The Importance of Code Reviews in Collaborative Projects
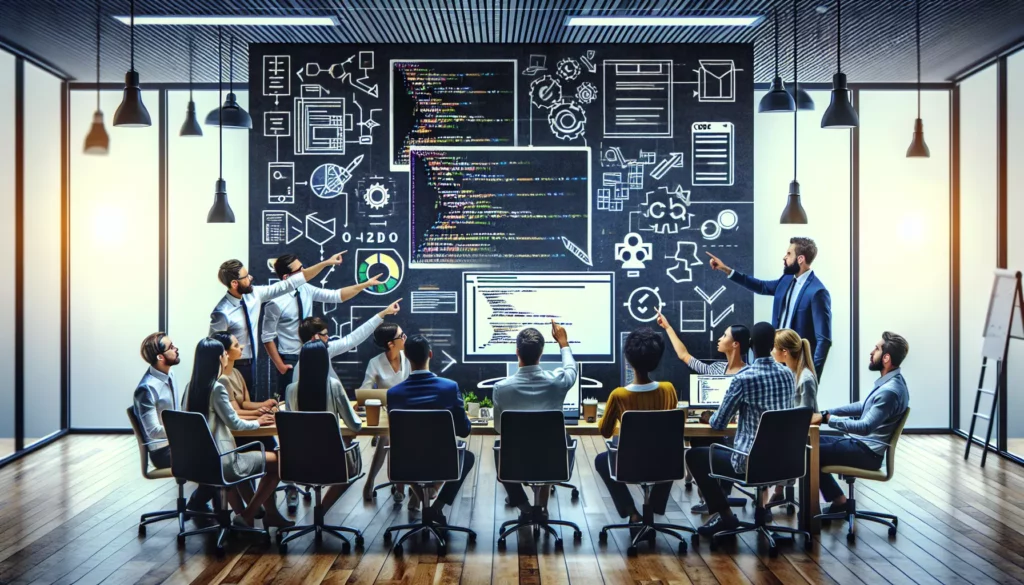
In the world of software development, collaboration is key to creating robust, efficient, and maintainable code. One of the most crucial practices in collaborative coding is the code review process. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at major tech companies, understanding and participating in code reviews is essential for your growth and success. In this comprehensive guide, we’ll explore the importance of code reviews in collaborative projects, their benefits, best practices, and how they contribute to overall code quality and team dynamics.
What is a Code Review?
A code review is a systematic examination of source code by peers or senior developers. It’s a quality assurance practice where developers other than the author inspect the code for bugs, logic errors, and adherence to coding standards. Code reviews can be conducted in various ways, including pair programming, over-the-shoulder reviews, or through dedicated tools and platforms.
The Benefits of Code Reviews
1. Improved Code Quality
One of the primary benefits of code reviews is the improvement in overall code quality. When multiple eyes examine a piece of code, it’s more likely that bugs, inefficiencies, and potential issues will be caught before they make it into production. This leads to more robust and reliable software.
2. Knowledge Sharing
Code reviews provide an excellent opportunity for knowledge sharing within a team. Junior developers can learn from more experienced colleagues, while senior developers can gain fresh perspectives from newer team members. This cross-pollination of ideas and techniques helps to elevate the entire team’s skill level.
3. Consistency in Coding Standards
Through code reviews, teams can ensure that coding standards and best practices are consistently applied across the project. This leads to more maintainable and readable code, which is crucial for long-term project success.
4. Early Bug Detection
Catching bugs early in the development process is far more cost-effective than fixing them in later stages or after deployment. Code reviews serve as an additional layer of testing, helping to identify and resolve issues before they become more significant problems.
5. Improved Team Collaboration
Regular code reviews foster a culture of collaboration and open communication within development teams. They provide a structured way for team members to interact, share ideas, and work together towards common goals.
Best Practices for Effective Code Reviews
1. Set Clear Objectives
Before starting a code review, it’s essential to establish clear objectives. Are you looking for bugs, checking for adherence to coding standards, or evaluating the overall design? Having a clear focus helps reviewers provide more targeted and valuable feedback.
2. Keep Reviews Small and Frequent
Large code reviews can be overwhelming and time-consuming. Aim for smaller, more frequent reviews to make the process more manageable and to catch issues earlier in the development cycle.
3. Use Automated Tools
Leverage automated code review tools to handle routine checks like code formatting, style violations, and potential security issues. This allows human reviewers to focus on more complex aspects of the code.
4. Foster a Positive Environment
Code reviews should be constructive, not confrontational. Encourage a positive atmosphere where feedback is given and received professionally and respectfully. Remember, the goal is to improve the code, not criticize the developer.
5. Follow a Checklist
Develop a code review checklist tailored to your team’s needs. This ensures consistency across reviews and helps catch common issues. Here’s a sample checklist:
- Does the code follow the project’s style guide?
- Are there any obvious logic errors in the code?
- Are all edge cases handled?
- Is the code sufficiently documented?
- Are there any performance concerns?
- Is the code DRY (Don’t Repeat Yourself)?
- Are security best practices followed?
Code Review Tools and Platforms
Several tools and platforms can streamline the code review process. Some popular options include:
- GitHub Pull Requests
- GitLab Merge Requests
- Bitbucket Pull Requests
- Gerrit
- Crucible
- Review Board
These tools often integrate with version control systems and provide features like inline commenting, approval workflows, and integration with continuous integration/continuous deployment (CI/CD) pipelines.
The Code Review Process
A typical code review process might look like this:
- The developer completes a feature or bug fix and creates a pull request.
- One or more reviewers are assigned to the pull request.
- Reviewers examine the code, leaving comments and suggestions.
- The original developer addresses the feedback, making necessary changes.
- Reviewers re-examine the updated code.
- Once all issues are resolved, the code is approved and merged into the main branch.
Common Challenges in Code Reviews and How to Overcome Them
1. Time Constraints
Code reviews can be time-consuming, especially for large projects. To address this:
- Prioritize reviews based on the complexity and impact of the changes.
- Implement a rotation system to distribute the review workload among team members.
- Set aside dedicated time for code reviews in your team’s schedule.
2. Ego and Defensiveness
Sometimes, developers may feel defensive about their code. To mitigate this:
- Foster a culture of constructive feedback and continuous improvement.
- Focus on the code, not the person.
- Encourage developers to view code reviews as learning opportunities.
3. Lack of Context
Reviewers may not always have full context about the changes. To address this:
- Provide clear descriptions and context in pull requests.
- Link to relevant issues or documentation.
- Encourage questions and discussions during the review process.
Code Reviews and Algorithmic Thinking
For those focusing on algorithmic thinking and preparing for technical interviews, code reviews can be particularly valuable. They provide opportunities to:
- Analyze different approaches to solving problems
- Discuss time and space complexity of solutions
- Explore edge cases and potential optimizations
- Practice explaining and defending technical decisions
Consider the following example of a simple algorithm to find the maximum element in an array:
def find_max(arr):
if not arr:
return None
max_val = arr[0]
for num in arr[1:]:
if num > max_val:
max_val = num
return max_val
In a code review, you might discuss:
- The time complexity of O(n) and why it’s optimal for this problem
- The space complexity of O(1) and how it’s achieved
- How the function handles edge cases like an empty array
- Potential improvements or alternative implementations
Code Reviews and FAANG-style Interview Preparation
For those aiming to work at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), participating in and conducting code reviews can be excellent preparation for technical interviews. Here’s why:
1. Problem-Solving Skills
Code reviews often involve analyzing solutions to complex problems, similar to what you might encounter in a technical interview. Regularly reviewing others’ code exposes you to different problem-solving approaches and helps you develop a critical eye for efficient solutions.
2. Communication Skills
Technical interviews at FAANG companies often include collaborative coding sessions or discussions about your approach. Code reviews help you practice articulating your thoughts about code, explaining design decisions, and discussing trade-offs – all crucial skills for these interviews.
3. Attention to Detail
FAANG interviews often test your ability to spot edge cases and potential issues in code. Regular participation in code reviews sharpens this skill as you learn to identify subtle bugs or inefficiencies in others’ code.
4. System Design Understanding
For more senior roles, FAANG interviews often include system design questions. Code reviews, especially for larger features or architectural changes, can help you develop a better understanding of how different components of a system interact.
Implementing Code Reviews in Your Learning Journey
If you’re a beginner or learning on your own, you might wonder how to incorporate code reviews into your learning process. Here are some suggestions:
1. Open Source Contributions
Contributing to open source projects is an excellent way to participate in code reviews. Many projects welcome contributions from beginners and have established code review processes.
2. Peer Learning Groups
Form or join a group of peers who are also learning to code. Share your projects and conduct code reviews for each other. This can be done in person or through online platforms.
3. Online Coding Platforms
Some online coding platforms and communities offer code review features. For example, CodePen has a Collab Mode where others can review and comment on your code.
4. Mentorship Programs
Look for mentorship programs where experienced developers can review your code and provide feedback. Many tech communities and bootcamps offer such opportunities.
5. Self-Review
While not as effective as peer reviews, you can practice self-review by revisiting your old code after some time. Try to approach it with fresh eyes and critically evaluate your past solutions.
Advanced Topics in Code Reviews
1. Code Review Metrics
As teams mature in their code review practices, they might start tracking metrics to improve the process. Some useful metrics include:
- Review turnaround time
- Number of comments per review
- Defect detection rate
- Code churn (amount of code changed after review)
These metrics can help teams identify bottlenecks in the review process and areas for improvement.
2. AI-Assisted Code Reviews
Artificial Intelligence is increasingly being used to augment human code reviews. AI tools can:
- Automatically detect common coding errors
- Suggest optimizations
- Identify potential security vulnerabilities
- Check for consistency with coding standards
While AI can’t replace human reviewers, it can significantly streamline the process by handling routine checks and allowing humans to focus on higher-level concerns.
3. Code Reviews in Different Development Methodologies
The approach to code reviews may vary depending on the development methodology used:
- Agile: In Agile methodologies, code reviews are often integrated into the daily workflow, with frequent, small reviews aligned with sprint cycles.
- Waterfall: In more traditional Waterfall approaches, code reviews might be more formal and conducted at specific phases of the development lifecycle.
- DevOps: In a DevOps environment, code reviews are often automated as much as possible and integrated into the CI/CD pipeline.
Conclusion
Code reviews are a fundamental practice in collaborative software development, offering numerous benefits from improved code quality to knowledge sharing and team collaboration. Whether you’re a beginner learning to code or an experienced developer preparing for technical interviews at major tech companies, mastering the art of code reviews will significantly enhance your skills and career prospects.
Remember, effective code reviews are not about finding fault, but about collective improvement. They’re an opportunity to learn, share knowledge, and work together towards creating better software. By embracing code reviews and implementing best practices, you’ll not only improve your own coding skills but also contribute to a more collaborative and efficient development environment.
As you continue your coding journey, whether you’re working on personal projects, contributing to open source, or preparing for technical interviews, make code reviews an integral part of your process. The skills you develop through participating in and conducting code reviews will serve you well throughout your career in software development.