Why Learning GitHub Actions Can Improve Your Workflow
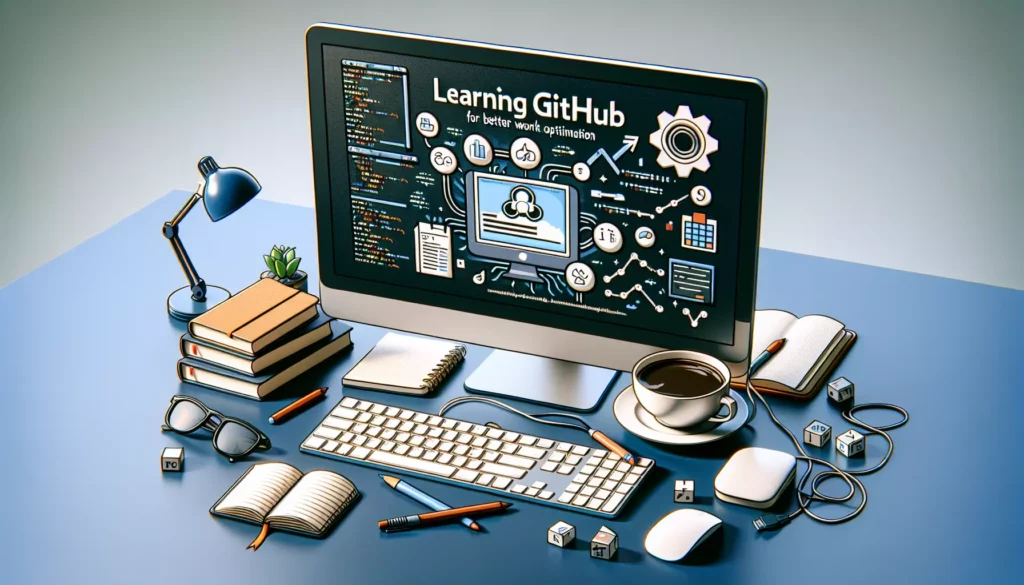
In the ever-evolving landscape of software development, staying ahead of the curve is crucial. As coding education platforms like AlgoCademy continue to emphasize the importance of practical skills and efficient workflows, one tool stands out as a game-changer: GitHub Actions. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, understanding and leveraging GitHub Actions can significantly enhance your development process and make you a more attractive candidate in the job market.
What are GitHub Actions?
GitHub Actions is a powerful automation tool integrated directly into the GitHub platform. It allows developers to create custom workflows that can be triggered by various events within their GitHub repositories. These workflows can automate tasks such as building, testing, and deploying code, as well as performing other operations like sending notifications or updating documentation.
At its core, GitHub Actions is designed to streamline the software development lifecycle, making it easier for individuals and teams to implement continuous integration and continuous deployment (CI/CD) practices.
Why GitHub Actions Matter for Your Coding Journey
As you progress through your coding education, whether through platforms like AlgoCademy or self-study, incorporating GitHub Actions into your skill set can provide numerous benefits:
1. Automating Repetitive Tasks
One of the primary advantages of GitHub Actions is its ability to automate repetitive tasks. As a developer, you’ll often find yourself performing the same actions repeatedly, such as running tests, linting code, or generating documentation. With GitHub Actions, you can create workflows that automatically execute these tasks whenever you push code to your repository or create a pull request.
For example, you could set up a workflow that automatically runs your test suite every time you push code:
name: Run Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
This simple workflow checks out your code, sets up Python, installs dependencies, and runs your tests automatically. By automating these tasks, you can focus more on writing code and solving problems, which is especially valuable when preparing for technical interviews.
2. Ensuring Code Quality
Maintaining high code quality is crucial, especially when you’re learning and trying to develop good habits. GitHub Actions can help enforce code quality standards by automatically running linters, formatters, and other code analysis tools. This not only helps you catch errors early but also ensures that your code adheres to best practices and style guidelines.
Here’s an example of a workflow that runs a linter on your Python code:
name: Lint Code
on: [push, pull_request]
jobs:
lint:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install flake8
- name: Run linter
run: flake8 .
By incorporating such workflows into your projects, you’ll develop a habit of writing clean, consistent code – a skill highly valued by potential employers.
3. Streamlining Collaboration
As you progress in your coding journey, you’ll likely start collaborating with others on projects. GitHub Actions can significantly improve the collaboration process by automating checks on pull requests. For instance, you can set up workflows that automatically review code, run tests, and provide feedback before a pull request is merged.
This not only speeds up the review process but also ensures that all code contributions meet the project’s standards. Here’s an example of a workflow that runs on pull requests:
name: Pull Request Checks
on:
pull_request:
branches: [ main ]
jobs:
check:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
- name: Run linter
run: flake8 .
This workflow ensures that all pull requests pass tests and meet coding standards before they can be merged, promoting a culture of quality and collaboration.
4. Continuous Integration and Deployment
Understanding and implementing CI/CD practices is crucial for modern software development. GitHub Actions makes it easy to set up CI/CD pipelines, allowing you to automatically build, test, and deploy your applications. This is particularly important when preparing for technical interviews or working on projects that simulate real-world development environments.
Here’s a basic example of a CI/CD workflow for a Python application:
name: CI/CD
on:
push:
branches: [ main ]
jobs:
build-and-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.x'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
- name: Deploy to production
if: success()
run: |
# Add your deployment steps here
echo "Deploying to production"
By implementing such workflows, you’ll gain practical experience with CI/CD concepts, making you better prepared for real-world development scenarios and technical interviews.
5. Learning DevOps Concepts
As you delve deeper into GitHub Actions, you’ll naturally start to encounter and understand various DevOps concepts. This includes understanding infrastructure as code, environment variables, secrets management, and more. These skills are increasingly in demand and can set you apart when applying for developer positions.
For instance, learning how to manage secrets securely in GitHub Actions is an important DevOps skill:
name: Use Secrets
on: [push]
jobs:
example-job:
runs-on: ubuntu-latest
steps:
- name: Use a secret
env:
SUPER_SECRET: ${{ secrets.SUPER_SECRET }}
run: |
echo "This step uses a secret"
In this example, you’re learning how to securely use sensitive information like API keys or passwords in your workflows without exposing them in your code.
Integrating GitHub Actions into Your Learning Journey
Now that we’ve explored the benefits of GitHub Actions, let’s discuss how you can integrate this tool into your coding education:
1. Start with Simple Workflows
Begin by creating simple workflows for your existing projects. Start with basic tasks like running tests or linting code. As you become more comfortable, gradually add more complex actions to your workflows.
2. Explore the GitHub Actions Marketplace
GitHub provides a marketplace with thousands of pre-built actions that you can incorporate into your workflows. Explore this marketplace to find actions that can help automate various aspects of your development process.
3. Practice with Real-World Scenarios
As you learn new concepts on platforms like AlgoCademy, try to implement them using GitHub Actions. For example, if you’re learning about unit testing, create a workflow that automatically runs your tests on every push.
4. Contribute to Open Source Projects
Many open-source projects use GitHub Actions. Contributing to these projects can give you real-world experience with collaborative workflows and CI/CD practices.
5. Document Your Learning
As you experiment with GitHub Actions, document your learning process. This can be valuable when discussing your skills in interviews or when collaborating with others.
GitHub Actions and Technical Interviews
When preparing for technical interviews, especially for positions at major tech companies, demonstrating proficiency with tools like GitHub Actions can set you apart from other candidates. Here’s how:
1. Showcasing Real-World Skills
By incorporating GitHub Actions into your projects, you demonstrate that you understand modern development practices. This shows potential employers that you’re capable of working in a professional development environment.
2. Efficiency and Automation
Your ability to automate repetitive tasks shows that you value efficiency and can optimize workflows. This is a highly desirable trait in the fast-paced tech industry.
3. Problem-Solving Abilities
Creating effective GitHub Actions workflows requires problem-solving skills. You need to think about the logic of your workflow, handle potential errors, and optimize for performance. These are all skills that interviewers look for.
4. Continuous Learning
By adopting tools like GitHub Actions, you demonstrate a commitment to continuous learning and staying up-to-date with industry trends. This is crucial in the ever-evolving field of software development.
5. Collaborative Skills
Understanding how to use GitHub Actions in a team setting shows that you have experience with collaborative development practices, a key skill for any software developer.
Advanced GitHub Actions Concepts
As you become more comfortable with basic GitHub Actions, you can explore more advanced concepts to further enhance your skills:
1. Matrix Builds
Matrix builds allow you to test your code across multiple versions or configurations simultaneously. This is particularly useful for ensuring your code works across different environments:
name: Matrix Build
on: [push]
jobs:
test:
runs-on: ubuntu-latest
strategy:
matrix:
python-version: [3.7, 3.8, 3.9]
steps:
- uses: actions/checkout@v2
- name: Set up Python ${{ matrix.python-version }}
uses: actions/setup-python@v2
with:
python-version: ${{ matrix.python-version }}
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: python -m unittest discover tests
This workflow tests your code against Python versions 3.7, 3.8, and 3.9 simultaneously, ensuring compatibility across different versions.
2. Conditional Workflows
You can create workflows that only run under certain conditions. This is useful for optimizing your CI/CD process:
name: Conditional Workflow
on:
push:
paths:
- '**.py'
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run tests
run: python -m unittest discover tests
This workflow only runs when Python files are changed, saving resources by not running unnecessary tests.
3. Creating Custom Actions
While the GitHub Marketplace offers many pre-built actions, you can also create your own custom actions for specific tasks. This is a great way to modularize common tasks in your workflows:
name: "Custom Action"
description: "A custom action"
inputs:
who-to-greet:
description: "Who to greet"
required: true
default: "World"
outputs:
time:
description: "The time we greeted you"
runs:
using: "docker"
image: "Dockerfile"
args:
- ${{ inputs.who-to-greet }}
This is a simple custom action that greets a user and outputs the time. Creating custom actions demonstrates advanced knowledge of GitHub Actions and can be a great talking point in interviews.
4. Environment and Deployment Management
GitHub Actions allows you to manage different environments (e.g., staging, production) and control deployments:
name: Deploy
on:
push:
branches:
- main
jobs:
deploy-to-staging:
runs-on: ubuntu-latest
environment: staging
steps:
- uses: actions/checkout@v2
- name: Deploy to staging
run: |
# Add your staging deployment steps here
deploy-to-production:
needs: deploy-to-staging
runs-on: ubuntu-latest
environment: production
steps:
- uses: actions/checkout@v2
- name: Deploy to production
run: |
# Add your production deployment steps here
This workflow demonstrates a deployment pipeline where code is first deployed to a staging environment, and then to production. Understanding how to manage different environments is crucial for real-world development scenarios.
Conclusion
Learning GitHub Actions is more than just adding another tool to your toolkit. It’s about embracing modern development practices, improving your workflow efficiency, and preparing yourself for the challenges of professional software development. As you continue your coding education journey with platforms like AlgoCademy, incorporating GitHub Actions into your learning process can provide you with practical, real-world skills that are highly valued in the industry.
Remember, the key to mastering GitHub Actions, like any other skill, is practice. Start small, experiment with different workflows, and gradually increase the complexity as you become more comfortable. By the time you’re ready for technical interviews at major tech companies, you’ll have a solid understanding of CI/CD practices, automation, and collaborative development – all crucial skills for today’s software engineers.
So, don’t wait. Start exploring GitHub Actions today and take your coding skills to the next level. Your future self (and potential employers) will thank you for it!