How to Leverage Git for Managing Multiple Versions of Your Codebase
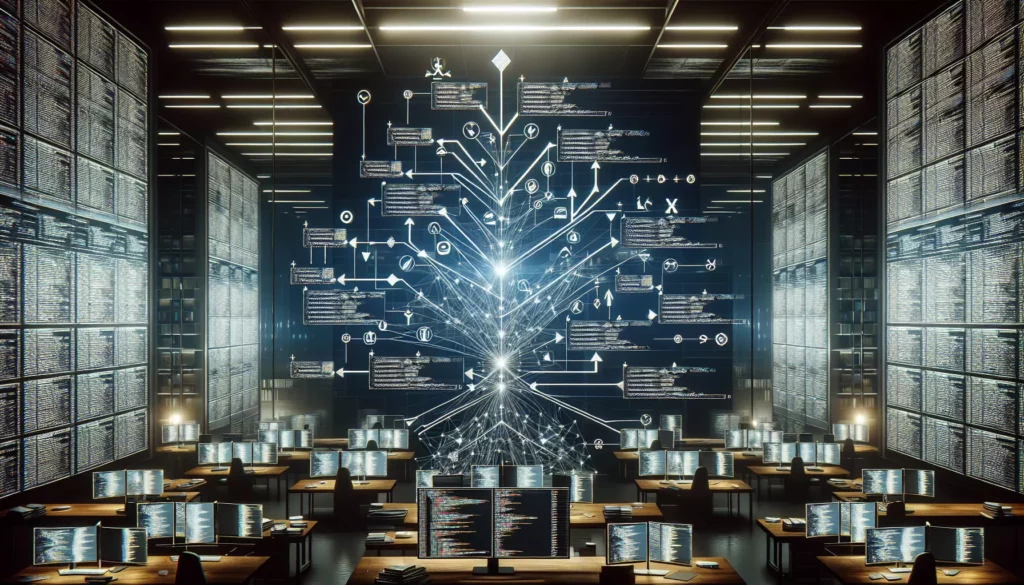
In the world of software development, managing multiple versions of your codebase is crucial for maintaining a smooth workflow, collaborating with team members, and keeping track of changes over time. Git, a distributed version control system, has become the go-to tool for developers worldwide to achieve these goals. In this comprehensive guide, we’ll explore how to leverage Git effectively to manage multiple versions of your codebase, enhancing your productivity and collaboration skills.
Table of Contents
- Introduction to Git
- Basic Git Concepts
- Setting Up Git
- Branching Strategies
- Merging and Resolving Conflicts
- Tagging Releases
- Working with Remote Repositories
- Advanced Git Techniques
- Best Practices for Version Management
- Git Tools and Integrations
- Conclusion
1. Introduction to Git
Git is a distributed version control system created by Linus Torvalds in 2005. It was designed to handle everything from small to very large projects with speed and efficiency. Git allows multiple developers to work on the same project simultaneously, tracking changes and managing different versions of the codebase.
Key features of Git include:
- Distributed development
- Strong support for non-linear development
- Efficient handling of large projects
- Cryptographic authentication of history
- Toolkit-based design
Understanding and leveraging Git’s capabilities can significantly improve your coding workflow and collaboration with other developers.
2. Basic Git Concepts
Before diving into advanced Git usage, it’s essential to understand some basic concepts:
Repository (Repo)
A repository is a directory where Git has been initialized to start version controlling your files. This is typically your project’s root folder.
Commit
A commit is a snapshot of your repository at a specific point in time. It includes all tracked changes since the last commit.
Branch
A branch is an independent line of development. It allows you to work on different features or experiments without affecting the main codebase.
Merge
Merging is the process of combining different branches into a single branch, typically to integrate new features into the main codebase.
Remote
A remote is a common repository that all team members use to exchange their changes. It’s typically hosted on a code hosting service like GitHub or GitLab.
3. Setting Up Git
To start using Git, you need to set it up on your local machine:
Installation
Download and install Git from the official Git website.
Configuration
After installation, configure your Git username and email:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Initializing a Repository
To start version-controlling a project, navigate to your project directory and run:
git init
This creates a new Git repository in your current directory.
4. Branching Strategies
Branching is a core concept in Git that allows you to diverge from the main line of development and continue to work without messing with that main line. Here are some common branching strategies:
Feature Branching
Create a new branch for each feature or bug fix:
git checkout -b feature/new-login-page
Git Flow
A more structured branching model that defines specific branch types:
- Master: for production-ready code
- Develop: for ongoing development
- Feature: for new features
- Release: for preparing new production releases
- Hotfix: for quick fixes to production code
GitHub Flow
A simpler alternative to Git Flow, focusing on regular deployments:
- Master branch should always be deployable
- Create feature branches from master
- Open a pull request early for discussion
- Merge to master after review and deploy immediately
5. Merging and Resolving Conflicts
Merging is the process of integrating changes from one branch into another. Sometimes, conflicts may arise when Git can’t automatically merge changes.
Basic Merging
To merge a feature branch into master:
git checkout master
git merge feature/new-login-page
Resolving Conflicts
If conflicts occur, Git will mark the conflicting areas in the affected files. You need to manually resolve these conflicts, then stage and commit the changes:
git add <conflicted-file>
git commit -m "Resolved merge conflict"
Pull Requests
In collaborative environments, it’s common to use pull requests (PRs) for merging. PRs allow for code review and discussion before changes are merged into the main branch.
6. Tagging Releases
Tags are references to specific points in Git history, typically used to mark release points. They provide a way to capture a point in history that is used for a marked version release.
Creating Tags
To create a lightweight tag:
git tag v1.0.0
For an annotated tag with a message:
git tag -a v1.0.0 -m "Release version 1.0.0"
Pushing Tags
Tags are not automatically pushed to remote repositories. To push tags:
git push origin v1.0.0
Or to push all tags:
git push origin --tags
7. Working with Remote Repositories
Remote repositories are versions of your project that are hosted on the Internet or network somewhere. Working with remotes allows you to collaborate with other developers.
Adding a Remote
To add a new remote:
git remote add origin https://github.com/username/repo.git
Fetching and Pulling
To fetch changes from a remote without merging:
git fetch origin
To fetch and merge changes:
git pull origin master
Pushing Changes
To push your local changes to a remote:
git push origin master
8. Advanced Git Techniques
As you become more comfortable with Git, you can leverage advanced techniques to manage your codebase more effectively:
Rebasing
Rebasing is an alternative to merging that can create a cleaner project history:
git checkout feature
git rebase master
Interactive Rebasing
Interactive rebasing allows you to modify commits as you move them to a new base:
git rebase -i HEAD~3
Cherry-picking
Cherry-picking allows you to pick specific commits from one branch and apply them to another:
git cherry-pick <commit-hash>
Stashing
Stashing allows you to temporarily store modified, tracked files in order to switch branches:
git stash
git stash pop
9. Best Practices for Version Management
To effectively manage multiple versions of your codebase, consider these best practices:
- Commit often and in logical units
- Write clear and concise commit messages
- Use branches for new features and bug fixes
- Keep the master branch stable and deployable
- Use pull requests for code review
- Tag important milestones and releases
- Keep your repository clean (use .gitignore)
- Regularly fetch and rebase with the main branch
- Use meaningful branch names (e.g., feature/user-authentication)
- Delete branches after merging
10. Git Tools and Integrations
While Git is powerful on its own, various tools and integrations can enhance your workflow:
GUI Clients
- GitKraken
- SourceTree
- GitHub Desktop
IDE Integrations
- VS Code Git integration
- IntelliJ IDEA Git integration
- Eclipse EGit
CI/CD Tools
- Jenkins
- Travis CI
- GitLab CI/CD
- GitHub Actions
Code Hosting Platforms
- GitHub
- GitLab
- Bitbucket
11. Conclusion
Leveraging Git for managing multiple versions of your codebase is an essential skill for modern software development. By mastering Git’s features and adopting best practices, you can streamline your workflow, collaborate more effectively with team members, and maintain a clean, organized codebase.
Remember that becoming proficient with Git takes time and practice. Start with the basics, gradually incorporate more advanced techniques, and don’t be afraid to experiment in a test repository. As you grow more comfortable with Git, you’ll find it an invaluable tool in your development toolkit.
For those preparing for technical interviews or looking to enhance their coding skills, understanding Git is crucial. Many technical interviews, especially for positions at major tech companies, may include questions about version control and collaborative coding practices. Platforms like AlgoCademy can provide additional resources and practice problems to help you master not only Git but also the algorithmic thinking and problem-solving skills needed for successful technical interviews.
By effectively leveraging Git, you’re not just managing your code – you’re setting yourself up for success in the dynamic and collaborative world of software development. Happy coding, and may your repositories always be conflict-free!