Understanding the Difference Between Git Merge and Rebase
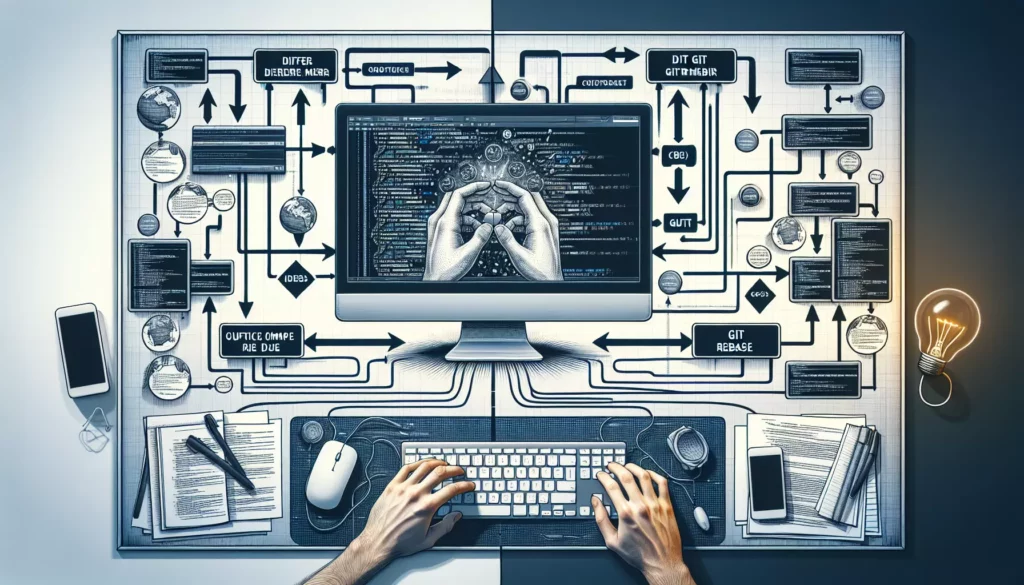
In the world of version control and collaborative software development, Git stands out as an indispensable tool. Two of its most powerful features, merge and rebase, are fundamental to managing branches and integrating changes. However, understanding when and how to use each can be a challenge for many developers. In this comprehensive guide, we’ll dive deep into the intricacies of Git merge and rebase, exploring their differences, use cases, and best practices.
What is Git Merge?
Git merge is a command used to combine two branches. When you merge one branch into another, Git creates a new commit that combines the changes from both branches. This new commit, often called a “merge commit,” has two parent commits – one from each of the merged branches.
How Git Merge Works
Let’s break down the merge process step by step:
- You start on your current branch (let’s call it ‘main’).
- You run the command
git merge feature-branch
. - Git identifies the common ancestor commit of ‘main’ and ‘feature-branch’.
- Git applies all the changes made in ‘feature-branch’ since the common ancestor to ‘main’.
- If there are no conflicts, Git automatically creates a new merge commit.
- If there are conflicts, Git pauses the merge process and asks you to resolve them manually.
Example of Git Merge
Here’s a simple example of how to perform a merge:
git checkout main
git merge feature-branch
This will merge the ‘feature-branch’ into ‘main’.
What is Git Rebase?
Git rebase is an alternative to merging. Instead of creating a new commit to combine branches, rebase moves or “replays” the commits from one branch onto another. This results in a linear history, as if all the work was done sequentially, even if it was actually done in parallel.
How Git Rebase Works
The rebase process can be broken down as follows:
- You start on your feature branch.
- You run the command
git rebase main
. - Git identifies the common ancestor commit of your feature branch and ‘main’.
- Git temporarily removes all commits on your feature branch that came after the common ancestor.
- Git applies all new commits from ‘main’ to your feature branch.
- Git then reapplies your temporary commits one by one on top of the updated branch.
Example of Git Rebase
Here’s how you would typically perform a rebase:
git checkout feature-branch
git rebase main
This will rebase ‘feature-branch’ onto ‘main’.
Key Differences Between Merge and Rebase
Now that we understand how both merge and rebase work, let’s explore their key differences:
1. History Preservation
Merge: Preserves the entire history of your project, including all parallel development work. The resulting history shows exactly when and how merges occurred.
Rebase: Creates a linear history by moving commits. This can make the project history cleaner and easier to follow, but it doesn’t preserve the context of when parallel work was done.
2. Commit Hashes
Merge: Doesn’t change existing commits. All commit hashes remain the same, and a new merge commit is created.
Rebase: Creates new commits with new hashes for all commits that are rebased. This is because each commit is being “replayed” on top of a new base.
3. Conflict Resolution
Merge: Conflicts are resolved in a single merge commit. You deal with all conflicts at once.
Rebase: Conflicts are resolved for each commit as it’s being replayed. This can mean resolving the same conflict multiple times if several commits modified the same part of a file.
4. Workflow Impact
Merge: Generally safer for public branches as it doesn’t rewrite history. It’s often preferred for long-running branches.
Rebase: Better for cleaning up your local work before sharing. It’s often used for short-lived feature branches or when you want to clean up your commits before merging.
When to Use Merge vs Rebase
Choosing between merge and rebase depends on your specific situation and team workflow. Here are some guidelines:
Use Merge When:
- You want to preserve the exact history of your branch.
- You’re working on a public branch that others are using as a base.
- You want to create an explicit merge commit to signify the integration of a feature.
- You’re pulling changes from a remote branch into your local branch.
Use Rebase When:
- You want to maintain a clean, linear project history.
- You’re working on a private feature branch that only you use.
- You want to clean up your local commits before sharing your work.
- You want to integrate the latest changes from the main branch into your feature branch.
Best Practices for Using Merge and Rebase
To make the most of these powerful Git features, consider the following best practices:
For Merge:
- Use descriptive commit messages: When creating a merge commit, write a clear message that explains what’s being merged and why.
- Resolve conflicts carefully: Take the time to understand and correctly resolve any conflicts that arise during a merge.
- Use feature branches: Develop new features in separate branches and merge them back to the main branch when complete.
- Regularly merge from the main branch: Keep your feature branches up-to-date by regularly merging changes from the main branch.
For Rebase:
- Don’t rebase public branches: Avoid rebasing branches that other developers are working on to prevent history conflicts.
- Use interactive rebase to clean up commits: Before pushing your changes, use
git rebase -i
to squash, edit, or reorder your commits. - Understand the Golden Rule of Rebasing: Never rebase commits that have been pushed to a public repository.
- Communicate with your team: If you’re using rebase in a team setting, make sure everyone understands and agrees to the workflow.
Advanced Techniques: Cherry-Picking and Squashing
While merge and rebase are the primary tools for integrating changes, Git offers additional techniques for more specific scenarios:
Cherry-Picking
Cherry-picking allows you to apply a specific commit from one branch to another. This can be useful when you want to apply a particular fix or feature without merging an entire branch.
To cherry-pick a commit:
git cherry-pick <commit-hash>
This will apply the changes from the specified commit to your current branch.
Squashing Commits
Squashing is the process of combining multiple commits into one. This is often done to clean up a branch’s history before merging it into the main branch.
You can squash commits using an interactive rebase:
git rebase -i HEAD~3
This opens an interactive rebase for the last 3 commits. You can then mark commits to be squashed.
Handling Conflicts
Both merge and rebase can lead to conflicts when Git can’t automatically reconcile differences between branches. Here’s how to handle conflicts in each case:
Resolving Merge Conflicts
- When a conflict occurs, Git will pause the merge process.
- Open the conflicting files and look for conflict markers (<<<<<<<, =======, >>>>>>>).
- Manually edit the files to resolve the conflicts.
- Use
git add
to mark the resolved files. - Complete the merge with
git merge --continue
.
Resolving Rebase Conflicts
- During a rebase, Git will pause when it encounters a conflict.
- Resolve conflicts in the same way as with merges.
- Use
git add
to mark resolved files. - Continue the rebase with
git rebase --continue
. - Repeat for each commit being rebased if necessary.
Git Workflows: Integrating Merge and Rebase
Different teams and projects may adopt various Git workflows that incorporate both merge and rebase. Here are two popular workflows:
GitHub Flow
This simple workflow is centered around the main branch:
- Create a feature branch from main.
- Make changes and commit to the feature branch.
- Open a pull request to propose changes to main.
- Discuss and review the code in the pull request.
- Deploy and test the changes.
- Merge the feature branch into main.
In this workflow, merges are typically used to integrate feature branches back into main.
Git Flow
This more complex workflow uses multiple branches:
- main: Represents the official release history.
- develop: Serves as an integration branch for features.
- feature branches: Used to develop new features.
- release branches: Prepare for a new production release.
- hotfix branches: Used to quickly patch production releases.
In Git Flow, both merge and rebase can be used. Rebase might be used to keep feature branches up-to-date with develop, while merges are used to integrate completed features.
Tools and Visualizations
Understanding and managing complex Git histories can be challenging. Fortunately, there are several tools available to help visualize and navigate Git branches:
Command Line Tools
git log --graph --oneline --all
: Provides a text-based visualization of your Git history.git reflog
: Shows a log of all reference updates in your repository, useful for recovering lost commits after a rebase.
GUI Tools
- GitKraken: A powerful Git GUI that provides clear visualizations of branches and commits.
- SourceTree: Another popular Git GUI that offers intuitive branch management and visualization.
- Git Extensions: An open-source Git GUI that integrates well with Windows.
IDE Integrations
Many Integrated Development Environments (IDEs) offer built-in Git visualization and management tools:
- Visual Studio Code: Offers a Git sidebar and built-in terminal for Git operations.
- IntelliJ IDEA: Provides comprehensive Git integration with visual diff tools and branch management.
- Eclipse: Offers Git integration through the EGit plugin.
Common Pitfalls and How to Avoid Them
While Git merge and rebase are powerful tools, they can lead to issues if not used correctly. Here are some common pitfalls and how to avoid them:
1. Rebasing Public Branches
Pitfall: Rebasing branches that have been pushed to a public repository can cause conflicts for other developers.
Solution: Only rebase local, unpublished branches. If you need to update a public branch, use merge instead.
2. Losing Commits During Rebase
Pitfall: Improperly executed rebases can result in lost commits.
Solution: Always create a backup branch before rebasing. Use git reflog
to recover lost commits if necessary.
3. Merge Conflicts Overload
Pitfall: Letting branches diverge for too long can lead to numerous, complex merge conflicts.
Solution: Regularly integrate changes from the main branch into your feature branches to minimize conflicts.
4. Incorrect Conflict Resolution
Pitfall: Hastily resolving conflicts without fully understanding the changes can introduce bugs.
Solution: Take time to understand each conflict. Consult with team members if necessary. Use tools like git diff
to understand changes clearly.
Conclusion
Understanding the difference between Git merge and rebase is crucial for effective version control and collaboration in software development. While merge preserves the full history of your project and is safer for public branches, rebase offers a cleaner, linear history that can be beneficial for private or feature branches.
Remember, there’s no one-size-fits-all solution. The choice between merge and rebase often depends on your specific workflow, team preferences, and the nature of your project. By understanding the strengths and weaknesses of each approach, you can make informed decisions that enhance your development process.
As you continue to work with Git, experiment with both merge and rebase in different scenarios. Practice resolving conflicts, visualizing your Git history, and communicating your Git strategy with your team. With time and experience, you’ll develop an intuition for when to use each technique, allowing you to maintain a clean, understandable project history while collaborating effectively with others.
Remember, the goal of version control is not just to track changes, but to facilitate collaboration and maintain the integrity of your project. Whether you choose to merge or rebase, always prioritize clear communication, careful conflict resolution, and a thorough understanding of your project’s history. Happy coding!