Best Practices for Using Git and GitHub in Team Projects
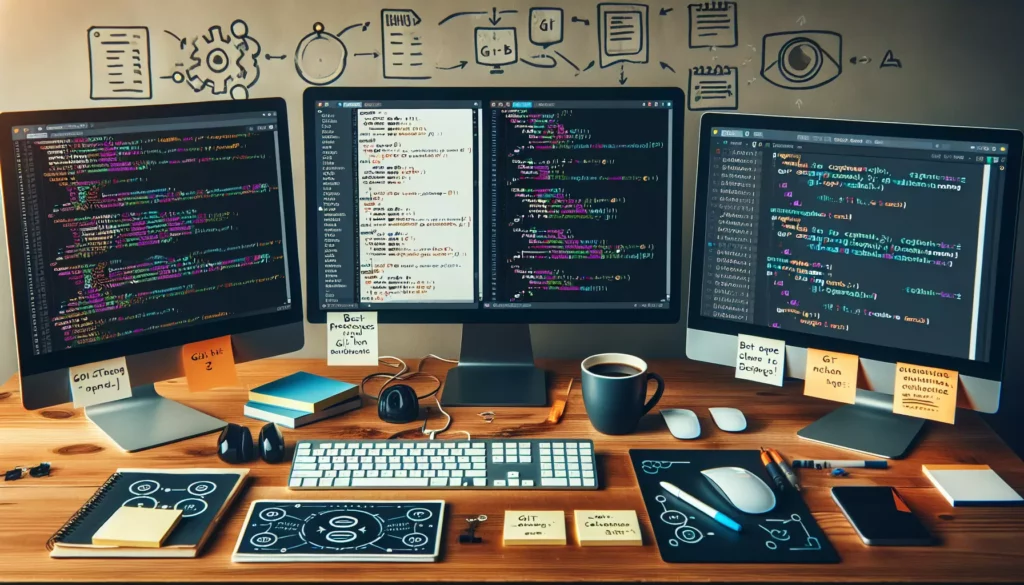
In the world of software development, collaboration is key. As projects grow in complexity and team sizes increase, having a robust version control system becomes crucial. Git, along with platforms like GitHub, has become the de facto standard for managing code repositories and facilitating teamwork. In this comprehensive guide, we’ll explore the best practices for using Git and GitHub in team projects, helping you streamline your workflow and boost productivity.
Understanding Git and GitHub
Before diving into best practices, let’s briefly review what Git and GitHub are:
- Git: A distributed version control system that allows developers to track changes in their code, create branches for experimentation, and merge changes from multiple contributors.
- GitHub: A web-based platform that provides hosting for Git repositories, along with additional collaboration features like pull requests, issue tracking, and project management tools.
Now, let’s explore the best practices that will help your team make the most of these powerful tools.
1. Establish a Clear Branching Strategy
A well-defined branching strategy is essential for maintaining a clean and organized repository. One popular approach is the GitFlow workflow, which uses the following branch types:
- Master: The main branch that contains production-ready code.
- Develop: The integration branch for ongoing development work.
- Feature: Branches for developing new features.
- Release: Branches for preparing releases.
- Hotfix: Branches for quick fixes to production issues.
Regardless of the specific strategy you choose, ensure that your team agrees on and consistently follows the chosen approach.
2. Write Meaningful Commit Messages
Clear and descriptive commit messages are crucial for understanding the history of your project. Follow these guidelines for effective commit messages:
- Use the imperative mood (e.g., “Add feature” instead of “Added feature”).
- Keep the first line short (ideally under 50 characters) and concise.
- Provide more detailed explanations in the commit body if necessary.
- Reference relevant issue numbers or pull requests.
Example of a good commit message:
Add user authentication feature
- Implement login and registration forms
- Set up JWT token-based authentication
- Create protected routes for authenticated users
Closes #123
3. Use Pull Requests for Code Reviews
Pull requests (PRs) are an excellent way to facilitate code reviews and discussions before merging changes into the main branch. Follow these best practices for pull requests:
- Create small, focused PRs that address a single feature or bug fix.
- Provide a clear description of the changes and their purpose.
- Include relevant screenshots or GIFs for UI changes.
- Assign reviewers and use GitHub’s review features to discuss and resolve issues.
- Set up automated checks (e.g., continuous integration) to run on PRs.
4. Keep Your Repository Clean
Maintaining a clean repository helps improve performance and makes it easier for team members to navigate the codebase. Consider the following practices:
- Use a
.gitignore
file to exclude unnecessary files and directories from version control. - Regularly delete merged branches to reduce clutter.
- Use Git LFS (Large File Storage) for managing large binary files.
- Avoid committing sensitive information like API keys or passwords.
5. Leverage GitHub Actions for Automation
GitHub Actions allow you to automate various aspects of your development workflow. Some useful applications include:
- Running tests and linters on pull requests.
- Automatically deploying your application to staging or production environments.
- Generating documentation or release notes.
- Sending notifications to team communication channels.
Here’s an example of a simple GitHub Actions workflow that runs tests on pull requests:
<?xml version="1.0" encoding="UTF-8"?>
<workflow>
<name>Run Tests</name>
<on>
<pull_request>
<branches>
<branch>main</branch>
</branches>
</pull_request>
</on>
<jobs>
<job>
<name>test</name>
<runs-on>ubuntu-latest</runs-on>
<steps>
<step>
<uses>actions/checkout@v2</uses>
</step>
<step>
<name>Install dependencies</name>
<run>npm ci</run>
</step>
<step>
<name>Run tests</name>
<run>npm test</run>
</step>
</steps>
</job>
</jobs>
</workflow>
6. Use Semantic Versioning
Adopting semantic versioning (SemVer) for your project releases helps communicate the nature of changes to your users and other developers. The format is MAJOR.MINOR.PATCH, where:
- MAJOR version increments for incompatible API changes
- MINOR version increments for backwards-compatible new features
- PATCH version increments for backwards-compatible bug fixes
Use Git tags to mark release versions in your repository:
git tag -a v1.0.0 -m "Release version 1.0.0"
git push origin v1.0.0
7. Document Your Project
Proper documentation is crucial for onboarding new team members and maintaining the project long-term. Consider including the following in your repository:
- A comprehensive README.md file with project overview, setup instructions, and contribution guidelines.
- CONTRIBUTING.md file detailing the process for submitting contributions.
- In-code documentation and comments for complex functions or algorithms.
- API documentation for libraries or frameworks.
8. Utilize GitHub Issues for Task Management
GitHub Issues can serve as a powerful task management tool for your team. Best practices include:
- Using labels to categorize issues (e.g., bug, feature, documentation).
- Assigning issues to team members responsible for addressing them.
- Creating templates for bug reports and feature requests to ensure consistent information.
- Using milestones to group related issues and track progress towards project goals.
9. Implement Continuous Integration and Continuous Deployment (CI/CD)
Setting up a CI/CD pipeline can significantly improve your team’s productivity and code quality. Consider implementing the following:
- Automated testing on every push or pull request.
- Code quality checks using tools like ESLint, Prettier, or SonarQube.
- Automated deployment to staging environments for review.
- One-click deployments to production environments.
You can use GitHub Actions or integrate with third-party CI/CD tools like Jenkins, Travis CI, or CircleCI.
10. Protect Your Main Branch
To maintain the integrity of your main branch, enable branch protection rules in GitHub. Some recommended settings include:
- Requiring pull request reviews before merging.
- Requiring status checks to pass before merging.
- Enforcing signed commits.
- Restricting who can push to the branch.
11. Use GitHub Projects for Project Management
GitHub Projects provides a flexible way to organize and track your team’s work. You can create custom boards with columns representing different stages of your workflow (e.g., To Do, In Progress, Review, Done). This visual representation helps team members understand the current state of the project and prioritize tasks effectively.
12. Leverage GitHub Discussions for Team Communication
GitHub Discussions offers a dedicated space for team conversations that don’t necessarily belong in issues or pull requests. Use this feature for:
- Brainstorming new features or architectural decisions.
- Sharing knowledge and best practices.
- Collecting feedback from users or contributors.
- Announcing important project updates or releases.
13. Implement Code Owners
Use a CODEOWNERS file in your repository to automatically assign reviewers to pull requests based on the files changed. This ensures that the right team members review relevant code changes. Here’s an example CODEOWNERS file:
# Global code owners
* @global-owner1 @global-owner2
# Frontend code owners
/src/frontend/ @frontend-team
# Backend code owners
/src/backend/ @backend-team
# Documentation owners
*.md @docs-team
14. Use GitHub Security Features
Take advantage of GitHub’s built-in security features to protect your project:
- Enable Dependabot alerts to receive notifications about vulnerable dependencies.
- Use Dependabot version updates to automatically create pull requests for outdated dependencies.
- Configure GitHub Advanced Security features like code scanning and secret scanning (available for GitHub Enterprise).
- Regularly review and update your repository’s security settings.
15. Optimize Your Git Workflow
Encourage your team to adopt efficient Git practices:
- Use
git fetch
andgit rebase
instead ofgit pull
to keep commit history clean. - Utilize interactive rebasing to clean up commits before pushing to remote.
- Learn and use Git aliases for frequently used commands.
- Understand and leverage Git hooks for automating tasks like code formatting or running tests before commits.
16. Conduct Regular Repository Maintenance
Periodically perform maintenance tasks to keep your repository healthy:
- Archive or delete old, unused branches.
- Review and update project dependencies.
- Optimize repository size by removing large files from Git history if necessary.
- Update documentation to reflect any changes in project structure or processes.
17. Encourage Collaboration and Knowledge Sharing
Foster a culture of collaboration within your team:
- Encourage pair programming sessions for complex tasks.
- Organize code review sessions to discuss best practices and share knowledge.
- Create and maintain a team wiki or knowledge base for documenting processes and solutions to common problems.
- Celebrate team achievements and recognize individual contributions.
Conclusion
Implementing these best practices for using Git and GitHub in team projects will help your team work more efficiently, maintain high code quality, and foster a collaborative development environment. Remember that these practices should be tailored to your team’s specific needs and continuously refined based on feedback and experience.
By leveraging the full power of Git and GitHub, your team can focus on what matters most: building great software and solving complex problems. As you continue to grow and evolve as a development team, don’t forget to periodically review and update your practices to ensure they’re still serving your needs effectively.
Happy coding, and may your repositories always be clean and your merges conflict-free!