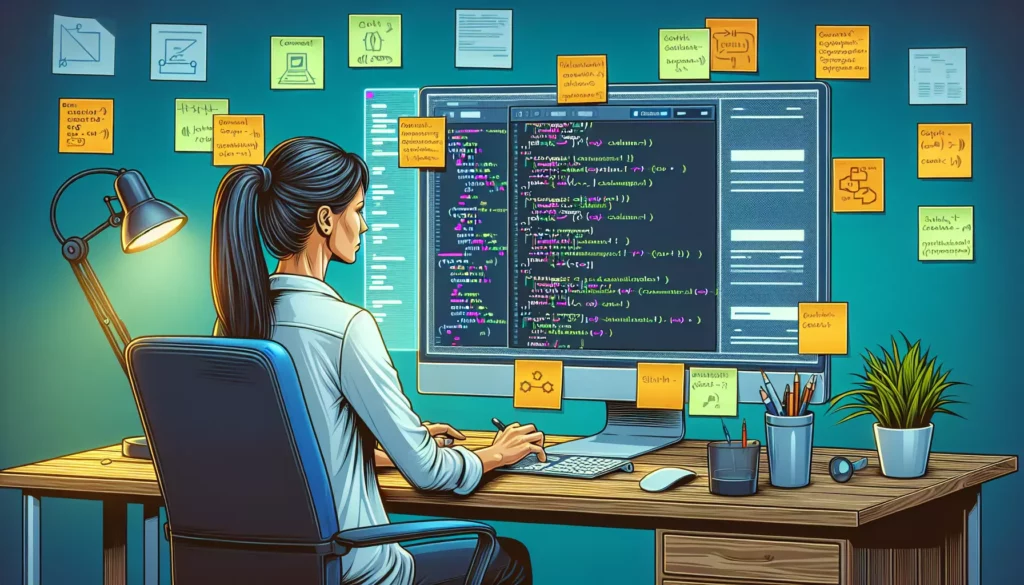
Code reviews are an essential part of the software development process, helping teams maintain code quality, share knowledge, and catch potential issues before they make it into production. GitHub, as one of the most popular platforms for version control and collaboration, offers powerful tools for conducting effective code reviews. In this comprehensive guide, we’ll explore the best practices for conducting successful code reviews in GitHub, from setting up the review process to providing constructive feedback.
Table of Contents
- Understanding Code Reviews
- Setting Up GitHub for Code Reviews
- Preparing for a Code Review
- Conducting the Code Review
- Providing Effective Feedback
- Responding to Feedback
- Resolving Conflicts and Merging
- Best Practices for Code Reviews
- Automated Code Review Tools
- Measuring the Impact of Code Reviews
- Conclusion
1. Understanding Code Reviews
Before diving into the specifics of conducting code reviews on GitHub, it’s crucial to understand what code reviews are and why they’re important.
What is a Code Review?
A code review is a systematic examination of source code by peers to identify bugs, improve code quality, and ensure adherence to coding standards and best practices. It’s a collaborative process where developers share their work with colleagues for feedback and suggestions.
Why are Code Reviews Important?
- Quality Assurance: Catch bugs and issues early in the development process.
- Knowledge Sharing: Spread knowledge about the codebase across the team.
- Consistency: Maintain coding standards and style guidelines.
- Mentorship: Provide learning opportunities for junior developers.
- Collaboration: Foster a culture of teamwork and shared responsibility.
2. Setting Up GitHub for Code Reviews
GitHub provides several features to facilitate code reviews. Here’s how to set up your repository for effective reviews:
Branch Protection Rules
Set up branch protection rules to ensure that code is reviewed before merging:
- Go to your repository’s Settings
- Click on “Branches” in the left sidebar
- Under “Branch protection rules,” click “Add rule”
- Enter the branch name pattern (e.g., “main” or “develop”)
- Check “Require pull request reviews before merging”
- Set the number of required approving reviews
- Optionally, enable other protection settings
- Click “Create” or “Save changes”
Pull Request Template
Create a pull request template to standardize the information provided with each PR:
- In your repository, create a new file named
PULL_REQUEST_TEMPLATE.md
- Place it in the root directory, or in a
.github
folder - Add the desired content to the template
Here’s an example of a simple pull request template:
## Description
[Provide a brief description of the changes in this PR]
## Related Issue
[Link to the related issue, if applicable]
## Type of Change
- [ ] Bug fix
- [ ] New feature
- [ ] Breaking change
- [ ] Documentation update
## How Has This Been Tested?
[Describe the tests you ran to verify your changes]
## Checklist:
- [ ] My code follows the style guidelines of this project
- [ ] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [ ] I have made corresponding changes to the documentation
- [ ] My changes generate no new warnings
- [ ] I have added tests that prove my fix is effective or that my feature works
- [ ] New and existing unit tests pass locally with my changes
3. Preparing for a Code Review
Before submitting your code for review, take these steps to ensure a smooth review process:
Self-Review
Conduct a thorough self-review of your code before submitting it for review. This includes:
- Checking for logical errors and bugs
- Ensuring code readability and maintainability
- Verifying adherence to coding standards and best practices
- Running tests and ensuring they pass
- Reviewing your commit history for clarity and coherence
Creating a Pull Request
When creating a pull request (PR) in GitHub:
- Give your PR a clear, descriptive title
- Fill out the PR template thoroughly
- Link to any related issues or documentation
- Provide context and rationale for your changes
- Highlight any areas where you’re particularly seeking feedback
Choosing Reviewers
Select appropriate reviewers for your PR:
- Choose reviewers familiar with the affected code or system
- Include a mix of senior and junior developers when possible
- Consider domain experts for specialized areas
- Avoid overloading the same reviewers repeatedly
4. Conducting the Code Review
When reviewing code on GitHub, follow these steps for a thorough and effective review:
Understanding the Context
- Read the PR description and any linked issues
- Understand the purpose and scope of the changes
- Review the commit history to see the evolution of the changes
Reviewing the Code
- Start with a high-level overview of the changes
- Look for logical errors, potential bugs, and edge cases
- Check for adherence to coding standards and best practices
- Evaluate code readability and maintainability
- Consider performance implications
- Verify test coverage and quality
Using GitHub’s Review Features
GitHub offers several features to streamline the review process:
- Line Comments: Click the “+” icon next to a line to add a comment
- Suggestions: Use the suggestion feature to propose specific code changes
- File View: Toggle between “Unified” and “Split” views for easier comparison
- Review Summary: Submit your review with comments, approval, or request changes
5. Providing Effective Feedback
The way you communicate feedback can greatly impact the effectiveness of the code review. Here are some tips for providing constructive feedback:
Be Specific and Actionable
Provide clear, specific feedback that the author can act upon. For example:
Instead of: "This function is confusing."
Try: "The function 'processData' could be more clear if we split it into smaller, more focused functions. For example, we could extract the data validation logic into a separate 'validateData' function."
Use a Constructive Tone
Frame your feedback in a positive, constructive manner:
Instead of: "This is wrong. You should use a HashMap."
Try: "Using a HashMap here could improve the performance of this operation. Have you considered this approach?"
Ask Questions
Use questions to encourage discussion and understand the author’s reasoning:
"What was the reasoning behind using a for-loop here instead of a stream operation? I'm curious if there are performance considerations I'm not aware of."
Provide Examples
When suggesting alternatives, provide examples or links to resources:
"We could simplify this code using the new Optional API. Here's an example of how it might look:
Optional.ofNullable(user)
.map(User::getName)
.orElse("Anonymous");
You can find more information about Optional in the Java documentation: [link]"
Acknowledge Good Work
Don’t forget to highlight positive aspects of the code:
"Great job on refactoring this class! The new structure is much more maintainable and easier to understand."
6. Responding to Feedback
As the author of the code being reviewed, responding to feedback appropriately is crucial for a productive review process:
Be Open to Feedback
- Approach feedback with an open mind
- Remember that the goal is to improve the code, not to criticize you personally
- View the review as a learning opportunity
Respond to All Comments
- Address each comment, even if it’s just to acknowledge it
- If you agree with a suggestion, implement the change and mark the comment as resolved
- If you disagree, explain your reasoning politely and clearly
Ask for Clarification
If you don’t understand a comment or suggestion, don’t hesitate to ask for clarification:
"I'm not sure I understand the suggestion to use a factory pattern here. Could you elaborate on how that would improve the code structure?"
Engage in Constructive Discussion
Use the review as an opportunity for meaningful discussion about code quality and design:
"That's an interesting suggestion to use dependency injection here. I hadn't considered that approach. Let's discuss the pros and cons of this change."
7. Resolving Conflicts and Merging
Once the review process is complete and changes have been approved, it’s time to merge the code. Here’s how to handle this final stage:
Resolving Merge Conflicts
If GitHub indicates that there are merge conflicts:
- Pull the latest changes from the base branch
- Merge the base branch into your feature branch locally
- Resolve conflicts in your local environment
- Push the resolved changes to your feature branch
- Update the pull request
Final Review
Before merging:
- Ensure all comments have been addressed
- Verify that all required approvals have been obtained
- Check that all CI/CD checks are passing
Merging Options
GitHub offers several merging options:
- Create a merge commit: Preserves the entire branch history
- Squash and merge: Combines all commits into a single commit
- Rebase and merge: Applies changes as if they were made directly on the base branch
Choose the option that best fits your team’s workflow and branching strategy.
8. Best Practices for Code Reviews
To maximize the effectiveness of your code reviews, consider adopting these best practices:
Review Regularly and Often
- Aim for small, frequent reviews rather than large, infrequent ones
- Set aside dedicated time for code reviews
- Try to review code within 24 hours of submission
Focus on Important Issues
- Prioritize logical errors, security issues, and performance problems
- Don’t get bogged down in minor style issues that can be handled by automated tools
- Look for patterns rather than nitpicking every small detail
Foster a Positive Review Culture
- Emphasize that reviews are about improving code, not criticizing the author
- Encourage both giving and receiving feedback gracefully
- Recognize and praise good code and improvements
Use Checklists
Develop and use checklists to ensure consistency in reviews. For example:
- [ ] Code adheres to our style guide
- [ ] Functions are small and focused
- [ ] Variable and function names are clear and descriptive
- [ ] Error handling is robust
- [ ] Unit tests cover critical paths
- [ ] Documentation is up-to-date
- [ ] No hardcoded secrets or sensitive information
- [ ] Performance considerations have been addressed
Pair Programming as a Complement
Consider using pair programming in addition to code reviews, especially for complex features or when onboarding new team members.
9. Automated Code Review Tools
While human review is irreplaceable, automated tools can significantly enhance the code review process. Here are some types of tools to consider integrating with your GitHub workflow:
Static Analysis Tools
- ESLint/TSLint: For JavaScript/TypeScript linting
- Rubocop: For Ruby code analysis
- PyLint: For Python code checking
- SonarQube: For multi-language code quality and security analysis
Code Formatters
- Prettier: For consistent formatting of JavaScript, CSS, and more
- Black: For Python code formatting
- gofmt: For Go code formatting
Security Scanners
- Dependabot: For checking dependencies for known vulnerabilities
- Snyk: For finding and fixing vulnerabilities in dependencies
- OWASP Dependency-Check: For identifying project dependencies and checking for known vulnerabilities
Test Coverage Tools
- Codecov: For tracking code coverage over time
- Coveralls: For displaying code coverage results and trends
Integrating Automated Tools with GitHub
To integrate these tools with your GitHub workflow:
- Set up the tools in your local development environment
- Configure them to run in your CI/CD pipeline (e.g., GitHub Actions)
- Enable status checks in your repository settings to require passing checks before merging
- Consider using GitHub Apps or Actions from the GitHub Marketplace for easy integration
10. Measuring the Impact of Code Reviews
To ensure your code review process is effective and to identify areas for improvement, consider tracking these metrics:
Quantitative Metrics
- Time to First Review: How long it takes for a PR to receive its first review
- Review Turnaround Time: The average time it takes to complete a review
- Number of Review Iterations: How many rounds of review a typical PR goes through
- Defect Density: The number of bugs found in production relative to the size of the codebase
- Code Churn: The rate at which code is rewritten or deleted after being introduced
Qualitative Metrics
- Code Quality Improvement: Subjective assessment of code quality over time
- Knowledge Sharing: Increased understanding of the codebase across the team
- Team Satisfaction: Developer satisfaction with the review process
Tools for Measuring Code Review Impact
- GitHub Insights: Provides metrics on pull requests and code review activity
- Code Climate: Offers code quality metrics and trends
- Pull Panda: Provides analytics on pull request and review performance
Continuous Improvement
Regularly review these metrics and gather feedback from your team to continuously improve your code review process. Consider holding retrospectives specifically focused on code reviews to identify pain points and successes.
11. Conclusion
Conducting successful code reviews in GitHub is a crucial skill for modern software development teams. By following the practices outlined in this guide, you can create a more efficient, collaborative, and educational review process that leads to higher quality code and a more knowledgeable team.
Remember that code reviews are not just about finding bugs or enforcing standards; they’re an opportunity for learning, mentorship, and collective ownership of the codebase. Embrace the process, be open to feedback, and continuously strive to improve both your code and your review skills.
As you implement these practices, keep in mind that every team is unique. Don’t be afraid to experiment and adapt these guidelines to fit your specific needs and culture. The most important thing is to maintain a positive, constructive atmosphere where everyone feels empowered to contribute to the quality and success of your software projects.
Happy coding and reviewing!