How to Use GitLab for Version Control and CI/CD
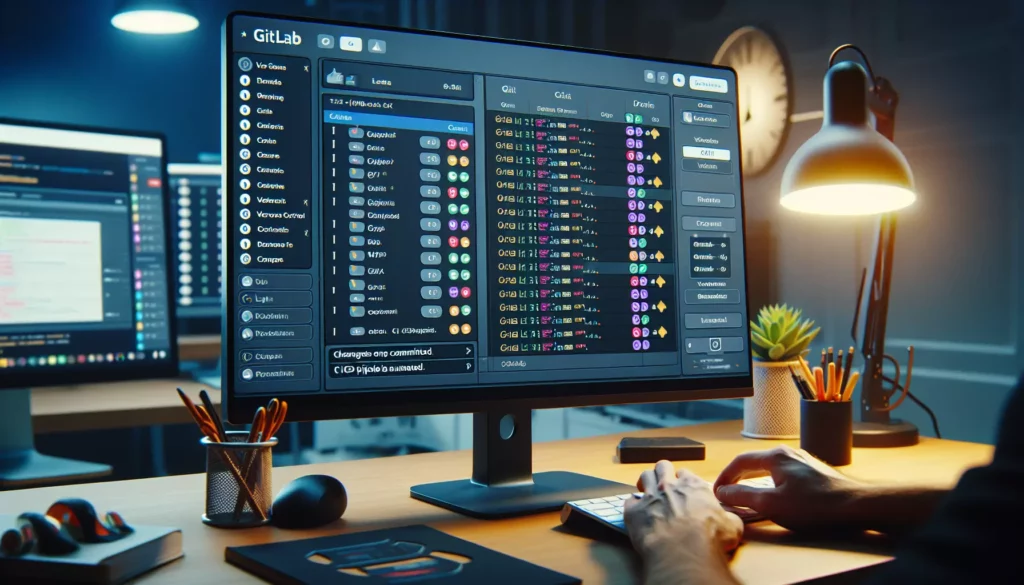
In the world of software development, efficient version control and streamlined deployment processes are crucial for success. GitLab, a web-based DevOps lifecycle tool, offers a comprehensive solution for both version control and Continuous Integration/Continuous Deployment (CI/CD). In this guide, we’ll explore how to leverage GitLab’s powerful features to enhance your development workflow, from managing your code to automating your deployment pipeline.
Table of Contents
- Introduction to GitLab
- Using GitLab for Version Control
- Implementing CI/CD with GitLab
- Best Practices for GitLab Usage
- Advanced GitLab Features
- Conclusion
1. Introduction to GitLab
GitLab is an open-source platform that provides a Git-repository manager with wiki, issue-tracking, and CI/CD pipeline features, using an open-source license. It offers a complete DevOps platform, enabling software development teams to collaborate effectively, manage code, and automate their software delivery process.
Key Features of GitLab:
- Git repository management
- Issue tracking and project management
- Continuous Integration and Continuous Deployment
- Code review and collaboration tools
- Built-in container registry
- Kubernetes integration
- Security scanning and monitoring
GitLab can be self-hosted or used as a cloud-based service, making it flexible for various team sizes and project requirements.
2. Using GitLab for Version Control
Version control is at the heart of GitLab’s functionality. Here’s how to effectively use GitLab for managing your codebase:
Setting Up a GitLab Repository
- Log in to your GitLab account
- Click on “New project” or “Create a project”
- Choose “Create blank project”
- Fill in the project details (name, description, visibility)
- Click “Create project”
Cloning the Repository
To start working with your new repository, you’ll need to clone it to your local machine:
git clone https://gitlab.com/your-username/your-project.git
cd your-project
Basic Git Operations in GitLab
Here are some essential Git commands you’ll use frequently with GitLab:
- Add changes:
git add .
- Commit changes:
git commit -m "Your commit message"
- Push changes:
git push origin main
- Pull changes:
git pull origin main
- Create a new branch:
git checkout -b new-feature
- Switch branches:
git checkout branch-name
Merge Requests
GitLab’s Merge Requests (equivalent to GitHub’s Pull Requests) are a key feature for code review and collaboration:
- Push your feature branch to GitLab
- Go to the GitLab web interface
- Click on “Merge Requests” in the left sidebar
- Click “New merge request”
- Select your feature branch as the source and the main branch as the target
- Fill in the title and description
- Assign reviewers and add labels if needed
- Click “Submit merge request”
3. Implementing CI/CD with GitLab
GitLab’s integrated CI/CD capabilities allow you to automate your build, test, and deployment processes. Here’s how to set up a basic CI/CD pipeline:
Creating a .gitlab-ci.yml File
The .gitlab-ci.yml
file is the heart of GitLab CI/CD. Create this file in your project’s root directory:
stages:
- build
- test
- deploy
build-job:
stage: build
script:
- echo "Building the project..."
- npm install
- npm run build
test-job:
stage: test
script:
- echo "Running tests..."
- npm run test
deploy-job:
stage: deploy
script:
- echo "Deploying application..."
- npm run deploy
only:
- main
This basic configuration defines three stages: build, test, and deploy. Each stage has a job with specific scripts to run.
Understanding GitLab Runners
GitLab Runners are agents that run your CI/CD jobs. You can use shared runners provided by GitLab or set up your own:
- Go to your project’s “Settings” > “CI/CD” > “Runners”
- Choose between shared runners or specific runners
- If using specific runners, follow GitLab’s instructions to install and register a runner
Configuring Environments
GitLab allows you to define different environments for your deployments:
deploy-staging:
stage: deploy
script:
- echo "Deploying to staging..."
environment:
name: staging
url: https://staging.example.com
only:
- develop
deploy-production:
stage: deploy
script:
- echo "Deploying to production..."
environment:
name: production
url: https://example.com
only:
- main
Monitoring CI/CD Pipelines
To monitor your CI/CD pipelines:
- Go to your project’s “CI/CD” > “Pipelines”
- View the status of current and past pipelines
- Click on a pipeline to see detailed job information
- Check job logs for debugging if a job fails
4. Best Practices for GitLab Usage
To make the most of GitLab, consider these best practices:
Branching Strategy
Implement a clear branching strategy, such as GitFlow or GitHub Flow:
- Use feature branches for new features or bug fixes
- Keep the main branch stable and deployable
- Use release branches for preparing new releases
Commit Messages
Write clear, descriptive commit messages:
feat: add user authentication feature
- Implement JWT-based authentication
- Add login and registration endpoints
- Create middleware for protected routes
Code Review
Encourage thorough code reviews:
- Use merge request templates to standardize information
- Set up merge request approval rules
- Use GitLab’s code quality tools and integrations
CI/CD Best Practices
- Keep your CI/CD pipelines fast and efficient
- Use caching to speed up builds
- Implement parallel jobs where possible
- Use environment variables for sensitive information
5. Advanced GitLab Features
GitLab offers several advanced features to enhance your development workflow:
GitLab Pages
Host static websites directly from your GitLab repository:
pages:
stage: deploy
script:
- mkdir .public
- cp -r * .public
- mv .public public
artifacts:
paths:
- public
only:
- main
GitLab Container Registry
Store and manage Docker images within GitLab:
build-docker:
stage: build
image: docker:latest
services:
- docker:dind
script:
- docker build -t my-app .
- docker push registry.gitlab.com/my-group/my-project/my-app:latest
GitLab Security Scanning
Integrate security scanning into your CI/CD pipeline:
include:
- template: Security/SAST.gitlab-ci.yml
- template: Security/Dependency-Scanning.gitlab-ci.yml
- template: Security/Container-Scanning.gitlab-ci.yml
GitLab Auto DevOps
Enable Auto DevOps to automatically set up a comprehensive CI/CD pipeline:
- Go to your project’s “Settings” > “CI/CD” > “Auto DevOps”
- Enable Auto DevOps
- Configure your Kubernetes cluster if needed
6. Conclusion
GitLab provides a powerful, all-in-one solution for version control and CI/CD, enabling development teams to streamline their workflows and improve collaboration. By leveraging GitLab’s features for code management, automated testing, and deployment, you can significantly enhance your software development process.
As you become more comfortable with GitLab, explore its advanced features to further optimize your development pipeline. Remember that effective use of GitLab, like any tool, requires consistent practice and a willingness to adapt to new methodologies.
Whether you’re a solo developer or part of a large team, GitLab’s flexibility and comprehensive feature set make it an excellent choice for managing your projects from inception to deployment. By following the practices outlined in this guide, you’ll be well on your way to leveraging GitLab’s full potential in your development workflow.