Common Git Mistakes and How to Avoid Them
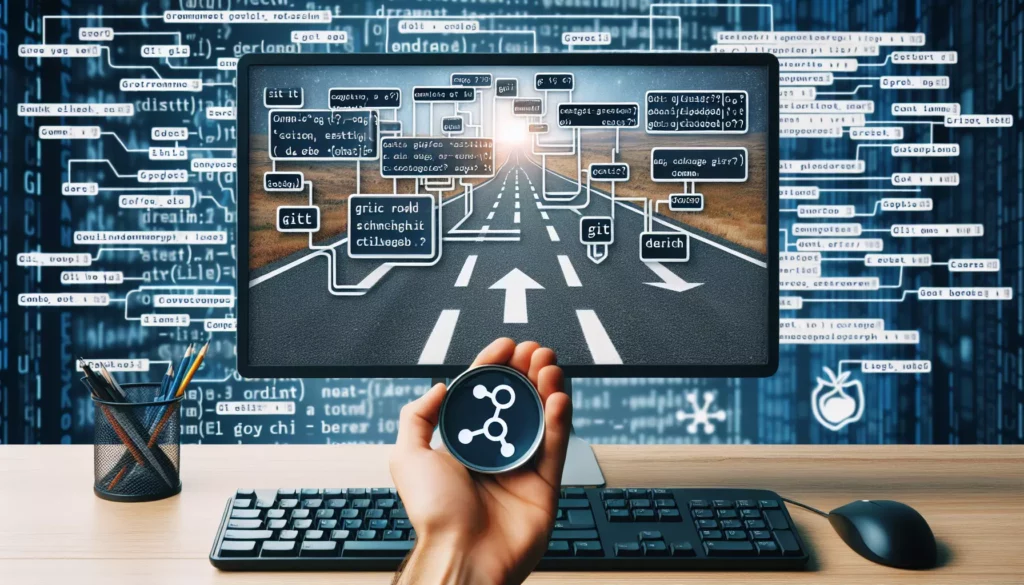
Git is an essential tool for developers, but it can be tricky to master. Even experienced programmers occasionally stumble when using Git. In this comprehensive guide, we’ll explore common Git mistakes and provide practical solutions to avoid them. Whether you’re a beginner or an advanced user preparing for technical interviews at major tech companies, understanding these pitfalls will help you become a more proficient Git user.
1. Committing Large Files
One of the most common mistakes in Git is committing large files, which can bloat your repository and slow down cloning and fetching operations.
The Problem:
Git is designed for tracking changes in source code, not for managing large binary files or datasets. When you commit large files, they become part of your repository’s history, making it difficult to remove them later.
How to Avoid:
- Use .gitignore: Create a .gitignore file in your repository’s root directory and list patterns for files you want Git to ignore.
- Use Git LFS (Large File Storage): For large files that need version control, consider using Git LFS, which stores large files separately from your main repository.
- Be mindful of file sizes: Before committing, review the files you’re about to add and consider if they really need to be in version control.
Example .gitignore:
# Ignore all .log files
*.log
# Ignore the build directory
/build/
# Ignore all files in the temp directory
/temp/*
# Ignore .env files
.env
2. Committing Sensitive Information
Accidentally committing sensitive information like API keys, passwords, or private configuration files is a serious security risk.
The Problem:
Once sensitive information is committed and pushed to a remote repository, it becomes part of the Git history. Even if you delete the file later, the information remains in the repository’s history.
How to Avoid:
- Use environment variables: Store sensitive information in environment variables instead of hardcoding them in your files.
- Use .gitignore: Add patterns to your .gitignore file to exclude sensitive files from version control.
- Use git-secrets: Implement git-secrets to prevent committing secrets and credentials into Git repositories.
- Review changes before committing: Always use
git diff
orgit status
to review changes before committing.
Example: Using environment variables in Python
import os
# Instead of:
# api_key = "your_secret_api_key"
# Use:
api_key = os.environ.get("API_KEY")
3. Not Using Branches Properly
Failing to use branches effectively can lead to messy code integration and make it difficult to manage different features or versions of your project.
The Problem:
Working directly on the main branch or creating too many long-lived branches can lead to conflicts, make it harder to review code, and complicate the release process.
How to Avoid:
- Use feature branches: Create a new branch for each feature or bug fix you’re working on.
- Keep branches short-lived: Merge feature branches back into the main branch as soon as the work is complete and reviewed.
- Use a branching strategy: Implement a branching strategy like GitFlow or GitHub Flow to standardize your development process.
- Regularly sync with the main branch: Frequently merge or rebase your feature branch with the main branch to minimize conflicts.
Example: Creating and switching to a feature branch
# Create and switch to a new feature branch
git checkout -b feature/new-login-page
# Make your changes and commit them
git add .
git commit -m "Implement new login page design"
# Push the branch to the remote repository
git push -u origin feature/new-login-page
4. Ignoring Merge Conflicts
Merge conflicts are an inevitable part of collaborative development, but ignoring them or resolving them incorrectly can lead to code loss or introduce bugs.
The Problem:
When multiple developers work on the same files, Git may not be able to automatically merge changes. Ignoring these conflicts or resolving them hastily can result in lost work or introduce errors into your codebase.
How to Avoid:
- Communicate with your team: Coordinate with team members to minimize conflicts on the same files.
- Resolve conflicts promptly: Address merge conflicts as soon as they occur, rather than letting them accumulate.
- Use visual merge tools: Utilize tools like GitKraken, SourceTree, or VS Code’s built-in merge conflict resolver to visualize and resolve conflicts more easily.
- Test after resolving: Always test your code after resolving merge conflicts to ensure the merged code works as expected.
Example: Resolving a merge conflict
# Attempt to merge changes from the main branch
git merge main
# If conflicts occur, you'll see a message like:
# CONFLICT (content): Merge conflict in file.txt
# Automatic merge failed; fix conflicts and then commit the result.
# Open the conflicting file and look for conflict markers:
# <<<<<<< HEAD
# Your changes
# =======
# Changes from the branch you're merging
# >>>>>>> main
# Manually edit the file to resolve the conflict
# Remove the conflict markers and keep the desired code
# After resolving, stage the changes
git add file.txt
# Complete the merge by committing
git commit -m "Resolve merge conflict in file.txt"
5. Overusing Force Push
Force pushing (git push --force
) can be a powerful tool, but it’s often misused, leading to lost commits and collaboration difficulties.
The Problem:
Force pushing overwrites the remote branch with your local branch, potentially erasing commits made by other team members. This can lead to lost work and confusion among collaborators.
How to Avoid:
- Use force push sparingly: Only use force push when absolutely necessary, such as when rebasing a feature branch.
- Communicate with your team: If you must force push, inform your team members so they can update their local repositories accordingly.
- Use –force-with-lease: Instead of
--force
, use--force-with-lease
to ensure you don’t overwrite others’ work unintentionally. - Avoid force pushing to shared branches: Never force push to branches that other team members are actively working on, especially the main branch.
Example: Using force-with-lease
# Instead of:
# git push --force origin feature-branch
# Use:
git push --force-with-lease origin feature-branch
6. Not Using .gitignore Effectively
Failing to set up or maintain a proper .gitignore file can lead to unnecessary files being tracked, bloating your repository, and potentially exposing sensitive information.
The Problem:
Without a well-configured .gitignore file, you may accidentally commit files that shouldn’t be in version control, such as build artifacts, temporary files, or local configuration files.
How to Avoid:
- Create a .gitignore file: Start your project with a .gitignore file tailored to your development environment and project type.
- Use templates: Utilize pre-made .gitignore templates for your specific language or framework from resources like gitignore.io.
- Regularly update .gitignore: As your project evolves, update your .gitignore file to include new patterns for files that shouldn’t be tracked.
- Use global .gitignore: Set up a global .gitignore file for patterns that apply to all your projects, such as OS-specific files or editor configurations.
Example: Creating and using a .gitignore file
# Create a .gitignore file
touch .gitignore
# Add patterns to ignore
echo "*.log" >> .gitignore
echo "node_modules/" >> .gitignore
echo ".env" >> .gitignore
# If you've already committed files that should be ignored
git rm --cached file_to_ignore.log
git commit -m "Remove ignored file"
7. Not Understanding Git’s Three Trees
Many Git users struggle because they don’t fully understand Git’s three-tree architecture: the working directory, the staging area (index), and the commit history.
The Problem:
Misunderstanding how changes move between these three “trees” can lead to confusion about which changes are being tracked, staged, or committed.
How to Avoid:
- Learn the basics: Take time to understand Git’s architecture and how changes flow through the three trees.
- Use git status frequently: Regularly check the status of your working directory and staging area.
- Understand staging: Learn how to use the staging area effectively to prepare your commits.
- Practice with interactive staging: Use
git add -i
orgit add -p
to stage changes more granularly.
Example: Moving changes through Git’s three trees
# Make changes in your working directory
echo "New content" > file.txt
# Check the status
git status
# Stage the changes (move to the index)
git add file.txt
# Check status again
git status
# Commit the changes (move to the commit history)
git commit -m "Add new content to file.txt"
# Verify the commit
git log -1
8. Committing Without a Clear Message
Writing unclear, vague, or uninformative commit messages makes it difficult to understand the history of your project and can hinder collaboration.
The Problem:
Poor commit messages make it challenging to review code changes, understand the project’s evolution, and identify the purpose of specific commits when needed.
How to Avoid:
- Use a consistent format: Adopt a standard format for your commit messages, such as the conventional commits specification.
- Be descriptive: Clearly explain what changes were made and why.
- Keep it concise: Aim for a short (50 characters or less) summary line, followed by a more detailed explanation if necessary.
- Use imperative mood: Write commit messages as if you’re giving commands (e.g., “Fix bug” instead of “Fixed bug”).
Example: Writing good commit messages
# Bad commit message
git commit -m "Fixed stuff"
# Good commit message
git commit -m "Fix login page validation bug
- Add email format validation
- Improve error messaging for invalid inputs
- Update unit tests for new validation logic"
9. Not Using Git Hooks
Git hooks are powerful scripts that can automate tasks and enforce standards in your development workflow. Not utilizing them means missing out on opportunities to improve code quality and consistency.
The Problem:
Without Git hooks, you may forget to run tests, linters, or formatting tools before committing or pushing code, leading to inconsistent code quality or broken builds.
How to Avoid:
- Implement pre-commit hooks: Use pre-commit hooks to run linters, formatters, and tests before each commit.
- Use pre-push hooks: Implement pre-push hooks to run more comprehensive tests or checks before pushing to a remote repository.
- Utilize commit-msg hooks: Use commit-msg hooks to enforce commit message standards.
- Share hooks with your team: Use tools like Husky to share Git hooks configurations with your team through version control.
Example: Setting up a pre-commit hook
# Create a pre-commit hook file
touch .git/hooks/pre-commit
chmod +x .git/hooks/pre-commit
# Add content to the pre-commit hook
echo "#!/bin/sh
npm run lint
npm test" > .git/hooks/pre-commit
10. Not Understanding Git Rebase
Git rebase is a powerful tool for maintaining a clean project history, but misunderstanding or misusing it can lead to confusion and lost commits.
The Problem:
Improper use of rebase can rewrite project history, potentially causing conflicts with collaborators and making it difficult to track changes over time.
How to Avoid:
- Learn rebase thoroughly: Take the time to understand how rebase works and when it’s appropriate to use it.
- Use rebase on local branches: Primarily use rebase for cleaning up your local feature branches before merging.
- Avoid rebasing shared branches: Never rebase branches that have been pushed and are being used by others.
- Communicate with your team: If you need to rebase a shared branch, ensure all team members are aware and know how to handle the changes.
Example: Using rebase to update a feature branch
# Switch to your feature branch
git checkout feature-branch
# Fetch the latest changes from the remote
git fetch origin
# Rebase your branch on top of the latest main branch
git rebase origin/main
# If conflicts occur, resolve them and continue the rebase
git add .
git rebase --continue
# Force push the updated branch (only if it's a personal branch)
git push --force-with-lease origin feature-branch
Conclusion
Understanding and avoiding these common Git mistakes will significantly improve your development workflow and collaboration skills. As you prepare for technical interviews or work on complex projects, remember that mastering Git is an ongoing process. Regular practice, continuous learning, and staying up-to-date with Git best practices will help you become a more proficient developer.
At AlgoCademy, we emphasize the importance of not just coding skills, but also the tools and practices that support efficient software development. Git is an essential part of this toolkit. By avoiding these common pitfalls and adopting best practices, you’ll be better prepared for the challenges of professional software development, whether you’re aiming for a position at a major tech company or working on your own projects.
Remember, the key to avoiding Git mistakes is to understand Git’s underlying concepts, communicate effectively with your team, and always approach version control with care and attention to detail. Happy coding, and may your repositories always be clean and conflict-free!